mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 08:20:31 +00:00
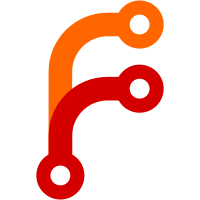
This removes the need for passing the flag to use Dart_ExecuteInternalCommand, which is done in several tests that otherwise have nothing to do with testing pragmas. Also adds status file skips for precomp-win targets that currently crash due to https://github.com/dart-lang/sdk/issues/40579. TEST=CQ Bug: https://github.com/dart-lang/sdk/issues/46059, https://github.com/dart-lang/sdk/issues/46061 Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-win-release-x64-try,vm-kernel-precomp-nnbd-win-release-x64-try,vm-kernel-win-debug-x64-try Change-Id: I3024ad9bedb7a74abaaaa1020b7525e5d8b1bd47 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/200461 Commit-Queue: Clement Skau <cskau@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com>
54 lines
1.5 KiB
Dart
54 lines
1.5 KiB
Dart
// Copyright (c) 2019, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
//
|
|
// Dart test program for testing dart:ffi struct pointers.
|
|
//
|
|
// VMOptions=--deterministic
|
|
//
|
|
// SharedObjects=ffi_test_functions
|
|
//
|
|
// TODO(37295): Merge this file with regress_37511_test.dart when callback
|
|
// support lands.
|
|
|
|
// @dart = 2.9
|
|
|
|
import 'dart:ffi';
|
|
|
|
import 'ffi_test_helpers.dart';
|
|
|
|
/// Estimate of how many allocations functions in `functionsToTest` do at most.
|
|
const gcAfterNAllocationsMax = 10;
|
|
|
|
void main() {
|
|
for (Function() f in functionsToTest) {
|
|
f(); // Ensure code is compiled.
|
|
|
|
for (int n = 1; n <= gcAfterNAllocationsMax; n++) {
|
|
collectOnNthAllocation(n);
|
|
f();
|
|
}
|
|
}
|
|
}
|
|
|
|
final List<Function()> functionsToTest = [
|
|
// Callback trampolines.
|
|
doFromFunction,
|
|
() => callbackSmallDouble(dartFunctionPointer),
|
|
];
|
|
|
|
// Callback trampoline helpers.
|
|
typedef NativeCallbackTest = Int32 Function(Pointer);
|
|
typedef NativeCallbackTestFn = int Function(Pointer);
|
|
|
|
final callbackSmallDouble =
|
|
ffiTestFunctions.lookupFunction<NativeCallbackTest, NativeCallbackTestFn>(
|
|
"TestSimpleMultiply");
|
|
|
|
typedef SimpleMultiplyType = Double Function(Double);
|
|
double simpleMultiply(double x) => x * 1.337;
|
|
|
|
final doFromFunction =
|
|
() => Pointer.fromFunction<SimpleMultiplyType>(simpleMultiply, 0.0);
|
|
|
|
final dartFunctionPointer = doFromFunction();
|