mirror of
https://github.com/dart-lang/sdk
synced 2024-11-05 18:22:09 +00:00
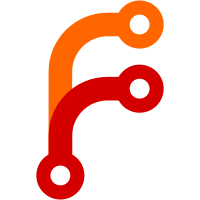
Change-Id: Ib8721cf976803d8d1b9d723b7e691e344c768b67 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/149881 Reviewed-by: Sigmund Cherem <sigmund@google.com> Commit-Queue: Joshua Litt <joshualitt@google.com>
38 lines
1 KiB
Dart
38 lines
1 KiB
Dart
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
// Interpolation effect analysis test.
|
|
|
|
get never => new DateTime.now().millisecondsSinceEpoch == 0;
|
|
|
|
class A {
|
|
int a = 0;
|
|
toString() {
|
|
++a;
|
|
return 'A';
|
|
}
|
|
}
|
|
|
|
// Many interpolations to make function too big to inline.
|
|
// Summary for [fmt] must include effects from toString().
|
|
fmt(x) => '$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x$x';
|
|
|
|
test(a) {
|
|
if (a == null) return;
|
|
if (never) a.a += 1;
|
|
var b = a.a; // field load
|
|
var c = fmt(a); // field modified through implicit call to toString()
|
|
var d = a.a; // field re-load
|
|
Expect.equals('A 0 AAAAAAAAAAAAAAAAAAAAAAAAAAAAAA 30', '$a $b $c $d');
|
|
|
|
// Extra use of [fmt] to prevent inlining on basis of single reference.
|
|
Expect.equals('', fmt(''));
|
|
}
|
|
|
|
main() {
|
|
test(null);
|
|
test(new A());
|
|
}
|