mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 10:10:22 +00:00
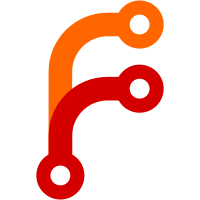
Change-Id: I48fcc8443a0980c435e87642cf6a7aede07e68f0 Reviewed-on: https://dart-review.googlesource.com/17161 Commit-Queue: Peter von der Ahé <ahe@google.com> Reviewed-by: Aske Simon Christensen <askesc@google.com> Reviewed-by: Sigmund Cherem <sigmund@google.com> Reviewed-by: Zach Anderson <zra@google.com>
32 lines
1.2 KiB
Dart
32 lines
1.2 KiB
Dart
// Copyright (c) 2017, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'dart:io' show File, exit, stderr;
|
|
|
|
import 'dart:isolate' show RawReceivePort;
|
|
|
|
import 'dart:convert' show JsonEncoder;
|
|
|
|
import 'package:yaml/yaml.dart' show loadYaml;
|
|
|
|
main(List<String> arguments) async {
|
|
var port = new RawReceivePort();
|
|
if (arguments.length != 2) {
|
|
stderr.writeln("Usage: yaml2json.dart input.yaml output.json");
|
|
exit(1);
|
|
}
|
|
Uri input = Uri.base.resolve(arguments[0]);
|
|
Uri output = Uri.base.resolve(arguments[1]);
|
|
Map yaml = loadYaml(await new File.fromUri(input).readAsString());
|
|
Map<String, dynamic> result = new Map<String, dynamic>();
|
|
result["comment:0"] = "NOTE: THIS FILE IS GENERATED. DO NOT EDIT.";
|
|
result["comment:1"] =
|
|
"Instead modify '${arguments[0]}' and follow the instructions therein.";
|
|
for (String key in yaml.keys) {
|
|
result[key] = yaml[key];
|
|
}
|
|
File file = new File.fromUri(output);
|
|
await file.writeAsString(const JsonEncoder.withIndent(" ").convert(result));
|
|
port.close();
|
|
}
|