mirror of
https://github.com/dart-lang/sdk
synced 2024-09-20 07:31:33 +00:00
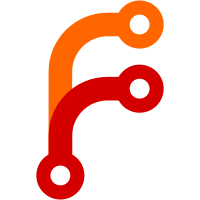
You can use the same constructor multiple times in a single constant evaluation. For example: class A { final List<A> deps; const A({this.deps: const []}); } const a = const A(); const b = const A(deps: const [a]); main() { print(b); } The program above will evaluate the 'A' constructor multiple times when compiling the constant for 'b', but that's okay, as long as we don't examine the same field (or local) multiple times. Change-Id: Icda198ccb47ea91a046134f55deba9041fcf1f4a Reviewed-on: https://dart-review.googlesource.com/15989 Commit-Queue: Harry Terkelsen <het@google.com> Reviewed-by: Emily Fortuna <efortuna@google.com>
16 lines
379 B
Dart
16 lines
379 B
Dart
// Copyright (c) 2017, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
class A {
|
|
final List<A> children;
|
|
const A({this.children: const []});
|
|
}
|
|
|
|
const a = const A();
|
|
const b = const A(children: const [a]);
|
|
|
|
main() {
|
|
print(b);
|
|
}
|