mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 14:32:24 +00:00
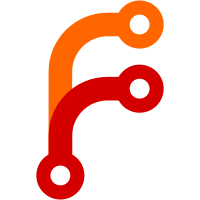
This is a preparation CL to remove the --enable-isolate-groups flag in the VM. The following tests were only running in --no-enable-isolate-groups and are therefore obsolete now: - runtime/tests/vm/dart/regress_47468_test.dart - runtime/tests/vm/dart_2/regress_47468_test.dart - tests/lib/isolate/illegal_msg_function_test.dart - tests/lib_2/isolate/illegal_msg_function_test.dart TEST=Changes tests only. Change-Id: I6257cb667eebca66a649614d3010139dd2cdd3ab Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/219100 Reviewed-by: Alexander Aprelev <aam@google.com> Commit-Queue: Martin Kustermann <kustermann@google.com>
41 lines
826 B
Dart
41 lines
826 B
Dart
// Copyright (c) 2017, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
library regress;
|
|
|
|
import 'dart:isolate';
|
|
|
|
abstract class N {}
|
|
|
|
class J extends N {
|
|
final String s;
|
|
J(this.s);
|
|
}
|
|
|
|
class K extends N {
|
|
final int i;
|
|
K(this.i);
|
|
}
|
|
|
|
void isolate(Object portObj) {
|
|
SendPort port = portObj as SendPort;
|
|
port.send(N);
|
|
port.send(new J("8" * 4));
|
|
for (int i = 0; i < 80000; i++) {
|
|
port.send(new K(i));
|
|
}
|
|
port.send('done');
|
|
}
|
|
|
|
main() async {
|
|
var recv = new RawReceivePort();
|
|
recv.handler = (k) {
|
|
if (k is J) print(k.s.length);
|
|
if (k is String) {
|
|
print(k);
|
|
recv.close();
|
|
}
|
|
};
|
|
var iso = await Isolate.spawn(isolate, recv.sendPort);
|
|
}
|