mirror of
https://github.com/dart-lang/sdk
synced 2024-09-05 16:41:07 +00:00
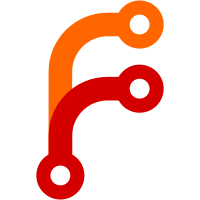
Closes: https://github.com/dart-lang/sdk/issues/40233 This CL creates nnbd versions of the tests and runs them on the nnbd sdk. This CL does not (1) migrate sdk_nnbd/lib/ffi fully yet, and does not (2) fix all the tests/ffi (which is NNBD tests) yet. Uncovered new issues: Issue: https://github.com/dart-lang/sdk/issues/40234 nullptr should have type Pointer<Never>. Issue: https://github.com/dart-lang/sdk/issues/40247 Structs need external fields. Issue: https://github.com/dart-lang/sdk/issues/40271 Callbacks hit assert in debug. Change-Id: Icb1b83577e03ed283165eb17703fc8dfc7fa5960 Cq-Include-Trybots: luci.dart.try:vm-ffi-android-debug-arm-try,vm-ffi-android-debug-arm64-try,app-kernel-linux-debug-x64-try,vm-kernel-linux-debug-ia32-try,vm-kernel-win-debug-x64-try,vm-kernel-win-debug-ia32-try,vm-kernel-precomp-linux-debug-x64-try,vm-dartkb-linux-release-x64-abi-try,vm-kernel-precomp-android-release-arm64-try,vm-kernel-asan-linux-release-x64-try,vm-kernel-linux-release-simarm-try,vm-kernel-linux-release-simarm64-try,vm-kernel-precomp-android-release-arm_x64-try,vm-kernel-precomp-obfuscate-linux-release-x64-try,dart-sdk-linux-try,analyzer-analysis-server-linux-try,analyzer-linux-release-try,front-end-linux-release-x64-try,vm-kernel-precomp-win-release-x64-try,analyzer-nnbd-linux-release-try,dart2js-nnbd-linux-x64-chrome-try,ddc-nnbd-linux-release-chrome-try,front-end-nnbd-linux-release-x64-try,vm-kernel-nnbd-linux-debug-x64-try,vm-kernel-nnbd-linux-release-x64-try Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/132604 Commit-Queue: Daco Harkes <dacoharkes@google.com> Reviewed-by: Alexander Thomas <athom@google.com> Reviewed-by: Bob Nystrom <rnystrom@google.com>
52 lines
1.6 KiB
Dart
52 lines
1.6 KiB
Dart
// Copyright (c) 2020, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
//
|
|
// Dart test program for testing dart:ffi function pointers.
|
|
//
|
|
// VMOptions=
|
|
// VMOptions=--deterministic --optimization-counter-threshold=10
|
|
// VMOptions=--use-slow-path
|
|
// VMOptions=--use-slow-path --stacktrace-every=100
|
|
// VMOptions=--write-protect-code --no-dual-map-code
|
|
// VMOptions=--write-protect-code --no-dual-map-code --use-slow-path
|
|
// VMOptions=--write-protect-code --no-dual-map-code --stacktrace-every=100
|
|
// SharedObjects=ffi_test_functions
|
|
|
|
import 'dart:ffi';
|
|
|
|
import "package:expect/expect.dart";
|
|
|
|
import 'callback_tests_utils.dart';
|
|
import 'dylib_utils.dart';
|
|
|
|
void main() {
|
|
for (int i = 0; i < 100; ++i) {
|
|
testSumFloatsAndDoubles();
|
|
testSumFloatsAndDoublesCallback();
|
|
}
|
|
}
|
|
|
|
DynamicLibrary ffiTestFunctions = dlopenPlatformSpecific("ffi_test_functions");
|
|
|
|
final sumFloatsAndDoubles = ffiTestFunctions.lookupFunction<
|
|
Double Function(Float, Double, Float),
|
|
double Function(double, double, double)>("SumFloatsAndDoubles");
|
|
|
|
void testSumFloatsAndDoubles() {
|
|
Expect.approxEquals(6.0, sumFloatsAndDoubles(1.0, 2.0, 3.0));
|
|
}
|
|
|
|
void testSumFloatsAndDoublesCallback() {
|
|
CallbackTest(
|
|
"SumFloatsAndDoubles",
|
|
Pointer.fromFunction<Double Function(Float, Double, Float)>(
|
|
sumFloatsAndDoublesDart, 0.0))
|
|
.run();
|
|
}
|
|
|
|
double sumFloatsAndDoublesDart(double a, double b, double c) {
|
|
print("sumFloatsAndDoublesDart($a, $b, $c)");
|
|
return a + b + c;
|
|
}
|