mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 00:13:50 +00:00
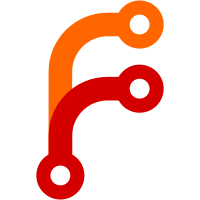
This CL implements `NativeFinalizer` in the GC. `FinalizerEntry`s are extended to track `external_size` and in which `Heap::Space` the finalizable value is. On attaching a native finalizer, the external size is added to the relevant heap. When the finalizable value is promoted from new to old space, the external size is promoted as well. And when a native finalizer is run or is detached, the external size is removed from the relevant heap again. In contrast to Dart `Finalizer`s, `NativeFinalizer`s are run on isolate shutdown. When the `NativeFinalizer`s themselves are collected, the finalizers are not run. Users should stick the native finalizer in a global variable to ensure finalization. We will revisit this design when we add send and exit support, because there is a design space to explore what to do in that case. This current solution promises the least to users. In this implementation native finalizers have a Dart entry to clean up the entries from the `all_entries` field of the finalizer. We should consider using another data structure that avoids the need for this Dart entry. See the TODO left in the code. Bug: https://github.com/dart-lang/sdk/issues/47777 TEST=runtime/tests/vm/dart(_2)/isolates/fast_object_copy_test.dart TEST=runtime/vm/object_test.cc TEST=tests/ffi(_2)/vmspecific_native_finalizer_* Change-Id: I8f594c80c3c344ad83e1f2de10de028eb8456121 Cq-Include-Trybots: luci.dart.try:vm-kernel-reload-rollback-linux-debug-x64-try,vm-kernel-reload-linux-debug-x64-try,vm-ffi-android-debug-arm64c-try,dart-sdk-mac-arm64-try,vm-kernel-mac-release-arm64-try,pkg-mac-release-arm64-try,vm-kernel-precomp-nnbd-mac-release-arm64-try,vm-kernel-win-debug-x64c-try,vm-kernel-win-debug-x64-try,vm-kernel-precomp-win-debug-x64c-try,vm-kernel-nnbd-win-release-ia32-try,vm-ffi-android-debug-arm-try,vm-precomp-ffi-qemu-linux-release-arm-try,vm-kernel-mac-debug-x64-try,vm-kernel-nnbd-mac-debug-x64-try,vm-kernel-nnbd-linux-debug-ia32-try,benchmark-linux-try,flutter-frontend-try,pkg-linux-debug-try,vm-kernel-asan-linux-release-x64-try,vm-kernel-gcc-linux-try,vm-kernel-optcounter-threshold-linux-release-x64-try,vm-kernel-precomp-linux-debug-simarm_x64-try,vm-kernel-precomp-obfuscate-linux-release-x64-try,vm-kernel-precomp-linux-debug-x64-try,vm-kernel-precomp-linux-debug-x64c-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/236320 Reviewed-by: Martin Kustermann <kustermann@google.com> Reviewed-by: Slava Egorov <vegorov@google.com> Commit-Queue: Daco Harkes <dacoharkes@google.com>
170 lines
5.8 KiB
C++
170 lines
5.8 KiB
C++
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
#include "vm/bootstrap.h"
|
|
|
|
#include "include/dart_api.h"
|
|
|
|
#include "vm/bootstrap_natives.h"
|
|
#include "vm/dart_api_impl.h"
|
|
#include "vm/object.h"
|
|
#include "vm/object_store.h"
|
|
#include "vm/service_isolate.h"
|
|
|
|
namespace dart {
|
|
|
|
// Helper macros for declaring and defining native entries.
|
|
#define REGISTER_NATIVE_ENTRY(name, count) \
|
|
{"" #name, BootstrapNatives::DN_##name, count},
|
|
|
|
// List all native functions implemented in the vm or core bootstrap dart
|
|
// libraries so that we can resolve the native function to it's entry
|
|
// point.
|
|
static const struct NativeEntries {
|
|
const char* name_;
|
|
BootstrapNativeFunction function_;
|
|
int argument_count_;
|
|
} BootStrapEntries[] = {BOOTSTRAP_NATIVE_LIST(REGISTER_NATIVE_ENTRY)
|
|
#if !defined(DART_PRECOMPILED_RUNTIME)
|
|
MIRRORS_BOOTSTRAP_NATIVE_LIST(REGISTER_NATIVE_ENTRY)
|
|
#endif // !DART_PRECOMPILED_RUNTIME
|
|
};
|
|
|
|
#define REGISTER_FFI_NATIVE_ENTRY(name, return_type, argument_types) \
|
|
{"" #name, reinterpret_cast<void*>(BootstrapNatives::FN_##name)},
|
|
|
|
static const struct FfiNativeEntries {
|
|
const char* name_;
|
|
void* function_;
|
|
} BootStrapFfiEntries[] = {
|
|
BOOTSTRAP_FFI_NATIVE_LIST(REGISTER_FFI_NATIVE_ENTRY)};
|
|
|
|
Dart_NativeFunction BootstrapNatives::Lookup(Dart_Handle name,
|
|
int argument_count,
|
|
bool* auto_setup_scope) {
|
|
Thread* thread = Thread::Current();
|
|
TransitionNativeToVM transition(thread);
|
|
const Object& obj = Object::Handle(thread->zone(), Api::UnwrapHandle(name));
|
|
if (!obj.IsString()) {
|
|
return NULL;
|
|
}
|
|
ASSERT(auto_setup_scope);
|
|
*auto_setup_scope = false;
|
|
const char* function_name = obj.ToCString();
|
|
ASSERT(function_name != NULL);
|
|
int num_entries = sizeof(BootStrapEntries) / sizeof(struct NativeEntries);
|
|
for (int i = 0; i < num_entries; i++) {
|
|
const struct NativeEntries* entry = &(BootStrapEntries[i]);
|
|
if ((strcmp(function_name, entry->name_) == 0) &&
|
|
(entry->argument_count_ == argument_count)) {
|
|
return reinterpret_cast<Dart_NativeFunction>(entry->function_);
|
|
}
|
|
}
|
|
return NULL;
|
|
}
|
|
|
|
void* BootstrapNatives::LookupFfiNative(const char* name,
|
|
uintptr_t argument_count) {
|
|
int num_entries =
|
|
sizeof(BootStrapFfiEntries) / sizeof(struct FfiNativeEntries);
|
|
for (int i = 0; i < num_entries; i++) {
|
|
const struct FfiNativeEntries* entry = &(BootStrapFfiEntries[i]);
|
|
if (strcmp(name, entry->name_) == 0) {
|
|
return entry->function_;
|
|
}
|
|
}
|
|
return nullptr;
|
|
}
|
|
|
|
const uint8_t* BootstrapNatives::Symbol(Dart_NativeFunction nf) {
|
|
int num_entries = sizeof(BootStrapEntries) / sizeof(struct NativeEntries);
|
|
for (int i = 0; i < num_entries; i++) {
|
|
const struct NativeEntries* entry = &(BootStrapEntries[i]);
|
|
if (reinterpret_cast<Dart_NativeFunction>(entry->function_) == nf) {
|
|
return reinterpret_cast<const uint8_t*>(entry->name_);
|
|
}
|
|
}
|
|
return NULL;
|
|
}
|
|
|
|
void Bootstrap::SetupNativeResolver() {
|
|
Library& library = Library::Handle();
|
|
|
|
Dart_NativeEntryResolver resolver = BootstrapNatives::Lookup;
|
|
|
|
Dart_FfiNativeResolver ffi_native_resolver =
|
|
BootstrapNatives::LookupFfiNative;
|
|
|
|
Dart_NativeEntrySymbol symbol_resolver = BootstrapNatives::Symbol;
|
|
|
|
library = Library::AsyncLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
|
|
library = Library::CollectionLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
|
|
library = Library::ConvertLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
|
|
library = Library::CoreLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
|
|
library = Library::DeveloperLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
|
|
library = Library::FfiLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
|
|
library = Library::InternalLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
library.set_ffi_native_resolver(ffi_native_resolver);
|
|
|
|
library = Library::IsolateLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
|
|
library = Library::MathLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
|
|
#if !defined(DART_PRECOMPILED_RUNTIME)
|
|
library = Library::MirrorsLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
#endif
|
|
|
|
library = Library::TypedDataLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
|
|
library = Library::VMServiceLibrary();
|
|
ASSERT(!library.IsNull());
|
|
library.set_native_entry_resolver(resolver);
|
|
library.set_native_entry_symbol_resolver(symbol_resolver);
|
|
}
|
|
|
|
bool Bootstrap::IsBootstrapResolver(Dart_NativeEntryResolver resolver) {
|
|
return resolver == BootstrapNatives::Lookup;
|
|
}
|
|
|
|
} // namespace dart
|