mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
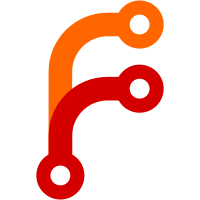
Type canonicalization is deferred between in may involve cycles. Instances are canonicalized eagerly, but their type arguments are not necessarily canonical yet, which can lead to incorrect canonicalization of the instance. The work around is to include the most popular type arguments in the serializer's set of base objects, bypassing deferred canonicalization. Other type arguments will continue to fail. Bug: https://github.com/dart-lang/sdk/issues/33430 Change-Id: Ia992b3ebc2974b54acb5c88b3e1d836f6ec1f1b8 Reviewed-on: https://dart-review.googlesource.com/61721 Commit-Queue: Ryan Macnak <rmacnak@google.com> Reviewed-by: Siva Annamalai <asiva@google.com>
77 lines
1.9 KiB
C++
77 lines
1.9 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_SNAPSHOT_IDS_H_
|
|
#define RUNTIME_VM_SNAPSHOT_IDS_H_
|
|
|
|
#include "vm/dart_entry.h"
|
|
#include "vm/raw_object.h"
|
|
|
|
namespace dart {
|
|
|
|
// Index for predefined singleton objects used in a snapshot.
|
|
enum {
|
|
kNullObject = 0,
|
|
kSentinelObject,
|
|
kTransitionSentinelObject,
|
|
kEmptyArrayObject,
|
|
kZeroArrayObject,
|
|
kTrueValue,
|
|
kFalseValue,
|
|
// Marker for special encoding of double objects in message snapshots.
|
|
kDoubleObject,
|
|
// Object id has been optimized away; reader should use next available id.
|
|
kOmittedObjectId,
|
|
|
|
kClassIdsOffset = kOmittedObjectId,
|
|
|
|
// The class ids of predefined classes are included in this list
|
|
// at an offset of kClassIdsOffset.
|
|
|
|
kObjectType = (kNumPredefinedCids + kClassIdsOffset),
|
|
kNullType,
|
|
kDynamicType,
|
|
kVoidType,
|
|
kFunctionType,
|
|
kNumberType,
|
|
kSmiType,
|
|
kMintType,
|
|
kDoubleType,
|
|
kIntType,
|
|
kBoolType,
|
|
kStringType,
|
|
kArrayType,
|
|
kIntTypeArguments,
|
|
kDoubleTypeArguments,
|
|
kStringTypeArguments,
|
|
kStringDynamicTypeArguments,
|
|
kStringStringTypeArguments,
|
|
kEmptyTypeArguments,
|
|
|
|
kExtractorParameterTypes,
|
|
kExtractorParameterNames,
|
|
kEmptyContextScopeObject,
|
|
kImplicitClosureScopeObject,
|
|
kEmptyObjectPool,
|
|
kEmptyDescriptors,
|
|
kEmptyVarDescriptors,
|
|
kEmptyExceptionHandlers,
|
|
kCachedArgumentsDescriptor0,
|
|
kCachedArgumentsDescriptorN =
|
|
(kCachedArgumentsDescriptor0 +
|
|
ArgumentsDescriptor::kCachedDescriptorCount - 1),
|
|
kCachedICDataArray0,
|
|
kCachedICDataArrayN =
|
|
(kCachedICDataArray0 + ICData::kCachedICDataArrayCount - 1),
|
|
|
|
kInstanceObjectId,
|
|
kStaticImplicitClosureObjectId,
|
|
kMaxPredefinedObjectIds,
|
|
kInvalidIndex = -1,
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_SNAPSHOT_IDS_H_
|