mirror of
https://github.com/dart-lang/sdk
synced 2024-09-15 22:59:47 +00:00
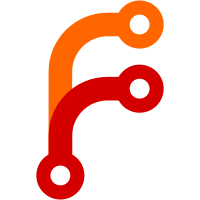
Also relands the followup CLs: "Perform non-covariant checks when dynamically invoking callables." "Use AreValidArguments so that names are checked as well." Original description of first CL: This change only affects compilation when running in non-precompiled mode with --no-lazy-dispatchers enabled. Instead of always compiling in non-covariant checks, even for closures not called dynamically, remove the non-covariant checks from the closure and instead do the non-covariant checks for dynamic calls during the NoSuchMethodForCallStub fallback by calling Function::DoArgumentTypesMatch. Adds two overloads for Function::DoArgumentTypesMatch, one which takes a function type argument vector and one which takes neither an instantiator type argument vector or a function type argument vector. For the versions that are not explicitly passed a type argument vector, an appropriate one is constructed using the arguments. If there is not enough information in the arguments, then we fall back to assuming the empty type argument vector for the instantiator case and instantiating to bounds in the function type argument case. Fixes Function::DoArgumentTypesMatch to handle generic functions and to check arguments appropriately according to the active null safety mode. For generic functions, the provided or resulting function type vector has non-covariant checks performed against the type parameter bounds. This change uncovered one test that was incorrectly passing in strong mode, see https://github.com/dart-lang/sdk/issues/42688 for details. Original description of second CL: The VM only does this when the callable function does not expect dynamic invocations. Otherwise, performing the checks would be redundant, as the function body already contains the appropriate non-covariant checks. Third CL had no additional description. Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-linux-debug-x64-try,vm-dartkb-linux-release-x64-try,vm-kernel-reload-linux-release-x64-try, vm-kernel-reload-rollback-linux-debug-x64-try Bug: https://github.com/dart-lang/sdk/issues/40813 Change-Id: I1a3e9c1865103a8d716e1cad814267caffaaadf2 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/154688 Reviewed-by: Martin Kustermann <kustermann@google.com>
90 lines
2.7 KiB
Dart
90 lines
2.7 KiB
Dart
// Copyright (c) 2017, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// VMOptions=--lazy-dispatchers
|
|
// VMOptions=--no-lazy-dispatchers
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
// Test that noSuchMethod calls behave as expected for dynamic object invocations.
|
|
class BaseClass {
|
|
final dynamic finalField = "final!";
|
|
|
|
baz() => "baz!!";
|
|
get bla => (() => "bla!!");
|
|
}
|
|
|
|
class ReturnInvocationName extends BaseClass {
|
|
var _bar;
|
|
|
|
ReturnInvocationName(this._bar);
|
|
|
|
noSuchMethod(Invocation invocation) {
|
|
var name = invocation.memberName.toString();
|
|
var match = new RegExp(r'Symbol\("([^"]+)"\)').matchAsPrefix(name);
|
|
return match != null ? match.group(1) : name;
|
|
}
|
|
|
|
bar() {
|
|
return _bar;
|
|
}
|
|
}
|
|
|
|
class Foo {}
|
|
|
|
main() {
|
|
dynamic x = new ReturnInvocationName(42);
|
|
Expect.equals('final!', x.finalField);
|
|
Expect.equals('foo', x.finalField = "foo", 'should call noSuchMethod');
|
|
Expect.equals('final!', x.finalField, 'field was not set');
|
|
|
|
Expect.equals('_prototype', x._prototype);
|
|
Expect.equals('_prototype', x._prototype());
|
|
|
|
Expect.equals('prototype', x.prototype);
|
|
Expect.equals('prototype', x.prototype());
|
|
|
|
Expect.equals('constructor', x.constructor);
|
|
Expect.equals('constructor', x.constructor());
|
|
|
|
Expect.equals('__proto__', x.__proto__);
|
|
Expect.equals('__proto__', x.__proto__);
|
|
|
|
Expect.equals(42, x.bar());
|
|
Expect.equals(42, (x.bar)());
|
|
|
|
Expect.equals('unary-', -x);
|
|
Expect.equals('+', x + 42);
|
|
Expect.equals('[]', x[4]);
|
|
|
|
dynamic b = new BaseClass();
|
|
Expect.equals('final!', b.finalField);
|
|
Expect.throwsNoSuchMethodError(() => b.finalField = "foo");
|
|
Expect.equals('final!', b.finalField, 'field was not set');
|
|
|
|
// Verify that noSuchMethod errors are triggered even when the JS object
|
|
// happens to have a matching member name.
|
|
dynamic f = new Foo();
|
|
Expect.throwsNoSuchMethodError(() => f.prototype);
|
|
Expect.throwsNoSuchMethodError(() => f.prototype());
|
|
Expect.throwsNoSuchMethodError(() => f.prototype = 42);
|
|
|
|
Expect.throwsNoSuchMethodError(() => f.constructor);
|
|
Expect.throwsNoSuchMethodError(() => f.constructor());
|
|
Expect.throwsNoSuchMethodError(() => f.constructor = 42);
|
|
|
|
Expect.throwsNoSuchMethodError(() => f.__proto__);
|
|
|
|
// These are valid JS properties but not Dart methods.
|
|
Expect.throwsNoSuchMethodError(() => f.toLocaleString);
|
|
|
|
Expect.throwsNoSuchMethodError(() => f.hasOwnProperty);
|
|
|
|
f = (int x) {};
|
|
// Calls with the wrong number of arguments should be NoSuchMethodErrors.
|
|
Expect.throwsNoSuchMethodError(() => f());
|
|
Expect.throwsNoSuchMethodError(() => f('hi', '!'));
|
|
Expect.throwsNoSuchMethodError(() => f(x: 42));
|
|
}
|