mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
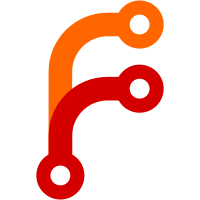
While adding support for new compact bytecode instructions, VM also keeps support for old bytecode instructions to preserve backwards compatibility and allow soft transition. This change is separate from bytecode generator changes in order to test VM with old bytecode generator. Corresponding bytecode generator changes: https://dart-review.googlesource.com/c/sdk/+/99400 Change-Id: Icf5ceee7d51f27ffe3f79d0eae81e0ddc0a7e855 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/101062 Reviewed-by: Ryan Macnak <rmacnak@google.com>
48 lines
1.5 KiB
C++
48 lines
1.5 KiB
C++
// Copyright (c) 2018, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#include "vm/globals.h"
|
|
#if !defined(DART_PRECOMPILED_RUNTIME)
|
|
|
|
#include "vm/instructions.h"
|
|
#include "vm/instructions_kbc.h"
|
|
|
|
#include "vm/constants_kbc.h"
|
|
#include "vm/native_entry.h"
|
|
|
|
namespace dart {
|
|
|
|
RawTypedData* KBCNativeCallPattern::GetNativeEntryDataAt(
|
|
uword pc,
|
|
const Bytecode& bytecode) {
|
|
ASSERT(bytecode.ContainsInstructionAt(pc));
|
|
|
|
const KBCInstr* return_addr = reinterpret_cast<const KBCInstr*>(pc);
|
|
const KBCInstr* instr =
|
|
reinterpret_cast<const KBCInstr*>(bytecode.PayloadStart());
|
|
ASSERT(instr < return_addr);
|
|
while (!KernelBytecode::IsNativeCallOpcode(instr)) {
|
|
instr = KernelBytecode::Next(instr);
|
|
if (instr >= return_addr) {
|
|
FATAL1(
|
|
"Unable to find NativeCall bytecode instruction"
|
|
" corresponding to PC %" Px,
|
|
pc);
|
|
}
|
|
}
|
|
|
|
intptr_t native_entry_data_pool_index = KernelBytecode::DecodeD(instr);
|
|
const ObjectPool& obj_pool = ObjectPool::Handle(bytecode.object_pool());
|
|
TypedData& native_entry_data = TypedData::Handle();
|
|
native_entry_data ^= obj_pool.ObjectAt(native_entry_data_pool_index);
|
|
// Native calls to recognized functions should never be patched.
|
|
ASSERT(NativeEntryData(native_entry_data).kind() ==
|
|
MethodRecognizer::kUnknown);
|
|
return native_entry_data.raw();
|
|
}
|
|
|
|
} // namespace dart
|
|
|
|
#endif // !defined(DART_PRECOMPILED_RUNTIME)
|