mirror of
https://github.com/dart-lang/sdk
synced 2024-09-18 22:01:19 +00:00
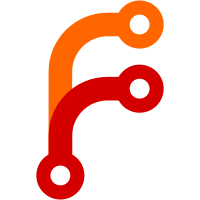
New scheme for checking function subtyping: 1) Generate an $isX predicate on objects that are statically known subtypes of a given function type 2) Generate a signature encoding the whole function type on objects when a runtime check is needed. A check succeeds if either 1) or 2) is true. If too many predicates are needed a signature is generated instead, and if a signature is needed, no predicates are generated. Maximum number of predicates is currently determined by the magic constant MAX_FUNCTION_TYPE_PREDICATES in emitter.dart. R=karlklose@google.com Committed: https://code.google.com/p/dart/source/detail?r=24286 Review URL: https://codereview.chromium.org//12334070 git-svn-id: https://dart.googlecode.com/svn/branches/bleeding_edge/dart@24309 260f80e4-7a28-3924-810f-c04153c831b5
41 lines
751 B
Dart
41 lines
751 B
Dart
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
// Dart test program for constructors and initializers.
|
|
|
|
// Check function subtyping for implicit setters.
|
|
|
|
import 'package:expect/expect.dart';
|
|
|
|
typedef void Foo();
|
|
class A<T> {}
|
|
|
|
class C {
|
|
Foo foo;
|
|
A<int> bar;
|
|
}
|
|
|
|
class D {
|
|
Foo foo;
|
|
A<int> bar;
|
|
}
|
|
|
|
test(var c) {
|
|
bool inCheckedMode = false;
|
|
try {
|
|
var x = 42;
|
|
String a = x;
|
|
} catch (e) {
|
|
inCheckedMode = true;
|
|
}
|
|
if (inCheckedMode) {
|
|
Expect.throws(() => c.foo = 1, (e) => true);
|
|
}
|
|
c.foo = () {};
|
|
}
|
|
|
|
void main() {
|
|
test(new C());
|
|
test(new D());
|
|
}
|