mirror of
https://github.com/dart-lang/sdk
synced 2024-09-15 23:19:47 +00:00
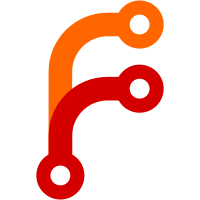
Extend test_runner VMOptions support with an ability to specify paths relative to temporary compilation directory. // VMOptions=--foo=$TEST_COMPILATION_DIR/foo.file The same directory will also be passed as an environment variable to execution command. Migrate most of the tests which used to write stuff into the SDK root to use this feature. I am leaving vm/dart/causal/* tests unmigrated because migrating requires time consuming manual update of expectations (which encode raw line numbers). I have a follow up CL which changes how these tests are written which will make migration trivial. Change-Id: Id53008be66de8ff18623efac27ff15750f407749 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/300600 Reviewed-by: William Hesse <whesse@google.com> Commit-Queue: Slava Egorov <vegorov@google.com>
80 lines
2.1 KiB
Dart
80 lines
2.1 KiB
Dart
// Copyright (c) 2017, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// @dart = 2.9
|
|
|
|
/// VMOptions=--dwarf-stack-traces --save-debugging-info=$TEST_COMPILATION_DIR/dwarf_obfuscate.so --obfuscate
|
|
|
|
import 'dart:io';
|
|
|
|
import 'package:native_stack_traces/native_stack_traces.dart';
|
|
import 'package:path/path.dart' as path;
|
|
|
|
import 'dwarf_stack_trace_test.dart' as base;
|
|
|
|
@pragma("vm:prefer-inline")
|
|
bar() {
|
|
// Keep the 'throw' and its argument on separate lines.
|
|
throw // force linebreak with dart format
|
|
"Hello, Dwarf!";
|
|
}
|
|
|
|
@pragma("vm:never-inline")
|
|
foo() {
|
|
bar();
|
|
}
|
|
|
|
Future<void> main() async {
|
|
String rawStack = "";
|
|
try {
|
|
foo();
|
|
} catch (e, st) {
|
|
rawStack = st.toString();
|
|
}
|
|
|
|
if (path.basenameWithoutExtension(Platform.executable) !=
|
|
"dart_precompiled_runtime") {
|
|
return; // Not running from an AOT compiled snapshot.
|
|
}
|
|
|
|
if (Platform.isAndroid) {
|
|
return; // Generated dwarf.so not available on the test device.
|
|
}
|
|
|
|
final dwarf = Dwarf.fromFile(path.join(
|
|
Platform.environment['TEST_COMPILATION_DIR'], "dwarf_obfuscate.so"));
|
|
|
|
await base.checkStackTrace(rawStack, dwarf, expectedCallsInfo);
|
|
}
|
|
|
|
final expectedCallsInfo = <List<DartCallInfo>>[
|
|
// The first frame should correspond to the throw in bar, which was inlined
|
|
// into foo (so we'll get information for two calls for that PC address).
|
|
[
|
|
DartCallInfo(
|
|
function: "bar",
|
|
filename: "dwarf_stack_trace_obfuscate_test.dart",
|
|
line: 19,
|
|
column: 3,
|
|
inlined: true),
|
|
DartCallInfo(
|
|
function: "foo",
|
|
filename: "dwarf_stack_trace_obfuscate_test.dart",
|
|
line: 25,
|
|
column: 3,
|
|
inlined: false)
|
|
],
|
|
// The second frame corresponds to call to foo in main.
|
|
[
|
|
DartCallInfo(
|
|
function: "main",
|
|
filename: "dwarf_stack_trace_obfuscate_test.dart",
|
|
line: 31,
|
|
column: 5,
|
|
inlined: false)
|
|
],
|
|
// Don't assume anything about any of the frames below the call to foo
|
|
// in main, as this makes the test too brittle.
|
|
];
|