mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 08:20:31 +00:00
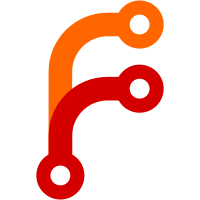
The debian packages on the release branches are now uploaded to the version directory only when the tools/VERSION file changes, allowing cherry-picks to land asynchronously on the beta/stable branches via gerrit without clobbering any existing release artifacts. This logic matches the changes made to the recipe for the dart-sdk builders. This change is required to enforce our data security policies for mandatory code review on the beta and stable branches using the new release pipeline workflow. This change needs to be cherry-picked to beta and stable before launching the new cherry-pick workflow to avoid clobbering artifacts. This change is safe because it doesn't affect any aspect of the Dart SDK except the final upload of .deb files that has been locally tested. Match the dart-sdk builder logic by also uploading by git hash and to the latest directory. Bug: b/256599191 Change-Id: Ib0d4853482edd1bd68f459fc97410fe61b4981c4 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/266685 Commit-Queue: Jonas Termansen <sortie@google.com> Reviewed-by: William Hesse <whesse@google.com>
58 lines
2.3 KiB
Python
Executable file
58 lines
2.3 KiB
Python
Executable file
#!/usr/bin/env python3
|
|
|
|
# Copyright (c) 2019, the Dart project authors. Please see the AUTHORS file
|
|
# for details. All rights reserved. Use of this source code is governed by a
|
|
# BSD-style license that can be found in the LICENSE file.
|
|
|
|
import os
|
|
import sys
|
|
sys.path.append(os.path.join(os.path.dirname(__file__), '..', 'bots'))
|
|
import bot_utils
|
|
|
|
utils = bot_utils.GetUtils()
|
|
|
|
HOST_OS = utils.GuessOS()
|
|
|
|
|
|
def ArchiveArtifacts(tarfile, builddir, channel, subdir):
|
|
namer = bot_utils.GCSNamer(channel=channel)
|
|
gsutil = bot_utils.GSUtil()
|
|
# Archive the src tar to the src dir
|
|
remote_tarfile = '/'.join(
|
|
[namer.src_directory(subdir),
|
|
os.path.basename(tarfile)])
|
|
gsutil.upload(tarfile, remote_tarfile)
|
|
# Archive all files except the tar file to the linux packages dir
|
|
for entry in os.listdir(builddir):
|
|
full_path = os.path.join(builddir, entry)
|
|
# We expect a flat structure, not subdirectories
|
|
assert (os.path.isfile(full_path))
|
|
if full_path != tarfile:
|
|
package_dir = namer.linux_packages_directory(subdir)
|
|
remote_file = '/'.join([package_dir, os.path.basename(entry)])
|
|
gsutil.upload(full_path, remote_file)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
bot_name = os.environ.get('BUILDBOT_BUILDERNAME')
|
|
channel = bot_utils.GetChannelFromName(bot_name)
|
|
if os.environ.get('DART_EXPERIMENTAL_BUILD') == '1':
|
|
print('Not uploading artifacts on experimental builds')
|
|
elif channel == bot_utils.Channel.TRY:
|
|
print('Not uploading artifacts on try builds')
|
|
elif channel == bot_utils.Channel.BLEEDING_EDGE:
|
|
print('Not uploading artifacts on bleeding edge')
|
|
else:
|
|
builddir = os.path.join(bot_utils.DART_DIR, utils.GetBuildDir(HOST_OS),
|
|
'src_and_installation')
|
|
version = utils.GetVersion()
|
|
tarfilename = 'dart-%s.tar.gz' % version
|
|
tarfile = os.path.join(builddir, tarfilename)
|
|
commit = utils.GetGitRevision()
|
|
is_new_version = bot_utils.run(
|
|
['git', 'diff', '--name-only', 'HEAD~1', 'tools/VERSION'])[0] != ''
|
|
subdirs = [f'hash/{commit}']
|
|
if is_new_version:
|
|
subdirs.extend([version, 'latest'])
|
|
for subdir in subdirs:
|
|
ArchiveArtifacts(tarfile, builddir, channel, subdir)
|