mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
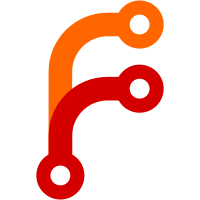
The relocated addresses are populated during BSS::Initialize() for natively loaded ELF snapshots, and during the non-native loader when it is used. Putting this information in the BSS segment avoids having to change the embedder interface, since we only need this information for AOT snapshots. This also avoids depending on our ELF snapshot layout to reverse-engineer the DSO base for ELF-compiled snapshots. We now always print the DSO base for both the VM and isolate in non-symbolic stack traces, not just for ELF-compiled snapshots. However, we still only print the relocated addresses in individual stack frames if we're guaranteed they match those in separately saved debugging information. Bug: https://github.com/dart-lang/sdk/issues/41880 Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-linux-release-x64-try,vm-kernel-precomp-linux-product-x64-try,vm-kernel-precomp-linux-debug-x64-try,vm-kernel-precomp-win-release-x64-try,vm-kernel-precomp-mac-release-simarm64-try Change-Id: I4837262f78e6e73a32eb7e24ef7a68ccb8ec2669 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/148441 Commit-Queue: Tess Strickland <sstrickl@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com>
47 lines
1.5 KiB
C++
47 lines
1.5 KiB
C++
// Copyright (c) 2019, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#ifndef RUNTIME_VM_BSS_RELOCS_H_
|
|
#define RUNTIME_VM_BSS_RELOCS_H_
|
|
|
|
#include "platform/allocation.h"
|
|
|
|
namespace dart {
|
|
class Thread;
|
|
|
|
class BSS : public AllStatic {
|
|
public:
|
|
// Entries found in both the VM and isolate BSS come first. Each has its own
|
|
// portion of the BSS segment, so just the indices are shared, not the values
|
|
// stored at the index.
|
|
enum class Relocation : intptr_t {
|
|
InstructionsRelocatedAddress,
|
|
// End of shared entries.
|
|
DRT_GetThreadForNativeCallback,
|
|
// End of isolate-only entries.
|
|
};
|
|
|
|
static constexpr intptr_t kVmEntryCount =
|
|
static_cast<intptr_t>(Relocation::InstructionsRelocatedAddress) + 1;
|
|
|
|
static constexpr intptr_t kIsolateEntryCount =
|
|
static_cast<intptr_t>(Relocation::DRT_GetThreadForNativeCallback) + 1;
|
|
|
|
static constexpr intptr_t RelocationIndex(Relocation reloc) {
|
|
return static_cast<intptr_t>(reloc);
|
|
}
|
|
|
|
static void Initialize(Thread* current, uword* bss, bool vm);
|
|
|
|
// Currently only used externally by LoadedElf::ResolveSymbols() to set the
|
|
// relocated address without changing the embedder interface.
|
|
static void InitializeBSSEntry(BSS::Relocation relocation,
|
|
uword new_value,
|
|
uword* bss_start);
|
|
};
|
|
|
|
} // namespace dart
|
|
|
|
#endif // RUNTIME_VM_BSS_RELOCS_H_
|