mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 03:07:49 +00:00
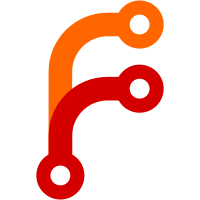
This adds the `close()` method to `DynamicLibrary`, which uses `dlclose()` on Unix and `FreeLibrary` on Windows to close a dynamic FFI library. TEST=tests/ffi/dylib_close_test.dart Closes https://github.com/dart-lang/sdk/issues/40159 Cq-Include-Trybots: luci.dart.try:vm-ffi-android-debug-arm-try,vm-ffi-android-debug-arm64c-try,vm-ffi-qemu-linux-release-riscv64-try,vm-ffi-qemu-linux-release-arm-try,dart2wasm-linux-d8-try,dart2wasm-linux-chrome-try,vm-reload-rollback-linux-debug-x64-try,vm-reload-linux-debug-x64-try,vm-win-debug-x64c-try,vm-win-debug-x64-try,vm-aot-android-release-arm_x64-try,vm-mac-debug-arm64-try,vm-mac-debug-x64-try,vm-asan-linux-release-x64-try,vm-aot-msan-linux-release-x64-try,vm-aot-android-release-arm64c-try,vm-aot-android-release-arm_x64-try,vm-aot-linux-debug-x64-try,vm-aot-linux-debug-x64c-try,vm-aot-mac-release-x64-try,vm-aot-mac-release-arm64-try,vm-aot-win-debug-x64c-try,vm-aot-win-release-x64-try CoreLibraryReviewExempt: `dart:ffi` VM only API. Change-Id: I73af98677b481902fe548bdcee56964a0195faf0 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/307680 Reviewed-by: Daco Harkes <dacoharkes@google.com> Commit-Queue: Daco Harkes <dacoharkes@google.com>
38 lines
904 B
Dart
38 lines
904 B
Dart
// Copyright (c) 2023, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
//
|
|
// SharedObjects=ffi_test_functions
|
|
|
|
// @dart=2.9
|
|
|
|
import 'dart:ffi';
|
|
import 'dart:io';
|
|
|
|
import 'package:expect/expect.dart';
|
|
|
|
import 'dylib_utils.dart';
|
|
|
|
void main() {
|
|
testClose();
|
|
testUnclosable();
|
|
}
|
|
|
|
void testClose() {
|
|
final lib = dlopenPlatformSpecific("ffi_test_functions");
|
|
lib.lookup('ReturnMaxUint8');
|
|
|
|
lib.close();
|
|
Expect.throwsStateError(
|
|
() => lib.lookup('ReturnMaxUint8'), 'Illegal lookup in closed library');
|
|
lib.close(); // Duplicate close should not crash.
|
|
}
|
|
|
|
void testUnclosable() {
|
|
final proc = DynamicLibrary.process();
|
|
final exec = DynamicLibrary.executable();
|
|
|
|
Expect.throwsStateError(proc.close);
|
|
Expect.throwsStateError(exec.close);
|
|
}
|