mirror of
https://github.com/dart-lang/sdk
synced 2024-09-18 21:41:19 +00:00
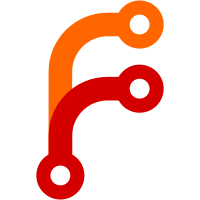
Remove _RemoteStackTrace from dart:isolate and use the new version instead. R=sgjesse@google.com Review URL: https://codereview.chromium.org//1088433004 git-svn-id: https://dart.googlecode.com/svn/branches/bleeding_edge/dart@45088 260f80e4-7a28-3924-810f-c04153c831b5
42 lines
1.3 KiB
Dart
42 lines
1.3 KiB
Dart
// Copyright (c) 2015, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import "package:expect/expect.dart";
|
|
import "package:async_helper/async_helper.dart";
|
|
import "dart:async";
|
|
|
|
void main() {
|
|
StackTrace stack;
|
|
try { throw 0; } catch (e, s) { stack = s; }
|
|
var string = "$stack";
|
|
StackTrace stringTrace = new StackTrace.fromString(string);
|
|
Expect.isTrue(stringTrace is StackTrace);
|
|
Expect.equals(stack.toString(), stringTrace.toString());
|
|
|
|
string = "some random string, nothing like a StackTrace";
|
|
stringTrace = new StackTrace.fromString(string);
|
|
Expect.isTrue(stringTrace is StackTrace);
|
|
Expect.equals(string, stringTrace.toString());
|
|
|
|
// Use stacktrace asynchronously.
|
|
asyncStart();
|
|
var c = new Completer();
|
|
c.completeError(0, stringTrace);
|
|
c.future.then((v) {
|
|
throw "Unexpected value: $v";
|
|
}, onError: (e, s) {
|
|
Expect.equals(string, s.toString());
|
|
}).then((_) {
|
|
var c = new StreamController();
|
|
c.stream.listen((v) {
|
|
throw "Unexpected value: $v";
|
|
}, onError: (e, s) {
|
|
Expect.equals(string, s.toString());
|
|
asyncEnd();
|
|
});
|
|
c.addError(0, stringTrace);
|
|
c.close();
|
|
});
|
|
}
|