mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 08:20:31 +00:00
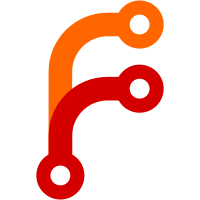
This removes the need for passing the flag to use Dart_ExecuteInternalCommand, which is done in several tests that otherwise have nothing to do with testing pragmas. Also adds status file skips for precomp-win targets that currently crash due to https://github.com/dart-lang/sdk/issues/40579. TEST=CQ Bug: https://github.com/dart-lang/sdk/issues/46059, https://github.com/dart-lang/sdk/issues/46061 Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-win-release-x64-try,vm-kernel-precomp-nnbd-win-release-x64-try,vm-kernel-win-debug-x64-try Change-Id: I3024ad9bedb7a74abaaaa1020b7525e5d8b1bd47 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/200461 Commit-Queue: Clement Skau <cskau@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com>
66 lines
1.7 KiB
Dart
66 lines
1.7 KiB
Dart
// Copyright (c) 2020, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// @dart = 2.9
|
|
|
|
// Check that JumpToFrame does not use LR clobbered by slow path of
|
|
// TransitionNativeToGenerated.
|
|
// VMOptions=--use-slow-path
|
|
|
|
import 'dart:ffi';
|
|
|
|
import 'package:ffi/ffi.dart';
|
|
import 'package:expect/expect.dart';
|
|
|
|
typedef CallbackDartType = Object Function();
|
|
typedef CallbackNativeType = Handle Function();
|
|
|
|
Object alwaysThrows() {
|
|
throw 'exception';
|
|
}
|
|
|
|
void alwaysCatches(CallbackDartType f) {
|
|
try {
|
|
propagateError(f());
|
|
} catch (e) {
|
|
Expect.equals('exception', e);
|
|
return;
|
|
}
|
|
Expect.isTrue(false);
|
|
}
|
|
|
|
void main() {
|
|
final ptr = Pointer.fromFunction<CallbackNativeType>(alwaysThrows);
|
|
final f = ptr.asFunction<CallbackDartType>();
|
|
alwaysCatches(f);
|
|
}
|
|
|
|
typedef Dart_PropagateError_NativeType = Void Function(Handle);
|
|
typedef Dart_PropagateError_DartType = void Function(Object);
|
|
|
|
final Dart_PropagateError_DartType propagateError = () {
|
|
final Pointer<_DartApi> dlapi = NativeApi.initializeApiDLData.cast();
|
|
for (int i = 0; dlapi.ref.functions[i].name != nullptr; i++) {
|
|
final name = dlapi.ref.functions[i].name.cast<Utf8>().toDartString();
|
|
if (name == 'Dart_PropagateError') {
|
|
return dlapi.ref.functions[i].function
|
|
.cast<NativeFunction<Dart_PropagateError_NativeType>>()
|
|
.asFunction<Dart_PropagateError_DartType>();
|
|
}
|
|
}
|
|
throw 'Not found';
|
|
}();
|
|
|
|
class _DartEntry extends Struct {
|
|
Pointer<Int8> name;
|
|
Pointer<Void> function;
|
|
}
|
|
|
|
class _DartApi extends Struct {
|
|
@Int32()
|
|
int major;
|
|
@Int32()
|
|
int minor;
|
|
Pointer<_DartEntry> functions;
|
|
}
|