mirror of
https://github.com/dart-lang/sdk
synced 2024-09-20 00:01:59 +00:00
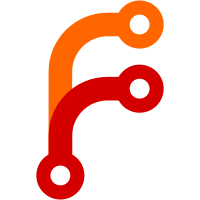
This reverts commit d9a306fc59
.
Reason for revert:
=================================================================
==176913==ERROR: LeakSanitizer: detected memory leaks
Direct leak of 13 byte(s) in 1 object(s) allocated from:
#0 0x55d94882d540 in __interceptor_strdup /b/s/w/ir/kitchen-workdir/llvm-project/compiler-rt/lib/asan/asan_interceptors.cc:447:3
#1 0x55d9491abcd7 in dart::KernelIsolate::AddExperimentalFlag(char const*) ../../out/DebugX64/../../runtime/vm/kernel_isolate.cc:382:28
#2 0x55d9490af7d2 in dart::Flags::SetFlagFromString(dart::Flag*, char const*) ../../out/DebugX64/../../runtime/vm/flags.cc:338:7
#3 0x55d9490b0221 in dart::Flags::Parse(char const*) ../../out/DebugX64/../../runtime/vm/flags.cc:399:12
#4 0x55d9490b0538 in dart::Flags::ProcessCommandLineFlags(int, char const**) ../../out/DebugX64/../../runtime/vm/flags.cc:438:5
#5 0x55d9488885df in dart::bin::main(int, char**) ../../out/DebugX64/../../runtime/bin/main.cc:1149:11
#6 0x55d94888ad9a in main ../../out/DebugX64/../../runtime/bin/main.cc:1245:3
#7 0x7f5ccc4a452a in __libc_start_main (/lib/x86_64-linux-gnu/libc.so.6+0x2352a)
SUMMARY: AddressSanitizer: 13 byte(s) leaked in 1 allocation(s).
Original change's description:
> [vm] Avoid allocating monitors globally - embedders in certain situations will run into reported leakes due to them
>
> Change-Id: Ied0446aebfdd8fb7e15c510cdf4160ff9ad013a6
> Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/122148
> Reviewed-by: Ryan Macnak <rmacnak@google.com>
> Commit-Queue: Martin Kustermann <kustermann@google.com>
TBR=kustermann@google.com,rmacnak@google.com,asiva@google.com
Change-Id: I2c98bf7e0e565c6f8c016b4832d5587868c65a83
No-Presubmit: true
No-Tree-Checks: true
No-Try: true
Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/122680
Reviewed-by: Aart Bik <ajcbik@google.com>
Commit-Queue: Aart Bik <ajcbik@google.com>
108 lines
3.2 KiB
C++
108 lines
3.2 KiB
C++
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_SERVICE_ISOLATE_H_
|
|
#define RUNTIME_VM_SERVICE_ISOLATE_H_
|
|
|
|
#include "include/dart_api.h"
|
|
|
|
#include "vm/allocation.h"
|
|
#include "vm/os_thread.h"
|
|
|
|
namespace dart {
|
|
|
|
class Isolate;
|
|
class ObjectPointerVisitor;
|
|
class SendPort;
|
|
|
|
class ServiceIsolate : public AllStatic {
|
|
public:
|
|
static const char* kName;
|
|
static bool NameEquals(const char* name);
|
|
|
|
static bool Exists();
|
|
static bool IsRunning();
|
|
static bool IsServiceIsolate(const Isolate* isolate);
|
|
static bool IsServiceIsolateDescendant(const Isolate* isolate);
|
|
static Dart_Port Port();
|
|
|
|
static Dart_Port WaitForLoadPort();
|
|
static Dart_Port LoadPort();
|
|
|
|
// Returns `true` if the request was sucessfully sent. If it was, the
|
|
// [reply_port] will receive a Dart_TypedData_kUint8 response json.
|
|
//
|
|
// If sending the rpc failed and [error] is not `nullptr` then [error] might
|
|
// be set to a string containting the reason for the failure. If so, the
|
|
// caller is responsible for free()ing the error.
|
|
static bool SendServiceRpc(uint8_t* request_json,
|
|
intptr_t request_json_length,
|
|
Dart_Port reply_port,
|
|
char** error);
|
|
|
|
static void Run();
|
|
static bool SendIsolateStartupMessage();
|
|
static bool SendIsolateShutdownMessage();
|
|
static void SendServiceExitMessage();
|
|
static void Shutdown();
|
|
|
|
static void BootVmServiceLibrary();
|
|
|
|
static void RequestServerInfo(const SendPort& sp);
|
|
static void ControlWebServer(const SendPort& sp, bool enable);
|
|
|
|
static void SetServerAddress(const char* address);
|
|
|
|
// Returns the server's web address or NULL if none is running.
|
|
static const char* server_address() { return server_address_; }
|
|
|
|
static void VisitObjectPointers(ObjectPointerVisitor* visitor);
|
|
|
|
private:
|
|
static void KillServiceIsolate();
|
|
|
|
// Does not need a current thread.
|
|
static Dart_Port WaitForLoadPortInternal();
|
|
|
|
protected:
|
|
static void SetServicePort(Dart_Port port);
|
|
static void SetServiceIsolate(Isolate* isolate);
|
|
static void SetLoadPort(Dart_Port port);
|
|
static void FinishedExiting();
|
|
static void FinishedInitializing();
|
|
static void InitializingFailed(char* error);
|
|
static void MaybeMakeServiceIsolate(Isolate* isolate);
|
|
static Dart_IsolateGroupCreateCallback create_group_callback() {
|
|
return create_group_callback_;
|
|
}
|
|
|
|
static Dart_IsolateGroupCreateCallback create_group_callback_;
|
|
static Monitor* monitor_;
|
|
enum State {
|
|
kStopped,
|
|
kStarting,
|
|
kStarted,
|
|
kStopping,
|
|
};
|
|
static State state_;
|
|
static Isolate* isolate_;
|
|
static Dart_Port port_;
|
|
static Dart_Port load_port_;
|
|
static Dart_Port origin_;
|
|
static char* server_address_;
|
|
|
|
// If starting the service-isolate failed, this error might provide the reason
|
|
// for the failure.
|
|
static char* startup_failure_reason_;
|
|
|
|
friend class Dart;
|
|
friend class Isolate;
|
|
friend class RunServiceTask;
|
|
friend class ServiceIsolateNatives;
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_SERVICE_ISOLATE_H_
|