mirror of
https://github.com/dart-lang/sdk
synced 2024-11-02 10:49:00 +00:00
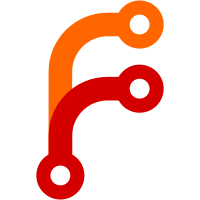
This reverts commit 5cbf10febe
.
TEST=pkg/dds/devtools_observatory_connection_test.dart
Change-Id: I682d009f784b930094dae9b7d4e9a66efffb6ee8
Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/198561
Reviewed-by: Siva Annamalai <asiva@google.com>
75 lines
2.4 KiB
Dart
75 lines
2.4 KiB
Dart
// Copyright (c) 2021, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
// @dart=2.10
|
|
|
|
import 'dart:convert';
|
|
import 'dart:io';
|
|
|
|
import 'package:dds/dds.dart';
|
|
|
|
import 'package:test/test.dart';
|
|
import 'common/test_helper.dart';
|
|
|
|
// Regression test for https://github.com/dart-lang/sdk/issues/45933.
|
|
|
|
void main() {
|
|
Process process;
|
|
DartDevelopmentService dds;
|
|
|
|
setUp(() async {
|
|
// We don't care what's actually running in the target process for this
|
|
// test, so we're just using an existing one that invokes `debugger()` so
|
|
// we know it won't exit before we can connect.
|
|
process = await spawnDartProcess(
|
|
'get_stream_history_script.dart',
|
|
pauseOnStart: false,
|
|
);
|
|
});
|
|
|
|
tearDown(() async {
|
|
await dds?.shutdown();
|
|
process?.kill();
|
|
dds = null;
|
|
process = null;
|
|
});
|
|
|
|
defineTest({bool authCodesEnabled}) {
|
|
test(
|
|
'Ensure Observatory and DevTools assets are available with '
|
|
'${authCodesEnabled ? '' : 'no'} auth codes', () async {
|
|
dds = await DartDevelopmentService.startDartDevelopmentService(
|
|
remoteVmServiceUri,
|
|
devToolsConfiguration: DevToolsConfiguration(
|
|
enable: true,
|
|
customBuildDirectoryPath: Platform.script.resolve(
|
|
'../../../third_party/devtools/web',
|
|
),
|
|
),
|
|
);
|
|
expect(dds.isRunning, true);
|
|
|
|
final client = HttpClient();
|
|
|
|
// Check that Observatory assets are accessible.
|
|
final observatoryRequest = await client.getUrl(dds.uri);
|
|
final observatoryResponse = await observatoryRequest.close();
|
|
expect(observatoryResponse.statusCode, 200);
|
|
final observatoryContent =
|
|
await observatoryResponse.transform(utf8.decoder).join();
|
|
expect(observatoryContent, startsWith('<!DOCTYPE html>'));
|
|
|
|
// Check that DevTools assets are accessible.
|
|
final devtoolsRequest = await client.getUrl(dds.devToolsUri);
|
|
final devtoolsResponse = await devtoolsRequest.close();
|
|
expect(devtoolsResponse.statusCode, 200);
|
|
final devtoolsContent =
|
|
await devtoolsResponse.transform(utf8.decoder).join();
|
|
expect(devtoolsContent, startsWith('<!DOCTYPE html>'));
|
|
});
|
|
}
|
|
|
|
defineTest(authCodesEnabled: true);
|
|
defineTest(authCodesEnabled: false);
|
|
}
|