mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
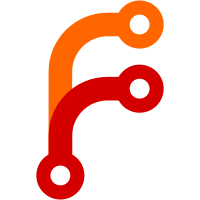
* Migrate to python3; drop python support. * Update Windows toolchain support. * Remove some unused methods. * Python 2.7 is still needed on Windows. * Update gsutil to a version that supports python3. Fixes: https://github.com/dart-lang/sdk/issues/28793 TEST=Manually tested common user journeys. Change-Id: I663a22b237a548bb82dc2e601e399e3bc3649211 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/192182 Reviewed-by: William Hesse <whesse@google.com> Reviewed-by: Alexander Aprelev <aam@google.com>
55 lines
1.9 KiB
Python
Executable file
55 lines
1.9 KiB
Python
Executable file
#!/usr/bin/env python3
|
|
# Copyright (c) 2016, the Dart project authors. Please see the AUTHORS file
|
|
# for details. All rights reserved. Use of this source code is governed by a
|
|
# BSD-style license that can be found in the LICENSE file.
|
|
"""Tool for listing Dart source files.
|
|
|
|
If the first argument is 'relative', the script produces paths relative to the
|
|
current working directory. If the first argument is 'absolute', the script
|
|
produces absolute paths.
|
|
|
|
Usage:
|
|
python3 tools/list_dart_files.py {absolute, relative} <directory> <pattern>
|
|
"""
|
|
|
|
import os
|
|
import re
|
|
import sys
|
|
|
|
|
|
def main(argv):
|
|
mode = argv[1]
|
|
if mode not in ['absolute', 'relative']:
|
|
raise Exception("First argument must be 'absolute' or 'relative'")
|
|
directory = argv[2]
|
|
if mode in 'absolute' and not os.path.isabs(directory):
|
|
directory = os.path.realpath(directory)
|
|
|
|
pattern = None
|
|
if len(argv) > 3:
|
|
pattern = re.compile(argv[3])
|
|
|
|
for root, directories, files in os.walk(directory):
|
|
# We only care about actual source files, not generated code or tests.
|
|
for skip_dir in ['.git', 'gen', 'test']:
|
|
if skip_dir in directories:
|
|
directories.remove(skip_dir)
|
|
|
|
# If we are looking at the root directory, filter the immediate
|
|
# subdirectories by the given pattern.
|
|
if pattern and root == directory:
|
|
directories[:] = filter(pattern.match, directories)
|
|
|
|
for filename in files:
|
|
if filename.endswith(
|
|
'.dart') and not filename.endswith('_test.dart'):
|
|
if mode in 'absolute':
|
|
fullname = os.path.join(directory, root, filename)
|
|
else:
|
|
fullname = os.path.relpath(os.path.join(root, filename))
|
|
fullname = fullname.replace(os.sep, '/')
|
|
print(fullname)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
sys.exit(main(sys.argv))
|