mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
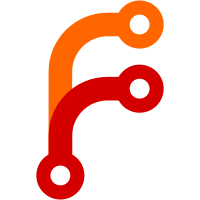
This reverts commit d79787771e
.
Reason for revert:
This caused significant performance regressions on our protobuf_decode* benchmarks (probably on more benchmarks, but we don't yet have results due to some perf infra issue).
To avoid letting the regressions land for downstream users we'll revert it.
We should measure performance impact before landing this again.
Original change's description:
> [vm] Repair the resolver abstraction, take 2.
>
> - Move resolution logic that accumulated in the runtime entries into Resolver.
> - Improve NoSuchMethodError for closures when --no-lazy-dispatchers
> - Fix concurrent modification of Null class by nullability propagation.
>
> Change-Id: Ib05b431a289d847785032dda46e1bbcb524b7343
> Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/98428
> Commit-Queue: Ryan Macnak <rmacnak@google.com>
> Reviewed-by: Régis Crelier <regis@google.com>
TBR=rmacnak@google.com,alexmarkov@google.com,regis@google.com
Change-Id: I4f0f0a0d7c6018fc5968aafdce30f6d2e7495059
No-Presubmit: true
No-Tree-Checks: true
No-Try: true
Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/99140
Reviewed-by: Martin Kustermann <kustermann@google.com>
76 lines
3.1 KiB
C++
76 lines
3.1 KiB
C++
// Copyright (c) 2011, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_RESOLVER_H_
|
|
#define RUNTIME_VM_RESOLVER_H_
|
|
|
|
#include "vm/allocation.h"
|
|
|
|
namespace dart {
|
|
|
|
// Forward declarations.
|
|
class Array;
|
|
class Class;
|
|
class Instance;
|
|
class Library;
|
|
class RawFunction;
|
|
class String;
|
|
class ArgumentsDescriptor;
|
|
|
|
// Resolver abstracts functionality needed to resolve dart functions at
|
|
// invocations.
|
|
class Resolver : public AllStatic {
|
|
public:
|
|
// Resolve specified dart instance function.
|
|
static RawFunction* ResolveDynamic(const Instance& receiver,
|
|
const String& function_name,
|
|
const ArgumentsDescriptor& args_desc);
|
|
|
|
// If 'allow_add' is true we may add a function to the class during lookup.
|
|
static RawFunction* ResolveDynamicForReceiverClass(
|
|
const Class& receiver_class,
|
|
const String& function_name,
|
|
const ArgumentsDescriptor& args_desc,
|
|
bool allow_add = true);
|
|
|
|
// If 'allow_add' is true we may add a function to the class during lookup.
|
|
static RawFunction* ResolveDynamicAnyArgs(Zone* zone,
|
|
const Class& receiver_class,
|
|
const String& function_name,
|
|
bool allow_add = true);
|
|
|
|
// Resolve specified dart static function. If library.IsNull, use
|
|
// either application library or core library if no application library
|
|
// exists. Passing negative num_arguments means that the function
|
|
// will be resolved by name only.
|
|
// Otherwise null is returned if the number or names of arguments are not
|
|
// valid for the resolved function.
|
|
static RawFunction* ResolveStatic(const Library& library,
|
|
const String& cls_name,
|
|
const String& function_name,
|
|
intptr_t type_args_len,
|
|
intptr_t num_arguments,
|
|
const Array& argument_names);
|
|
|
|
// Resolve specified dart static function with specified arity. Only resolves
|
|
// public functions.
|
|
static RawFunction* ResolveStatic(const Class& cls,
|
|
const String& function_name,
|
|
intptr_t type_args_len,
|
|
intptr_t num_arguments,
|
|
const Array& argument_names);
|
|
|
|
// Resolve specified dart static function with specified arity. Resolves both
|
|
// public and private functions.
|
|
static RawFunction* ResolveStaticAllowPrivate(const Class& cls,
|
|
const String& function_name,
|
|
intptr_t type_args_len,
|
|
intptr_t num_arguments,
|
|
const Array& argument_names);
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_RESOLVER_H_
|