mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
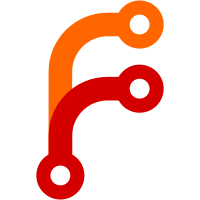
App-jit and core-jit snapshots generated on one machine can be copied to another machine with different CPU family/model, so it is incorrect to use CPU features of the host machine to generate code for snapshots. This change adds --target-unknown-cpu option and enables it when generating app-jit and core-jit snapshots in standalone Dart VM and gen_snapshot. Currently, this flag disables SSE4.1, popcnt and ABM on ia32 and x64, and integer division instruction on ARM. Also, new flag enables testing of roundsd instruction availability at run time on x64 (similarly to AOT). TEST=ci Fixes https://github.com/dart-lang/sdk/issues/47907 Fixes https://github.com/flutter/flutter/issues/94181 Change-Id: Id28448052a21df4bae30b39e62b8532e55d4c901 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/223960 Reviewed-by: Ryan Macnak <rmacnak@google.com> Commit-Queue: Alexander Markov <alexmarkov@google.com>
72 lines
2.1 KiB
C++
72 lines
2.1 KiB
C++
// Copyright (c) 2014, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_CPU_X64_H_
|
|
#define RUNTIME_VM_CPU_X64_H_
|
|
|
|
#if !defined(RUNTIME_VM_CPU_H_)
|
|
#error Do not include cpu_x64.h directly; use cpu.h instead.
|
|
#endif
|
|
|
|
#include "vm/allocation.h"
|
|
#include "vm/flags.h"
|
|
|
|
namespace dart {
|
|
|
|
DECLARE_FLAG(bool, use_sse41);
|
|
|
|
class HostCPUFeatures : public AllStatic {
|
|
public:
|
|
static void Init();
|
|
static void Cleanup();
|
|
static const char* hardware() {
|
|
DEBUG_ASSERT(initialized_);
|
|
return hardware_;
|
|
}
|
|
static bool sse2_supported() {
|
|
DEBUG_ASSERT(initialized_);
|
|
return sse2_supported_;
|
|
}
|
|
static bool sse4_1_supported() {
|
|
DEBUG_ASSERT(initialized_);
|
|
return sse4_1_supported_ && FLAG_use_sse41 && !FLAG_target_unknown_cpu;
|
|
}
|
|
static bool popcnt_supported() {
|
|
DEBUG_ASSERT(initialized_);
|
|
return popcnt_supported_ && !FLAG_target_unknown_cpu;
|
|
}
|
|
static bool abm_supported() {
|
|
DEBUG_ASSERT(initialized_);
|
|
return abm_supported_ && !FLAG_target_unknown_cpu;
|
|
}
|
|
|
|
private:
|
|
static const char* hardware_;
|
|
static bool sse2_supported_;
|
|
static bool sse4_1_supported_;
|
|
static bool popcnt_supported_;
|
|
static bool abm_supported_;
|
|
#if defined(DEBUG)
|
|
static bool initialized_;
|
|
#endif
|
|
};
|
|
|
|
class TargetCPUFeatures : public AllStatic {
|
|
public:
|
|
static void Init() { HostCPUFeatures::Init(); }
|
|
static void Cleanup() { HostCPUFeatures::Cleanup(); }
|
|
static const char* hardware() { return HostCPUFeatures::hardware(); }
|
|
static bool sse2_supported() { return HostCPUFeatures::sse2_supported(); }
|
|
static bool sse4_1_supported() { return HostCPUFeatures::sse4_1_supported(); }
|
|
static bool popcnt_supported() { return HostCPUFeatures::popcnt_supported(); }
|
|
static bool abm_supported() { return HostCPUFeatures::abm_supported(); }
|
|
static bool double_truncate_round_supported() {
|
|
return HostCPUFeatures::sse4_1_supported();
|
|
}
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_CPU_X64_H_
|