mirror of
https://github.com/dart-lang/sdk
synced 2024-09-18 21:51:18 +00:00
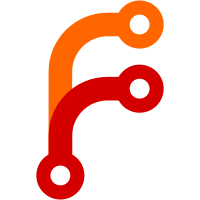
According to our typing rules a Set<Apple> is a Set<Fruit>. However, before this change, we couldn't use it as a Set<Fruit>: === bool foo(Set<Fruit> fruits) { // Would yield a type-error since we actually got a Set<Apple>. return fruits.contains(new Banana()); } foo(new Set<Apple>()); === R=ajohnsen@google.com, blois@google.com, lrn@google.com Review URL: https://codereview.chromium.org//14246008 git-svn-id: https://dart.googlecode.com/svn/branches/bleeding_edge/dart@24326 260f80e4-7a28-3924-810f-c04153c831b5
24 lines
672 B
Dart
24 lines
672 B
Dart
// Copyright (c) 2011, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
class A { const A(); }
|
|
class B extends A { const B(); }
|
|
|
|
main() {
|
|
var set1 = new Set<B>();
|
|
set1.add(const B());
|
|
var set2 = new Set<B>();
|
|
var list = <B>[ const B() ];
|
|
var set3 = list.toSet();
|
|
|
|
var sets = [set1, set2, set3];
|
|
for (var setToTest in sets) {
|
|
// Test that the set accepts a list that is not of the same type:
|
|
// Set<B>.retainAll(List<A>)
|
|
setToTest.retainAll(<A>[new A()]);
|
|
}
|
|
}
|