mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 00:29:48 +00:00
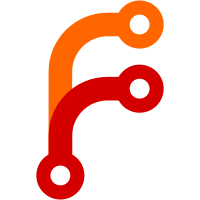
Tested: No new tests. Change-Id: Idf19ce8b6743b221841e6cef6b2a80e8ab37860e Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/354260 Auto-Submit: Lasse Nielsen <lrn@google.com> Reviewed-by: Johnni Winther <johnniwinther@google.com> Reviewed-by: Nate Bosch <nbosch@google.com> Reviewed-by: Daco Harkes <dacoharkes@google.com> Commit-Queue: Lasse Nielsen <lrn@google.com>
53 lines
1.6 KiB
Dart
53 lines
1.6 KiB
Dart
// Copyright (c) 2023, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// Tests that the representation variable may undergo field promotion, assuming
|
|
// that it's private.
|
|
//
|
|
// These tests specifically exercise extension types whose representation type
|
|
// is nullable, since those are handled differently by the front end than
|
|
// extension types whose representation type is non-nullable.
|
|
|
|
import '../static_type_helper.dart';
|
|
|
|
extension type E(Object Function()? _f) {
|
|
testImplicitThisAccess() {
|
|
if (_f != null) {
|
|
_f.expectStaticType<Exactly<Object Function()>>();
|
|
_f().expectStaticType<Exactly<Object>>();
|
|
}
|
|
}
|
|
|
|
testExplicitThisAccess() {
|
|
if (this._f != null) {
|
|
this._f.expectStaticType<Exactly<Object Function()>>();
|
|
this._f().expectStaticType<Exactly<Object>>();
|
|
}
|
|
}
|
|
}
|
|
|
|
testGeneralPropertyAccess(E e) {
|
|
if ((e)._f != null) {
|
|
(e)._f.expectStaticType<Exactly<Object Function()>>();
|
|
(e)._f().expectStaticType<Exactly<Object>>();
|
|
}
|
|
}
|
|
|
|
testPrefixedIdentifierAccess(E e) {
|
|
// Note: the analyzer has a special representation for property accesses of
|
|
// the form `IDENTIFIER.IDENTIFIER`, so we test this form separately.
|
|
if (e._f != null) {
|
|
e._f.expectStaticType<Exactly<Object Function()>>();
|
|
e._f().expectStaticType<Exactly<Object>>();
|
|
}
|
|
}
|
|
|
|
main() {
|
|
int Function() f = () => 0;
|
|
E(f).testImplicitThisAccess();
|
|
E(f).testExplicitThisAccess();
|
|
testGeneralPropertyAccess(E(f));
|
|
testPrefixedIdentifierAccess(E(f));
|
|
}
|