mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
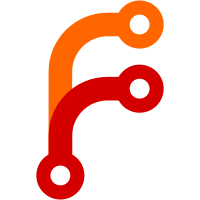
dart-bytecode, arm64: +4.742% geomean dart-bytecode-jit-unopt, arm64: +12.73% geomean dart2js-compile, x64: +3.635% geomean In the polymorphic and unlinked cases, call to a stub the does a linear scan against an ICData. In the monomorphic case, call to a prologue of the expected target function that checks the expected receiver class. There is additional indirection in the JIT version compared to the AOT version to also tick a usage counter so the inliner can make good decisions. In the megamorphic case, call to a stub that does a hash table lookup against a MegamorphicCache. Megamorphic call sites face a loss of precision in usage counts. The call site count is not recorded and the usage counter of the target function is used as an approximation. Monomorphic and megamorphic calls sites are reset to the polymorphic/unlinked state on hot reload. Monomorphic and megamorphic calls sites do not check the stepping state, so they are reset to the polymorphic/unlinked state when stepping begins and disabled. Back-edges now increment the usage counter in addition to checking it. This ensures function with loops containing monomorphic calls will eventually cross the optimization threshold. Fixed backwards use of kMonomorphicEntryOffset and kPolymorphicEntryOffset. Fixed C stack overflow when bouncing between the KBC interpreter and a simulator. Bug: https://github.com/dart-lang/sdk/issues/26780 Bug: https://github.com/dart-lang/sdk/issues/36409 Bug: https://github.com/dart-lang/sdk/issues/36731 Change-Id: I78a49cccd962703a459288e71ce246ed845df474 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/102820 Commit-Queue: Ryan Macnak <rmacnak@google.com> Reviewed-by: Alexander Markov <alexmarkov@google.com>
132 lines
5.1 KiB
C++
132 lines
5.1 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
// Class for patching compiled code.
|
|
|
|
#ifndef RUNTIME_VM_CODE_PATCHER_H_
|
|
#define RUNTIME_VM_CODE_PATCHER_H_
|
|
|
|
#include "vm/allocation.h"
|
|
#include "vm/native_entry.h"
|
|
|
|
namespace dart {
|
|
|
|
// Forward declaration.
|
|
class Code;
|
|
class ICData;
|
|
class RawArray;
|
|
class RawCode;
|
|
class RawFunction;
|
|
class RawObject;
|
|
|
|
#if defined(TARGET_ARCH_IA32) || defined(TARGET_ARCH_DBC)
|
|
// Stack-allocated class to create a scope where the specified region
|
|
// [address, address + size] has write access enabled. This is used
|
|
// when patching generated code. Access is reset to read-execute in
|
|
// the destructor of this scope.
|
|
// Dual mapping of instructions pages is not supported on these target arch.
|
|
class WritableInstructionsScope : public ValueObject {
|
|
public:
|
|
WritableInstructionsScope(uword address, intptr_t size);
|
|
~WritableInstructionsScope();
|
|
|
|
private:
|
|
const uword address_;
|
|
const intptr_t size_;
|
|
};
|
|
#endif // defined(TARGET_ARCH_IA32) || defined(TARGET_ARCH_DBC)
|
|
|
|
class CodePatcher : public AllStatic {
|
|
public:
|
|
// Dart static calls have a distinct, machine-dependent code pattern.
|
|
|
|
// Patch static call before return_address in given code to the new target.
|
|
static void PatchStaticCallAt(uword return_address,
|
|
const Code& code,
|
|
const Code& new_target);
|
|
|
|
// Return the target address of the static call before return_address
|
|
// in given code.
|
|
static RawCode* GetStaticCallTargetAt(uword return_address, const Code& code);
|
|
|
|
// Get instance call information. Returns the call target and sets the output
|
|
// parameter data if non-NULL.
|
|
static RawCode* GetInstanceCallAt(uword return_address,
|
|
const Code& caller_code,
|
|
Object* data);
|
|
|
|
// Change the state of an instance call by patching the corresponding object
|
|
// pool entries (non-IA32) or instructions (IA32).
|
|
static void PatchInstanceCallAt(uword return_address,
|
|
const Code& caller_code,
|
|
const Object& data,
|
|
const Code& target);
|
|
|
|
// Return target of an unoptimized static call and its ICData object
|
|
// (calls target via a stub).
|
|
static RawFunction* GetUnoptimizedStaticCallAt(uword return_address,
|
|
const Code& code,
|
|
ICData* ic_data);
|
|
|
|
static void InsertDeoptimizationCallAt(uword start);
|
|
|
|
static void PatchPoolPointerCallAt(uword return_address,
|
|
const Code& code,
|
|
const Code& new_target);
|
|
|
|
static void PatchSwitchableCallAt(uword return_address,
|
|
const Code& caller_code,
|
|
const Object& data,
|
|
const Code& target);
|
|
static RawObject* GetSwitchableCallDataAt(uword return_address,
|
|
const Code& caller_code);
|
|
static RawCode* GetSwitchableCallTargetAt(uword return_address,
|
|
const Code& caller_code);
|
|
|
|
#if defined(TARGET_ARCH_DBC)
|
|
static NativeFunctionWrapper GetNativeCallAt(uword return_address,
|
|
const Code& caller_code,
|
|
NativeFunction* target);
|
|
#else
|
|
static RawCode* GetNativeCallAt(uword return_address,
|
|
const Code& caller_code,
|
|
NativeFunction* target);
|
|
#endif
|
|
|
|
#if defined(TARGET_ARCH_DBC)
|
|
static void PatchNativeCallAt(uword return_address,
|
|
const Code& caller_code,
|
|
NativeFunction target,
|
|
NativeFunctionWrapper trampoline);
|
|
#else
|
|
static void PatchNativeCallAt(uword return_address,
|
|
const Code& caller_code,
|
|
NativeFunction target,
|
|
const Code& trampoline);
|
|
#endif
|
|
|
|
static intptr_t GetSubtypeTestCachePoolIndex(uword return_address);
|
|
};
|
|
|
|
// Beginning from [end - size] we compare [size] bytes with [pattern]. All
|
|
// [0..255] values in [pattern] have to match, negative values are skipped.
|
|
//
|
|
// Example pattern: `[0x3d, 0x8b, -1, -1]`.
|
|
bool MatchesPattern(uword end, const int16_t* pattern, intptr_t size);
|
|
|
|
class KBCPatcher : public AllStatic {
|
|
public:
|
|
static NativeFunctionWrapper GetNativeCallAt(uword return_address,
|
|
const Bytecode& bytecode,
|
|
NativeFunction* function);
|
|
|
|
static void PatchNativeCallAt(uword return_address,
|
|
const Bytecode& bytecode,
|
|
NativeFunction function,
|
|
NativeFunctionWrapper trampoline);
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_CODE_PATCHER_H_
|