mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
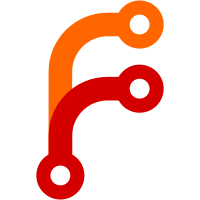
The new implementation is based on suspend/resume stubs and doesn't use desugaring of async functions on kernel AST. Previously, new implementation of async/async* was only supported in AOT mode. This change adds all necessary bits for the JIT mode: * Suspending variable-length frames (for unoptimized code). * Handling of Code and pool pointers in Dart stack frames. * OSR. * Deoptimization. * Hot reload. * Debugger. The new implementation is not enabled in JIT mode yet. Design doc: go/compact-async-await. TEST=ci Issue: https://github.com/dart-lang/sdk/issues/48378 Change-Id: I477d6684bdce7cbc1edb179ae2271ff598b7dcc5 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/246081 Reviewed-by: Martin Kustermann <kustermann@google.com> Reviewed-by: Johnni Winther <johnniwinther@google.com> Commit-Queue: Alexander Markov <alexmarkov@google.com> Reviewed-by: Slava Egorov <vegorov@google.com>
54 lines
1.3 KiB
C++
54 lines
1.3 KiB
C++
// Copyright (c) 2021, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_PENDING_DEOPTS_H_
|
|
#define RUNTIME_VM_PENDING_DEOPTS_H_
|
|
|
|
#if defined(SHOULD_NOT_INCLUDE_RUNTIME)
|
|
#error "Should not include runtime"
|
|
#endif
|
|
|
|
#include "vm/growable_array.h"
|
|
|
|
namespace dart {
|
|
|
|
class PendingLazyDeopt {
|
|
public:
|
|
PendingLazyDeopt(uword fp, uword pc) : fp_(fp), pc_(pc) {}
|
|
uword fp() { return fp_; }
|
|
uword pc() { return pc_; }
|
|
void set_pc(uword pc) { pc_ = pc; }
|
|
|
|
private:
|
|
uword fp_;
|
|
uword pc_;
|
|
};
|
|
|
|
class PendingDeopts {
|
|
public:
|
|
enum ClearReason {
|
|
kClearDueToThrow,
|
|
kClearDueToDeopt,
|
|
};
|
|
PendingDeopts();
|
|
~PendingDeopts();
|
|
|
|
bool HasPendingDeopts() { return pending_deopts_->length() > 0; }
|
|
|
|
void AddPendingDeopt(uword fp, uword pc);
|
|
uword FindPendingDeopt(uword fp);
|
|
void ClearPendingDeoptsBelow(uword fp, ClearReason reason);
|
|
void ClearPendingDeoptsAtOrBelow(uword fp, ClearReason reason);
|
|
uword RemapExceptionPCForDeopt(uword program_counter,
|
|
uword frame_pointer,
|
|
bool* clear_deopt);
|
|
|
|
private:
|
|
MallocGrowableArray<PendingLazyDeopt>* pending_deopts_;
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_PENDING_DEOPTS_H_
|