mirror of
https://github.com/dart-lang/sdk
synced 2024-09-19 14:32:49 +00:00
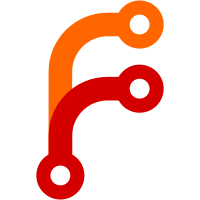
This reverts commit 67f0d4daf0
, and further optimises constructor contexts by preventing empty contexts.
Reason for revert: Includes fix for Flutter engine unit test failures.
Fixes https://github.com/dart-lang/sdk/issues/53506
Original change's description:
[dart2wasm] Replace `struct.new_default` with `struct.new` for object allocation.
When using the `struct.new_default` instruction for object allocation,
fields are always nullable and mutable. By using the `struct.new`
instruction instead, class fields can now have the same mutability and
nullability in Wasm as declared in Dart. In addition, the class ID and
type parameters (which are also stored in an object's struct), can now
be immutable and nonnullable as well.
To do this, object construction is now split into three functions:
(1) Initializer: evaluates initializers for instance fields and
constructor initializers (this constructor before super constructor).
(2) Constructor body: executes the constructor body (super constructor
before this constructor), with `this` pointed to the constructed object.
(3) Constructor allocator: which calls (1), allocates the object using
`struct.new`, then calls (2).
Because fields now have the correct mutability and nullability in Wasm,
this removes unnecessary null checks for nonnullable fields, and may
allow for better optimisations by Binaryen.
Fixes https://github.com/dart-lang/sdk/issues/51492
Change-Id: I13499bdc412f474bc76473115b6e63d6954f4d23
Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/326080
Reviewed-by: Ömer Ağacan <omersa@google.com>
Commit-Queue: Jess Lally <jessicalally@google.com>
Reviewed-by: Aske Simon Christensen <askesc@google.com>
80 lines
2.7 KiB
Dart
80 lines
2.7 KiB
Dart
// Copyright (c) 2022, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'package:kernel/ast.dart';
|
|
|
|
// Extend references with flags to more easily identify getters and setters.
|
|
|
|
extension GetterSetterReference on Reference {
|
|
bool get isImplicitGetter {
|
|
Member member = asMember;
|
|
return member is Field && member.getterReference == this;
|
|
}
|
|
|
|
bool get isImplicitSetter {
|
|
Member member = asMember;
|
|
return member is Field && member.setterReference == this;
|
|
}
|
|
|
|
bool get isGetter {
|
|
Member member = asMember;
|
|
return (member is Procedure && member.isGetter) || isImplicitGetter;
|
|
}
|
|
|
|
bool get isSetter {
|
|
Member member = asMember;
|
|
return (member is Procedure && member.isSetter) || isImplicitSetter;
|
|
}
|
|
}
|
|
|
|
// Extend procedures with a tearOffReference that refers to the tear-off
|
|
// implementation for that procedure. This enables a Reference to refer to any
|
|
// implementation relating to a member, including its tear-off, which it can't
|
|
// do in plain kernel.
|
|
|
|
// Use Expandos to avoid keeping the procedure alive.
|
|
final Expando<Reference> _tearOffReference = Expando();
|
|
final Expando<Reference> _typeCheckerReference = Expando();
|
|
final Expando<Reference> _initializerReference = Expando();
|
|
final Expando<Reference> _constructorBodyReference = Expando();
|
|
|
|
extension CustomReference on Member {
|
|
Reference get tearOffReference =>
|
|
_tearOffReference[this] ??= Reference()..node = this;
|
|
|
|
Reference get typeCheckerReference =>
|
|
_typeCheckerReference[this] ??= Reference()..node = this;
|
|
|
|
Reference get initializerReference =>
|
|
_initializerReference[this] ??= Reference()..node = this;
|
|
|
|
Reference get constructorBodyReference =>
|
|
_constructorBodyReference[this] ??= Reference()..node = this;
|
|
}
|
|
|
|
extension IsCustomReference on Reference {
|
|
bool get isTearOffReference => _tearOffReference[asMember] == this;
|
|
|
|
bool get isTypeCheckerReference => _typeCheckerReference[asMember] == this;
|
|
|
|
bool get isInitializerReference => _initializerReference[asMember] == this;
|
|
|
|
bool get isConstructorBodyReference =>
|
|
_constructorBodyReference[asMember] == this;
|
|
}
|
|
|
|
extension ReferenceAs on Member {
|
|
Reference referenceAs({required bool getter, required bool setter}) {
|
|
assert(!getter || !setter); // members cannot be both setter and getter
|
|
Member member = this;
|
|
return member is Field
|
|
? setter
|
|
? member.setterReference!
|
|
: member.getterReference
|
|
: getter && member is Procedure && member.kind == ProcedureKind.Method
|
|
? member.tearOffReference
|
|
: member.reference;
|
|
}
|
|
}
|