mirror of
https://github.com/dart-lang/sdk
synced 2024-09-15 23:49:47 +00:00
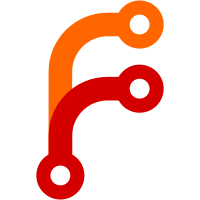
Mark tests that contain errors about using a class as a mixin to use language version 2.19 where that's not an error. This may not fix all of the tests because it's the language version of the library where the class is declared that matters, not where the class is used as a mixin. But most tests have all of their declarations in the same library, so this should fix most. Change-Id: I910439ebd2f10f731418dc588b7e4619a0841c16 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/285923 Reviewed-by: Jake Macdonald <jakemac@google.com> Commit-Queue: Jake Macdonald <jakemac@google.com>
88 lines
1.9 KiB
Dart
88 lines
1.9 KiB
Dart
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// TODO(51557): Decide if the mixins being applied in this test should be
|
|
// "mixin", "mixin class" or the test should be left at 2.19.
|
|
// @dart=2.19
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
class S {}
|
|
|
|
class M1 {}
|
|
|
|
class M2 {}
|
|
|
|
class C = S with M1;
|
|
class D = S with M1, M2;
|
|
class E = S with M2, M1;
|
|
|
|
class F extends E {}
|
|
|
|
class C_ = S with M1;
|
|
class D_ = S with M1, M2;
|
|
class E_ = S with M2, M1;
|
|
|
|
class F_ extends E_ {}
|
|
|
|
main() {
|
|
var c = new C();
|
|
Expect.isTrue(c is C);
|
|
Expect.isFalse(c is D);
|
|
Expect.isFalse(c is E);
|
|
Expect.isFalse(c is F);
|
|
Expect.isTrue(c is S);
|
|
Expect.isTrue(c is M1);
|
|
Expect.isFalse(c is M2);
|
|
|
|
var d = new D();
|
|
Expect.isFalse(d is C);
|
|
Expect.isTrue(d is D);
|
|
Expect.isFalse(d is E);
|
|
Expect.isFalse(d is F);
|
|
Expect.isTrue(d is S);
|
|
Expect.isTrue(d is M1);
|
|
Expect.isTrue(d is M2);
|
|
|
|
var e = new E();
|
|
Expect.isFalse(e is C);
|
|
Expect.isFalse(e is D);
|
|
Expect.isTrue(e is E);
|
|
Expect.isFalse(e is F);
|
|
Expect.isTrue(e is S);
|
|
Expect.isTrue(e is M1);
|
|
Expect.isTrue(e is M2);
|
|
|
|
var f = new F();
|
|
Expect.isFalse(f is C);
|
|
Expect.isFalse(f is D);
|
|
Expect.isTrue(f is E);
|
|
Expect.isTrue(f is F);
|
|
Expect.isTrue(f is S);
|
|
Expect.isTrue(f is M1);
|
|
Expect.isTrue(f is M2);
|
|
|
|
// Make sure we get a new class for each mixin
|
|
// application (at least the named ones).
|
|
Expect.isFalse(c is C_);
|
|
Expect.isFalse(c is D_);
|
|
Expect.isFalse(c is E_);
|
|
Expect.isFalse(c is F_);
|
|
|
|
Expect.isFalse(d is C_);
|
|
Expect.isFalse(d is D_);
|
|
Expect.isFalse(d is E_);
|
|
Expect.isFalse(d is F_);
|
|
|
|
Expect.isFalse(e is C_);
|
|
Expect.isFalse(e is D_);
|
|
Expect.isFalse(e is E_);
|
|
Expect.isFalse(e is F_);
|
|
|
|
Expect.isFalse(f is C_);
|
|
Expect.isFalse(f is D_);
|
|
Expect.isFalse(f is E_);
|
|
Expect.isFalse(f is F_);
|
|
}
|