mirror of
https://github.com/dart-lang/sdk
synced 2024-09-15 23:39:48 +00:00
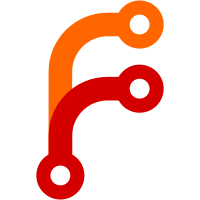
The ffi-callback related information on the [Thread] object is metadata corresponding to ffi-callback-trampoline [Function] objects. There is nothing thread or isolate specific about it. Moving it away from [Thread] is needed because an [Isolate] can have different [Thread] objects across its lifetime (see [0]): When the stack of an isolate is empty, we reserve now the right to re-cycle the [Thread]. If the isolate later runs again, it may get a new [Thread] object. This CL moves this information from [Thread] to the [ObjectStore]. In addition we make the compiler be responsible for populating this metadata - instead of doing this per-call site of `Pointer.fromFunction()`. It will be preserved across snapshot writing & snapshot reading (for AppJIT as well as AppAOT). Similarly the JIT trampolines that are on Isolate aren't isolate specific and can go to [IsolateGroup]. This simplifies doing the above as the compiler can allocate those as well. The effect is that [Thread] object gets smaller, GC doesn't have to visit the 2 slots per-thread. It comes at expense of 2 more loads when invoking the callback. [0] https://dart-review.googlesource.com/c/sdk/+/297920 TEST=Regression test is vm/ffi{,_2}/invoke_callback_after_suspension_test Change-Id: Ifde46a9f6e79819b5c0e359c3d3998d1d93b9b1e Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/303700 Reviewed-by: Daco Harkes <dacoharkes@google.com> Reviewed-by: Liam Appelbe <liama@google.com> Reviewed-by: Ryan Macnak <rmacnak@google.com> Commit-Queue: Martin Kustermann <kustermann@google.com>
42 lines
1.2 KiB
Dart
42 lines
1.2 KiB
Dart
// Copyright (c) 2023, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// @dart = 2.9
|
|
|
|
// SharedObjects=ffi_test_functions
|
|
|
|
import 'dart:async';
|
|
import 'dart:isolate';
|
|
import 'dart:ffi';
|
|
|
|
import 'callback_tests_utils.dart';
|
|
|
|
typedef SimpleAdditionType = Int32 Function(Int32, Int32);
|
|
int simpleAddition(int x, int y) {
|
|
print("simpleAddition($x, $y)");
|
|
return x + y;
|
|
}
|
|
|
|
void main() async {
|
|
// The main isolate is very special and cannot be suspended (due to having an
|
|
// active api scope throughout it's lifetime), so we run this test in a helper
|
|
// isolate.
|
|
const int count = 50;
|
|
final futures = <Future>[];
|
|
for (int i = 0; i < count; ++i) {
|
|
futures.add(Isolate.run(() async {
|
|
// First make the callback pointer.
|
|
final callbackFunctionPointer =
|
|
Pointer.fromFunction<SimpleAdditionType>(simpleAddition, 0);
|
|
|
|
// Then cause suspenion of [Thread].
|
|
await Future.delayed(const Duration(seconds: 1));
|
|
|
|
// Then make use of callback.
|
|
CallbackTest("SimpleAddition", callbackFunctionPointer).run();
|
|
}));
|
|
}
|
|
await Future.wait(futures);
|
|
}
|