mirror of
https://github.com/dart-lang/sdk
synced 2024-11-05 18:22:09 +00:00
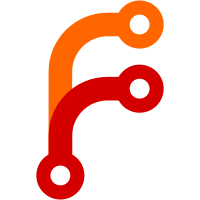
Interesting tests requiring status file or other changes: type_variable_promotion_test type_variable_scope_test type_variable_static_context_negative_test - changed from negative to compile-time error unresolved_top_level_method_negative_test - changed from negative to compile-time error unary_plus_negative_test unhandled_exception_negative_test unbound_getter_test unresolved_in_factory_negative_test - unresolved_top_level_var_negative_test - changed from negative to compile-time error unresolved_top_level_method_negative_test - changed from negative to compile-time error vm/async_await_catch_stacktrace_test BUG= R=lrn@google.com Review-Url: https://codereview.chromium.org/3008723002 .
40 lines
897 B
Dart
40 lines
897 B
Dart
// Copyright (c) 2011, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
// Dart test program to check that we can resolve unqualified identifiers
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
class B {
|
|
B(x, y) : b = y {}
|
|
var b;
|
|
|
|
get_b() {
|
|
// Resolving unqualified instance method.
|
|
return really_really_get_it();
|
|
}
|
|
|
|
really_really_get_it() {
|
|
return 5;
|
|
}
|
|
}
|
|
|
|
class UnqualNameTest {
|
|
static eleven() {
|
|
return 11;
|
|
}
|
|
|
|
static testMain() {
|
|
var o = new B(3, 5);
|
|
Expect.equals(11, eleven()); // Unqualified static method call.
|
|
Expect.equals(5, o.get_b());
|
|
|
|
// Check whether we handle variable initializers correctly.
|
|
var a = 1, x, b = a + 3;
|
|
Expect.equals(5, a + b);
|
|
}
|
|
}
|
|
|
|
main() {
|
|
UnqualNameTest.testMain();
|
|
}
|