mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
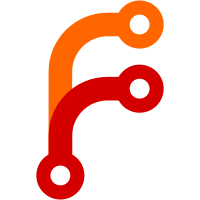
Threads in the native or blocked states don't prevent safepoints, so they may run concurrently with a safepoint operation like GC. It is not safe for handles to be allocated while the GC is visiting them, so these threads must not allocate handles. Assert only threads in the VM or generated states, which prevent safepoints until they check in, may allocate handles. (Generated code does not allocate handles, but leaf runtime entries remain in the generated state.) Bug: https://github.com/dart-lang/sdk/issues/34883 Change-Id: I1a211778f7ef96b53a2405f0ee9dde7871b122b6 Reviewed-on: https://dart-review.googlesource.com/c/81540 Commit-Queue: Ryan Macnak <rmacnak@google.com> Reviewed-by: Siva Annamalai <asiva@google.com> Reviewed-by: Vyacheslav Egorov <vegorov@google.com>
111 lines
2.6 KiB
C++
111 lines
2.6 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#include "vm/growable_array.h"
|
|
#include "platform/assert.h"
|
|
#include "vm/symbols.h"
|
|
#include "vm/unit_test.h"
|
|
|
|
namespace dart {
|
|
|
|
template <class GrowableArrayInt, class GrowableArrayInt64>
|
|
void TestGrowableArray() {
|
|
GrowableArrayInt g;
|
|
EXPECT_EQ(0, g.length());
|
|
EXPECT(g.is_empty());
|
|
g.Add(5);
|
|
EXPECT_EQ(5, g[0]);
|
|
EXPECT_EQ(1, g.length());
|
|
EXPECT(!g.is_empty());
|
|
g.Add(3);
|
|
const GrowableArrayInt& temp = g;
|
|
EXPECT_EQ(5, temp[0]);
|
|
EXPECT_EQ(3, temp[1]);
|
|
for (int i = 0; i < 10000; i++) {
|
|
g.Add(i);
|
|
}
|
|
EXPECT_EQ(10002, g.length());
|
|
EXPECT_EQ(10000 - 1, g.Last());
|
|
|
|
GrowableArrayInt64 f(10);
|
|
EXPECT_EQ(0, f.length());
|
|
f.Add(-1LL);
|
|
f.Add(15LL);
|
|
EXPECT_EQ(2, f.length());
|
|
for (int64_t l = 0; l < 100; l++) {
|
|
f.Add(l);
|
|
}
|
|
EXPECT_EQ(102, f.length());
|
|
EXPECT_EQ(100 - 1, f.Last());
|
|
EXPECT_EQ(-1LL, f[0]);
|
|
|
|
GrowableArrayInt h;
|
|
EXPECT_EQ(0, h.length());
|
|
h.Add(101);
|
|
h.Add(102);
|
|
h.Add(103);
|
|
EXPECT_EQ(3, h.length());
|
|
EXPECT_EQ(103, h.Last());
|
|
h.RemoveLast();
|
|
EXPECT_EQ(2, h.length());
|
|
EXPECT_EQ(102, h.Last());
|
|
h.RemoveLast();
|
|
EXPECT_EQ(1, h.length());
|
|
EXPECT_EQ(101, h.Last());
|
|
h.RemoveLast();
|
|
EXPECT_EQ(0, h.length());
|
|
EXPECT(h.is_empty());
|
|
h.Add(-8899);
|
|
h.Add(7908);
|
|
EXPECT(!h.is_empty());
|
|
h.Clear();
|
|
EXPECT(h.is_empty());
|
|
}
|
|
|
|
TEST_CASE(GrowableArray) {
|
|
TestGrowableArray<GrowableArray<int>, GrowableArray<int64_t> >();
|
|
}
|
|
|
|
TEST_CASE(MallocGrowableArray) {
|
|
TestGrowableArray<MallocGrowableArray<int>, MallocGrowableArray<int64_t> >();
|
|
}
|
|
|
|
static int greatestFirst(const int* a, const int* b) {
|
|
if (*a > *b) {
|
|
return -1;
|
|
} else if (*a < *b) {
|
|
return 1;
|
|
} else {
|
|
return 0;
|
|
}
|
|
}
|
|
|
|
TEST_CASE(GrowableArraySort) {
|
|
GrowableArray<int> g;
|
|
g.Add(12);
|
|
g.Add(4);
|
|
g.Add(64);
|
|
g.Add(8);
|
|
g.Sort(greatestFirst);
|
|
EXPECT_EQ(64, g[0]);
|
|
EXPECT_EQ(4, g.Last());
|
|
}
|
|
|
|
ISOLATE_UNIT_TEST_CASE(GrowableHandlePtr) {
|
|
Zone* zone = Thread::Current()->zone();
|
|
GrowableHandlePtrArray<const String> test1(zone, 1);
|
|
EXPECT_EQ(0, test1.length());
|
|
test1.Add(Symbols::Int());
|
|
EXPECT(test1[0].raw() == Symbols::Int().raw());
|
|
EXPECT_EQ(1, test1.length());
|
|
|
|
ZoneGrowableHandlePtrArray<const String>* test2 =
|
|
new ZoneGrowableHandlePtrArray<const String>(zone, 1);
|
|
test2->Add(Symbols::GetterPrefix());
|
|
EXPECT((*test2)[0].raw() == Symbols::GetterPrefix().raw());
|
|
EXPECT_EQ(1, test2->length());
|
|
}
|
|
|
|
} // namespace dart
|