mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
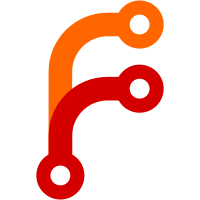
This change bakes binary search table which maps PC ranges to corresponding stack maps and Code objects (if still present in the snapshot) into RO data section of the snapshot - instead of constructing it at load time. This allows to considerably reduce amount of work done when loading Code cluster for programs which have majority of their Code objects discarded (i.e. in DWARF stack traces mode): as we no longer write / read any information for discarded Code objects. This CL also changes program visitor to deduplicate Code objects if their instructions are deduplicated in AOT mode. Only a single Code object can be choose as a representative for the given PC range so it does not make sense to write multiple Code objects into the snapshot which refer to the same Instructions. The overall improvement is hard to quantify but ReadProgramSnapshot shows the following improvement when starting a large Flutter application on a slow Android device: before 223.55±59.94 (192.02 .. 391.74) ms after 178.06±47.03 (151.31 .. 291.34) ms This CL packs CompressedStackMaps next to the binary search table itself allowing us to address them via offsets instead of pointers. Snapshot sizes are actually affected positively by this change. On the same large Flutter application I see DWARF stack traces on: -1.34% total SO size DWARF stack traces off: -1.63% total SO size Issue https://github.com/dart-lang/sdk/issues/46116 TEST=ci Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-dwarf-linux-product-x64-try,vm-kernel-precomp-linux-debug-simarm64c-try,vm-kernel-precomp-linux-debug-simarm_x64-try,vm-kernel-precomp-linux-debug-x64-try,vm-kernel-precomp-linux-debug-x64c-try,vm-kernel-precomp-linux-product-x64-try,vm-kernel-precomp-linux-release-simarm-try,vm-kernel-precomp-linux-release-simarm64-try,vm-kernel-precomp-linux-release-simarm_x64-try,vm-kernel-precomp-linux-release-x64-try Change-Id: Ic997045a33daa81ec68df462a0792915885df66b Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/220766 Reviewed-by: Alexander Markov <alexmarkov@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com> Commit-Queue: Slava Egorov <vegorov@google.com>
121 lines
4.1 KiB
C++
121 lines
4.1 KiB
C++
// Copyright (c) 2018, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#include "vm/reverse_pc_lookup_cache.h"
|
|
|
|
#include "vm/isolate.h"
|
|
#include "vm/object.h"
|
|
#include "vm/object_store.h"
|
|
#include "vm/stub_code.h"
|
|
|
|
namespace dart {
|
|
|
|
CodePtr ReversePc::FindCodeInGroup(IsolateGroup* group,
|
|
uword pc,
|
|
bool is_return_address) {
|
|
#if defined(DART_PRECOMPILED_RUNTIME)
|
|
// This can run in the middle of GC and must not allocate handles.
|
|
NoSafepointScope no_safepoint;
|
|
|
|
if (is_return_address) {
|
|
pc--;
|
|
}
|
|
|
|
// This expected number of tables is low (one per loading unit), so we go
|
|
// through them linearly. If this changes, would could sort the table list
|
|
// during deserialization and binary search for the table.
|
|
GrowableObjectArrayPtr tables = group->object_store()->instructions_tables();
|
|
intptr_t tables_length = Smi::Value(tables->untag()->length());
|
|
for (intptr_t i = 0; i < tables_length; i++) {
|
|
InstructionsTablePtr table = static_cast<InstructionsTablePtr>(
|
|
tables->untag()->data()->untag()->element(i));
|
|
CodePtr code = InstructionsTable::FindCode(table, pc);
|
|
if (code != Code::null()) {
|
|
return code;
|
|
}
|
|
}
|
|
#endif // defined(DART_PRECOMPILED_RUNTIME)
|
|
return Code::null();
|
|
}
|
|
|
|
const UntaggedCompressedStackMaps::Payload* ReversePc::FindStackMapInGroup(
|
|
IsolateGroup* group,
|
|
uword pc,
|
|
bool is_return_address,
|
|
uword* code_start,
|
|
const UntaggedCompressedStackMaps::Payload** global_table) {
|
|
#if defined(DART_PRECOMPILED_RUNTIME)
|
|
// This can run in the middle of GC and must not allocate handles.
|
|
NoSafepointScope no_safepoint;
|
|
|
|
if (is_return_address) {
|
|
pc--;
|
|
}
|
|
|
|
// This expected number of tables is low (one per loading unit), so we go
|
|
// through them linearly. If this changes, would could sort the table list
|
|
// during deserialization and binary search for the table.
|
|
GrowableObjectArrayPtr tables = group->object_store()->instructions_tables();
|
|
intptr_t tables_length = Smi::Value(tables->untag()->length());
|
|
for (intptr_t i = 0; i < tables_length; i++) {
|
|
InstructionsTablePtr table = static_cast<InstructionsTablePtr>(
|
|
tables->untag()->data()->untag()->element(i));
|
|
auto map = InstructionsTable::FindStackMap(table, pc, code_start);
|
|
if (map != nullptr) {
|
|
// Take global table from the first table.
|
|
table = static_cast<InstructionsTablePtr>(
|
|
tables->untag()->data()->untag()->element(0));
|
|
*global_table = InstructionsTable::GetCanonicalStackMap(table);
|
|
return map;
|
|
}
|
|
}
|
|
#endif // defined(DART_PRECOMPILED_RUNTIME)
|
|
|
|
*code_start = 0;
|
|
return nullptr;
|
|
}
|
|
|
|
const UntaggedCompressedStackMaps::Payload* ReversePc::FindStackMap(
|
|
IsolateGroup* group,
|
|
uword pc,
|
|
bool is_return_address,
|
|
uword* code_start,
|
|
const UntaggedCompressedStackMaps::Payload** global_table) {
|
|
ASSERT(FLAG_precompiled_mode);
|
|
NoSafepointScope no_safepoint;
|
|
|
|
auto map = FindStackMapInGroup(group, pc, is_return_address, code_start,
|
|
global_table);
|
|
if (map == nullptr) {
|
|
map = FindStackMapInGroup(Dart::vm_isolate_group(), pc, is_return_address,
|
|
code_start, global_table);
|
|
}
|
|
return map;
|
|
}
|
|
|
|
CodePtr ReversePc::FindCode(IsolateGroup* group,
|
|
uword pc,
|
|
bool is_return_address) {
|
|
ASSERT(FLAG_precompiled_mode);
|
|
NoSafepointScope no_safepoint;
|
|
|
|
auto code_descriptor = FindCodeInGroup(group, pc, is_return_address);
|
|
if (code_descriptor == Code::null()) {
|
|
code_descriptor =
|
|
FindCodeInGroup(Dart::vm_isolate_group(), pc, is_return_address);
|
|
}
|
|
return code_descriptor;
|
|
}
|
|
|
|
CodePtr ReversePc::Lookup(IsolateGroup* group,
|
|
uword pc,
|
|
bool is_return_address) {
|
|
ASSERT(FLAG_precompiled_mode);
|
|
NoSafepointScope no_safepoint;
|
|
|
|
return FindCode(group, pc, is_return_address);
|
|
}
|
|
|
|
} // namespace dart
|