mirror of
https://github.com/dart-lang/sdk
synced 2024-09-18 22:01:19 +00:00
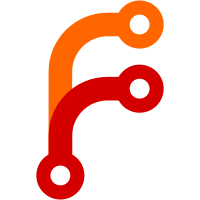
Add IterableMixin and IterableBase to dart:collection. For now, the implementation is duplicated, because mixins and const constructors don't mix. We hope that mixins will be improved in the future to allow using the mixin to define the base class. Review URL: https://codereview.chromium.org//14022007 git-svn-id: https://dart.googlecode.com/svn/branches/bleeding_edge/dart@21457 260f80e4-7a28-3924-810f-c04153c831b5
53 lines
1.4 KiB
Dart
53 lines
1.4 KiB
Dart
// Copyright (c) 2012, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import "dart:collection";
|
|
|
|
abstract class Link<T> extends IterableBase<T> {
|
|
// does not match constructor for LinkFactory
|
|
factory Link(T head, [Link<T> tail]) = LinkFactory<T>; /// static type warning
|
|
Link<T> prepend(T element);
|
|
}
|
|
|
|
abstract class EmptyLink<T> extends Link<T> {
|
|
const factory EmptyLink() = LinkTail<T>;
|
|
}
|
|
|
|
class LinkFactory<T> {
|
|
factory LinkFactory(head, [Link tail]) { }
|
|
}
|
|
|
|
// Does not implement all of Iterable
|
|
class AbstractLink<T> implements Link<T> {
|
|
const AbstractLink();
|
|
Link<T> prepend(T element) {
|
|
return new Link<T>(element, this);
|
|
}
|
|
}
|
|
|
|
// Does not implement all of Iterable
|
|
class LinkTail<T> extends AbstractLink<T>
|
|
implements EmptyLink<T> {
|
|
const LinkTail();
|
|
}
|
|
|
|
// Does not implement all of Iterable
|
|
class LinkEntry<T> extends AbstractLink<T> {
|
|
LinkEntry(T head, Link<T> realTail);
|
|
}
|
|
|
|
class Fisk {
|
|
// instantiation of abstract class
|
|
Link<String> nodes = const EmptyLink(); /// static type warning
|
|
}
|
|
|
|
main() {
|
|
new Fisk();
|
|
// instantiation of abstract class
|
|
new EmptyLink<String>().prepend('hest'); /// static type warning
|
|
// instantiation of abstract class
|
|
const EmptyLink<String>().prepend('fisk'); /// static type warning
|
|
}
|
|
|