mirror of
https://github.com/python/cpython
synced 2024-09-19 21:01:47 +00:00
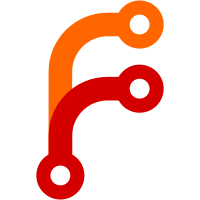
Check additional include directories for SSL Don't build modules that are linked into the Python binary statically Factored out the detection of Tkinter out into a method, since it's the most complicated module to set up Simplify the logic for detecting Tkinter
527 lines
22 KiB
Python
527 lines
22 KiB
Python
# To be fixed:
|
|
# Implement --disable-modules setting
|
|
|
|
import sys, os, string, getopt
|
|
from distutils import sysconfig
|
|
from distutils.core import Extension, setup
|
|
from distutils.command.build_ext import build_ext
|
|
|
|
# This global variable is used to hold the list of modules to be disabled.
|
|
disabled_module_list = []
|
|
|
|
def find_file(filename, std_dirs, paths):
|
|
"""Searches for the directory where a given file is located,
|
|
and returns a possibly-empty list of additional directories, or None
|
|
if the file couldn't be found at all.
|
|
|
|
'filename' is the name of a file, such as readline.h or libcrypto.a.
|
|
'std_dirs' is the list of standard system directories; if the
|
|
file is found in one of them, no additional directives are needed.
|
|
'paths' is a list of additional locations to check; if the file is
|
|
found in one of them, the resulting list will contain the directory.
|
|
"""
|
|
|
|
# Check the standard locations
|
|
for dir in std_dirs:
|
|
f = os.path.join(dir, filename)
|
|
if os.path.exists(f): return []
|
|
|
|
# Check the additional directories
|
|
for dir in paths:
|
|
f = os.path.join(dir, filename)
|
|
if os.path.exists(f):
|
|
return [dir]
|
|
|
|
# Not found anywhere
|
|
return None
|
|
|
|
def find_library_file(compiler, libname, std_dirs, paths):
|
|
filename = compiler.library_filename(libname, lib_type='shared')
|
|
result = find_file(filename, std_dirs, paths)
|
|
if result is not None: return result
|
|
|
|
filename = compiler.library_filename(libname, lib_type='static')
|
|
result = find_file(filename, std_dirs, paths)
|
|
return result
|
|
|
|
def module_enabled(extlist, modname):
|
|
"""Returns whether the module 'modname' is present in the list
|
|
of extensions 'extlist'."""
|
|
extlist = [ext for ext in extlist if ext.name == modname]
|
|
return len(extlist)
|
|
|
|
class PyBuildExt(build_ext):
|
|
|
|
def build_extensions(self):
|
|
from distutils.ccompiler import new_compiler
|
|
from distutils.sysconfig import customize_compiler
|
|
|
|
# Detect which modules should be compiled
|
|
self.detect_modules()
|
|
|
|
# Remove modules that are present on the disabled list
|
|
self.extensions = [ext for ext in self.extensions
|
|
if ext.name not in disabled_module_list]
|
|
|
|
# Fix up the autodetected modules, prefixing all the source files
|
|
# with Modules/ and adding Python's include directory to the path.
|
|
(srcdir,) = sysconfig.get_config_vars('srcdir')
|
|
|
|
#
|
|
moddir = os.path.join(os.getcwd(), 'Modules', srcdir)
|
|
moddir = os.path.normpath(moddir)
|
|
srcdir, tail = os.path.split(moddir)
|
|
srcdir = os.path.normpath(srcdir)
|
|
moddir = os.path.normpath(moddir)
|
|
|
|
for ext in self.extensions[:]:
|
|
ext.sources = [ os.path.join(moddir, filename)
|
|
for filename in ext.sources ]
|
|
ext.include_dirs.append( '.' ) # to get config.h
|
|
|
|
# Try importing a module; if it's already been built statically,
|
|
# don't build it here
|
|
try:
|
|
__import__(ext.name)
|
|
except ImportError:
|
|
pass # Not built, so this is what we expect
|
|
else:
|
|
self.extensions.remove(ext)
|
|
|
|
build_ext.build_extensions(self)
|
|
|
|
def detect_modules(self):
|
|
# Ensure that /usr/local is always used
|
|
if '/usr/local/lib' not in self.compiler.library_dirs:
|
|
self.compiler.library_dirs.append('/usr/local/lib')
|
|
if '/usr/local/include' not in self.compiler.include_dirs:
|
|
self.compiler.include_dirs.append( '/usr/local/include' )
|
|
|
|
# lib_dirs and inc_dirs are used to search for files;
|
|
# if a file is found in one of those directories, it can
|
|
# be assumed that no additional -I,-L directives are needed.
|
|
lib_dirs = self.compiler.library_dirs + ['/lib', '/usr/lib']
|
|
inc_dirs = ['/usr/include'] + self.compiler.include_dirs
|
|
exts = []
|
|
|
|
# XXX Omitted modules: gl, pure, dl, SGI-specific modules
|
|
|
|
#
|
|
# The following modules are all pretty straightforward, and compile
|
|
# on pretty much any POSIXish platform.
|
|
#
|
|
|
|
# Some modules that are normally always on:
|
|
exts.append( Extension('regex', ['regexmodule.c', 'regexpr.c']) )
|
|
exts.append( Extension('pcre', ['pcremodule.c', 'pypcre.c']) )
|
|
|
|
exts.append( Extension('xreadlines', ['xreadlinesmodule.c']) )
|
|
|
|
# array objects
|
|
exts.append( Extension('array', ['arraymodule.c']) )
|
|
# complex math library functions
|
|
exts.append( Extension('cmath', ['cmathmodule.c'], libraries=['m']) )
|
|
# math library functions, e.g. sin()
|
|
exts.append( Extension('math', ['mathmodule.c'], libraries=['m']) )
|
|
# fast string operations implemented in C
|
|
exts.append( Extension('strop', ['stropmodule.c']) )
|
|
# time operations and variables
|
|
exts.append( Extension('time', ['timemodule.c'], libraries=['m']) )
|
|
# operator.add() and similar goodies
|
|
exts.append( Extension('operator', ['operator.c']) )
|
|
# access to the builtin codecs and codec registry
|
|
exts.append( Extension('_codecs', ['_codecsmodule.c']) )
|
|
# static Unicode character database
|
|
exts.append( Extension('unicodedata', ['unicodedata.c', 'unicodedatabase.c']) )
|
|
# Unicode Character Name expansion hash table
|
|
exts.append( Extension('ucnhash', ['ucnhash.c']) )
|
|
# access to ISO C locale support
|
|
exts.append( Extension('_locale', ['_localemodule.c']) )
|
|
|
|
# Modules with some UNIX dependencies -- on by default:
|
|
# (If you have a really backward UNIX, select and socket may not be
|
|
# supported...)
|
|
|
|
# fcntl(2) and ioctl(2)
|
|
exts.append( Extension('fcntl', ['fcntlmodule.c']) )
|
|
# pwd(3)
|
|
exts.append( Extension('pwd', ['pwdmodule.c']) )
|
|
# grp(3)
|
|
exts.append( Extension('grp', ['grpmodule.c']) )
|
|
# posix (UNIX) errno values
|
|
exts.append( Extension('errno', ['errnomodule.c']) )
|
|
# select(2); not on ancient System V
|
|
exts.append( Extension('select', ['selectmodule.c']) )
|
|
|
|
# The md5 module implements the RSA Data Security, Inc. MD5
|
|
# Message-Digest Algorithm, described in RFC 1321. The necessary files
|
|
# md5c.c and md5.h are included here.
|
|
exts.append( Extension('md5', ['md5module.c', 'md5c.c']) )
|
|
|
|
# The sha module implements the SHA checksum algorithm.
|
|
# (NIST's Secure Hash Algorithm.)
|
|
exts.append( Extension('sha', ['shamodule.c']) )
|
|
|
|
# Tommy Burnette's 'new' module (creates new empty objects of certain kinds):
|
|
exts.append( Extension('new', ['newmodule.c']) )
|
|
|
|
# Helper module for various ascii-encoders
|
|
exts.append( Extension('binascii', ['binascii.c']) )
|
|
|
|
# Fred Drake's interface to the Python parser
|
|
exts.append( Extension('parser', ['parsermodule.c']) )
|
|
|
|
# Digital Creations' cStringIO and cPickle
|
|
exts.append( Extension('cStringIO', ['cStringIO.c']) )
|
|
exts.append( Extension('cPickle', ['cPickle.c']) )
|
|
|
|
# Memory-mapped files (also works on Win32).
|
|
exts.append( Extension('mmap', ['mmapmodule.c']) )
|
|
|
|
# Lance Ellinghaus's modules:
|
|
# enigma-inspired encryption
|
|
exts.append( Extension('rotor', ['rotormodule.c']) )
|
|
# syslog daemon interface
|
|
exts.append( Extension('syslog', ['syslogmodule.c']) )
|
|
|
|
# George Neville-Neil's timing module:
|
|
exts.append( Extension('timing', ['timingmodule.c']) )
|
|
|
|
#
|
|
# Here ends the simple stuff. From here on, modules need certain libraries,
|
|
# are platform-specific, or present other surprises.
|
|
#
|
|
|
|
# Multimedia modules
|
|
# These don't work for 64-bit platforms!!!
|
|
# These represent audio samples or images as strings:
|
|
|
|
# Disabled on 64-bit platforms
|
|
if sys.maxint != 9223372036854775807L:
|
|
# Operations on audio samples
|
|
exts.append( Extension('audioop', ['audioop.c']) )
|
|
# Operations on images
|
|
exts.append( Extension('imageop', ['imageop.c']) )
|
|
# Read SGI RGB image files (but coded portably)
|
|
exts.append( Extension('rgbimg', ['rgbimgmodule.c']) )
|
|
|
|
# readline
|
|
if (self.compiler.find_library_file(lib_dirs, 'readline')):
|
|
exts.append( Extension('readline', ['readline.c'],
|
|
libraries=['readline', 'termcap']) )
|
|
|
|
# The crypt module is now disabled by default because it breaks builds
|
|
# on many systems (where -lcrypt is needed), e.g. Linux (I believe).
|
|
|
|
if self.compiler.find_library_file(lib_dirs, 'crypt'):
|
|
libs = ['crypt']
|
|
else:
|
|
libs = []
|
|
exts.append( Extension('crypt', ['cryptmodule.c'], libraries=libs) )
|
|
|
|
# socket(2)
|
|
# Detect SSL support for the socket module
|
|
ssl_incs = find_file('openssl/ssl.h', inc_dirs,
|
|
['/usr/local/ssl/include']
|
|
)
|
|
ssl_libs = find_library_file(self.compiler, 'ssl',lib_dirs,
|
|
['/usr/local/ssl/lib'] )
|
|
|
|
if (ssl_incs is not None and
|
|
ssl_libs is not None):
|
|
exts.append( Extension('_socket', ['socketmodule.c'],
|
|
include_dirs = ssl_incs,
|
|
library_dirs = ssl_libs,
|
|
libraries = ['ssl', 'crypto'],
|
|
define_macros = [('USE_SSL',1)] ) )
|
|
else:
|
|
exts.append( Extension('_socket', ['socketmodule.c']) )
|
|
|
|
# Modules that provide persistent dictionary-like semantics. You will
|
|
# probably want to arrange for at least one of them to be available on
|
|
# your machine, though none are defined by default because of library
|
|
# dependencies. The Python module anydbm.py provides an
|
|
# implementation independent wrapper for these; dumbdbm.py provides
|
|
# similar functionality (but slower of course) implemented in Python.
|
|
|
|
# The standard Unix dbm module:
|
|
if (self.compiler.find_library_file(lib_dirs, 'ndbm')):
|
|
exts.append( Extension('dbm', ['dbmmodule.c'],
|
|
libraries = ['ndbm'] ) )
|
|
else:
|
|
exts.append( Extension('dbm', ['dbmmodule.c']) )
|
|
|
|
# Anthony Baxter's gdbm module. GNU dbm(3) will require -lgdbm:
|
|
if (self.compiler.find_library_file(lib_dirs, 'gdbm')):
|
|
exts.append( Extension('gdbm', ['gdbmmodule.c'],
|
|
libraries = ['gdbm'] ) )
|
|
|
|
# Berkeley DB interface.
|
|
#
|
|
# This requires the Berkeley DB code, see
|
|
# ftp://ftp.cs.berkeley.edu/pub/4bsd/db.1.85.tar.gz
|
|
#
|
|
# Edit the variables DB and DBPORT to point to the db top directory
|
|
# and the subdirectory of PORT where you built it.
|
|
#
|
|
# (See http://electricrain.com/greg/python/bsddb3/ for an interface to
|
|
# BSD DB 3.x.)
|
|
|
|
# Note: If a db.h file is found by configure, bsddb will be enabled
|
|
# automatically via Setup.config.in. It only needs to be enabled here
|
|
# if it is not automatically enabled there; check the generated
|
|
# Setup.config before enabling it here.
|
|
|
|
db_incs = find_file('db_185.h', inc_dirs, [])
|
|
if (db_incs is not None and
|
|
self.compiler.find_library_file(lib_dirs, 'db') ):
|
|
exts.append( Extension('bsddb', ['bsddbmodule.c'],
|
|
include_dirs = db_incs,
|
|
libraries = ['db'] ) )
|
|
|
|
# The mpz module interfaces to the GNU Multiple Precision library.
|
|
# You need to ftp the GNU MP library.
|
|
# This was originally written and tested against GMP 1.2 and 1.3.2.
|
|
# It has been modified by Rob Hooft to work with 2.0.2 as well, but I
|
|
# haven't tested it recently. For a more complete module,
|
|
# refer to pympz.sourceforge.net.
|
|
|
|
# A compatible MP library unencombered by the GPL also exists. It was
|
|
# posted to comp.sources.misc in volume 40 and is widely available from
|
|
# FTP archive sites. One URL for it is:
|
|
# ftp://gatekeeper.dec.com/.b/usenet/comp.sources.misc/volume40/fgmp/part01.Z
|
|
|
|
# Anthony Baxter's gdbm module. GNU dbm(3) will require -lgdbm:
|
|
if (self.compiler.find_library_file(lib_dirs, 'gmp')):
|
|
exts.append( Extension('mpz', ['mpzmodule.c'],
|
|
libraries = ['gmp'] ) )
|
|
|
|
|
|
# Unix-only modules
|
|
if sys.platform not in ['mac', 'win32']:
|
|
# Steen Lumholt's termios module
|
|
exts.append( Extension('termios', ['termios.c']) )
|
|
# Jeremy Hylton's rlimit interface
|
|
exts.append( Extension('resource', ['resource.c']) )
|
|
|
|
if (self.compiler.find_library_file(lib_dirs, 'nsl')):
|
|
exts.append( Extension('nis', ['nismodule.c'],
|
|
libraries = ['nsl']) )
|
|
|
|
# Curses support, requring the System V version of curses, often
|
|
# provided by the ncurses library.
|
|
if sys.platform == 'sunos4':
|
|
include_dirs += ['/usr/5include']
|
|
lib_dirs += ['/usr/5lib']
|
|
|
|
if (self.compiler.find_library_file(lib_dirs, 'ncurses')):
|
|
curses_libs = ['ncurses']
|
|
exts.append( Extension('_curses', ['_cursesmodule.c'],
|
|
libraries = curses_libs) )
|
|
elif (self.compiler.find_library_file(lib_dirs, 'curses')):
|
|
if (self.compiler.find_library_file(lib_dirs, 'terminfo')):
|
|
curses_libs = ['curses', 'terminfo']
|
|
else:
|
|
curses_libs = ['curses', 'termcap']
|
|
|
|
exts.append( Extension('_curses', ['_cursesmodule.c'],
|
|
libraries = curses_libs) )
|
|
|
|
# If the curses module is enabled, check for the panel module
|
|
if (os.path.exists('Modules/_curses_panel.c') and
|
|
module_enabled(exts, '_curses') and
|
|
self.compiler.find_library_file(lib_dirs, 'panel')):
|
|
exts.append( Extension('_curses_panel', ['_curses_panel.c'],
|
|
libraries = ['panel'] + curses_libs) )
|
|
|
|
|
|
|
|
# Lee Busby's SIGFPE modules.
|
|
# The library to link fpectl with is platform specific.
|
|
# Choose *one* of the options below for fpectl:
|
|
|
|
if sys.platform == 'irix5':
|
|
# For SGI IRIX (tested on 5.3):
|
|
exts.append( Extension('fpectl', ['fpectlmodule.c'],
|
|
libraries=['fpe']) )
|
|
elif 0: # XXX how to detect SunPro?
|
|
# For Solaris with SunPro compiler (tested on Solaris 2.5 with SunPro C 4.2):
|
|
# (Without the compiler you don't have -lsunmath.)
|
|
#fpectl fpectlmodule.c -R/opt/SUNWspro/lib -lsunmath -lm
|
|
pass
|
|
else:
|
|
# For other systems: see instructions in fpectlmodule.c.
|
|
#fpectl fpectlmodule.c ...
|
|
exts.append( Extension('fpectl', ['fpectlmodule.c']) )
|
|
|
|
|
|
# Andrew Kuchling's zlib module.
|
|
# This require zlib 1.1.3 (or later).
|
|
# See http://www.cdrom.com/pub/infozip/zlib/
|
|
if (self.compiler.find_library_file(lib_dirs, 'z')):
|
|
exts.append( Extension('zlib', ['zlibmodule.c'],
|
|
libraries = ['z']) )
|
|
|
|
# Interface to the Expat XML parser
|
|
#
|
|
# Expat is written by James Clark and must be downloaded separately
|
|
# (see below). The pyexpat module was written by Paul Prescod after a
|
|
# prototype by Jack Jansen.
|
|
#
|
|
# The Expat dist includes Windows .lib and .dll files. Home page is at
|
|
# http://www.jclark.com/xml/expat.html, the current production release is
|
|
# always ftp://ftp.jclark.com/pub/xml/expat.zip.
|
|
#
|
|
# EXPAT_DIR, below, should point to the expat/ directory created by
|
|
# unpacking the Expat source distribution.
|
|
#
|
|
# Note: the expat build process doesn't yet build a libexpat.a; you can
|
|
# do this manually while we try convince the author to add it. To do so,
|
|
# cd to EXPAT_DIR, run "make" if you have not done so, then run:
|
|
#
|
|
# ar cr libexpat.a xmltok/*.o xmlparse/*.o
|
|
#
|
|
expat_defs = []
|
|
expat_incs = find_file('expat.h', inc_dirs, [])
|
|
if expat_incs is not None:
|
|
# expat.h was found
|
|
expat_defs = [('HAVE_EXPAT_H', 1)]
|
|
else:
|
|
expat_incs = find_file('xmlparse.h', inc_dirs, [])
|
|
|
|
if (expat_incs and
|
|
self.compiler.find_library_file(lib_dirs, 'expat')):
|
|
exts.append( Extension('pyexpat', ['pyexpat.c'],
|
|
define_macros = expat_defs,
|
|
libraries = ['expat']) )
|
|
|
|
# Platform-specific libraries
|
|
plat = sys.platform
|
|
if plat == 'linux2':
|
|
# Linux-specific modules
|
|
exts.append( Extension('linuxaudiodev', ['linuxaudiodev.c']) )
|
|
|
|
if plat == 'sunos5':
|
|
# SunOS specific modules
|
|
exts.append( Extension('sunaudiodev', ['sunaudiodev.c']) )
|
|
|
|
self.extensions.extend(exts)
|
|
|
|
# Call the method for detecting whether _tkinter can be compiled
|
|
self.detect_tkinter(inc_dirs, lib_dirs)
|
|
|
|
|
|
def detect_tkinter(self, inc_dirs, lib_dirs):
|
|
# The _tkinter module.
|
|
#
|
|
# The command for _tkinter is long and site specific. Please
|
|
# uncomment and/or edit those parts as indicated. If you don't have a
|
|
# specific extension (e.g. Tix or BLT), leave the corresponding line
|
|
# commented out. (Leave the trailing backslashes in! If you
|
|
# experience strange errors, you may want to join all uncommented
|
|
# lines and remove the backslashes -- the backslash interpretation is
|
|
# done by the shell's "read" command and it may not be implemented on
|
|
# every system.
|
|
|
|
# Assume we haven't found any of the libraries or include files
|
|
tcllib = tklib = tcl_includes = tk_includes = None
|
|
for version in ['8.4', '8.3', '8.2', '8.1', '8.0']:
|
|
tklib = self.compiler.find_library_file(lib_dirs,
|
|
'tk' + version )
|
|
tcllib = self.compiler.find_library_file(lib_dirs,
|
|
'tcl' + version )
|
|
if tklib and tcllib:
|
|
# Exit the loop when we've found the Tcl/Tk libraries
|
|
break
|
|
|
|
# Now check for the header files
|
|
if tklib and tcllib:
|
|
# Check for the include files on Debian, where
|
|
# they're put in /usr/include/{tcl,tk}X.Y
|
|
debian_tcl_include = ( '/usr/include/tcl' + version )
|
|
debian_tk_include = ( '/usr/include/tk' + version )
|
|
tcl_includes = find_file('tcl.h', inc_dirs,
|
|
[debian_tcl_include]
|
|
)
|
|
tk_includes = find_file('tk.h', inc_dirs,
|
|
[debian_tk_include]
|
|
)
|
|
|
|
if (tcllib is None or tklib is None and
|
|
tcl_includes is None or tk_includes is None):
|
|
# Something's missing, so give up
|
|
return
|
|
|
|
# OK... everything seems to be present for Tcl/Tk.
|
|
|
|
include_dirs = [] ; libs = [] ; defs = [] ; added_lib_dirs = []
|
|
for dir in tcl_includes + tk_includes:
|
|
if dir not in include_dirs:
|
|
include_dirs.append(dir)
|
|
|
|
# Check for various platform-specific directories
|
|
if sys.platform == 'sunos5':
|
|
include_dirs.append('/usr/openwin/include')
|
|
added_lib_dirs.append('/usr/openwin/lib')
|
|
elif os.path.exists('/usr/X11R6/include'):
|
|
include_dirs.append('/usr/X11R6/include')
|
|
added_lib_dirs.append('/usr/X11R6/lib')
|
|
elif os.path.exists('/usr/X11R5/include'):
|
|
include_dirs.append('/usr/X11R5/include')
|
|
added_lib_dirs.append('/usr/X11R5/lib')
|
|
else:
|
|
# Assume default location for X11
|
|
include_dirs.append('/usr/X11/include')
|
|
added_lib_dirs.append('/usr/X11/lib')
|
|
|
|
# Check for Tix extension
|
|
if self.compiler.find_library_file(lib_dirs + added_lib_dirs, 'tix4.1.8.0'):
|
|
defs.append( ('WITH_TIX', 1) )
|
|
libs.append('tix4.1.8.0')
|
|
|
|
# Check for BLT extension
|
|
if self.compiler.find_library_file(lib_dirs + added_lib_dirs, 'BLT8.0'):
|
|
defs.append( ('WITH_BLT', 1) )
|
|
libs.append('BLT8.0')
|
|
|
|
# Add the Tcl/Tk libraries
|
|
libs.append('tk'+version)
|
|
libs.append('tcl'+version)
|
|
|
|
if sys.platform in ['aix3', 'aix4']:
|
|
libs.append('ld')
|
|
|
|
# Finally, link with the X11 libraries
|
|
libs.append('X11')
|
|
|
|
ext = Extension('_tkinter', ['_tkinter.c', 'tkappinit.c'],
|
|
define_macros=[('WITH_APPINIT', 1)] + defs,
|
|
include_dirs = include_dirs,
|
|
libraries = libs,
|
|
library_dirs = added_lib_dirs,
|
|
)
|
|
self.extensions.append(ext)
|
|
|
|
# XXX handle these, but how to detect?
|
|
# *** Uncomment and edit for PIL (TkImaging) extension only:
|
|
# -DWITH_PIL -I../Extensions/Imaging/libImaging tkImaging.c \
|
|
# *** Uncomment and edit for TOGL extension only:
|
|
# -DWITH_TOGL togl.c \
|
|
# *** Uncomment these for TOGL extension only:
|
|
# -lGL -lGLU -lXext -lXmu \
|
|
|
|
def main():
|
|
setup(name = 'Python standard library',
|
|
version = '%d.%d' % sys.version_info[:2],
|
|
cmdclass = {'build_ext':PyBuildExt},
|
|
# The struct module is defined here, because build_ext won't be
|
|
# called unless there's at least one extension module defined.
|
|
ext_modules=[Extension('struct', ['structmodule.c'])]
|
|
)
|
|
|
|
# --install-platlib
|
|
if __name__ == '__main__':
|
|
sysconfig.set_python_build()
|
|
main()
|
|
|