mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 00:29:48 +00:00
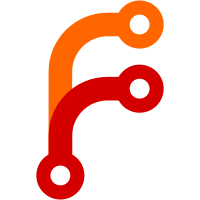
Note that this is not a breaking change, since the CFE already correctly flags the error condition. Fixes #55734. Bug: https://github.com/dart-lang/sdk/issues/55734 Change-Id: I5570e0840ce20c2a761d88f698b9876f7543bd8e Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/366680 Reviewed-by: Brian Wilkerson <brianwilkerson@google.com> Reviewed-by: Konstantin Shcheglov <scheglov@google.com> Commit-Queue: Paul Berry <paulberry@google.com>
47 lines
1.5 KiB
Dart
47 lines
1.5 KiB
Dart
// Copyright (c) 2024, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// This test verifies that implicit `.call` tearoff does not occur when the
|
|
// static type of the expression is a nullable type variable, or a type variable
|
|
// whose bound is nullable.
|
|
|
|
import '../static_type_helper.dart';
|
|
|
|
class C {
|
|
void call() {}
|
|
}
|
|
|
|
void testTypeVariableExtendsCallableClass<T extends C>(T? t) {
|
|
context<void Function()>(t);
|
|
// ^
|
|
// [analyzer] COMPILE_TIME_ERROR.ARGUMENT_TYPE_NOT_ASSIGNABLE
|
|
// [cfe] Can't tear off method 'call' from a potentially null value.
|
|
}
|
|
|
|
void testTypeVariableExtendsNullableCallableClass<T extends C?>(T t) {
|
|
context<void Function()>(t);
|
|
// ^
|
|
// [analyzer] COMPILE_TIME_ERROR.ARGUMENT_TYPE_NOT_ASSIGNABLE
|
|
// [cfe] Can't tear off method 'call' from a potentially null value.
|
|
}
|
|
|
|
void testTypeVariablePromotedToNullableCallableClass<T>(T t) {
|
|
if (t is C?) {
|
|
context<void Function()>(t);
|
|
// ^
|
|
// [analyzer] COMPILE_TIME_ERROR.ARGUMENT_TYPE_NOT_ASSIGNABLE
|
|
// [cfe] Can't tear off method 'call' from a potentially null value.
|
|
}
|
|
}
|
|
|
|
void testTypeVariableExtendsNullableTypeVariable<T extends U?, U extends C>(
|
|
T t) {
|
|
context<void Function()>(t);
|
|
// ^
|
|
// [analyzer] COMPILE_TIME_ERROR.ARGUMENT_TYPE_NOT_ASSIGNABLE
|
|
// [cfe] Can't tear off method 'call' from a potentially null value.
|
|
}
|
|
|
|
main() {}
|