mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
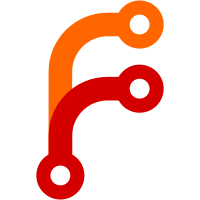
Fixes a scaling limitation where compiling N functions using the same guarded field or CHA guarded interface will result in O(N^2) comparisons when registering the dependencies. Don't use Instructions' address as the hash, as this gets relocated between the snapshot writer and reader. TEST=ci Bug: https://github.com/dart-lang/sdk/issues/51125 Change-Id: I9a13d57455e10865d9c5f7c12009d869a4ef0488 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/279753 Reviewed-by: Martin Kustermann <kustermann@google.com> Commit-Queue: Ryan Macnak <rmacnak@google.com>
40 lines
1.1 KiB
C++
40 lines
1.1 KiB
C++
// Copyright (c) 2014, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_HEAP_WEAK_CODE_H_
|
|
#define RUNTIME_VM_HEAP_WEAK_CODE_H_
|
|
|
|
#include "vm/allocation.h"
|
|
#include "vm/globals.h"
|
|
|
|
namespace dart {
|
|
|
|
class WeakArray;
|
|
class Code;
|
|
|
|
// Helper class to handle an array of code weak properties. Implements
|
|
// registration and disabling of stored code objects.
|
|
class WeakCodeReferences : public ValueObject {
|
|
public:
|
|
explicit WeakCodeReferences(const WeakArray& value) : array_(value) {}
|
|
virtual ~WeakCodeReferences() {}
|
|
|
|
void Register(const Code& value);
|
|
|
|
virtual void UpdateArrayTo(const WeakArray& array) = 0;
|
|
virtual void ReportDeoptimization(const Code& code) = 0;
|
|
virtual void ReportSwitchingCode(const Code& code) = 0;
|
|
|
|
void DisableCode(bool are_mutators_stopped);
|
|
|
|
bool HasCodes() const;
|
|
|
|
private:
|
|
const WeakArray& array_; // Array of Code objects.
|
|
DISALLOW_COPY_AND_ASSIGN(WeakCodeReferences);
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_HEAP_WEAK_CODE_H_
|