mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 08:44:27 +00:00
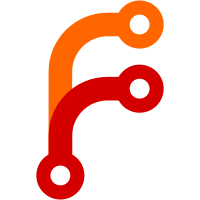
TEST=build VM Closes: https://github.com/dart-lang/sdk/pull/50862 GitOrigin-RevId: ae54b37666f70f670ee3af11c984c7e9a7e9da26 Change-Id: I459fda0439a1cd368f488a70d84ee6bb915e60bb Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/277761 Commit-Queue: Martin Kustermann <kustermann@google.com> Reviewed-by: Daco Harkes <dacoharkes@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com>
1629 lines
34 KiB
Dart
1629 lines
34 KiB
Dart
// Copyright (c) 2020, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
//
|
|
// This file has been automatically generated. Please do not edit it manually.
|
|
// Generated by tests/ffi/generator/structs_by_value_tests_generator.dart.
|
|
//
|
|
// SharedObjects=ffi_test_functions
|
|
// VMOptions=
|
|
// VMOptions=--deterministic --optimization-counter-threshold=90
|
|
// VMOptions=--use-slow-path
|
|
// VMOptions=--use-slow-path --stacktrace-every=100
|
|
|
|
import 'dart:ffi';
|
|
|
|
import "package:expect/expect.dart";
|
|
import "package:ffi/ffi.dart";
|
|
|
|
import 'dylib_utils.dart';
|
|
|
|
// Reuse the compound classes.
|
|
import 'function_structs_by_value_generated_compounds.dart';
|
|
|
|
final ffiTestFunctions = dlopenPlatformSpecific("ffi_test_functions");
|
|
void main() {
|
|
for (int i = 0; i < 100; ++i) {
|
|
testReturnStruct1ByteInt();
|
|
testReturnStruct3BytesHomogeneousUint8();
|
|
testReturnStruct3BytesInt2ByteAligned();
|
|
testReturnStruct4BytesHomogeneousInt16();
|
|
testReturnStruct7BytesHomogeneousUint8();
|
|
testReturnStruct7BytesInt4ByteAligned();
|
|
testReturnStruct8BytesInt();
|
|
testReturnStruct8BytesHomogeneousFloat();
|
|
testReturnStruct8BytesMixed();
|
|
testReturnStruct9BytesHomogeneousUint8();
|
|
testReturnStruct9BytesInt4Or8ByteAligned();
|
|
testReturnStruct12BytesHomogeneousFloat();
|
|
testReturnStruct16BytesHomogeneousFloat();
|
|
testReturnStruct16BytesMixed();
|
|
testReturnStruct16BytesMixed2();
|
|
testReturnStruct17BytesInt();
|
|
testReturnStruct19BytesHomogeneousUint8();
|
|
testReturnStruct20BytesHomogeneousInt32();
|
|
testReturnStruct20BytesHomogeneousFloat();
|
|
testReturnStruct32BytesHomogeneousDouble();
|
|
testReturnStruct40BytesHomogeneousDouble();
|
|
testReturnStruct1024BytesHomogeneousUint64();
|
|
testReturnStruct3BytesPackedInt();
|
|
testReturnStruct8BytesPackedInt();
|
|
testReturnStruct9BytesPackedMixed();
|
|
testReturnUnion4BytesMixed();
|
|
testReturnUnion8BytesNestedFloat();
|
|
testReturnUnion9BytesNestedInt();
|
|
testReturnUnion16BytesNestedFloat();
|
|
}
|
|
}
|
|
|
|
final returnStruct1ByteInt = ffiTestFunctions.lookupFunction<
|
|
Struct1ByteInt Function(Int8),
|
|
Struct1ByteInt Function(int)>("ReturnStruct1ByteInt");
|
|
|
|
/// Smallest struct with data.
|
|
void testReturnStruct1ByteInt() {
|
|
int a0;
|
|
|
|
a0 = -1;
|
|
|
|
final result = returnStruct1ByteInt(a0);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
}
|
|
|
|
final returnStruct3BytesHomogeneousUint8 = ffiTestFunctions.lookupFunction<
|
|
Struct3BytesHomogeneousUint8 Function(Uint8, Uint8, Uint8),
|
|
Struct3BytesHomogeneousUint8 Function(
|
|
int, int, int)>("ReturnStruct3BytesHomogeneousUint8");
|
|
|
|
/// Smaller than word size return value on all architectures.
|
|
void testReturnStruct3BytesHomogeneousUint8() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
|
|
a0 = 1;
|
|
a1 = 2;
|
|
a2 = 3;
|
|
|
|
final result = returnStruct3BytesHomogeneousUint8(a0, a1, a2);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
}
|
|
|
|
final returnStruct3BytesInt2ByteAligned = ffiTestFunctions.lookupFunction<
|
|
Struct3BytesInt2ByteAligned Function(Int16, Int8),
|
|
Struct3BytesInt2ByteAligned Function(
|
|
int, int)>("ReturnStruct3BytesInt2ByteAligned");
|
|
|
|
/// Smaller than word size return value on all architectures.
|
|
/// With alignment rules taken into account size is 4 bytes.
|
|
void testReturnStruct3BytesInt2ByteAligned() {
|
|
int a0;
|
|
int a1;
|
|
|
|
a0 = -1;
|
|
a1 = 2;
|
|
|
|
final result = returnStruct3BytesInt2ByteAligned(a0, a1);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
}
|
|
|
|
final returnStruct4BytesHomogeneousInt16 = ffiTestFunctions.lookupFunction<
|
|
Struct4BytesHomogeneousInt16 Function(Int16, Int16),
|
|
Struct4BytesHomogeneousInt16 Function(
|
|
int, int)>("ReturnStruct4BytesHomogeneousInt16");
|
|
|
|
/// Word size return value on 32 bit architectures..
|
|
void testReturnStruct4BytesHomogeneousInt16() {
|
|
int a0;
|
|
int a1;
|
|
|
|
a0 = -1;
|
|
a1 = 2;
|
|
|
|
final result = returnStruct4BytesHomogeneousInt16(a0, a1);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
}
|
|
|
|
final returnStruct7BytesHomogeneousUint8 = ffiTestFunctions.lookupFunction<
|
|
Struct7BytesHomogeneousUint8 Function(
|
|
Uint8, Uint8, Uint8, Uint8, Uint8, Uint8, Uint8),
|
|
Struct7BytesHomogeneousUint8 Function(int, int, int, int, int, int,
|
|
int)>("ReturnStruct7BytesHomogeneousUint8");
|
|
|
|
/// Non-wordsize return value.
|
|
void testReturnStruct7BytesHomogeneousUint8() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
int a3;
|
|
int a4;
|
|
int a5;
|
|
int a6;
|
|
|
|
a0 = 1;
|
|
a1 = 2;
|
|
a2 = 3;
|
|
a3 = 4;
|
|
a4 = 5;
|
|
a5 = 6;
|
|
a6 = 7;
|
|
|
|
final result = returnStruct7BytesHomogeneousUint8(a0, a1, a2, a3, a4, a5, a6);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
Expect.equals(a3, result.a3);
|
|
Expect.equals(a4, result.a4);
|
|
Expect.equals(a5, result.a5);
|
|
Expect.equals(a6, result.a6);
|
|
}
|
|
|
|
final returnStruct7BytesInt4ByteAligned = ffiTestFunctions.lookupFunction<
|
|
Struct7BytesInt4ByteAligned Function(Int32, Int16, Int8),
|
|
Struct7BytesInt4ByteAligned Function(
|
|
int, int, int)>("ReturnStruct7BytesInt4ByteAligned");
|
|
|
|
/// Non-wordsize return value.
|
|
/// With alignment rules taken into account size is 8 bytes.
|
|
void testReturnStruct7BytesInt4ByteAligned() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
|
|
a0 = -1;
|
|
a1 = 2;
|
|
a2 = -3;
|
|
|
|
final result = returnStruct7BytesInt4ByteAligned(a0, a1, a2);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
}
|
|
|
|
final returnStruct8BytesInt = ffiTestFunctions.lookupFunction<
|
|
Struct8BytesInt Function(Int16, Int16, Int32),
|
|
Struct8BytesInt Function(int, int, int)>("ReturnStruct8BytesInt");
|
|
|
|
/// Return value in integer registers on many architectures.
|
|
void testReturnStruct8BytesInt() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
|
|
a0 = -1;
|
|
a1 = 2;
|
|
a2 = -3;
|
|
|
|
final result = returnStruct8BytesInt(a0, a1, a2);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
}
|
|
|
|
final returnStruct8BytesHomogeneousFloat = ffiTestFunctions.lookupFunction<
|
|
Struct8BytesHomogeneousFloat Function(Float, Float),
|
|
Struct8BytesHomogeneousFloat Function(
|
|
double, double)>("ReturnStruct8BytesHomogeneousFloat");
|
|
|
|
/// Return value in FP registers on many architectures.
|
|
void testReturnStruct8BytesHomogeneousFloat() {
|
|
double a0;
|
|
double a1;
|
|
|
|
a0 = -1.0;
|
|
a1 = 2.0;
|
|
|
|
final result = returnStruct8BytesHomogeneousFloat(a0, a1);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
Expect.approxEquals(a1, result.a1);
|
|
}
|
|
|
|
final returnStruct8BytesMixed = ffiTestFunctions.lookupFunction<
|
|
Struct8BytesMixed Function(Float, Int16, Int16),
|
|
Struct8BytesMixed Function(double, int, int)>("ReturnStruct8BytesMixed");
|
|
|
|
/// Return value split over FP and integer register in x64.
|
|
void testReturnStruct8BytesMixed() {
|
|
double a0;
|
|
int a1;
|
|
int a2;
|
|
|
|
a0 = -1.0;
|
|
a1 = 2;
|
|
a2 = -3;
|
|
|
|
final result = returnStruct8BytesMixed(a0, a1, a2);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
}
|
|
|
|
final returnStruct9BytesHomogeneousUint8 = ffiTestFunctions.lookupFunction<
|
|
Struct9BytesHomogeneousUint8 Function(
|
|
Uint8, Uint8, Uint8, Uint8, Uint8, Uint8, Uint8, Uint8, Uint8),
|
|
Struct9BytesHomogeneousUint8 Function(int, int, int, int, int, int, int,
|
|
int, int)>("ReturnStruct9BytesHomogeneousUint8");
|
|
|
|
/// The minimum alignment of this struct is only 1 byte based on its fields.
|
|
/// Test that the memory backing these structs is the right size and that
|
|
/// dart:ffi trampolines do not write outside this size.
|
|
void testReturnStruct9BytesHomogeneousUint8() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
int a3;
|
|
int a4;
|
|
int a5;
|
|
int a6;
|
|
int a7;
|
|
int a8;
|
|
|
|
a0 = 1;
|
|
a1 = 2;
|
|
a2 = 3;
|
|
a3 = 4;
|
|
a4 = 5;
|
|
a5 = 6;
|
|
a6 = 7;
|
|
a7 = 8;
|
|
a8 = 9;
|
|
|
|
final result =
|
|
returnStruct9BytesHomogeneousUint8(a0, a1, a2, a3, a4, a5, a6, a7, a8);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
Expect.equals(a3, result.a3);
|
|
Expect.equals(a4, result.a4);
|
|
Expect.equals(a5, result.a5);
|
|
Expect.equals(a6, result.a6);
|
|
Expect.equals(a7, result.a7);
|
|
Expect.equals(a8, result.a8);
|
|
}
|
|
|
|
final returnStruct9BytesInt4Or8ByteAligned = ffiTestFunctions.lookupFunction<
|
|
Struct9BytesInt4Or8ByteAligned Function(Int64, Int8),
|
|
Struct9BytesInt4Or8ByteAligned Function(
|
|
int, int)>("ReturnStruct9BytesInt4Or8ByteAligned");
|
|
|
|
/// Return value in two integer registers on x64.
|
|
/// With alignment rules taken into account size is 12 or 16 bytes.
|
|
void testReturnStruct9BytesInt4Or8ByteAligned() {
|
|
int a0;
|
|
int a1;
|
|
|
|
a0 = -1;
|
|
a1 = 2;
|
|
|
|
final result = returnStruct9BytesInt4Or8ByteAligned(a0, a1);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
}
|
|
|
|
final returnStruct12BytesHomogeneousFloat = ffiTestFunctions.lookupFunction<
|
|
Struct12BytesHomogeneousFloat Function(Float, Float, Float),
|
|
Struct12BytesHomogeneousFloat Function(
|
|
double, double, double)>("ReturnStruct12BytesHomogeneousFloat");
|
|
|
|
/// Return value in FPU registers, but does not use all registers on arm hardfp
|
|
/// and arm64.
|
|
void testReturnStruct12BytesHomogeneousFloat() {
|
|
double a0;
|
|
double a1;
|
|
double a2;
|
|
|
|
a0 = -1.0;
|
|
a1 = 2.0;
|
|
a2 = -3.0;
|
|
|
|
final result = returnStruct12BytesHomogeneousFloat(a0, a1, a2);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
Expect.approxEquals(a1, result.a1);
|
|
Expect.approxEquals(a2, result.a2);
|
|
}
|
|
|
|
final returnStruct16BytesHomogeneousFloat = ffiTestFunctions.lookupFunction<
|
|
Struct16BytesHomogeneousFloat Function(Float, Float, Float, Float),
|
|
Struct16BytesHomogeneousFloat Function(
|
|
double, double, double, double)>("ReturnStruct16BytesHomogeneousFloat");
|
|
|
|
/// Return value in FPU registers on arm hardfp and arm64.
|
|
void testReturnStruct16BytesHomogeneousFloat() {
|
|
double a0;
|
|
double a1;
|
|
double a2;
|
|
double a3;
|
|
|
|
a0 = -1.0;
|
|
a1 = 2.0;
|
|
a2 = -3.0;
|
|
a3 = 4.0;
|
|
|
|
final result = returnStruct16BytesHomogeneousFloat(a0, a1, a2, a3);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
Expect.approxEquals(a1, result.a1);
|
|
Expect.approxEquals(a2, result.a2);
|
|
Expect.approxEquals(a3, result.a3);
|
|
}
|
|
|
|
final returnStruct16BytesMixed = ffiTestFunctions.lookupFunction<
|
|
Struct16BytesMixed Function(Double, Int64),
|
|
Struct16BytesMixed Function(double, int)>("ReturnStruct16BytesMixed");
|
|
|
|
/// Return value split over FP and integer register in x64.
|
|
void testReturnStruct16BytesMixed() {
|
|
double a0;
|
|
int a1;
|
|
|
|
a0 = -1.0;
|
|
a1 = 2;
|
|
|
|
final result = returnStruct16BytesMixed(a0, a1);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
}
|
|
|
|
final returnStruct16BytesMixed2 = ffiTestFunctions.lookupFunction<
|
|
Struct16BytesMixed2 Function(Float, Float, Float, Int32),
|
|
Struct16BytesMixed2 Function(
|
|
double, double, double, int)>("ReturnStruct16BytesMixed2");
|
|
|
|
/// Return value split over FP and integer register in x64.
|
|
/// The integer register contains half float half int.
|
|
void testReturnStruct16BytesMixed2() {
|
|
double a0;
|
|
double a1;
|
|
double a2;
|
|
int a3;
|
|
|
|
a0 = -1.0;
|
|
a1 = 2.0;
|
|
a2 = -3.0;
|
|
a3 = 4;
|
|
|
|
final result = returnStruct16BytesMixed2(a0, a1, a2, a3);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
Expect.approxEquals(a1, result.a1);
|
|
Expect.approxEquals(a2, result.a2);
|
|
Expect.equals(a3, result.a3);
|
|
}
|
|
|
|
final returnStruct17BytesInt = ffiTestFunctions.lookupFunction<
|
|
Struct17BytesInt Function(Int64, Int64, Int8),
|
|
Struct17BytesInt Function(int, int, int)>("ReturnStruct17BytesInt");
|
|
|
|
/// Return value returned in preallocated space passed by pointer on most ABIs.
|
|
/// Is non word size on purpose, to test that structs are rounded up to word size
|
|
/// on all ABIs.
|
|
void testReturnStruct17BytesInt() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
|
|
a0 = -1;
|
|
a1 = 2;
|
|
a2 = -3;
|
|
|
|
final result = returnStruct17BytesInt(a0, a1, a2);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
}
|
|
|
|
final returnStruct19BytesHomogeneousUint8 = ffiTestFunctions.lookupFunction<
|
|
Struct19BytesHomogeneousUint8 Function(
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8,
|
|
Uint8),
|
|
Struct19BytesHomogeneousUint8 Function(
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int)>("ReturnStruct19BytesHomogeneousUint8");
|
|
|
|
/// The minimum alignment of this struct is only 1 byte based on its fields.
|
|
/// Test that the memory backing these structs is the right size and that
|
|
/// dart:ffi trampolines do not write outside this size.
|
|
void testReturnStruct19BytesHomogeneousUint8() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
int a3;
|
|
int a4;
|
|
int a5;
|
|
int a6;
|
|
int a7;
|
|
int a8;
|
|
int a9;
|
|
int a10;
|
|
int a11;
|
|
int a12;
|
|
int a13;
|
|
int a14;
|
|
int a15;
|
|
int a16;
|
|
int a17;
|
|
int a18;
|
|
|
|
a0 = 1;
|
|
a1 = 2;
|
|
a2 = 3;
|
|
a3 = 4;
|
|
a4 = 5;
|
|
a5 = 6;
|
|
a6 = 7;
|
|
a7 = 8;
|
|
a8 = 9;
|
|
a9 = 10;
|
|
a10 = 11;
|
|
a11 = 12;
|
|
a12 = 13;
|
|
a13 = 14;
|
|
a14 = 15;
|
|
a15 = 16;
|
|
a16 = 17;
|
|
a17 = 18;
|
|
a18 = 19;
|
|
|
|
final result = returnStruct19BytesHomogeneousUint8(a0, a1, a2, a3, a4, a5, a6,
|
|
a7, a8, a9, a10, a11, a12, a13, a14, a15, a16, a17, a18);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
Expect.equals(a3, result.a3);
|
|
Expect.equals(a4, result.a4);
|
|
Expect.equals(a5, result.a5);
|
|
Expect.equals(a6, result.a6);
|
|
Expect.equals(a7, result.a7);
|
|
Expect.equals(a8, result.a8);
|
|
Expect.equals(a9, result.a9);
|
|
Expect.equals(a10, result.a10);
|
|
Expect.equals(a11, result.a11);
|
|
Expect.equals(a12, result.a12);
|
|
Expect.equals(a13, result.a13);
|
|
Expect.equals(a14, result.a14);
|
|
Expect.equals(a15, result.a15);
|
|
Expect.equals(a16, result.a16);
|
|
Expect.equals(a17, result.a17);
|
|
Expect.equals(a18, result.a18);
|
|
}
|
|
|
|
final returnStruct20BytesHomogeneousInt32 = ffiTestFunctions.lookupFunction<
|
|
Struct20BytesHomogeneousInt32 Function(Int32, Int32, Int32, Int32, Int32),
|
|
Struct20BytesHomogeneousInt32 Function(
|
|
int, int, int, int, int)>("ReturnStruct20BytesHomogeneousInt32");
|
|
|
|
/// Return value too big to go in cpu registers on arm64.
|
|
void testReturnStruct20BytesHomogeneousInt32() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
int a3;
|
|
int a4;
|
|
|
|
a0 = -1;
|
|
a1 = 2;
|
|
a2 = -3;
|
|
a3 = 4;
|
|
a4 = -5;
|
|
|
|
final result = returnStruct20BytesHomogeneousInt32(a0, a1, a2, a3, a4);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
Expect.equals(a3, result.a3);
|
|
Expect.equals(a4, result.a4);
|
|
}
|
|
|
|
final returnStruct20BytesHomogeneousFloat = ffiTestFunctions.lookupFunction<
|
|
Struct20BytesHomogeneousFloat Function(Float, Float, Float, Float, Float),
|
|
Struct20BytesHomogeneousFloat Function(double, double, double, double,
|
|
double)>("ReturnStruct20BytesHomogeneousFloat");
|
|
|
|
/// Return value too big to go in FPU registers on x64, arm hardfp and arm64.
|
|
void testReturnStruct20BytesHomogeneousFloat() {
|
|
double a0;
|
|
double a1;
|
|
double a2;
|
|
double a3;
|
|
double a4;
|
|
|
|
a0 = -1.0;
|
|
a1 = 2.0;
|
|
a2 = -3.0;
|
|
a3 = 4.0;
|
|
a4 = -5.0;
|
|
|
|
final result = returnStruct20BytesHomogeneousFloat(a0, a1, a2, a3, a4);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
Expect.approxEquals(a1, result.a1);
|
|
Expect.approxEquals(a2, result.a2);
|
|
Expect.approxEquals(a3, result.a3);
|
|
Expect.approxEquals(a4, result.a4);
|
|
}
|
|
|
|
final returnStruct32BytesHomogeneousDouble = ffiTestFunctions.lookupFunction<
|
|
Struct32BytesHomogeneousDouble Function(Double, Double, Double, Double),
|
|
Struct32BytesHomogeneousDouble Function(double, double, double,
|
|
double)>("ReturnStruct32BytesHomogeneousDouble");
|
|
|
|
/// Return value in FPU registers on arm64.
|
|
void testReturnStruct32BytesHomogeneousDouble() {
|
|
double a0;
|
|
double a1;
|
|
double a2;
|
|
double a3;
|
|
|
|
a0 = -1.0;
|
|
a1 = 2.0;
|
|
a2 = -3.0;
|
|
a3 = 4.0;
|
|
|
|
final result = returnStruct32BytesHomogeneousDouble(a0, a1, a2, a3);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
Expect.approxEquals(a1, result.a1);
|
|
Expect.approxEquals(a2, result.a2);
|
|
Expect.approxEquals(a3, result.a3);
|
|
}
|
|
|
|
final returnStruct40BytesHomogeneousDouble = ffiTestFunctions.lookupFunction<
|
|
Struct40BytesHomogeneousDouble Function(
|
|
Double, Double, Double, Double, Double),
|
|
Struct40BytesHomogeneousDouble Function(double, double, double, double,
|
|
double)>("ReturnStruct40BytesHomogeneousDouble");
|
|
|
|
/// Return value too big to go in FPU registers on arm64.
|
|
void testReturnStruct40BytesHomogeneousDouble() {
|
|
double a0;
|
|
double a1;
|
|
double a2;
|
|
double a3;
|
|
double a4;
|
|
|
|
a0 = -1.0;
|
|
a1 = 2.0;
|
|
a2 = -3.0;
|
|
a3 = 4.0;
|
|
a4 = -5.0;
|
|
|
|
final result = returnStruct40BytesHomogeneousDouble(a0, a1, a2, a3, a4);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
Expect.approxEquals(a1, result.a1);
|
|
Expect.approxEquals(a2, result.a2);
|
|
Expect.approxEquals(a3, result.a3);
|
|
Expect.approxEquals(a4, result.a4);
|
|
}
|
|
|
|
final returnStruct1024BytesHomogeneousUint64 = ffiTestFunctions.lookupFunction<
|
|
Struct1024BytesHomogeneousUint64 Function(
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64,
|
|
Uint64),
|
|
Struct1024BytesHomogeneousUint64 Function(
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int,
|
|
int)>("ReturnStruct1024BytesHomogeneousUint64");
|
|
|
|
/// Test 1kb struct.
|
|
void testReturnStruct1024BytesHomogeneousUint64() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
int a3;
|
|
int a4;
|
|
int a5;
|
|
int a6;
|
|
int a7;
|
|
int a8;
|
|
int a9;
|
|
int a10;
|
|
int a11;
|
|
int a12;
|
|
int a13;
|
|
int a14;
|
|
int a15;
|
|
int a16;
|
|
int a17;
|
|
int a18;
|
|
int a19;
|
|
int a20;
|
|
int a21;
|
|
int a22;
|
|
int a23;
|
|
int a24;
|
|
int a25;
|
|
int a26;
|
|
int a27;
|
|
int a28;
|
|
int a29;
|
|
int a30;
|
|
int a31;
|
|
int a32;
|
|
int a33;
|
|
int a34;
|
|
int a35;
|
|
int a36;
|
|
int a37;
|
|
int a38;
|
|
int a39;
|
|
int a40;
|
|
int a41;
|
|
int a42;
|
|
int a43;
|
|
int a44;
|
|
int a45;
|
|
int a46;
|
|
int a47;
|
|
int a48;
|
|
int a49;
|
|
int a50;
|
|
int a51;
|
|
int a52;
|
|
int a53;
|
|
int a54;
|
|
int a55;
|
|
int a56;
|
|
int a57;
|
|
int a58;
|
|
int a59;
|
|
int a60;
|
|
int a61;
|
|
int a62;
|
|
int a63;
|
|
int a64;
|
|
int a65;
|
|
int a66;
|
|
int a67;
|
|
int a68;
|
|
int a69;
|
|
int a70;
|
|
int a71;
|
|
int a72;
|
|
int a73;
|
|
int a74;
|
|
int a75;
|
|
int a76;
|
|
int a77;
|
|
int a78;
|
|
int a79;
|
|
int a80;
|
|
int a81;
|
|
int a82;
|
|
int a83;
|
|
int a84;
|
|
int a85;
|
|
int a86;
|
|
int a87;
|
|
int a88;
|
|
int a89;
|
|
int a90;
|
|
int a91;
|
|
int a92;
|
|
int a93;
|
|
int a94;
|
|
int a95;
|
|
int a96;
|
|
int a97;
|
|
int a98;
|
|
int a99;
|
|
int a100;
|
|
int a101;
|
|
int a102;
|
|
int a103;
|
|
int a104;
|
|
int a105;
|
|
int a106;
|
|
int a107;
|
|
int a108;
|
|
int a109;
|
|
int a110;
|
|
int a111;
|
|
int a112;
|
|
int a113;
|
|
int a114;
|
|
int a115;
|
|
int a116;
|
|
int a117;
|
|
int a118;
|
|
int a119;
|
|
int a120;
|
|
int a121;
|
|
int a122;
|
|
int a123;
|
|
int a124;
|
|
int a125;
|
|
int a126;
|
|
int a127;
|
|
|
|
a0 = 1;
|
|
a1 = 2;
|
|
a2 = 3;
|
|
a3 = 4;
|
|
a4 = 5;
|
|
a5 = 6;
|
|
a6 = 7;
|
|
a7 = 8;
|
|
a8 = 9;
|
|
a9 = 10;
|
|
a10 = 11;
|
|
a11 = 12;
|
|
a12 = 13;
|
|
a13 = 14;
|
|
a14 = 15;
|
|
a15 = 16;
|
|
a16 = 17;
|
|
a17 = 18;
|
|
a18 = 19;
|
|
a19 = 20;
|
|
a20 = 21;
|
|
a21 = 22;
|
|
a22 = 23;
|
|
a23 = 24;
|
|
a24 = 25;
|
|
a25 = 26;
|
|
a26 = 27;
|
|
a27 = 28;
|
|
a28 = 29;
|
|
a29 = 30;
|
|
a30 = 31;
|
|
a31 = 32;
|
|
a32 = 33;
|
|
a33 = 34;
|
|
a34 = 35;
|
|
a35 = 36;
|
|
a36 = 37;
|
|
a37 = 38;
|
|
a38 = 39;
|
|
a39 = 40;
|
|
a40 = 41;
|
|
a41 = 42;
|
|
a42 = 43;
|
|
a43 = 44;
|
|
a44 = 45;
|
|
a45 = 46;
|
|
a46 = 47;
|
|
a47 = 48;
|
|
a48 = 49;
|
|
a49 = 50;
|
|
a50 = 51;
|
|
a51 = 52;
|
|
a52 = 53;
|
|
a53 = 54;
|
|
a54 = 55;
|
|
a55 = 56;
|
|
a56 = 57;
|
|
a57 = 58;
|
|
a58 = 59;
|
|
a59 = 60;
|
|
a60 = 61;
|
|
a61 = 62;
|
|
a62 = 63;
|
|
a63 = 64;
|
|
a64 = 65;
|
|
a65 = 66;
|
|
a66 = 67;
|
|
a67 = 68;
|
|
a68 = 69;
|
|
a69 = 70;
|
|
a70 = 71;
|
|
a71 = 72;
|
|
a72 = 73;
|
|
a73 = 74;
|
|
a74 = 75;
|
|
a75 = 76;
|
|
a76 = 77;
|
|
a77 = 78;
|
|
a78 = 79;
|
|
a79 = 80;
|
|
a80 = 81;
|
|
a81 = 82;
|
|
a82 = 83;
|
|
a83 = 84;
|
|
a84 = 85;
|
|
a85 = 86;
|
|
a86 = 87;
|
|
a87 = 88;
|
|
a88 = 89;
|
|
a89 = 90;
|
|
a90 = 91;
|
|
a91 = 92;
|
|
a92 = 93;
|
|
a93 = 94;
|
|
a94 = 95;
|
|
a95 = 96;
|
|
a96 = 97;
|
|
a97 = 98;
|
|
a98 = 99;
|
|
a99 = 100;
|
|
a100 = 101;
|
|
a101 = 102;
|
|
a102 = 103;
|
|
a103 = 104;
|
|
a104 = 105;
|
|
a105 = 106;
|
|
a106 = 107;
|
|
a107 = 108;
|
|
a108 = 109;
|
|
a109 = 110;
|
|
a110 = 111;
|
|
a111 = 112;
|
|
a112 = 113;
|
|
a113 = 114;
|
|
a114 = 115;
|
|
a115 = 116;
|
|
a116 = 117;
|
|
a117 = 118;
|
|
a118 = 119;
|
|
a119 = 120;
|
|
a120 = 121;
|
|
a121 = 122;
|
|
a122 = 123;
|
|
a123 = 124;
|
|
a124 = 125;
|
|
a125 = 126;
|
|
a126 = 127;
|
|
a127 = 128;
|
|
|
|
final result = returnStruct1024BytesHomogeneousUint64(
|
|
a0,
|
|
a1,
|
|
a2,
|
|
a3,
|
|
a4,
|
|
a5,
|
|
a6,
|
|
a7,
|
|
a8,
|
|
a9,
|
|
a10,
|
|
a11,
|
|
a12,
|
|
a13,
|
|
a14,
|
|
a15,
|
|
a16,
|
|
a17,
|
|
a18,
|
|
a19,
|
|
a20,
|
|
a21,
|
|
a22,
|
|
a23,
|
|
a24,
|
|
a25,
|
|
a26,
|
|
a27,
|
|
a28,
|
|
a29,
|
|
a30,
|
|
a31,
|
|
a32,
|
|
a33,
|
|
a34,
|
|
a35,
|
|
a36,
|
|
a37,
|
|
a38,
|
|
a39,
|
|
a40,
|
|
a41,
|
|
a42,
|
|
a43,
|
|
a44,
|
|
a45,
|
|
a46,
|
|
a47,
|
|
a48,
|
|
a49,
|
|
a50,
|
|
a51,
|
|
a52,
|
|
a53,
|
|
a54,
|
|
a55,
|
|
a56,
|
|
a57,
|
|
a58,
|
|
a59,
|
|
a60,
|
|
a61,
|
|
a62,
|
|
a63,
|
|
a64,
|
|
a65,
|
|
a66,
|
|
a67,
|
|
a68,
|
|
a69,
|
|
a70,
|
|
a71,
|
|
a72,
|
|
a73,
|
|
a74,
|
|
a75,
|
|
a76,
|
|
a77,
|
|
a78,
|
|
a79,
|
|
a80,
|
|
a81,
|
|
a82,
|
|
a83,
|
|
a84,
|
|
a85,
|
|
a86,
|
|
a87,
|
|
a88,
|
|
a89,
|
|
a90,
|
|
a91,
|
|
a92,
|
|
a93,
|
|
a94,
|
|
a95,
|
|
a96,
|
|
a97,
|
|
a98,
|
|
a99,
|
|
a100,
|
|
a101,
|
|
a102,
|
|
a103,
|
|
a104,
|
|
a105,
|
|
a106,
|
|
a107,
|
|
a108,
|
|
a109,
|
|
a110,
|
|
a111,
|
|
a112,
|
|
a113,
|
|
a114,
|
|
a115,
|
|
a116,
|
|
a117,
|
|
a118,
|
|
a119,
|
|
a120,
|
|
a121,
|
|
a122,
|
|
a123,
|
|
a124,
|
|
a125,
|
|
a126,
|
|
a127);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
Expect.equals(a3, result.a3);
|
|
Expect.equals(a4, result.a4);
|
|
Expect.equals(a5, result.a5);
|
|
Expect.equals(a6, result.a6);
|
|
Expect.equals(a7, result.a7);
|
|
Expect.equals(a8, result.a8);
|
|
Expect.equals(a9, result.a9);
|
|
Expect.equals(a10, result.a10);
|
|
Expect.equals(a11, result.a11);
|
|
Expect.equals(a12, result.a12);
|
|
Expect.equals(a13, result.a13);
|
|
Expect.equals(a14, result.a14);
|
|
Expect.equals(a15, result.a15);
|
|
Expect.equals(a16, result.a16);
|
|
Expect.equals(a17, result.a17);
|
|
Expect.equals(a18, result.a18);
|
|
Expect.equals(a19, result.a19);
|
|
Expect.equals(a20, result.a20);
|
|
Expect.equals(a21, result.a21);
|
|
Expect.equals(a22, result.a22);
|
|
Expect.equals(a23, result.a23);
|
|
Expect.equals(a24, result.a24);
|
|
Expect.equals(a25, result.a25);
|
|
Expect.equals(a26, result.a26);
|
|
Expect.equals(a27, result.a27);
|
|
Expect.equals(a28, result.a28);
|
|
Expect.equals(a29, result.a29);
|
|
Expect.equals(a30, result.a30);
|
|
Expect.equals(a31, result.a31);
|
|
Expect.equals(a32, result.a32);
|
|
Expect.equals(a33, result.a33);
|
|
Expect.equals(a34, result.a34);
|
|
Expect.equals(a35, result.a35);
|
|
Expect.equals(a36, result.a36);
|
|
Expect.equals(a37, result.a37);
|
|
Expect.equals(a38, result.a38);
|
|
Expect.equals(a39, result.a39);
|
|
Expect.equals(a40, result.a40);
|
|
Expect.equals(a41, result.a41);
|
|
Expect.equals(a42, result.a42);
|
|
Expect.equals(a43, result.a43);
|
|
Expect.equals(a44, result.a44);
|
|
Expect.equals(a45, result.a45);
|
|
Expect.equals(a46, result.a46);
|
|
Expect.equals(a47, result.a47);
|
|
Expect.equals(a48, result.a48);
|
|
Expect.equals(a49, result.a49);
|
|
Expect.equals(a50, result.a50);
|
|
Expect.equals(a51, result.a51);
|
|
Expect.equals(a52, result.a52);
|
|
Expect.equals(a53, result.a53);
|
|
Expect.equals(a54, result.a54);
|
|
Expect.equals(a55, result.a55);
|
|
Expect.equals(a56, result.a56);
|
|
Expect.equals(a57, result.a57);
|
|
Expect.equals(a58, result.a58);
|
|
Expect.equals(a59, result.a59);
|
|
Expect.equals(a60, result.a60);
|
|
Expect.equals(a61, result.a61);
|
|
Expect.equals(a62, result.a62);
|
|
Expect.equals(a63, result.a63);
|
|
Expect.equals(a64, result.a64);
|
|
Expect.equals(a65, result.a65);
|
|
Expect.equals(a66, result.a66);
|
|
Expect.equals(a67, result.a67);
|
|
Expect.equals(a68, result.a68);
|
|
Expect.equals(a69, result.a69);
|
|
Expect.equals(a70, result.a70);
|
|
Expect.equals(a71, result.a71);
|
|
Expect.equals(a72, result.a72);
|
|
Expect.equals(a73, result.a73);
|
|
Expect.equals(a74, result.a74);
|
|
Expect.equals(a75, result.a75);
|
|
Expect.equals(a76, result.a76);
|
|
Expect.equals(a77, result.a77);
|
|
Expect.equals(a78, result.a78);
|
|
Expect.equals(a79, result.a79);
|
|
Expect.equals(a80, result.a80);
|
|
Expect.equals(a81, result.a81);
|
|
Expect.equals(a82, result.a82);
|
|
Expect.equals(a83, result.a83);
|
|
Expect.equals(a84, result.a84);
|
|
Expect.equals(a85, result.a85);
|
|
Expect.equals(a86, result.a86);
|
|
Expect.equals(a87, result.a87);
|
|
Expect.equals(a88, result.a88);
|
|
Expect.equals(a89, result.a89);
|
|
Expect.equals(a90, result.a90);
|
|
Expect.equals(a91, result.a91);
|
|
Expect.equals(a92, result.a92);
|
|
Expect.equals(a93, result.a93);
|
|
Expect.equals(a94, result.a94);
|
|
Expect.equals(a95, result.a95);
|
|
Expect.equals(a96, result.a96);
|
|
Expect.equals(a97, result.a97);
|
|
Expect.equals(a98, result.a98);
|
|
Expect.equals(a99, result.a99);
|
|
Expect.equals(a100, result.a100);
|
|
Expect.equals(a101, result.a101);
|
|
Expect.equals(a102, result.a102);
|
|
Expect.equals(a103, result.a103);
|
|
Expect.equals(a104, result.a104);
|
|
Expect.equals(a105, result.a105);
|
|
Expect.equals(a106, result.a106);
|
|
Expect.equals(a107, result.a107);
|
|
Expect.equals(a108, result.a108);
|
|
Expect.equals(a109, result.a109);
|
|
Expect.equals(a110, result.a110);
|
|
Expect.equals(a111, result.a111);
|
|
Expect.equals(a112, result.a112);
|
|
Expect.equals(a113, result.a113);
|
|
Expect.equals(a114, result.a114);
|
|
Expect.equals(a115, result.a115);
|
|
Expect.equals(a116, result.a116);
|
|
Expect.equals(a117, result.a117);
|
|
Expect.equals(a118, result.a118);
|
|
Expect.equals(a119, result.a119);
|
|
Expect.equals(a120, result.a120);
|
|
Expect.equals(a121, result.a121);
|
|
Expect.equals(a122, result.a122);
|
|
Expect.equals(a123, result.a123);
|
|
Expect.equals(a124, result.a124);
|
|
Expect.equals(a125, result.a125);
|
|
Expect.equals(a126, result.a126);
|
|
Expect.equals(a127, result.a127);
|
|
}
|
|
|
|
final returnStruct3BytesPackedInt = ffiTestFunctions.lookupFunction<
|
|
Struct3BytesPackedInt Function(Int8, Int16),
|
|
Struct3BytesPackedInt Function(int, int)>("ReturnStruct3BytesPackedInt");
|
|
|
|
/// Small struct with mis-aligned member.
|
|
void testReturnStruct3BytesPackedInt() {
|
|
int a0;
|
|
int a1;
|
|
|
|
a0 = -1;
|
|
a1 = 2;
|
|
|
|
final result = returnStruct3BytesPackedInt(a0, a1);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
}
|
|
|
|
final returnStruct8BytesPackedInt = ffiTestFunctions.lookupFunction<
|
|
Struct8BytesPackedInt Function(Uint8, Uint32, Uint8, Uint8, Uint8),
|
|
Struct8BytesPackedInt Function(
|
|
int, int, int, int, int)>("ReturnStruct8BytesPackedInt");
|
|
|
|
/// Struct with mis-aligned member.
|
|
void testReturnStruct8BytesPackedInt() {
|
|
int a0;
|
|
int a1;
|
|
int a2;
|
|
int a3;
|
|
int a4;
|
|
|
|
a0 = 1;
|
|
a1 = 2;
|
|
a2 = 3;
|
|
a3 = 4;
|
|
a4 = 5;
|
|
|
|
final result = returnStruct8BytesPackedInt(a0, a1, a2, a3, a4);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.equals(a1, result.a1);
|
|
Expect.equals(a2, result.a2);
|
|
Expect.equals(a3, result.a3);
|
|
Expect.equals(a4, result.a4);
|
|
}
|
|
|
|
final returnStruct9BytesPackedMixed = ffiTestFunctions.lookupFunction<
|
|
Struct9BytesPackedMixed Function(Uint8, Double),
|
|
Struct9BytesPackedMixed Function(
|
|
int, double)>("ReturnStruct9BytesPackedMixed");
|
|
|
|
/// Struct with mis-aligned member.
|
|
/// Tests backfilling of CPU and FPU registers.
|
|
void testReturnStruct9BytesPackedMixed() {
|
|
int a0;
|
|
double a1;
|
|
|
|
a0 = 1;
|
|
a1 = 2.0;
|
|
|
|
final result = returnStruct9BytesPackedMixed(a0, a1);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
Expect.approxEquals(a1, result.a1);
|
|
}
|
|
|
|
final returnUnion4BytesMixed = ffiTestFunctions.lookupFunction<
|
|
Union4BytesMixed Function(Uint32),
|
|
Union4BytesMixed Function(int)>("ReturnUnion4BytesMixed");
|
|
|
|
/// Returning a mixed integer/float union.
|
|
void testReturnUnion4BytesMixed() {
|
|
int a0;
|
|
|
|
a0 = 1;
|
|
|
|
final result = returnUnion4BytesMixed(a0);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0, result.a0);
|
|
}
|
|
|
|
final returnUnion8BytesNestedFloat = ffiTestFunctions.lookupFunction<
|
|
Union8BytesNestedFloat Function(Double),
|
|
Union8BytesNestedFloat Function(double)>("ReturnUnion8BytesNestedFloat");
|
|
|
|
/// Returning a floating point only union.
|
|
void testReturnUnion8BytesNestedFloat() {
|
|
double a0;
|
|
|
|
a0 = -1.0;
|
|
|
|
final result = returnUnion8BytesNestedFloat(a0);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0, result.a0);
|
|
}
|
|
|
|
final returnUnion9BytesNestedInt = ffiTestFunctions.lookupFunction<
|
|
Union9BytesNestedInt Function(Struct8BytesInt),
|
|
Union9BytesNestedInt Function(
|
|
Struct8BytesInt)>("ReturnUnion9BytesNestedInt");
|
|
|
|
/// Returning a mixed-size union.
|
|
void testReturnUnion9BytesNestedInt() {
|
|
final a0Pointer = calloc<Struct8BytesInt>();
|
|
final Struct8BytesInt a0 = a0Pointer.ref;
|
|
|
|
a0.a0 = -1;
|
|
a0.a1 = 2;
|
|
a0.a2 = -3;
|
|
|
|
final result = returnUnion9BytesNestedInt(a0);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.equals(a0.a0, result.a0.a0);
|
|
Expect.equals(a0.a1, result.a0.a1);
|
|
Expect.equals(a0.a2, result.a0.a2);
|
|
|
|
calloc.free(a0Pointer);
|
|
}
|
|
|
|
final returnUnion16BytesNestedFloat = ffiTestFunctions.lookupFunction<
|
|
Union16BytesNestedFloat Function(Struct8BytesHomogeneousFloat),
|
|
Union16BytesNestedFloat Function(
|
|
Struct8BytesHomogeneousFloat)>("ReturnUnion16BytesNestedFloat");
|
|
|
|
/// Returning union with homogenous floats.
|
|
void testReturnUnion16BytesNestedFloat() {
|
|
final a0Pointer = calloc<Struct8BytesHomogeneousFloat>();
|
|
final Struct8BytesHomogeneousFloat a0 = a0Pointer.ref;
|
|
|
|
a0.a0 = -1.0;
|
|
a0.a1 = 2.0;
|
|
|
|
final result = returnUnion16BytesNestedFloat(a0);
|
|
|
|
print("result = $result");
|
|
|
|
Expect.approxEquals(a0.a0, result.a0.a0);
|
|
Expect.approxEquals(a0.a1, result.a0.a1);
|
|
|
|
calloc.free(a0Pointer);
|
|
}
|