mirror of
https://github.com/dart-lang/sdk
synced 2024-10-14 13:18:09 +00:00
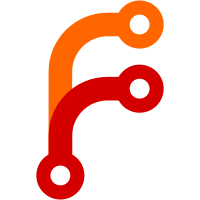
This never worked. It was silently accepted, at least if the future had no listeners, then any later attempt to use the future would cause a stack overflow or other impossible results. Moves some `_Future._complete` call out of try-catch. The `_complete` shouldn't throw (but before this fix it could). Moving them out of the `try`/`catch` makes such errors be reported as unhandled, instead of catching them and trying to complete the same future again with an error, when it's possibly in an inconsistent state. Fixes #43662. Based on https://github.com/dart-lang/sdk/issues/43662#issuecomment-2058870247 CoreLibraryReviewExempt: No response. Bug: http://dartbug.com/43662 Change-Id: I96a4f01bcd5b6cee93bba267299852569a9b905c Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/363060 Commit-Queue: Lasse Nielsen <lrn@google.com> Reviewed-by: Nate Bosch <nbosch@google.com> Reviewed-by: Martin Kustermann <kustermann@google.com>
57 lines
1.1 KiB
Dart
57 lines
1.1 KiB
Dart
// Copyright (c) 2013, the Dart project authors. Please see the AUTHORS file
|
|
// for details. All rights reserved. Use of this source code is governed by a
|
|
// BSD-style license that can be found in the LICENSE file.
|
|
|
|
import 'dart:async';
|
|
|
|
import 'package:async_helper/async_helper.dart';
|
|
import 'package:expect/expect.dart';
|
|
|
|
import 'catch_errors.dart';
|
|
|
|
class A {
|
|
add(x) => print(x);
|
|
}
|
|
|
|
var events = [];
|
|
|
|
body() {
|
|
events.add("body entry");
|
|
scheduleMicrotask(() {
|
|
events.add("run async body");
|
|
throw "foo";
|
|
});
|
|
return 499;
|
|
}
|
|
|
|
onAsyncHandler(fun) {
|
|
events.add("async handler");
|
|
scheduleMicrotask(fun);
|
|
events.add("async handler done");
|
|
}
|
|
|
|
onErrorHandler(e) {
|
|
events.add("error: $e");
|
|
}
|
|
|
|
main() {
|
|
asyncStart();
|
|
|
|
// Test that runZonedScheduleMicrotask works when async, error and done
|
|
// are used.
|
|
var result = runZonedScheduleMicrotask(body,
|
|
onScheduleMicrotask: onAsyncHandler, onError: onErrorHandler);
|
|
events.add("after");
|
|
Timer.run(() {
|
|
Expect.listEquals([
|
|
"body entry",
|
|
"async handler",
|
|
"async handler done",
|
|
"after",
|
|
"run async body",
|
|
"error: foo"
|
|
], events);
|
|
asyncEnd();
|
|
});
|
|
}
|