mirror of
https://github.com/python/cpython
synced 2024-11-02 12:55:22 +00:00
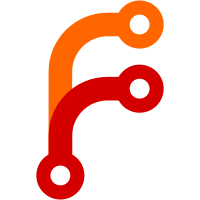
number of tests, all because of the codecs/_multibytecodecs issue described here (it's not a Py3K issue, just something Py3K discovers): http://mail.python.org/pipermail/python-dev/2006-April/064051.html Hye-Shik Chang promised to look for a fix, so no need to fix it here. The tests that are expected to break are: test_codecencodings_cn test_codecencodings_hk test_codecencodings_jp test_codecencodings_kr test_codecencodings_tw test_codecs test_multibytecodec This merge fixes an actual test failure (test_weakref) in this branch, though, so I believe merging is the right thing to do anyway.
66 lines
977 B
C
66 lines
977 B
C
|
|
/* Bitset primitives used by the parser generator */
|
|
|
|
#include "pgenheaders.h"
|
|
#include "bitset.h"
|
|
|
|
bitset
|
|
newbitset(int nbits)
|
|
{
|
|
int nbytes = NBYTES(nbits);
|
|
bitset ss = (char *)PyObject_MALLOC(sizeof(BYTE) * nbytes);
|
|
|
|
if (ss == NULL)
|
|
Py_FatalError("no mem for bitset");
|
|
|
|
ss += nbytes;
|
|
while (--nbytes >= 0)
|
|
*--ss = 0;
|
|
return ss;
|
|
}
|
|
|
|
void
|
|
delbitset(bitset ss)
|
|
{
|
|
PyObject_FREE(ss);
|
|
}
|
|
|
|
int
|
|
addbit(bitset ss, int ibit)
|
|
{
|
|
int ibyte = BIT2BYTE(ibit);
|
|
BYTE mask = BIT2MASK(ibit);
|
|
|
|
if (ss[ibyte] & mask)
|
|
return 0; /* Bit already set */
|
|
ss[ibyte] |= mask;
|
|
return 1;
|
|
}
|
|
|
|
#if 0 /* Now a macro */
|
|
int
|
|
testbit(bitset ss, int ibit)
|
|
{
|
|
return (ss[BIT2BYTE(ibit)] & BIT2MASK(ibit)) != 0;
|
|
}
|
|
#endif
|
|
|
|
int
|
|
samebitset(bitset ss1, bitset ss2, int nbits)
|
|
{
|
|
int i;
|
|
|
|
for (i = NBYTES(nbits); --i >= 0; )
|
|
if (*ss1++ != *ss2++)
|
|
return 0;
|
|
return 1;
|
|
}
|
|
|
|
void
|
|
mergebitset(bitset ss1, bitset ss2, int nbits)
|
|
{
|
|
int i;
|
|
|
|
for (i = NBYTES(nbits); --i >= 0; )
|
|
*ss1++ |= *ss2++;
|
|
}
|