mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
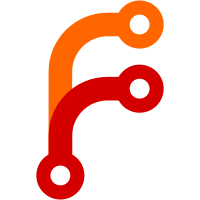
In Dart2, type bounds checking is either performed by the common front-end or by explicitly generated code, but not by type finalization or runtime anymore, as it was done in Dart1. Consequently, the class BoundedType is not needed anymore, and malbounded or malformed types are not seen by the runtime either. Change-Id: I5d6e4c68d153d6730fa7ff7f6d9dcfa611299c16 Reviewed-on: https://dart-review.googlesource.com/c/86687 Commit-Queue: Régis Crelier <regis@google.com> Reviewed-by: Ryan Macnak <rmacnak@google.com> Reviewed-by: Samir Jindel <sjindel@google.com>
76 lines
2.5 KiB
C++
76 lines
2.5 KiB
C++
// Copyright (c) 2014, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_REPORT_H_
|
|
#define RUNTIME_VM_REPORT_H_
|
|
|
|
#include "vm/allocation.h"
|
|
#include "vm/token_position.h"
|
|
|
|
namespace dart {
|
|
|
|
// Forward declarations.
|
|
class Error;
|
|
class ICData;
|
|
class RawString;
|
|
class Script;
|
|
class StackFrame;
|
|
class String;
|
|
|
|
class Report : AllStatic {
|
|
public:
|
|
enum Kind {
|
|
kWarning,
|
|
kError,
|
|
kBailout,
|
|
};
|
|
|
|
static const bool AtLocation = false;
|
|
static const bool AfterLocation = true;
|
|
|
|
// Report an already formatted error via a long jump.
|
|
DART_NORETURN static void LongJump(const Error& error);
|
|
|
|
// Concatenate and report an already formatted error and a new error message.
|
|
DART_NORETURN static void LongJumpF(const Error& prev_error,
|
|
const Script& script,
|
|
TokenPosition token_pos,
|
|
const char* format,
|
|
...) PRINTF_ATTRIBUTE(4, 5);
|
|
DART_NORETURN static void LongJumpV(const Error& prev_error,
|
|
const Script& script,
|
|
TokenPosition token_pos,
|
|
const char* format,
|
|
va_list args);
|
|
|
|
// Report a warning/jswarning/error/bailout message.
|
|
static void MessageF(Kind kind,
|
|
const Script& script,
|
|
TokenPosition token_pos,
|
|
bool report_after_token,
|
|
const char* format,
|
|
...) PRINTF_ATTRIBUTE(5, 6);
|
|
static void MessageV(Kind kind,
|
|
const Script& script,
|
|
TokenPosition token_pos,
|
|
bool report_after_token,
|
|
const char* format,
|
|
va_list args);
|
|
|
|
// Prepend a source snippet to the message.
|
|
// A null script means no source and a negative token_pos means no position.
|
|
static RawString* PrependSnippet(Kind kind,
|
|
const Script& script,
|
|
TokenPosition token_pos,
|
|
bool report_after_token,
|
|
const String& message);
|
|
|
|
private:
|
|
DISALLOW_COPY_AND_ASSIGN(Report);
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_REPORT_H_
|