mirror of
https://github.com/python/cpython
synced 2024-09-04 15:56:13 +00:00
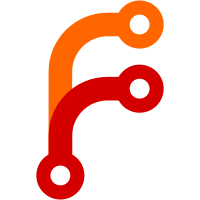
svn+ssh://pythondev@svn.python.org/python/trunk ........ r61981 | amaury.forgeotdarc | 2008-03-28 01:21:34 +0100 (Fri, 28 Mar 2008) | 2 lines test_future3.py is a regular test file, and should be part of the test suite ........ r61984 | jeffrey.yasskin | 2008-03-28 05:11:18 +0100 (Fri, 28 Mar 2008) | 6 lines Kill a race in test_threading in which the exception info in a thread finishing up after it was joined had a traceback pointing to that thread's (deleted) target attribute, while the test was trying to check that the target was destroyed. Big thanks to Antoine Pitrou for diagnosing the race and pointing out sys.exc_clear() to kill the exception early. This fixes issue 2496. ........ r61985 | neal.norwitz | 2008-03-28 05:41:34 +0100 (Fri, 28 Mar 2008) | 1 line Allow use of other ports so the test can pass if 9091 is in use ........ r61986 | jeffrey.yasskin | 2008-03-28 05:53:10 +0100 (Fri, 28 Mar 2008) | 2 lines Print more information the next time test_socket throws the wrong exception. ........ r61987 | neal.norwitz | 2008-03-28 05:58:51 +0100 (Fri, 28 Mar 2008) | 5 lines Revert r61969 which added casts to Py_CHARMASK to avoid compiler warnings. Rather than sprinkle casts throughout the code, change Py_CHARMASK to always cast it's result to an unsigned char. This should ensure we do the right thing when accessing an array with the result. ........ r61992 | neal.norwitz | 2008-03-28 06:34:59 +0100 (Fri, 28 Mar 2008) | 2 lines Fix compiler warning about finite() missing on Solaris. ........ r61993 | neal.norwitz | 2008-03-28 07:34:03 +0100 (Fri, 28 Mar 2008) | 11 lines Bug 1503: Get the test to pass on OSX. This should make the test more reliable, but I'm not convinced it is the right solution. We need to determine if this causes the test to hang on any platforms or do other bad things. Even if it gets the test to pass reliably, it might be that we want to fix this in socket. The socket returned from accept() is different on different platforms (inheriting attributes or not) and we might want to ensure that the attributes (at least blocking) is the same across all platforms. ........ r61997 | neal.norwitz | 2008-03-28 08:36:31 +0100 (Fri, 28 Mar 2008) | 1 line Name the main method correctly so the test is run ........ r61998 | gregory.p.smith | 2008-03-28 09:00:44 +0100 (Fri, 28 Mar 2008) | 7 lines This patch moves some tests from test_urllib2_net to test_urllib2_localnet. The moved tests use a local server rather than going out to external servers. Accepts patch from issue2429. Contributed by Jerry Seutter & Michael Foord (fuzzyman) at PyCon 2008. ........ r61999 | georg.brandl | 2008-03-28 09:06:56 +0100 (Fri, 28 Mar 2008) | 2 lines #2406: add examples to gzip docs. ........ r62000 | gregory.p.smith | 2008-03-28 09:32:09 +0100 (Fri, 28 Mar 2008) | 4 lines Accept patch issue2426 by Paul Kippes (kippesp). Adds sqlite3.Connection.iterdump to allow dumping of databases. ........
868 lines
28 KiB
Python
868 lines
28 KiB
Python
"""Thread module emulating a subset of Java's threading model."""
|
|
|
|
import sys as _sys
|
|
|
|
try:
|
|
import thread
|
|
except ImportError:
|
|
del _sys.modules[__name__]
|
|
raise
|
|
|
|
from time import time as _time, sleep as _sleep
|
|
from traceback import format_exc as _format_exc
|
|
from collections import deque
|
|
|
|
# Rename some stuff so "from threading import *" is safe
|
|
__all__ = ['activeCount', 'Condition', 'currentThread', 'enumerate', 'Event',
|
|
'Lock', 'RLock', 'Semaphore', 'BoundedSemaphore', 'Thread',
|
|
'Timer', 'setprofile', 'settrace', 'local', 'stack_size']
|
|
|
|
_start_new_thread = thread.start_new_thread
|
|
_allocate_lock = thread.allocate_lock
|
|
_get_ident = thread.get_ident
|
|
ThreadError = thread.error
|
|
del thread
|
|
|
|
|
|
# Debug support (adapted from ihooks.py).
|
|
# All the major classes here derive from _Verbose. We force that to
|
|
# be a new-style class so that all the major classes here are new-style.
|
|
# This helps debugging (type(instance) is more revealing for instances
|
|
# of new-style classes).
|
|
|
|
_VERBOSE = False
|
|
|
|
if __debug__:
|
|
|
|
class _Verbose(object):
|
|
|
|
def __init__(self, verbose=None):
|
|
if verbose is None:
|
|
verbose = _VERBOSE
|
|
self._verbose = verbose
|
|
|
|
def _note(self, format, *args):
|
|
if self._verbose:
|
|
format = format % args
|
|
format = "%s: %s\n" % (
|
|
currentThread().getName(), format)
|
|
_sys.stderr.write(format)
|
|
|
|
else:
|
|
# Disable this when using "python -O"
|
|
class _Verbose(object):
|
|
def __init__(self, verbose=None):
|
|
pass
|
|
def _note(self, *args):
|
|
pass
|
|
|
|
# Support for profile and trace hooks
|
|
|
|
_profile_hook = None
|
|
_trace_hook = None
|
|
|
|
def setprofile(func):
|
|
global _profile_hook
|
|
_profile_hook = func
|
|
|
|
def settrace(func):
|
|
global _trace_hook
|
|
_trace_hook = func
|
|
|
|
# Synchronization classes
|
|
|
|
Lock = _allocate_lock
|
|
|
|
def RLock(*args, **kwargs):
|
|
return _RLock(*args, **kwargs)
|
|
|
|
class _RLock(_Verbose):
|
|
|
|
def __init__(self, verbose=None):
|
|
_Verbose.__init__(self, verbose)
|
|
self._block = _allocate_lock()
|
|
self._owner = None
|
|
self._count = 0
|
|
|
|
def __repr__(self):
|
|
owner = self._owner
|
|
return "<%s(%s, %d)>" % (
|
|
self.__class__.__name__,
|
|
owner and owner.getName(),
|
|
self._count)
|
|
|
|
def acquire(self, blocking=1):
|
|
me = currentThread()
|
|
if self._owner is me:
|
|
self._count = self._count + 1
|
|
if __debug__:
|
|
self._note("%s.acquire(%s): recursive success", self, blocking)
|
|
return 1
|
|
rc = self._block.acquire(blocking)
|
|
if rc:
|
|
self._owner = me
|
|
self._count = 1
|
|
if __debug__:
|
|
self._note("%s.acquire(%s): initial success", self, blocking)
|
|
else:
|
|
if __debug__:
|
|
self._note("%s.acquire(%s): failure", self, blocking)
|
|
return rc
|
|
|
|
__enter__ = acquire
|
|
|
|
def release(self):
|
|
if self._owner is not currentThread():
|
|
raise RuntimeError("cannot release un-aquired lock")
|
|
self._count = count = self._count - 1
|
|
if not count:
|
|
self._owner = None
|
|
self._block.release()
|
|
if __debug__:
|
|
self._note("%s.release(): final release", self)
|
|
else:
|
|
if __debug__:
|
|
self._note("%s.release(): non-final release", self)
|
|
|
|
def __exit__(self, t, v, tb):
|
|
self.release()
|
|
|
|
# Internal methods used by condition variables
|
|
|
|
def _acquire_restore(self, state):
|
|
self._block.acquire()
|
|
self._count, self._owner = state
|
|
if __debug__:
|
|
self._note("%s._acquire_restore()", self)
|
|
|
|
def _release_save(self):
|
|
if __debug__:
|
|
self._note("%s._release_save()", self)
|
|
count = self._count
|
|
self._count = 0
|
|
owner = self._owner
|
|
self._owner = None
|
|
self._block.release()
|
|
return (count, owner)
|
|
|
|
def _is_owned(self):
|
|
return self._owner is currentThread()
|
|
|
|
|
|
def Condition(*args, **kwargs):
|
|
return _Condition(*args, **kwargs)
|
|
|
|
class _Condition(_Verbose):
|
|
|
|
def __init__(self, lock=None, verbose=None):
|
|
_Verbose.__init__(self, verbose)
|
|
if lock is None:
|
|
lock = RLock()
|
|
self._lock = lock
|
|
# Export the lock's acquire() and release() methods
|
|
self.acquire = lock.acquire
|
|
self.release = lock.release
|
|
# If the lock defines _release_save() and/or _acquire_restore(),
|
|
# these override the default implementations (which just call
|
|
# release() and acquire() on the lock). Ditto for _is_owned().
|
|
try:
|
|
self._release_save = lock._release_save
|
|
except AttributeError:
|
|
pass
|
|
try:
|
|
self._acquire_restore = lock._acquire_restore
|
|
except AttributeError:
|
|
pass
|
|
try:
|
|
self._is_owned = lock._is_owned
|
|
except AttributeError:
|
|
pass
|
|
self._waiters = []
|
|
|
|
def __enter__(self):
|
|
return self._lock.__enter__()
|
|
|
|
def __exit__(self, *args):
|
|
return self._lock.__exit__(*args)
|
|
|
|
def __repr__(self):
|
|
return "<Condition(%s, %d)>" % (self._lock, len(self._waiters))
|
|
|
|
def _release_save(self):
|
|
self._lock.release() # No state to save
|
|
|
|
def _acquire_restore(self, x):
|
|
self._lock.acquire() # Ignore saved state
|
|
|
|
def _is_owned(self):
|
|
# Return True if lock is owned by currentThread.
|
|
# This method is called only if __lock doesn't have _is_owned().
|
|
if self._lock.acquire(0):
|
|
self._lock.release()
|
|
return False
|
|
else:
|
|
return True
|
|
|
|
def wait(self, timeout=None):
|
|
if not self._is_owned():
|
|
raise RuntimeError("cannot wait on un-aquired lock")
|
|
waiter = _allocate_lock()
|
|
waiter.acquire()
|
|
self._waiters.append(waiter)
|
|
saved_state = self._release_save()
|
|
try: # restore state no matter what (e.g., KeyboardInterrupt)
|
|
if timeout is None:
|
|
waiter.acquire()
|
|
if __debug__:
|
|
self._note("%s.wait(): got it", self)
|
|
else:
|
|
# Balancing act: We can't afford a pure busy loop, so we
|
|
# have to sleep; but if we sleep the whole timeout time,
|
|
# we'll be unresponsive. The scheme here sleeps very
|
|
# little at first, longer as time goes on, but never longer
|
|
# than 20 times per second (or the timeout time remaining).
|
|
endtime = _time() + timeout
|
|
delay = 0.0005 # 500 us -> initial delay of 1 ms
|
|
while True:
|
|
gotit = waiter.acquire(0)
|
|
if gotit:
|
|
break
|
|
remaining = endtime - _time()
|
|
if remaining <= 0:
|
|
break
|
|
delay = min(delay * 2, remaining, .05)
|
|
_sleep(delay)
|
|
if not gotit:
|
|
if __debug__:
|
|
self._note("%s.wait(%s): timed out", self, timeout)
|
|
try:
|
|
self._waiters.remove(waiter)
|
|
except ValueError:
|
|
pass
|
|
else:
|
|
if __debug__:
|
|
self._note("%s.wait(%s): got it", self, timeout)
|
|
finally:
|
|
self._acquire_restore(saved_state)
|
|
|
|
def notify(self, n=1):
|
|
if not self._is_owned():
|
|
raise RuntimeError("cannot notify on un-aquired lock")
|
|
__waiters = self._waiters
|
|
waiters = __waiters[:n]
|
|
if not waiters:
|
|
if __debug__:
|
|
self._note("%s.notify(): no waiters", self)
|
|
return
|
|
self._note("%s.notify(): notifying %d waiter%s", self, n,
|
|
n!=1 and "s" or "")
|
|
for waiter in waiters:
|
|
waiter.release()
|
|
try:
|
|
__waiters.remove(waiter)
|
|
except ValueError:
|
|
pass
|
|
|
|
def notifyAll(self):
|
|
self.notify(len(self._waiters))
|
|
|
|
|
|
def Semaphore(*args, **kwargs):
|
|
return _Semaphore(*args, **kwargs)
|
|
|
|
class _Semaphore(_Verbose):
|
|
|
|
# After Tim Peters' semaphore class, but not quite the same (no maximum)
|
|
|
|
def __init__(self, value=1, verbose=None):
|
|
if value < 0:
|
|
raise ValueError("semaphore initial value must be >= 0")
|
|
_Verbose.__init__(self, verbose)
|
|
self._cond = Condition(Lock())
|
|
self._value = value
|
|
|
|
def acquire(self, blocking=1):
|
|
rc = False
|
|
self._cond.acquire()
|
|
while self._value == 0:
|
|
if not blocking:
|
|
break
|
|
if __debug__:
|
|
self._note("%s.acquire(%s): blocked waiting, value=%s",
|
|
self, blocking, self._value)
|
|
self._cond.wait()
|
|
else:
|
|
self._value = self._value - 1
|
|
if __debug__:
|
|
self._note("%s.acquire: success, value=%s",
|
|
self, self._value)
|
|
rc = True
|
|
self._cond.release()
|
|
return rc
|
|
|
|
__enter__ = acquire
|
|
|
|
def release(self):
|
|
self._cond.acquire()
|
|
self._value = self._value + 1
|
|
if __debug__:
|
|
self._note("%s.release: success, value=%s",
|
|
self, self._value)
|
|
self._cond.notify()
|
|
self._cond.release()
|
|
|
|
def __exit__(self, t, v, tb):
|
|
self.release()
|
|
|
|
|
|
def BoundedSemaphore(*args, **kwargs):
|
|
return _BoundedSemaphore(*args, **kwargs)
|
|
|
|
class _BoundedSemaphore(_Semaphore):
|
|
"""Semaphore that checks that # releases is <= # acquires"""
|
|
def __init__(self, value=1, verbose=None):
|
|
_Semaphore.__init__(self, value, verbose)
|
|
self._initial_value = value
|
|
|
|
def release(self):
|
|
if self._value >= self._initial_value:
|
|
raise ValueError("Semaphore released too many times")
|
|
return _Semaphore.release(self)
|
|
|
|
|
|
def Event(*args, **kwargs):
|
|
return _Event(*args, **kwargs)
|
|
|
|
class _Event(_Verbose):
|
|
|
|
# After Tim Peters' event class (without is_posted())
|
|
|
|
def __init__(self, verbose=None):
|
|
_Verbose.__init__(self, verbose)
|
|
self._cond = Condition(Lock())
|
|
self._flag = False
|
|
|
|
def isSet(self):
|
|
return self._flag
|
|
|
|
def set(self):
|
|
self._cond.acquire()
|
|
try:
|
|
self._flag = True
|
|
self._cond.notifyAll()
|
|
finally:
|
|
self._cond.release()
|
|
|
|
def clear(self):
|
|
self._cond.acquire()
|
|
try:
|
|
self._flag = False
|
|
finally:
|
|
self._cond.release()
|
|
|
|
def wait(self, timeout=None):
|
|
self._cond.acquire()
|
|
try:
|
|
if not self._flag:
|
|
self._cond.wait(timeout)
|
|
finally:
|
|
self._cond.release()
|
|
|
|
# Helper to generate new thread names
|
|
_counter = 0
|
|
def _newname(template="Thread-%d"):
|
|
global _counter
|
|
_counter = _counter + 1
|
|
return template % _counter
|
|
|
|
# Active thread administration
|
|
_active_limbo_lock = _allocate_lock()
|
|
_active = {} # maps thread id to Thread object
|
|
_limbo = {}
|
|
|
|
|
|
# Main class for threads
|
|
|
|
class Thread(_Verbose):
|
|
|
|
__initialized = False
|
|
# Need to store a reference to sys.exc_info for printing
|
|
# out exceptions when a thread tries to use a global var. during interp.
|
|
# shutdown and thus raises an exception about trying to perform some
|
|
# operation on/with a NoneType
|
|
__exc_info = _sys.exc_info
|
|
# Keep sys.exc_clear too to clear the exception just before
|
|
# allowing .join() to return.
|
|
#XXX __exc_clear = _sys.exc_clear
|
|
|
|
def __init__(self, group=None, target=None, name=None,
|
|
args=(), kwargs=None, verbose=None):
|
|
assert group is None, "group argument must be None for now"
|
|
_Verbose.__init__(self, verbose)
|
|
if kwargs is None:
|
|
kwargs = {}
|
|
self._target = target
|
|
self._name = str(name or _newname())
|
|
self._args = args
|
|
self._kwargs = kwargs
|
|
self._daemonic = self._set_daemon()
|
|
self._started = Event()
|
|
self._stopped = False
|
|
self._block = Condition(Lock())
|
|
self._initialized = True
|
|
# sys.stderr is not stored in the class like
|
|
# sys.exc_info since it can be changed between instances
|
|
self._stderr = _sys.stderr
|
|
|
|
def _set_daemon(self):
|
|
# Overridden in _MainThread and _DummyThread
|
|
return currentThread().isDaemon()
|
|
|
|
def __repr__(self):
|
|
assert self._initialized, "Thread.__init__() was not called"
|
|
status = "initial"
|
|
if self._started.isSet():
|
|
status = "started"
|
|
if self._stopped:
|
|
status = "stopped"
|
|
if self._daemonic:
|
|
status = status + " daemon"
|
|
return "<%s(%s, %s)>" % (self.__class__.__name__, self._name, status)
|
|
|
|
def start(self):
|
|
if not self._initialized:
|
|
raise RuntimeError("thread.__init__() not called")
|
|
|
|
if self._started.isSet():
|
|
raise RuntimeError("thread already started")
|
|
if __debug__:
|
|
self._note("%s.start(): starting thread", self)
|
|
_active_limbo_lock.acquire()
|
|
_limbo[self] = self
|
|
_active_limbo_lock.release()
|
|
_start_new_thread(self._bootstrap, ())
|
|
self._started.wait()
|
|
|
|
def run(self):
|
|
try:
|
|
if self._target:
|
|
self._target(*self._args, **self._kwargs)
|
|
finally:
|
|
# Avoid a refcycle if the thread is running a function with
|
|
# an argument that has a member that points to the thread.
|
|
del self._target, self._args, self._kwargs
|
|
|
|
def _bootstrap(self):
|
|
# Wrapper around the real bootstrap code that ignores
|
|
# exceptions during interpreter cleanup. Those typically
|
|
# happen when a daemon thread wakes up at an unfortunate
|
|
# moment, finds the world around it destroyed, and raises some
|
|
# random exception *** while trying to report the exception in
|
|
# _bootstrap_inner() below ***. Those random exceptions
|
|
# don't help anybody, and they confuse users, so we suppress
|
|
# them. We suppress them only when it appears that the world
|
|
# indeed has already been destroyed, so that exceptions in
|
|
# _bootstrap_inner() during normal business hours are properly
|
|
# reported. Also, we only suppress them for daemonic threads;
|
|
# if a non-daemonic encounters this, something else is wrong.
|
|
try:
|
|
self._bootstrap_inner()
|
|
except:
|
|
if self._daemonic and _sys is None:
|
|
return
|
|
raise
|
|
|
|
def _bootstrap_inner(self):
|
|
try:
|
|
self._started.set()
|
|
_active_limbo_lock.acquire()
|
|
_active[_get_ident()] = self
|
|
del _limbo[self]
|
|
_active_limbo_lock.release()
|
|
if __debug__:
|
|
self._note("%s._bootstrap(): thread started", self)
|
|
|
|
if _trace_hook:
|
|
self._note("%s._bootstrap(): registering trace hook", self)
|
|
_sys.settrace(_trace_hook)
|
|
if _profile_hook:
|
|
self._note("%s._bootstrap(): registering profile hook", self)
|
|
_sys.setprofile(_profile_hook)
|
|
|
|
try:
|
|
self.run()
|
|
except SystemExit:
|
|
if __debug__:
|
|
self._note("%s._bootstrap(): raised SystemExit", self)
|
|
except:
|
|
if __debug__:
|
|
self._note("%s._bootstrap(): unhandled exception", self)
|
|
# If sys.stderr is no more (most likely from interpreter
|
|
# shutdown) use self._stderr. Otherwise still use sys (as in
|
|
# _sys) in case sys.stderr was redefined since the creation of
|
|
# self.
|
|
if _sys:
|
|
_sys.stderr.write("Exception in thread %s:\n%s\n" %
|
|
(self.getName(), _format_exc()))
|
|
else:
|
|
# Do the best job possible w/o a huge amt. of code to
|
|
# approximate a traceback (code ideas from
|
|
# Lib/traceback.py)
|
|
exc_type, exc_value, exc_tb = self._exc_info()
|
|
try:
|
|
print((
|
|
"Exception in thread " + self.getName() +
|
|
" (most likely raised during interpreter shutdown):"), file=self._stderr)
|
|
print((
|
|
"Traceback (most recent call last):"), file=self._stderr)
|
|
while exc_tb:
|
|
print((
|
|
' File "%s", line %s, in %s' %
|
|
(exc_tb.tb_frame.f_code.co_filename,
|
|
exc_tb.tb_lineno,
|
|
exc_tb.tb_frame.f_code.co_name)), file=self._stderr)
|
|
exc_tb = exc_tb.tb_next
|
|
print(("%s: %s" % (exc_type, exc_value)), file=self._stderr)
|
|
# Make sure that exc_tb gets deleted since it is a memory
|
|
# hog; deleting everything else is just for thoroughness
|
|
finally:
|
|
del exc_type, exc_value, exc_tb
|
|
else:
|
|
if __debug__:
|
|
self._note("%s._bootstrap(): normal return", self)
|
|
finally:
|
|
# Prevent a race in
|
|
# test_threading.test_no_refcycle_through_target when
|
|
# the exception keeps the target alive past when we
|
|
# assert that it's dead.
|
|
#XXX self.__exc_clear()
|
|
pass
|
|
finally:
|
|
with _active_limbo_lock:
|
|
self._stop()
|
|
try:
|
|
# We don't call self.__delete() because it also
|
|
# grabs _active_limbo_lock.
|
|
del _active[_get_ident()]
|
|
except:
|
|
pass
|
|
|
|
def _stop(self):
|
|
self._block.acquire()
|
|
self._stopped = True
|
|
self._block.notifyAll()
|
|
self._block.release()
|
|
|
|
def _delete(self):
|
|
"Remove current thread from the dict of currently running threads."
|
|
|
|
# Notes about running with dummy_thread:
|
|
#
|
|
# Must take care to not raise an exception if dummy_thread is being
|
|
# used (and thus this module is being used as an instance of
|
|
# dummy_threading). dummy_thread.get_ident() always returns -1 since
|
|
# there is only one thread if dummy_thread is being used. Thus
|
|
# len(_active) is always <= 1 here, and any Thread instance created
|
|
# overwrites the (if any) thread currently registered in _active.
|
|
#
|
|
# An instance of _MainThread is always created by 'threading'. This
|
|
# gets overwritten the instant an instance of Thread is created; both
|
|
# threads return -1 from dummy_thread.get_ident() and thus have the
|
|
# same key in the dict. So when the _MainThread instance created by
|
|
# 'threading' tries to clean itself up when atexit calls this method
|
|
# it gets a KeyError if another Thread instance was created.
|
|
#
|
|
# This all means that KeyError from trying to delete something from
|
|
# _active if dummy_threading is being used is a red herring. But
|
|
# since it isn't if dummy_threading is *not* being used then don't
|
|
# hide the exception.
|
|
|
|
_active_limbo_lock.acquire()
|
|
try:
|
|
try:
|
|
del _active[_get_ident()]
|
|
except KeyError:
|
|
if 'dummy_threading' not in _sys.modules:
|
|
raise
|
|
finally:
|
|
_active_limbo_lock.release()
|
|
|
|
def join(self, timeout=None):
|
|
if not self._initialized:
|
|
raise RuntimeError("Thread.__init__() not called")
|
|
if not self._started.isSet():
|
|
raise RuntimeError("cannot join thread before it is started")
|
|
if self is currentThread():
|
|
raise RuntimeError("cannot join current thread")
|
|
|
|
if __debug__:
|
|
if not self._stopped:
|
|
self._note("%s.join(): waiting until thread stops", self)
|
|
|
|
self._block.acquire()
|
|
try:
|
|
if timeout is None:
|
|
while not self._stopped:
|
|
self._block.wait()
|
|
if __debug__:
|
|
self._note("%s.join(): thread stopped", self)
|
|
else:
|
|
deadline = _time() + timeout
|
|
while not self._stopped:
|
|
delay = deadline - _time()
|
|
if delay <= 0:
|
|
if __debug__:
|
|
self._note("%s.join(): timed out", self)
|
|
break
|
|
self._block.wait(delay)
|
|
else:
|
|
if __debug__:
|
|
self._note("%s.join(): thread stopped", self)
|
|
finally:
|
|
self._block.release()
|
|
|
|
def getName(self):
|
|
assert self._initialized, "Thread.__init__() not called"
|
|
return self._name
|
|
|
|
def setName(self, name):
|
|
assert self._initialized, "Thread.__init__() not called"
|
|
self._name = str(name)
|
|
|
|
def isAlive(self):
|
|
assert self._initialized, "Thread.__init__() not called"
|
|
return self._started.isSet() and not self._stopped
|
|
|
|
def isDaemon(self):
|
|
assert self._initialized, "Thread.__init__() not called"
|
|
return self._daemonic
|
|
|
|
def setDaemon(self, daemonic):
|
|
if not self._initialized:
|
|
raise RuntimeError("Thread.__init__() not called")
|
|
if self._started.isSet():
|
|
raise RuntimeError("cannot set daemon status of active thread");
|
|
self._daemonic = daemonic
|
|
|
|
# The timer class was contributed by Itamar Shtull-Trauring
|
|
|
|
def Timer(*args, **kwargs):
|
|
return _Timer(*args, **kwargs)
|
|
|
|
class _Timer(Thread):
|
|
"""Call a function after a specified number of seconds:
|
|
|
|
t = Timer(30.0, f, args=[], kwargs={})
|
|
t.start()
|
|
t.cancel() # stop the timer's action if it's still waiting
|
|
"""
|
|
|
|
def __init__(self, interval, function, args=[], kwargs={}):
|
|
Thread.__init__(self)
|
|
self.interval = interval
|
|
self.function = function
|
|
self.args = args
|
|
self.kwargs = kwargs
|
|
self.finished = Event()
|
|
|
|
def cancel(self):
|
|
"""Stop the timer if it hasn't finished yet"""
|
|
self.finished.set()
|
|
|
|
def run(self):
|
|
self.finished.wait(self.interval)
|
|
if not self.finished.isSet():
|
|
self.function(*self.args, **self.kwargs)
|
|
self.finished.set()
|
|
|
|
# Special thread class to represent the main thread
|
|
# This is garbage collected through an exit handler
|
|
|
|
class _MainThread(Thread):
|
|
|
|
def __init__(self):
|
|
Thread.__init__(self, name="MainThread")
|
|
self._started.set()
|
|
_active_limbo_lock.acquire()
|
|
_active[_get_ident()] = self
|
|
_active_limbo_lock.release()
|
|
|
|
def _set_daemon(self):
|
|
return False
|
|
|
|
def _exitfunc(self):
|
|
self._stop()
|
|
t = _pickSomeNonDaemonThread()
|
|
if t:
|
|
if __debug__:
|
|
self._note("%s: waiting for other threads", self)
|
|
while t:
|
|
t.join()
|
|
t = _pickSomeNonDaemonThread()
|
|
if __debug__:
|
|
self._note("%s: exiting", self)
|
|
self._delete()
|
|
|
|
def _pickSomeNonDaemonThread():
|
|
for t in enumerate():
|
|
if not t.isDaemon() and t.isAlive():
|
|
return t
|
|
return None
|
|
|
|
|
|
# Dummy thread class to represent threads not started here.
|
|
# These aren't garbage collected when they die, nor can they be waited for.
|
|
# If they invoke anything in threading.py that calls currentThread(), they
|
|
# leave an entry in the _active dict forever after.
|
|
# Their purpose is to return *something* from currentThread().
|
|
# They are marked as daemon threads so we won't wait for them
|
|
# when we exit (conform previous semantics).
|
|
|
|
class _DummyThread(Thread):
|
|
|
|
def __init__(self):
|
|
Thread.__init__(self, name=_newname("Dummy-%d"))
|
|
|
|
# Thread.__block consumes an OS-level locking primitive, which
|
|
# can never be used by a _DummyThread. Since a _DummyThread
|
|
# instance is immortal, that's bad, so release this resource.
|
|
del self._block
|
|
|
|
|
|
self._started.set()
|
|
_active_limbo_lock.acquire()
|
|
_active[_get_ident()] = self
|
|
_active_limbo_lock.release()
|
|
|
|
def _set_daemon(self):
|
|
return True
|
|
|
|
def join(self, timeout=None):
|
|
assert False, "cannot join a dummy thread"
|
|
|
|
|
|
# Global API functions
|
|
|
|
def currentThread():
|
|
try:
|
|
return _active[_get_ident()]
|
|
except KeyError:
|
|
##print "currentThread(): no current thread for", _get_ident()
|
|
return _DummyThread()
|
|
|
|
def activeCount():
|
|
_active_limbo_lock.acquire()
|
|
count = len(_active) + len(_limbo)
|
|
_active_limbo_lock.release()
|
|
return count
|
|
|
|
def enumerate():
|
|
_active_limbo_lock.acquire()
|
|
active = list(_active.values()) + list(_limbo.values())
|
|
_active_limbo_lock.release()
|
|
return active
|
|
|
|
from thread import stack_size
|
|
|
|
# Create the main thread object,
|
|
# and make it available for the interpreter
|
|
# (Py_Main) as threading._shutdown.
|
|
|
|
_shutdown = _MainThread()._exitfunc
|
|
|
|
# get thread-local implementation, either from the thread
|
|
# module, or from the python fallback
|
|
|
|
try:
|
|
from thread import _local as local
|
|
except ImportError:
|
|
from _threading_local import local
|
|
|
|
|
|
# Self-test code
|
|
|
|
def _test():
|
|
|
|
class BoundedQueue(_Verbose):
|
|
|
|
def __init__(self, limit):
|
|
_Verbose.__init__(self)
|
|
self.mon = RLock()
|
|
self.rc = Condition(self.mon)
|
|
self.wc = Condition(self.mon)
|
|
self.limit = limit
|
|
self.queue = deque()
|
|
|
|
def put(self, item):
|
|
self.mon.acquire()
|
|
while len(self.queue) >= self.limit:
|
|
self._note("put(%s): queue full", item)
|
|
self.wc.wait()
|
|
self.queue.append(item)
|
|
self._note("put(%s): appended, length now %d",
|
|
item, len(self.queue))
|
|
self.rc.notify()
|
|
self.mon.release()
|
|
|
|
def get(self):
|
|
self.mon.acquire()
|
|
while not self.queue:
|
|
self._note("get(): queue empty")
|
|
self.rc.wait()
|
|
item = self.queue.popleft()
|
|
self._note("get(): got %s, %d left", item, len(self.queue))
|
|
self.wc.notify()
|
|
self.mon.release()
|
|
return item
|
|
|
|
class ProducerThread(Thread):
|
|
|
|
def __init__(self, queue, quota):
|
|
Thread.__init__(self, name="Producer")
|
|
self.queue = queue
|
|
self.quota = quota
|
|
|
|
def run(self):
|
|
from random import random
|
|
counter = 0
|
|
while counter < self.quota:
|
|
counter = counter + 1
|
|
self.queue.put("%s.%d" % (self.getName(), counter))
|
|
_sleep(random() * 0.00001)
|
|
|
|
|
|
class ConsumerThread(Thread):
|
|
|
|
def __init__(self, queue, count):
|
|
Thread.__init__(self, name="Consumer")
|
|
self.queue = queue
|
|
self.count = count
|
|
|
|
def run(self):
|
|
while self.count > 0:
|
|
item = self.queue.get()
|
|
print(item)
|
|
self.count = self.count - 1
|
|
|
|
NP = 3
|
|
QL = 4
|
|
NI = 5
|
|
|
|
Q = BoundedQueue(QL)
|
|
P = []
|
|
for i in range(NP):
|
|
t = ProducerThread(Q, NI)
|
|
t.setName("Producer-%d" % (i+1))
|
|
P.append(t)
|
|
C = ConsumerThread(Q, NI*NP)
|
|
for t in P:
|
|
t.start()
|
|
_sleep(0.000001)
|
|
C.start()
|
|
for t in P:
|
|
t.join()
|
|
C.join()
|
|
|
|
if __name__ == '__main__':
|
|
_test()
|