mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 12:24:24 +00:00
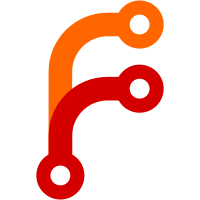
* Migrate to python3; drop python support. * Update Windows toolchain support. * Remove some unused methods. * Python 2.7 is still needed on Windows. * Update gsutil to a version that supports python3. Fixes: https://github.com/dart-lang/sdk/issues/28793 TEST=Manually tested common user journeys. Change-Id: I663a22b237a548bb82dc2e601e399e3bc3649211 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/192182 Reviewed-by: William Hesse <whesse@google.com> Reviewed-by: Alexander Aprelev <aam@google.com>
48 lines
1.6 KiB
Python
Executable file
48 lines
1.6 KiB
Python
Executable file
#!/usr/bin/env python3
|
|
# Copyright (c) 2021, the Dart project authors. Please see the AUTHORS file
|
|
# for details. All rights reserved. Use of this source code is governed by a
|
|
# BSD-style license that can be found in the LICENSE file.
|
|
#
|
|
# This python script is a wrapper around `git rev-parse --resolve-git-dir`.
|
|
# This is used for the build system to work with git worktrees.
|
|
|
|
import sys
|
|
import subprocess
|
|
import utils
|
|
|
|
|
|
def main():
|
|
try:
|
|
if len(sys.argv) != 3:
|
|
raise Exception('Expects exactly 2 arguments.')
|
|
args = ['git', 'rev-parse', '--resolve-git-dir', sys.argv[1]]
|
|
|
|
windows = utils.GuessOS() == 'win32'
|
|
if windows:
|
|
process = subprocess.Popen(args,
|
|
stdout=subprocess.PIPE,
|
|
stderr=subprocess.PIPE,
|
|
stdin=subprocess.PIPE,
|
|
shell=True,
|
|
universal_newlines=True)
|
|
else:
|
|
process = subprocess.Popen(args,
|
|
stdout=subprocess.PIPE,
|
|
stderr=subprocess.PIPE,
|
|
stdin=subprocess.PIPE,
|
|
shell=False,
|
|
universal_newlines=True)
|
|
|
|
outs, _ = process.communicate()
|
|
|
|
if process.returncode != 0:
|
|
raise Exception('Got non-0 exit code from git.')
|
|
|
|
print(outs.strip())
|
|
except:
|
|
# Fall back to fall-back path.
|
|
print(sys.argv[2])
|
|
|
|
|
|
if __name__ == '__main__':
|
|
sys.exit(main())
|