mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
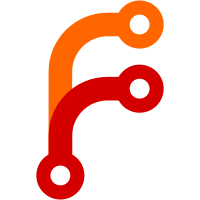
Size of a large application:
Before: 26628600
After: 21480120 (-19.3%)
Size of snapshots:
isolate_snapshot_framework.bin
Before: 9322496
After: 6782976 (-27.2%)
isolate_snapshot_product_framework.bin
Before: 9166848
After: 6602752 (-27.9%)
Regressions in tests:
1) Test language_2/type_alias_equality_test/04 fails similarly to default mode, as VM
does not implement comparison of function types according to the specification.
Previously this test was passing as function types were canonicalized in bytecode,
which was not always correct. This CL fixes the problem with canonicalization of
function types in bytecode and the test starts failing again.
2) Tests standalone_2/entrypoints_verification_test, standalone_2/io/test_extension_test,
standalone_2/io/test_extension_fail_test fail as native extensions are not supported
in bytecode yet. These tests start passing after df5e7aac17
,
which switched bytecode tests to kernel service (on x64), because kernel service doesn't
drop ASTs. This CL switches from reading AST library declarations to bytecode even if
AST is not removed, so tests fail again.
Change-Id: I8b7ba44bfa49d0b1599b2509553ff7c831a4e244
Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/104700
Reviewed-by: Ryan Macnak <rmacnak@google.com>
Reviewed-by: Martin Kustermann <kustermann@google.com>
Reviewed-by: Régis Crelier <regis@google.com>
Commit-Queue: Alexander Markov <alexmarkov@google.com>
118 lines
4.8 KiB
C++
118 lines
4.8 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_CLASS_FINALIZER_H_
|
|
#define RUNTIME_VM_CLASS_FINALIZER_H_
|
|
|
|
#include "vm/allocation.h"
|
|
#include "vm/growable_array.h"
|
|
#include "vm/object.h"
|
|
|
|
namespace dart {
|
|
|
|
// Traverses all pending, unfinalized classes, validates and marks them as
|
|
// finalized.
|
|
class ClassFinalizer : public AllStatic {
|
|
public:
|
|
typedef ZoneGrowableHandlePtrArray<const AbstractType> PendingTypes;
|
|
|
|
// Modes for finalization. The ordering is relevant.
|
|
enum FinalizationKind {
|
|
kFinalize, // Finalize type and type arguments.
|
|
kCanonicalize // Finalize and canonicalize.
|
|
};
|
|
|
|
// Finalize given type while parsing class cls.
|
|
// Also canonicalize and bound check type if applicable.
|
|
static RawAbstractType* FinalizeType(
|
|
const Class& cls,
|
|
const AbstractType& type,
|
|
FinalizationKind finalization = kCanonicalize,
|
|
PendingTypes* pending_types = NULL);
|
|
|
|
// Finalize the types in the functions's signature while parsing class cls.
|
|
static void FinalizeSignature(const Class& cls,
|
|
const Function& function,
|
|
FinalizationKind finalization = kCanonicalize);
|
|
|
|
// Return false if we still have classes pending to be finalized.
|
|
static bool AllClassesFinalized();
|
|
|
|
// Useful for sorting classes to make dispatch faster.
|
|
static void SortClasses();
|
|
static void RemapClassIds(intptr_t* old_to_new_cid);
|
|
static void RehashTypes();
|
|
static void ClearAllCode(bool including_nonchanging_cids = false);
|
|
|
|
// Return whether processing pending classes (ObjectStore::pending_classes_)
|
|
// failed. The function returns true if the processing was successful.
|
|
// If processing fails, an error message is set in the sticky error field
|
|
// in the object store.
|
|
static bool ProcessPendingClasses();
|
|
|
|
// Finalize the types appearing in the declaration of class 'cls', i.e. its
|
|
// type parameters and their upper bounds, its super type and interfaces.
|
|
// Note that the fields and functions have not been parsed yet (unless cls
|
|
// is an anonymous top level class).
|
|
static void FinalizeTypesInClass(const Class& cls);
|
|
|
|
// Register class in the lists of direct subclasses and direct implementors.
|
|
static void RegisterClassInHierarchy(Zone* zone, const Class& cls);
|
|
|
|
// Finalize the class including its fields and functions.
|
|
static void FinalizeClass(const Class& cls);
|
|
|
|
// Completes loading of the class, this populates the function
|
|
// and fields of the class.
|
|
//
|
|
// Returns Error::null() if there is no loading error.
|
|
static RawError* LoadClassMembers(const Class& cls);
|
|
|
|
#if !defined(DART_PRECOMPILED_RUNTIME)
|
|
// Verify that the classes have been properly prefinalized. This is
|
|
// needed during bootstrapping where the classes have been preloaded.
|
|
static void VerifyBootstrapClasses();
|
|
#endif // !defined(DART_PRECOMPILED_RUNTIME)
|
|
|
|
private:
|
|
static void AllocateEnumValues(const Class& enum_cls);
|
|
static void FinalizeTypeParameters(const Class& cls,
|
|
PendingTypes* pending_types = NULL);
|
|
static intptr_t ExpandAndFinalizeTypeArguments(const Class& cls,
|
|
const AbstractType& type,
|
|
PendingTypes* pending_types);
|
|
static void FinalizeTypeArguments(const Class& cls,
|
|
const TypeArguments& arguments,
|
|
intptr_t num_uninitialized_arguments,
|
|
PendingTypes* pending_types,
|
|
TrailPtr trail);
|
|
static void CheckRecursiveType(const Class& cls,
|
|
const AbstractType& type,
|
|
PendingTypes* pending_types);
|
|
static void FinalizeUpperBounds(
|
|
const Class& cls,
|
|
FinalizationKind finalization = kCanonicalize);
|
|
static void FinalizeMemberTypes(const Class& cls);
|
|
static void PrintClassInformation(const Class& cls);
|
|
|
|
static void ReportError(const Error& error);
|
|
static void ReportError(const Class& cls,
|
|
TokenPosition token_pos,
|
|
const char* format,
|
|
...) PRINTF_ATTRIBUTE(3, 4);
|
|
static void ReportErrors(const Error& prev_error,
|
|
const Class& cls,
|
|
TokenPosition token_pos,
|
|
const char* format,
|
|
...) PRINTF_ATTRIBUTE(4, 5);
|
|
|
|
// Verify implicit offsets recorded in the VM for direct access to fields of
|
|
// Dart instances (e.g: _TypedListView, _ByteDataView).
|
|
static void VerifyImplicitFieldOffsets();
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_CLASS_FINALIZER_H_
|