mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
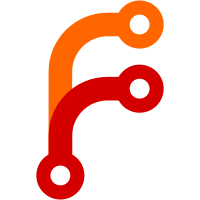
Before, we only wrapped the Instructions payloads in an InstructionsSection in precompiled snapshots with bare instructions mode enabled. This CL changes that to always add an InstructionsSection wrapper in precompiled snapshots, even when not in bare instructions mode, and moves all the information from the old ImageHeader object into the InstructionsSection object instead. Note that in non-bare mode, the InstructionsSection has no payload, and the Instructions objects follow the InstructionsSection object instead of being contained by it. This CL also wraps all implementations of AssemblyImageWriter and SnapshotTextObjectNamer methods in a single DART_PRECOMPILER ifdef block, since we only generate assembly in precompiled mode. This removes the need for the smaller DART_PRECOMPILER ifdef blocks scattered across the AssemblyImageWriter method implementations. Cq-Include-Trybots: luci.dart.try:vm-kernel-precomp-linux-debug-x64-try,vm-kernel-precomp-linux-debug-simarm_x64-try,vm-kernel-precomp-mac-release-simarm64-try,vm-kernel-mac-debug-x64-try,vm-kernel-win-debug-x64-try,vm-kernel-precomp-win-release-x64-try Change-Id: I3676fb5c6675845463707acbbb4759a465cfa342 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/165563 Reviewed-by: Daco Harkes <dacoharkes@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com> Reviewed-by: Ryan Macnak <rmacnak@google.com>
50 lines
2 KiB
C++
50 lines
2 KiB
C++
// Copyright (c) 2019, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#include "vm/bss_relocs.h"
|
|
#include "vm/native_symbol.h"
|
|
#include "vm/runtime_entry.h"
|
|
#include "vm/thread.h"
|
|
|
|
namespace dart {
|
|
|
|
void BSS::InitializeBSSEntry(BSS::Relocation relocation,
|
|
uword new_value,
|
|
uword* bss_start) {
|
|
std::atomic<uword>* slot = reinterpret_cast<std::atomic<uword>*>(
|
|
&bss_start[BSS::RelocationIndex(relocation)]);
|
|
uword old_value = slot->load(std::memory_order_relaxed);
|
|
// FullSnapshotReader::ReadProgramSnapshot, and thus BSS::Initialize, can
|
|
// get called multiple times for the same isolate in different threads, though
|
|
// the initialized value will be consistent and thus change only once. Avoid
|
|
// calling compare_exchange_strong unless we actually need to change the
|
|
// value, to avoid spurious read/write races by TSAN.
|
|
if (old_value == new_value) return;
|
|
if (!slot->compare_exchange_strong(old_value, new_value,
|
|
std::memory_order_relaxed)) {
|
|
RELEASE_ASSERT(old_value == new_value);
|
|
}
|
|
}
|
|
|
|
void BSS::Initialize(Thread* current, uword* bss_start, bool vm) {
|
|
auto const instructions = reinterpret_cast<uword>(
|
|
current->isolate_group()->source()->snapshot_instructions);
|
|
uword dso_base;
|
|
// Needed for assembly snapshots. For ELF snapshots, we set up the relocated
|
|
// address information directly in the text segment InstructionsSection.
|
|
if (NativeSymbolResolver::LookupSharedObject(instructions, &dso_base)) {
|
|
InitializeBSSEntry(Relocation::InstructionsRelocatedAddress,
|
|
instructions - dso_base, bss_start);
|
|
}
|
|
|
|
if (!vm) {
|
|
// Fill values at isolate-only indices.
|
|
InitializeBSSEntry(Relocation::DRT_GetThreadForNativeCallback,
|
|
reinterpret_cast<uword>(DLRT_GetThreadForNativeCallback),
|
|
bss_start);
|
|
}
|
|
}
|
|
|
|
} // namespace dart
|