mirror of
https://github.com/python/cpython
synced 2024-07-20 17:55:24 +00:00
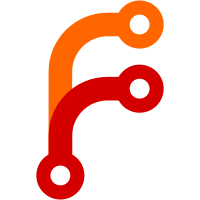
The invalid assignment rules are very delicate since the parser can easily raise an invalid assignment when a keyword argument is provided. As they are very deep into the grammar tree, is very difficult to specify in which contexts these rules can be used and in which don't. For that, we need to use a different version of the rule that doesn't do error checking in those situations where we don't want the rule to raise (keyword arguments and generator expressions). We also need to check if we are in left-recursive rule, as those can try to eagerly advance the parser even if the parse will fail at the end of the expression. Failing to do this allows the parser to start parsing a call as a tuple and incorrectly identify a keyword argument as an invalid assignment, before it realizes that it was not a tuple after all.
1021 lines
47 KiB
Plaintext
1021 lines
47 KiB
Plaintext
# PEG grammar for Python
|
|
|
|
@trailer '''
|
|
void *
|
|
_PyPegen_parse(Parser *p)
|
|
{
|
|
// Initialize keywords
|
|
p->keywords = reserved_keywords;
|
|
p->n_keyword_lists = n_keyword_lists;
|
|
p->soft_keywords = soft_keywords;
|
|
|
|
// Run parser
|
|
void *result = NULL;
|
|
if (p->start_rule == Py_file_input) {
|
|
result = file_rule(p);
|
|
} else if (p->start_rule == Py_single_input) {
|
|
result = interactive_rule(p);
|
|
} else if (p->start_rule == Py_eval_input) {
|
|
result = eval_rule(p);
|
|
} else if (p->start_rule == Py_func_type_input) {
|
|
result = func_type_rule(p);
|
|
} else if (p->start_rule == Py_fstring_input) {
|
|
result = fstring_rule(p);
|
|
}
|
|
|
|
return result;
|
|
}
|
|
|
|
// The end
|
|
'''
|
|
file[mod_ty]: a=[statements] ENDMARKER { _PyPegen_make_module(p, a) }
|
|
interactive[mod_ty]: a=statement_newline { _PyAST_Interactive(a, p->arena) }
|
|
eval[mod_ty]: a=expressions NEWLINE* ENDMARKER { _PyAST_Expression(a, p->arena) }
|
|
func_type[mod_ty]: '(' a=[type_expressions] ')' '->' b=expression NEWLINE* ENDMARKER { _PyAST_FunctionType(a, b, p->arena) }
|
|
fstring[expr_ty]: star_expressions
|
|
|
|
# type_expressions allow */** but ignore them
|
|
type_expressions[asdl_expr_seq*]:
|
|
| a=','.expression+ ',' '*' b=expression ',' '**' c=expression {
|
|
(asdl_expr_seq*)_PyPegen_seq_append_to_end(
|
|
p,
|
|
CHECK(asdl_seq*, _PyPegen_seq_append_to_end(p, a, b)),
|
|
c) }
|
|
| a=','.expression+ ',' '*' b=expression { (asdl_expr_seq*)_PyPegen_seq_append_to_end(p, a, b) }
|
|
| a=','.expression+ ',' '**' b=expression { (asdl_expr_seq*)_PyPegen_seq_append_to_end(p, a, b) }
|
|
| '*' a=expression ',' '**' b=expression {
|
|
(asdl_expr_seq*)_PyPegen_seq_append_to_end(
|
|
p,
|
|
CHECK(asdl_seq*, _PyPegen_singleton_seq(p, a)),
|
|
b) }
|
|
| '*' a=expression { (asdl_expr_seq*)_PyPegen_singleton_seq(p, a) }
|
|
| '**' a=expression { (asdl_expr_seq*)_PyPegen_singleton_seq(p, a) }
|
|
| a[asdl_expr_seq*]=','.expression+ {a}
|
|
|
|
statements[asdl_stmt_seq*]: a=statement+ { (asdl_stmt_seq*)_PyPegen_seq_flatten(p, a) }
|
|
statement[asdl_stmt_seq*]: a=compound_stmt { (asdl_stmt_seq*)_PyPegen_singleton_seq(p, a) } | a[asdl_stmt_seq*]=simple_stmts { a }
|
|
statement_newline[asdl_stmt_seq*]:
|
|
| a=compound_stmt NEWLINE { (asdl_stmt_seq*)_PyPegen_singleton_seq(p, a) }
|
|
| simple_stmts
|
|
| NEWLINE { (asdl_stmt_seq*)_PyPegen_singleton_seq(p, CHECK(stmt_ty, _PyAST_Pass(EXTRA))) }
|
|
| ENDMARKER { _PyPegen_interactive_exit(p) }
|
|
simple_stmts[asdl_stmt_seq*]:
|
|
| a=simple_stmt !';' NEWLINE { (asdl_stmt_seq*)_PyPegen_singleton_seq(p, a) } # Not needed, there for speedup
|
|
| a[asdl_stmt_seq*]=';'.simple_stmt+ [';'] NEWLINE { a }
|
|
# NOTE: assignment MUST precede expression, else parsing a simple assignment
|
|
# will throw a SyntaxError.
|
|
simple_stmt[stmt_ty] (memo):
|
|
| assignment
|
|
| e=star_expressions { _PyAST_Expr(e, EXTRA) }
|
|
| &'return' return_stmt
|
|
| &('import' | 'from') import_stmt
|
|
| &'raise' raise_stmt
|
|
| 'pass' { _PyAST_Pass(EXTRA) }
|
|
| &'del' del_stmt
|
|
| &'yield' yield_stmt
|
|
| &'assert' assert_stmt
|
|
| 'break' { _PyAST_Break(EXTRA) }
|
|
| 'continue' { _PyAST_Continue(EXTRA) }
|
|
| &'global' global_stmt
|
|
| &'nonlocal' nonlocal_stmt
|
|
compound_stmt[stmt_ty]:
|
|
| &('def' | '@' | ASYNC) function_def
|
|
| &'if' if_stmt
|
|
| &('class' | '@') class_def
|
|
| &('with' | ASYNC) with_stmt
|
|
| &('for' | ASYNC) for_stmt
|
|
| &'try' try_stmt
|
|
| &'while' while_stmt
|
|
| match_stmt
|
|
|
|
# NOTE: annotated_rhs may start with 'yield'; yield_expr must start with 'yield'
|
|
assignment[stmt_ty]:
|
|
| a=NAME ':' b=expression c=['=' d=annotated_rhs { d }] {
|
|
CHECK_VERSION(
|
|
stmt_ty,
|
|
6,
|
|
"Variable annotation syntax is",
|
|
_PyAST_AnnAssign(CHECK(expr_ty, _PyPegen_set_expr_context(p, a, Store)), b, c, 1, EXTRA)
|
|
) }
|
|
| a=('(' b=single_target ')' { b }
|
|
| single_subscript_attribute_target) ':' b=expression c=['=' d=annotated_rhs { d }] {
|
|
CHECK_VERSION(stmt_ty, 6, "Variable annotations syntax is", _PyAST_AnnAssign(a, b, c, 0, EXTRA)) }
|
|
| a[asdl_expr_seq*]=(z=star_targets '=' { z })+ b=(yield_expr | star_expressions) !'=' tc=[TYPE_COMMENT] {
|
|
_PyAST_Assign(a, b, NEW_TYPE_COMMENT(p, tc), EXTRA) }
|
|
| a=single_target b=augassign ~ c=(yield_expr | star_expressions) {
|
|
_PyAST_AugAssign(a, b->kind, c, EXTRA) }
|
|
| invalid_assignment
|
|
|
|
augassign[AugOperator*]:
|
|
| '+=' { _PyPegen_augoperator(p, Add) }
|
|
| '-=' { _PyPegen_augoperator(p, Sub) }
|
|
| '*=' { _PyPegen_augoperator(p, Mult) }
|
|
| '@=' { CHECK_VERSION(AugOperator*, 5, "The '@' operator is", _PyPegen_augoperator(p, MatMult)) }
|
|
| '/=' { _PyPegen_augoperator(p, Div) }
|
|
| '%=' { _PyPegen_augoperator(p, Mod) }
|
|
| '&=' { _PyPegen_augoperator(p, BitAnd) }
|
|
| '|=' { _PyPegen_augoperator(p, BitOr) }
|
|
| '^=' { _PyPegen_augoperator(p, BitXor) }
|
|
| '<<=' { _PyPegen_augoperator(p, LShift) }
|
|
| '>>=' { _PyPegen_augoperator(p, RShift) }
|
|
| '**=' { _PyPegen_augoperator(p, Pow) }
|
|
| '//=' { _PyPegen_augoperator(p, FloorDiv) }
|
|
|
|
global_stmt[stmt_ty]: 'global' a[asdl_expr_seq*]=','.NAME+ {
|
|
_PyAST_Global(CHECK(asdl_identifier_seq*, _PyPegen_map_names_to_ids(p, a)), EXTRA) }
|
|
nonlocal_stmt[stmt_ty]: 'nonlocal' a[asdl_expr_seq*]=','.NAME+ {
|
|
_PyAST_Nonlocal(CHECK(asdl_identifier_seq*, _PyPegen_map_names_to_ids(p, a)), EXTRA) }
|
|
|
|
yield_stmt[stmt_ty]: y=yield_expr { _PyAST_Expr(y, EXTRA) }
|
|
|
|
assert_stmt[stmt_ty]: 'assert' a=expression b=[',' z=expression { z }] { _PyAST_Assert(a, b, EXTRA) }
|
|
|
|
del_stmt[stmt_ty]:
|
|
| 'del' a=del_targets &(';' | NEWLINE) { _PyAST_Delete(a, EXTRA) }
|
|
| invalid_del_stmt
|
|
|
|
import_stmt[stmt_ty]: import_name | import_from
|
|
import_name[stmt_ty]: 'import' a=dotted_as_names { _PyAST_Import(a, EXTRA) }
|
|
# note below: the ('.' | '...') is necessary because '...' is tokenized as ELLIPSIS
|
|
import_from[stmt_ty]:
|
|
| 'from' a=('.' | '...')* b=dotted_name 'import' c=import_from_targets {
|
|
_PyAST_ImportFrom(b->v.Name.id, c, _PyPegen_seq_count_dots(a), EXTRA) }
|
|
| 'from' a=('.' | '...')+ 'import' b=import_from_targets {
|
|
_PyAST_ImportFrom(NULL, b, _PyPegen_seq_count_dots(a), EXTRA) }
|
|
import_from_targets[asdl_alias_seq*]:
|
|
| '(' a=import_from_as_names [','] ')' { a }
|
|
| import_from_as_names !','
|
|
| '*' { (asdl_alias_seq*)_PyPegen_singleton_seq(p, CHECK(alias_ty, _PyPegen_alias_for_star(p, EXTRA))) }
|
|
| invalid_import_from_targets
|
|
import_from_as_names[asdl_alias_seq*]:
|
|
| a[asdl_alias_seq*]=','.import_from_as_name+ { a }
|
|
import_from_as_name[alias_ty]:
|
|
| a=NAME b=['as' z=NAME { z }] { _PyAST_alias(a->v.Name.id,
|
|
(b) ? ((expr_ty) b)->v.Name.id : NULL,
|
|
EXTRA) }
|
|
dotted_as_names[asdl_alias_seq*]:
|
|
| a[asdl_alias_seq*]=','.dotted_as_name+ { a }
|
|
dotted_as_name[alias_ty]:
|
|
| a=dotted_name b=['as' z=NAME { z }] { _PyAST_alias(a->v.Name.id,
|
|
(b) ? ((expr_ty) b)->v.Name.id : NULL,
|
|
EXTRA) }
|
|
dotted_name[expr_ty]:
|
|
| a=dotted_name '.' b=NAME { _PyPegen_join_names_with_dot(p, a, b) }
|
|
| NAME
|
|
|
|
if_stmt[stmt_ty]:
|
|
| invalid_if_stmt
|
|
| 'if' a=named_expression ':' b=block c=elif_stmt {
|
|
_PyAST_If(a, b, CHECK(asdl_stmt_seq*, _PyPegen_singleton_seq(p, c)), EXTRA) }
|
|
| 'if' a=named_expression ':' b=block c=[else_block] { _PyAST_If(a, b, c, EXTRA) }
|
|
elif_stmt[stmt_ty]:
|
|
| invalid_elif_stmt
|
|
| 'elif' a=named_expression ':' b=block c=elif_stmt {
|
|
_PyAST_If(a, b, CHECK(asdl_stmt_seq*, _PyPegen_singleton_seq(p, c)), EXTRA) }
|
|
| 'elif' a=named_expression ':' b=block c=[else_block] { _PyAST_If(a, b, c, EXTRA) }
|
|
else_block[asdl_stmt_seq*]:
|
|
| invalid_else_stmt
|
|
| 'else' &&':' b=block { b }
|
|
|
|
while_stmt[stmt_ty]:
|
|
| invalid_while_stmt
|
|
| 'while' a=named_expression ':' b=block c=[else_block] { _PyAST_While(a, b, c, EXTRA) }
|
|
|
|
for_stmt[stmt_ty]:
|
|
| invalid_for_stmt
|
|
| 'for' t=star_targets 'in' ~ ex=star_expressions &&':' tc=[TYPE_COMMENT] b=block el=[else_block] {
|
|
_PyAST_For(t, ex, b, el, NEW_TYPE_COMMENT(p, tc), EXTRA) }
|
|
| ASYNC 'for' t=star_targets 'in' ~ ex=star_expressions &&':' tc=[TYPE_COMMENT] b=block el=[else_block] {
|
|
CHECK_VERSION(stmt_ty, 5, "Async for loops are", _PyAST_AsyncFor(t, ex, b, el, NEW_TYPE_COMMENT(p, tc), EXTRA)) }
|
|
| invalid_for_target
|
|
|
|
with_stmt[stmt_ty]:
|
|
| invalid_with_stmt_indent
|
|
| 'with' '(' a[asdl_withitem_seq*]=','.with_item+ ','? ')' ':' b=block {
|
|
_PyAST_With(a, b, NULL, EXTRA) }
|
|
| 'with' a[asdl_withitem_seq*]=','.with_item+ ':' tc=[TYPE_COMMENT] b=block {
|
|
_PyAST_With(a, b, NEW_TYPE_COMMENT(p, tc), EXTRA) }
|
|
| ASYNC 'with' '(' a[asdl_withitem_seq*]=','.with_item+ ','? ')' ':' b=block {
|
|
CHECK_VERSION(stmt_ty, 5, "Async with statements are", _PyAST_AsyncWith(a, b, NULL, EXTRA)) }
|
|
| ASYNC 'with' a[asdl_withitem_seq*]=','.with_item+ ':' tc=[TYPE_COMMENT] b=block {
|
|
CHECK_VERSION(stmt_ty, 5, "Async with statements are", _PyAST_AsyncWith(a, b, NEW_TYPE_COMMENT(p, tc), EXTRA)) }
|
|
| invalid_with_stmt
|
|
|
|
with_item[withitem_ty]:
|
|
| e=expression 'as' t=star_target &(',' | ')' | ':') { _PyAST_withitem(e, t, p->arena) }
|
|
| invalid_with_item
|
|
| e=expression { _PyAST_withitem(e, NULL, p->arena) }
|
|
|
|
try_stmt[stmt_ty]:
|
|
| invalid_try_stmt
|
|
| 'try' &&':' b=block f=finally_block { _PyAST_Try(b, NULL, NULL, f, EXTRA) }
|
|
| 'try' &&':' b=block ex[asdl_excepthandler_seq*]=except_block+ el=[else_block] f=[finally_block] { _PyAST_Try(b, ex, el, f, EXTRA) }
|
|
except_block[excepthandler_ty]:
|
|
| invalid_except_stmt_indent
|
|
| 'except' e=expression t=['as' z=NAME { z }] ':' b=block {
|
|
_PyAST_ExceptHandler(e, (t) ? ((expr_ty) t)->v.Name.id : NULL, b, EXTRA) }
|
|
| 'except' ':' b=block { _PyAST_ExceptHandler(NULL, NULL, b, EXTRA) }
|
|
| invalid_except_stmt
|
|
finally_block[asdl_stmt_seq*]:
|
|
| invalid_finally_stmt
|
|
| 'finally' &&':' a=block { a }
|
|
|
|
match_stmt[stmt_ty]:
|
|
| "match" subject=subject_expr ':' NEWLINE INDENT cases[asdl_match_case_seq*]=case_block+ DEDENT {
|
|
CHECK_VERSION(stmt_ty, 10, "Pattern matching is", _PyAST_Match(subject, cases, EXTRA)) }
|
|
| invalid_match_stmt
|
|
subject_expr[expr_ty]:
|
|
| value=star_named_expression ',' values=star_named_expressions? {
|
|
_PyAST_Tuple(CHECK(asdl_expr_seq*, _PyPegen_seq_insert_in_front(p, value, values)), Load, EXTRA) }
|
|
| named_expression
|
|
case_block[match_case_ty]:
|
|
| invalid_case_block
|
|
| "case" pattern=patterns guard=guard? ':' body=block {
|
|
_PyAST_match_case(pattern, guard, body, p->arena) }
|
|
guard[expr_ty]: 'if' guard=named_expression { guard }
|
|
|
|
patterns[pattern_ty]:
|
|
| patterns[asdl_pattern_seq*]=open_sequence_pattern {
|
|
_PyAST_MatchSequence(patterns, EXTRA) }
|
|
| pattern
|
|
pattern[pattern_ty]:
|
|
| as_pattern
|
|
| or_pattern
|
|
as_pattern[pattern_ty]:
|
|
| pattern=or_pattern 'as' target=pattern_capture_target {
|
|
_PyAST_MatchAs(pattern, target->v.Name.id, EXTRA) }
|
|
or_pattern[pattern_ty]:
|
|
| patterns[asdl_pattern_seq*]='|'.closed_pattern+ {
|
|
asdl_seq_LEN(patterns) == 1 ? asdl_seq_GET(patterns, 0) : _PyAST_MatchOr(patterns, EXTRA) }
|
|
closed_pattern[pattern_ty]:
|
|
| literal_pattern
|
|
| capture_pattern
|
|
| wildcard_pattern
|
|
| value_pattern
|
|
| group_pattern
|
|
| sequence_pattern
|
|
| mapping_pattern
|
|
| class_pattern
|
|
|
|
# Literal patterns are used for equality and identity constraints
|
|
literal_pattern[pattern_ty]:
|
|
| value=signed_number !('+' | '-') { _PyAST_MatchValue(value, EXTRA) }
|
|
| value=complex_number { _PyAST_MatchValue(value, EXTRA) }
|
|
| value=strings { _PyAST_MatchValue(value, EXTRA) }
|
|
| 'None' { _PyAST_MatchSingleton(Py_None, EXTRA) }
|
|
| 'True' { _PyAST_MatchSingleton(Py_True, EXTRA) }
|
|
| 'False' { _PyAST_MatchSingleton(Py_False, EXTRA) }
|
|
|
|
# Literal expressions are used to restrict permitted mapping pattern keys
|
|
literal_expr[expr_ty]:
|
|
| signed_number !('+' | '-')
|
|
| complex_number
|
|
| strings
|
|
| 'None' { _PyAST_Constant(Py_None, NULL, EXTRA) }
|
|
| 'True' { _PyAST_Constant(Py_True, NULL, EXTRA) }
|
|
| 'False' { _PyAST_Constant(Py_False, NULL, EXTRA) }
|
|
|
|
complex_number[expr_ty]:
|
|
| real=signed_real_number '+' imag=imaginary_number {
|
|
_PyAST_BinOp(real, Add, imag, EXTRA) }
|
|
| real=signed_real_number '-' imag=imaginary_number {
|
|
_PyAST_BinOp(real, Sub, imag, EXTRA) }
|
|
|
|
signed_number[expr_ty]:
|
|
| NUMBER
|
|
| '-' number=NUMBER { _PyAST_UnaryOp(USub, number, EXTRA) }
|
|
|
|
signed_real_number[expr_ty]:
|
|
| real_number
|
|
| '-' real=real_number { _PyAST_UnaryOp(USub, real, EXTRA) }
|
|
|
|
real_number[expr_ty]:
|
|
| real=NUMBER { _PyPegen_ensure_real(p, real) }
|
|
|
|
imaginary_number[expr_ty]:
|
|
| imag=NUMBER { _PyPegen_ensure_imaginary(p, imag) }
|
|
|
|
capture_pattern[pattern_ty]:
|
|
| target=pattern_capture_target { _PyAST_MatchAs(NULL, target->v.Name.id, EXTRA) }
|
|
|
|
pattern_capture_target[expr_ty]:
|
|
| !"_" name=NAME !('.' | '(' | '=') {
|
|
_PyPegen_set_expr_context(p, name, Store) }
|
|
|
|
wildcard_pattern[pattern_ty]:
|
|
| "_" { _PyAST_MatchAs(NULL, NULL, EXTRA) }
|
|
|
|
value_pattern[pattern_ty]:
|
|
| attr=attr !('.' | '(' | '=') { _PyAST_MatchValue(attr, EXTRA) }
|
|
attr[expr_ty]:
|
|
| value=name_or_attr '.' attr=NAME {
|
|
_PyAST_Attribute(value, attr->v.Name.id, Load, EXTRA) }
|
|
name_or_attr[expr_ty]:
|
|
| attr
|
|
| NAME
|
|
|
|
group_pattern[pattern_ty]:
|
|
| '(' pattern=pattern ')' { pattern }
|
|
|
|
sequence_pattern[pattern_ty]:
|
|
| '[' patterns=maybe_sequence_pattern? ']' { _PyAST_MatchSequence(patterns, EXTRA) }
|
|
| '(' patterns=open_sequence_pattern? ')' { _PyAST_MatchSequence(patterns, EXTRA) }
|
|
open_sequence_pattern[asdl_seq*]:
|
|
| pattern=maybe_star_pattern ',' patterns=maybe_sequence_pattern? {
|
|
_PyPegen_seq_insert_in_front(p, pattern, patterns) }
|
|
maybe_sequence_pattern[asdl_seq*]:
|
|
| patterns=','.maybe_star_pattern+ ','? { patterns }
|
|
maybe_star_pattern[pattern_ty]:
|
|
| star_pattern
|
|
| pattern
|
|
star_pattern[pattern_ty]:
|
|
| '*' target=pattern_capture_target {
|
|
_PyAST_MatchStar(target->v.Name.id, EXTRA) }
|
|
| '*' wildcard_pattern {
|
|
_PyAST_MatchStar(NULL, EXTRA) }
|
|
|
|
mapping_pattern[pattern_ty]:
|
|
| '{' '}' {
|
|
_PyAST_MatchMapping(NULL, NULL, NULL, EXTRA) }
|
|
| '{' rest=double_star_pattern ','? '}' {
|
|
_PyAST_MatchMapping(NULL, NULL, rest->v.Name.id, EXTRA) }
|
|
| '{' items=items_pattern ',' rest=double_star_pattern ','? '}' {
|
|
_PyAST_MatchMapping(
|
|
CHECK(asdl_expr_seq*, _PyPegen_get_pattern_keys(p, items)),
|
|
CHECK(asdl_pattern_seq*, _PyPegen_get_patterns(p, items)),
|
|
rest->v.Name.id,
|
|
EXTRA) }
|
|
| '{' items=items_pattern ','? '}' {
|
|
_PyAST_MatchMapping(
|
|
CHECK(asdl_expr_seq*, _PyPegen_get_pattern_keys(p, items)),
|
|
CHECK(asdl_pattern_seq*, _PyPegen_get_patterns(p, items)),
|
|
NULL,
|
|
EXTRA) }
|
|
items_pattern[asdl_seq*]:
|
|
| ','.key_value_pattern+
|
|
key_value_pattern[KeyPatternPair*]:
|
|
| key=(literal_expr | attr) ':' pattern=pattern {
|
|
_PyPegen_key_pattern_pair(p, key, pattern) }
|
|
double_star_pattern[expr_ty]:
|
|
| '**' target=pattern_capture_target { target }
|
|
|
|
class_pattern[pattern_ty]:
|
|
| cls=name_or_attr '(' ')' {
|
|
_PyAST_MatchClass(cls, NULL, NULL, NULL, EXTRA) }
|
|
| cls=name_or_attr '(' patterns=positional_patterns ','? ')' {
|
|
_PyAST_MatchClass(cls, patterns, NULL, NULL, EXTRA) }
|
|
| cls=name_or_attr '(' keywords=keyword_patterns ','? ')' {
|
|
_PyAST_MatchClass(
|
|
cls, NULL,
|
|
CHECK(asdl_identifier_seq*, _PyPegen_map_names_to_ids(p,
|
|
CHECK(asdl_expr_seq*, _PyPegen_get_pattern_keys(p, keywords)))),
|
|
CHECK(asdl_pattern_seq*, _PyPegen_get_patterns(p, keywords)),
|
|
EXTRA) }
|
|
| cls=name_or_attr '(' patterns=positional_patterns ',' keywords=keyword_patterns ','? ')' {
|
|
_PyAST_MatchClass(
|
|
cls,
|
|
patterns,
|
|
CHECK(asdl_identifier_seq*, _PyPegen_map_names_to_ids(p,
|
|
CHECK(asdl_expr_seq*, _PyPegen_get_pattern_keys(p, keywords)))),
|
|
CHECK(asdl_pattern_seq*, _PyPegen_get_patterns(p, keywords)),
|
|
EXTRA) }
|
|
positional_patterns[asdl_pattern_seq*]:
|
|
| args[asdl_pattern_seq*]=','.pattern+ { args }
|
|
keyword_patterns[asdl_seq*]:
|
|
| ','.keyword_pattern+
|
|
keyword_pattern[KeyPatternPair*]:
|
|
| arg=NAME '=' value=pattern { _PyPegen_key_pattern_pair(p, arg, value) }
|
|
|
|
return_stmt[stmt_ty]:
|
|
| 'return' a=[star_expressions] { _PyAST_Return(a, EXTRA) }
|
|
|
|
raise_stmt[stmt_ty]:
|
|
| 'raise' a=expression b=['from' z=expression { z }] { _PyAST_Raise(a, b, EXTRA) }
|
|
| 'raise' { _PyAST_Raise(NULL, NULL, EXTRA) }
|
|
|
|
function_def[stmt_ty]:
|
|
| d=decorators f=function_def_raw { _PyPegen_function_def_decorators(p, d, f) }
|
|
| function_def_raw
|
|
|
|
function_def_raw[stmt_ty]:
|
|
| invalid_def_raw
|
|
| 'def' n=NAME '(' params=[params] ')' a=['->' z=expression { z }] &&':' tc=[func_type_comment] b=block {
|
|
_PyAST_FunctionDef(n->v.Name.id,
|
|
(params) ? params : CHECK(arguments_ty, _PyPegen_empty_arguments(p)),
|
|
b, NULL, a, NEW_TYPE_COMMENT(p, tc), EXTRA) }
|
|
| ASYNC 'def' n=NAME '(' params=[params] ')' a=['->' z=expression { z }] &&':' tc=[func_type_comment] b=block {
|
|
CHECK_VERSION(
|
|
stmt_ty,
|
|
5,
|
|
"Async functions are",
|
|
_PyAST_AsyncFunctionDef(n->v.Name.id,
|
|
(params) ? params : CHECK(arguments_ty, _PyPegen_empty_arguments(p)),
|
|
b, NULL, a, NEW_TYPE_COMMENT(p, tc), EXTRA)
|
|
) }
|
|
func_type_comment[Token*]:
|
|
| NEWLINE t=TYPE_COMMENT &(NEWLINE INDENT) { t } # Must be followed by indented block
|
|
| invalid_double_type_comments
|
|
| TYPE_COMMENT
|
|
|
|
params[arguments_ty]:
|
|
| invalid_parameters
|
|
| parameters
|
|
|
|
parameters[arguments_ty]:
|
|
| a=slash_no_default b[asdl_arg_seq*]=param_no_default* c=param_with_default* d=[star_etc] {
|
|
_PyPegen_make_arguments(p, a, NULL, b, c, d) }
|
|
| a=slash_with_default b=param_with_default* c=[star_etc] {
|
|
_PyPegen_make_arguments(p, NULL, a, NULL, b, c) }
|
|
| a[asdl_arg_seq*]=param_no_default+ b=param_with_default* c=[star_etc] {
|
|
_PyPegen_make_arguments(p, NULL, NULL, a, b, c) }
|
|
| a=param_with_default+ b=[star_etc] { _PyPegen_make_arguments(p, NULL, NULL, NULL, a, b)}
|
|
| a=star_etc { _PyPegen_make_arguments(p, NULL, NULL, NULL, NULL, a) }
|
|
|
|
# Some duplication here because we can't write (',' | &')'),
|
|
# which is because we don't support empty alternatives (yet).
|
|
#
|
|
slash_no_default[asdl_arg_seq*]:
|
|
| a[asdl_arg_seq*]=param_no_default+ '/' ',' { a }
|
|
| a[asdl_arg_seq*]=param_no_default+ '/' &')' { a }
|
|
slash_with_default[SlashWithDefault*]:
|
|
| a=param_no_default* b=param_with_default+ '/' ',' { _PyPegen_slash_with_default(p, (asdl_arg_seq *)a, b) }
|
|
| a=param_no_default* b=param_with_default+ '/' &')' { _PyPegen_slash_with_default(p, (asdl_arg_seq *)a, b) }
|
|
|
|
star_etc[StarEtc*]:
|
|
| '*' a=param_no_default b=param_maybe_default* c=[kwds] {
|
|
_PyPegen_star_etc(p, a, b, c) }
|
|
| '*' ',' b=param_maybe_default+ c=[kwds] {
|
|
_PyPegen_star_etc(p, NULL, b, c) }
|
|
| a=kwds { _PyPegen_star_etc(p, NULL, NULL, a) }
|
|
| invalid_star_etc
|
|
|
|
kwds[arg_ty]: '**' a=param_no_default { a }
|
|
|
|
# One parameter. This *includes* a following comma and type comment.
|
|
#
|
|
# There are three styles:
|
|
# - No default
|
|
# - With default
|
|
# - Maybe with default
|
|
#
|
|
# There are two alternative forms of each, to deal with type comments:
|
|
# - Ends in a comma followed by an optional type comment
|
|
# - No comma, optional type comment, must be followed by close paren
|
|
# The latter form is for a final parameter without trailing comma.
|
|
#
|
|
param_no_default[arg_ty]:
|
|
| a=param ',' tc=TYPE_COMMENT? { _PyPegen_add_type_comment_to_arg(p, a, tc) }
|
|
| a=param tc=TYPE_COMMENT? &')' { _PyPegen_add_type_comment_to_arg(p, a, tc) }
|
|
param_with_default[NameDefaultPair*]:
|
|
| a=param c=default ',' tc=TYPE_COMMENT? { _PyPegen_name_default_pair(p, a, c, tc) }
|
|
| a=param c=default tc=TYPE_COMMENT? &')' { _PyPegen_name_default_pair(p, a, c, tc) }
|
|
param_maybe_default[NameDefaultPair*]:
|
|
| a=param c=default? ',' tc=TYPE_COMMENT? { _PyPegen_name_default_pair(p, a, c, tc) }
|
|
| a=param c=default? tc=TYPE_COMMENT? &')' { _PyPegen_name_default_pair(p, a, c, tc) }
|
|
param[arg_ty]: a=NAME b=annotation? { _PyAST_arg(a->v.Name.id, b, NULL, EXTRA) }
|
|
|
|
annotation[expr_ty]: ':' a=expression { a }
|
|
default[expr_ty]: '=' a=expression { a }
|
|
|
|
decorators[asdl_expr_seq*]: a[asdl_expr_seq*]=('@' f=named_expression NEWLINE { f })+ { a }
|
|
|
|
class_def[stmt_ty]:
|
|
| a=decorators b=class_def_raw { _PyPegen_class_def_decorators(p, a, b) }
|
|
| class_def_raw
|
|
class_def_raw[stmt_ty]:
|
|
| invalid_class_def_raw
|
|
| 'class' a=NAME b=['(' z=[arguments] ')' { z }] &&':' c=block {
|
|
_PyAST_ClassDef(a->v.Name.id,
|
|
(b) ? ((expr_ty) b)->v.Call.args : NULL,
|
|
(b) ? ((expr_ty) b)->v.Call.keywords : NULL,
|
|
c, NULL, EXTRA) }
|
|
|
|
block[asdl_stmt_seq*] (memo):
|
|
| NEWLINE INDENT a=statements DEDENT { a }
|
|
| simple_stmts
|
|
| invalid_block
|
|
|
|
star_expressions[expr_ty]:
|
|
| a=star_expression b=(',' c=star_expression { c })+ [','] {
|
|
_PyAST_Tuple(CHECK(asdl_expr_seq*, _PyPegen_seq_insert_in_front(p, a, b)), Load, EXTRA) }
|
|
| a=star_expression ',' { _PyAST_Tuple(CHECK(asdl_expr_seq*, _PyPegen_singleton_seq(p, a)), Load, EXTRA) }
|
|
| star_expression
|
|
star_expression[expr_ty] (memo):
|
|
| '*' a=bitwise_or { _PyAST_Starred(a, Load, EXTRA) }
|
|
| expression
|
|
|
|
star_named_expressions[asdl_expr_seq*]: a[asdl_expr_seq*]=','.star_named_expression+ [','] { a }
|
|
star_named_expression[expr_ty]:
|
|
| '*' a=bitwise_or { _PyAST_Starred(a, Load, EXTRA) }
|
|
| named_expression
|
|
|
|
|
|
assigment_expression[expr_ty]:
|
|
| a=NAME ':=' ~ b=expression { _PyAST_NamedExpr(CHECK(expr_ty, _PyPegen_set_expr_context(p, a, Store)), b, EXTRA) }
|
|
|
|
named_expression[expr_ty]:
|
|
| assigment_expression
|
|
| invalid_named_expression
|
|
| expression !':='
|
|
|
|
annotated_rhs[expr_ty]: yield_expr | star_expressions
|
|
|
|
expressions[expr_ty]:
|
|
| a=expression b=(',' c=expression { c })+ [','] {
|
|
_PyAST_Tuple(CHECK(asdl_expr_seq*, _PyPegen_seq_insert_in_front(p, a, b)), Load, EXTRA) }
|
|
| a=expression ',' { _PyAST_Tuple(CHECK(asdl_expr_seq*, _PyPegen_singleton_seq(p, a)), Load, EXTRA) }
|
|
| expression
|
|
expression[expr_ty] (memo):
|
|
| invalid_expression
|
|
| a=disjunction 'if' b=disjunction 'else' c=expression { _PyAST_IfExp(b, a, c, EXTRA) }
|
|
| disjunction
|
|
| lambdef
|
|
|
|
lambdef[expr_ty]:
|
|
| 'lambda' a=[lambda_params] ':' b=expression {
|
|
_PyAST_Lambda((a) ? a : CHECK(arguments_ty, _PyPegen_empty_arguments(p)), b, EXTRA) }
|
|
|
|
lambda_params[arguments_ty]:
|
|
| invalid_lambda_parameters
|
|
| lambda_parameters
|
|
|
|
# lambda_parameters etc. duplicates parameters but without annotations
|
|
# or type comments, and if there's no comma after a parameter, we expect
|
|
# a colon, not a close parenthesis. (For more, see parameters above.)
|
|
#
|
|
lambda_parameters[arguments_ty]:
|
|
| a=lambda_slash_no_default b[asdl_arg_seq*]=lambda_param_no_default* c=lambda_param_with_default* d=[lambda_star_etc] {
|
|
_PyPegen_make_arguments(p, a, NULL, b, c, d) }
|
|
| a=lambda_slash_with_default b=lambda_param_with_default* c=[lambda_star_etc] {
|
|
_PyPegen_make_arguments(p, NULL, a, NULL, b, c) }
|
|
| a[asdl_arg_seq*]=lambda_param_no_default+ b=lambda_param_with_default* c=[lambda_star_etc] {
|
|
_PyPegen_make_arguments(p, NULL, NULL, a, b, c) }
|
|
| a=lambda_param_with_default+ b=[lambda_star_etc] { _PyPegen_make_arguments(p, NULL, NULL, NULL, a, b)}
|
|
| a=lambda_star_etc { _PyPegen_make_arguments(p, NULL, NULL, NULL, NULL, a) }
|
|
|
|
lambda_slash_no_default[asdl_arg_seq*]:
|
|
| a[asdl_arg_seq*]=lambda_param_no_default+ '/' ',' { a }
|
|
| a[asdl_arg_seq*]=lambda_param_no_default+ '/' &':' { a }
|
|
lambda_slash_with_default[SlashWithDefault*]:
|
|
| a=lambda_param_no_default* b=lambda_param_with_default+ '/' ',' { _PyPegen_slash_with_default(p, (asdl_arg_seq *)a, b) }
|
|
| a=lambda_param_no_default* b=lambda_param_with_default+ '/' &':' { _PyPegen_slash_with_default(p, (asdl_arg_seq *)a, b) }
|
|
|
|
lambda_star_etc[StarEtc*]:
|
|
| '*' a=lambda_param_no_default b=lambda_param_maybe_default* c=[lambda_kwds] {
|
|
_PyPegen_star_etc(p, a, b, c) }
|
|
| '*' ',' b=lambda_param_maybe_default+ c=[lambda_kwds] {
|
|
_PyPegen_star_etc(p, NULL, b, c) }
|
|
| a=lambda_kwds { _PyPegen_star_etc(p, NULL, NULL, a) }
|
|
| invalid_lambda_star_etc
|
|
|
|
lambda_kwds[arg_ty]: '**' a=lambda_param_no_default { a }
|
|
|
|
lambda_param_no_default[arg_ty]:
|
|
| a=lambda_param ',' { a }
|
|
| a=lambda_param &':' { a }
|
|
lambda_param_with_default[NameDefaultPair*]:
|
|
| a=lambda_param c=default ',' { _PyPegen_name_default_pair(p, a, c, NULL) }
|
|
| a=lambda_param c=default &':' { _PyPegen_name_default_pair(p, a, c, NULL) }
|
|
lambda_param_maybe_default[NameDefaultPair*]:
|
|
| a=lambda_param c=default? ',' { _PyPegen_name_default_pair(p, a, c, NULL) }
|
|
| a=lambda_param c=default? &':' { _PyPegen_name_default_pair(p, a, c, NULL) }
|
|
lambda_param[arg_ty]: a=NAME { _PyAST_arg(a->v.Name.id, NULL, NULL, EXTRA) }
|
|
|
|
disjunction[expr_ty] (memo):
|
|
| a=conjunction b=('or' c=conjunction { c })+ { _PyAST_BoolOp(
|
|
Or,
|
|
CHECK(asdl_expr_seq*, _PyPegen_seq_insert_in_front(p, a, b)),
|
|
EXTRA) }
|
|
| conjunction
|
|
conjunction[expr_ty] (memo):
|
|
| a=inversion b=('and' c=inversion { c })+ { _PyAST_BoolOp(
|
|
And,
|
|
CHECK(asdl_expr_seq*, _PyPegen_seq_insert_in_front(p, a, b)),
|
|
EXTRA) }
|
|
| inversion
|
|
inversion[expr_ty] (memo):
|
|
| 'not' a=inversion { _PyAST_UnaryOp(Not, a, EXTRA) }
|
|
| comparison
|
|
comparison[expr_ty]:
|
|
| a=bitwise_or b=compare_op_bitwise_or_pair+ {
|
|
_PyAST_Compare(
|
|
a,
|
|
CHECK(asdl_int_seq*, _PyPegen_get_cmpops(p, b)),
|
|
CHECK(asdl_expr_seq*, _PyPegen_get_exprs(p, b)),
|
|
EXTRA) }
|
|
| bitwise_or
|
|
compare_op_bitwise_or_pair[CmpopExprPair*]:
|
|
| eq_bitwise_or
|
|
| noteq_bitwise_or
|
|
| lte_bitwise_or
|
|
| lt_bitwise_or
|
|
| gte_bitwise_or
|
|
| gt_bitwise_or
|
|
| notin_bitwise_or
|
|
| in_bitwise_or
|
|
| isnot_bitwise_or
|
|
| is_bitwise_or
|
|
eq_bitwise_or[CmpopExprPair*]: '==' a=bitwise_or { _PyPegen_cmpop_expr_pair(p, Eq, a) }
|
|
noteq_bitwise_or[CmpopExprPair*]:
|
|
| (tok='!=' { _PyPegen_check_barry_as_flufl(p, tok) ? NULL : tok}) a=bitwise_or {_PyPegen_cmpop_expr_pair(p, NotEq, a) }
|
|
lte_bitwise_or[CmpopExprPair*]: '<=' a=bitwise_or { _PyPegen_cmpop_expr_pair(p, LtE, a) }
|
|
lt_bitwise_or[CmpopExprPair*]: '<' a=bitwise_or { _PyPegen_cmpop_expr_pair(p, Lt, a) }
|
|
gte_bitwise_or[CmpopExprPair*]: '>=' a=bitwise_or { _PyPegen_cmpop_expr_pair(p, GtE, a) }
|
|
gt_bitwise_or[CmpopExprPair*]: '>' a=bitwise_or { _PyPegen_cmpop_expr_pair(p, Gt, a) }
|
|
notin_bitwise_or[CmpopExprPair*]: 'not' 'in' a=bitwise_or { _PyPegen_cmpop_expr_pair(p, NotIn, a) }
|
|
in_bitwise_or[CmpopExprPair*]: 'in' a=bitwise_or { _PyPegen_cmpop_expr_pair(p, In, a) }
|
|
isnot_bitwise_or[CmpopExprPair*]: 'is' 'not' a=bitwise_or { _PyPegen_cmpop_expr_pair(p, IsNot, a) }
|
|
is_bitwise_or[CmpopExprPair*]: 'is' a=bitwise_or { _PyPegen_cmpop_expr_pair(p, Is, a) }
|
|
|
|
bitwise_or[expr_ty]:
|
|
| a=bitwise_or '|' b=bitwise_xor { _PyAST_BinOp(a, BitOr, b, EXTRA) }
|
|
| bitwise_xor
|
|
bitwise_xor[expr_ty]:
|
|
| a=bitwise_xor '^' b=bitwise_and { _PyAST_BinOp(a, BitXor, b, EXTRA) }
|
|
| bitwise_and
|
|
bitwise_and[expr_ty]:
|
|
| a=bitwise_and '&' b=shift_expr { _PyAST_BinOp(a, BitAnd, b, EXTRA) }
|
|
| shift_expr
|
|
shift_expr[expr_ty]:
|
|
| a=shift_expr '<<' b=sum { _PyAST_BinOp(a, LShift, b, EXTRA) }
|
|
| a=shift_expr '>>' b=sum { _PyAST_BinOp(a, RShift, b, EXTRA) }
|
|
| sum
|
|
|
|
sum[expr_ty]:
|
|
| a=sum '+' b=term { _PyAST_BinOp(a, Add, b, EXTRA) }
|
|
| a=sum '-' b=term { _PyAST_BinOp(a, Sub, b, EXTRA) }
|
|
| term
|
|
term[expr_ty]:
|
|
| a=term '*' b=factor { _PyAST_BinOp(a, Mult, b, EXTRA) }
|
|
| a=term '/' b=factor { _PyAST_BinOp(a, Div, b, EXTRA) }
|
|
| a=term '//' b=factor { _PyAST_BinOp(a, FloorDiv, b, EXTRA) }
|
|
| a=term '%' b=factor { _PyAST_BinOp(a, Mod, b, EXTRA) }
|
|
| a=term '@' b=factor { CHECK_VERSION(expr_ty, 5, "The '@' operator is", _PyAST_BinOp(a, MatMult, b, EXTRA)) }
|
|
| factor
|
|
factor[expr_ty] (memo):
|
|
| '+' a=factor { _PyAST_UnaryOp(UAdd, a, EXTRA) }
|
|
| '-' a=factor { _PyAST_UnaryOp(USub, a, EXTRA) }
|
|
| '~' a=factor { _PyAST_UnaryOp(Invert, a, EXTRA) }
|
|
| power
|
|
power[expr_ty]:
|
|
| a=await_primary '**' b=factor { _PyAST_BinOp(a, Pow, b, EXTRA) }
|
|
| await_primary
|
|
await_primary[expr_ty] (memo):
|
|
| AWAIT a=primary { CHECK_VERSION(expr_ty, 5, "Await expressions are", _PyAST_Await(a, EXTRA)) }
|
|
| primary
|
|
primary[expr_ty]:
|
|
| invalid_primary # must be before 'primay genexp' because of invalid_genexp
|
|
| a=primary '.' b=NAME { _PyAST_Attribute(a, b->v.Name.id, Load, EXTRA) }
|
|
| a=primary b=genexp { _PyAST_Call(a, CHECK(asdl_expr_seq*, (asdl_expr_seq*)_PyPegen_singleton_seq(p, b)), NULL, EXTRA) }
|
|
| a=primary '(' b=[arguments] ')' {
|
|
_PyAST_Call(a,
|
|
(b) ? ((expr_ty) b)->v.Call.args : NULL,
|
|
(b) ? ((expr_ty) b)->v.Call.keywords : NULL,
|
|
EXTRA) }
|
|
| a=primary '[' b=slices ']' { _PyAST_Subscript(a, b, Load, EXTRA) }
|
|
| atom
|
|
|
|
slices[expr_ty]:
|
|
| a=slice !',' { a }
|
|
| a[asdl_expr_seq*]=','.slice+ [','] { _PyAST_Tuple(a, Load, EXTRA) }
|
|
slice[expr_ty]:
|
|
| a=[expression] ':' b=[expression] c=[':' d=[expression] { d }] { _PyAST_Slice(a, b, c, EXTRA) }
|
|
| a=named_expression { a }
|
|
atom[expr_ty]:
|
|
| NAME
|
|
| 'True' { _PyAST_Constant(Py_True, NULL, EXTRA) }
|
|
| 'False' { _PyAST_Constant(Py_False, NULL, EXTRA) }
|
|
| 'None' { _PyAST_Constant(Py_None, NULL, EXTRA) }
|
|
| &STRING strings
|
|
| NUMBER
|
|
| &'(' (tuple | group | genexp)
|
|
| &'[' (list | listcomp)
|
|
| &'{' (dict | set | dictcomp | setcomp)
|
|
| '...' { _PyAST_Constant(Py_Ellipsis, NULL, EXTRA) }
|
|
|
|
strings[expr_ty] (memo): a=STRING+ { _PyPegen_concatenate_strings(p, a) }
|
|
list[expr_ty]:
|
|
| '[' a=[star_named_expressions] ']' { _PyAST_List(a, Load, EXTRA) }
|
|
listcomp[expr_ty]:
|
|
| '[' a=named_expression b=for_if_clauses ']' { _PyAST_ListComp(a, b, EXTRA) }
|
|
| invalid_comprehension
|
|
tuple[expr_ty]:
|
|
| '(' a=[y=star_named_expression ',' z=[star_named_expressions] { _PyPegen_seq_insert_in_front(p, y, z) } ] ')' {
|
|
_PyAST_Tuple(a, Load, EXTRA) }
|
|
group[expr_ty]:
|
|
| '(' a=(yield_expr | named_expression) ')' { a }
|
|
| invalid_group
|
|
genexp[expr_ty]:
|
|
| '(' a=( assigment_expression | expression !':=') b=for_if_clauses ')' { _PyAST_GeneratorExp(a, b, EXTRA) }
|
|
| invalid_comprehension
|
|
set[expr_ty]: '{' a=star_named_expressions '}' { _PyAST_Set(a, EXTRA) }
|
|
setcomp[expr_ty]:
|
|
| '{' a=named_expression b=for_if_clauses '}' { _PyAST_SetComp(a, b, EXTRA) }
|
|
| invalid_comprehension
|
|
dict[expr_ty]:
|
|
| '{' a=[double_starred_kvpairs] '}' {
|
|
_PyAST_Dict(
|
|
CHECK(asdl_expr_seq*, _PyPegen_get_keys(p, a)),
|
|
CHECK(asdl_expr_seq*, _PyPegen_get_values(p, a)),
|
|
EXTRA) }
|
|
| '{' invalid_double_starred_kvpairs '}'
|
|
|
|
dictcomp[expr_ty]:
|
|
| '{' a=kvpair b=for_if_clauses '}' { _PyAST_DictComp(a->key, a->value, b, EXTRA) }
|
|
| invalid_dict_comprehension
|
|
double_starred_kvpairs[asdl_seq*]: a=','.double_starred_kvpair+ [','] { a }
|
|
double_starred_kvpair[KeyValuePair*]:
|
|
| '**' a=bitwise_or { _PyPegen_key_value_pair(p, NULL, a) }
|
|
| kvpair
|
|
kvpair[KeyValuePair*]: a=expression ':' b=expression { _PyPegen_key_value_pair(p, a, b) }
|
|
for_if_clauses[asdl_comprehension_seq*]:
|
|
| a[asdl_comprehension_seq*]=for_if_clause+ { a }
|
|
for_if_clause[comprehension_ty]:
|
|
| ASYNC 'for' a=star_targets 'in' ~ b=disjunction c[asdl_expr_seq*]=('if' z=disjunction { z })* {
|
|
CHECK_VERSION(comprehension_ty, 6, "Async comprehensions are", _PyAST_comprehension(a, b, c, 1, p->arena)) }
|
|
| 'for' a=star_targets 'in' ~ b=disjunction c[asdl_expr_seq*]=('if' z=disjunction { z })* {
|
|
_PyAST_comprehension(a, b, c, 0, p->arena) }
|
|
| invalid_for_target
|
|
|
|
yield_expr[expr_ty]:
|
|
| 'yield' 'from' a=expression { _PyAST_YieldFrom(a, EXTRA) }
|
|
| 'yield' a=[star_expressions] { _PyAST_Yield(a, EXTRA) }
|
|
|
|
arguments[expr_ty] (memo):
|
|
| a=args [','] &')' { a }
|
|
| invalid_arguments
|
|
args[expr_ty]:
|
|
| a[asdl_expr_seq*]=','.(starred_expression | ( assigment_expression | expression !':=') !'=')+ b=[',' k=kwargs {k}] {
|
|
_PyPegen_collect_call_seqs(p, a, b, EXTRA) }
|
|
| a=kwargs { _PyAST_Call(_PyPegen_dummy_name(p),
|
|
CHECK_NULL_ALLOWED(asdl_expr_seq*, _PyPegen_seq_extract_starred_exprs(p, a)),
|
|
CHECK_NULL_ALLOWED(asdl_keyword_seq*, _PyPegen_seq_delete_starred_exprs(p, a)),
|
|
EXTRA) }
|
|
|
|
kwargs[asdl_seq*]:
|
|
| a=','.kwarg_or_starred+ ',' b=','.kwarg_or_double_starred+ { _PyPegen_join_sequences(p, a, b) }
|
|
| ','.kwarg_or_starred+
|
|
| ','.kwarg_or_double_starred+
|
|
starred_expression[expr_ty]:
|
|
| '*' a=expression { _PyAST_Starred(a, Load, EXTRA) }
|
|
kwarg_or_starred[KeywordOrStarred*]:
|
|
| invalid_kwarg
|
|
| a=NAME '=' b=expression {
|
|
_PyPegen_keyword_or_starred(p, CHECK(keyword_ty, _PyAST_keyword(a->v.Name.id, b, EXTRA)), 1) }
|
|
| a=starred_expression { _PyPegen_keyword_or_starred(p, a, 0) }
|
|
kwarg_or_double_starred[KeywordOrStarred*]:
|
|
| invalid_kwarg
|
|
| a=NAME '=' b=expression {
|
|
_PyPegen_keyword_or_starred(p, CHECK(keyword_ty, _PyAST_keyword(a->v.Name.id, b, EXTRA)), 1) }
|
|
| '**' a=expression { _PyPegen_keyword_or_starred(p, CHECK(keyword_ty, _PyAST_keyword(NULL, a, EXTRA)), 1) }
|
|
|
|
# NOTE: star_targets may contain *bitwise_or, targets may not.
|
|
star_targets[expr_ty]:
|
|
| a=star_target !',' { a }
|
|
| a=star_target b=(',' c=star_target { c })* [','] {
|
|
_PyAST_Tuple(CHECK(asdl_expr_seq*, _PyPegen_seq_insert_in_front(p, a, b)), Store, EXTRA) }
|
|
star_targets_list_seq[asdl_expr_seq*]: a[asdl_expr_seq*]=','.star_target+ [','] { a }
|
|
star_targets_tuple_seq[asdl_expr_seq*]:
|
|
| a=star_target b=(',' c=star_target { c })+ [','] { (asdl_expr_seq*) _PyPegen_seq_insert_in_front(p, a, b) }
|
|
| a=star_target ',' { (asdl_expr_seq*) _PyPegen_singleton_seq(p, a) }
|
|
star_target[expr_ty] (memo):
|
|
| '*' a=(!'*' star_target) {
|
|
_PyAST_Starred(CHECK(expr_ty, _PyPegen_set_expr_context(p, a, Store)), Store, EXTRA) }
|
|
| target_with_star_atom
|
|
target_with_star_atom[expr_ty] (memo):
|
|
| a=t_primary '.' b=NAME !t_lookahead { _PyAST_Attribute(a, b->v.Name.id, Store, EXTRA) }
|
|
| a=t_primary '[' b=slices ']' !t_lookahead { _PyAST_Subscript(a, b, Store, EXTRA) }
|
|
| star_atom
|
|
star_atom[expr_ty]:
|
|
| a=NAME { _PyPegen_set_expr_context(p, a, Store) }
|
|
| '(' a=target_with_star_atom ')' { _PyPegen_set_expr_context(p, a, Store) }
|
|
| '(' a=[star_targets_tuple_seq] ')' { _PyAST_Tuple(a, Store, EXTRA) }
|
|
| '[' a=[star_targets_list_seq] ']' { _PyAST_List(a, Store, EXTRA) }
|
|
|
|
single_target[expr_ty]:
|
|
| single_subscript_attribute_target
|
|
| a=NAME { _PyPegen_set_expr_context(p, a, Store) }
|
|
| '(' a=single_target ')' { a }
|
|
single_subscript_attribute_target[expr_ty]:
|
|
| a=t_primary '.' b=NAME !t_lookahead { _PyAST_Attribute(a, b->v.Name.id, Store, EXTRA) }
|
|
| a=t_primary '[' b=slices ']' !t_lookahead { _PyAST_Subscript(a, b, Store, EXTRA) }
|
|
|
|
del_targets[asdl_expr_seq*]: a[asdl_expr_seq*]=','.del_target+ [','] { a }
|
|
del_target[expr_ty] (memo):
|
|
| a=t_primary '.' b=NAME !t_lookahead { _PyAST_Attribute(a, b->v.Name.id, Del, EXTRA) }
|
|
| a=t_primary '[' b=slices ']' !t_lookahead { _PyAST_Subscript(a, b, Del, EXTRA) }
|
|
| del_t_atom
|
|
del_t_atom[expr_ty]:
|
|
| a=NAME { _PyPegen_set_expr_context(p, a, Del) }
|
|
| '(' a=del_target ')' { _PyPegen_set_expr_context(p, a, Del) }
|
|
| '(' a=[del_targets] ')' { _PyAST_Tuple(a, Del, EXTRA) }
|
|
| '[' a=[del_targets] ']' { _PyAST_List(a, Del, EXTRA) }
|
|
|
|
targets[asdl_expr_seq*]: a[asdl_expr_seq*]=','.target+ [','] { a }
|
|
target[expr_ty] (memo):
|
|
| a=t_primary '.' b=NAME !t_lookahead { _PyAST_Attribute(a, b->v.Name.id, Store, EXTRA) }
|
|
| a=t_primary '[' b=slices ']' !t_lookahead { _PyAST_Subscript(a, b, Store, EXTRA) }
|
|
| t_atom
|
|
t_primary[expr_ty]:
|
|
| a=t_primary '.' b=NAME &t_lookahead { _PyAST_Attribute(a, b->v.Name.id, Load, EXTRA) }
|
|
| a=t_primary '[' b=slices ']' &t_lookahead { _PyAST_Subscript(a, b, Load, EXTRA) }
|
|
| a=t_primary b=genexp &t_lookahead {
|
|
_PyAST_Call(a, CHECK(asdl_expr_seq*, (asdl_expr_seq*)_PyPegen_singleton_seq(p, b)), NULL, EXTRA) }
|
|
| a=t_primary '(' b=[arguments] ')' &t_lookahead {
|
|
_PyAST_Call(a,
|
|
(b) ? ((expr_ty) b)->v.Call.args : NULL,
|
|
(b) ? ((expr_ty) b)->v.Call.keywords : NULL,
|
|
EXTRA) }
|
|
| a=atom &t_lookahead { a }
|
|
t_lookahead: '(' | '[' | '.'
|
|
t_atom[expr_ty]:
|
|
| a=NAME { _PyPegen_set_expr_context(p, a, Store) }
|
|
| '(' a=target ')' { _PyPegen_set_expr_context(p, a, Store) }
|
|
| '(' b=[targets] ')' { _PyAST_Tuple(b, Store, EXTRA) }
|
|
| '[' b=[targets] ']' { _PyAST_List(b, Store, EXTRA) }
|
|
|
|
|
|
# From here on, there are rules for invalid syntax with specialised error messages
|
|
invalid_arguments:
|
|
| a=args ',' '*' { RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "iterable argument unpacking follows keyword argument unpacking") }
|
|
| a=expression b=for_if_clauses ',' [args | expression for_if_clauses] {
|
|
RAISE_SYNTAX_ERROR_KNOWN_RANGE(a, PyPegen_last_item(b, comprehension_ty)->target, "Generator expression must be parenthesized") }
|
|
| a=NAME b='=' expression for_if_clauses {
|
|
RAISE_SYNTAX_ERROR_KNOWN_RANGE(a, b, "invalid syntax. Maybe you meant '==' or ':=' instead of '='?")}
|
|
| a=args for_if_clauses { _PyPegen_nonparen_genexp_in_call(p, a) }
|
|
| args ',' a=expression b=for_if_clauses {
|
|
RAISE_SYNTAX_ERROR_KNOWN_RANGE(a, asdl_seq_GET(b, b->size-1)->target, "Generator expression must be parenthesized") }
|
|
| a=args ',' args { _PyPegen_arguments_parsing_error(p, a) }
|
|
invalid_kwarg:
|
|
| a=NAME b='=' expression for_if_clauses {
|
|
RAISE_SYNTAX_ERROR_KNOWN_RANGE(a, b, "invalid syntax. Maybe you meant '==' or ':=' instead of '='?")}
|
|
| !(NAME '=') a=expression b='=' {
|
|
RAISE_SYNTAX_ERROR_KNOWN_RANGE(
|
|
a, b, "expression cannot contain assignment, perhaps you meant \"==\"?") }
|
|
|
|
expression_without_invalid[expr_ty]:
|
|
| a=disjunction 'if' b=disjunction 'else' c=expression { _PyAST_IfExp(b, a, c, EXTRA) }
|
|
| disjunction
|
|
| lambdef
|
|
invalid_expression:
|
|
# !(NAME STRING) is not matched so we don't show this error with some invalid string prefixes like: kf"dsfsdf"
|
|
# Soft keywords need to also be ignored because they can be parsed as NAME NAME
|
|
| !(NAME STRING | SOFT_KEYWORD) a=disjunction b=expression_without_invalid {
|
|
RAISE_SYNTAX_ERROR_KNOWN_RANGE(a, b, "invalid syntax. Perhaps you forgot a comma?") }
|
|
|
|
invalid_named_expression:
|
|
| a=expression ':=' expression {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(
|
|
a, "cannot use assignment expressions with %s", _PyPegen_get_expr_name(a)) }
|
|
| a=NAME '=' b=bitwise_or !('='|':=') {
|
|
p->in_raw_rule ? NULL : RAISE_SYNTAX_ERROR_KNOWN_RANGE(a, b, "invalid syntax. Maybe you meant '==' or ':=' instead of '='?") }
|
|
| !(list|tuple|genexp|'True'|'None'|'False') a=bitwise_or b='=' bitwise_or !('='|':=') {
|
|
p->in_raw_rule ? NULL : RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "cannot assign to %s here. Maybe you meant '==' instead of '='?",
|
|
_PyPegen_get_expr_name(a)) }
|
|
|
|
invalid_assignment:
|
|
| a=invalid_ann_assign_target ':' expression {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(
|
|
a,
|
|
"only single target (not %s) can be annotated",
|
|
_PyPegen_get_expr_name(a)
|
|
)}
|
|
| a=star_named_expression ',' star_named_expressions* ':' expression {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "only single target (not tuple) can be annotated") }
|
|
| a=expression ':' expression {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "illegal target for annotation") }
|
|
| (star_targets '=')* a=star_expressions '=' {
|
|
RAISE_SYNTAX_ERROR_INVALID_TARGET(STAR_TARGETS, a) }
|
|
| (star_targets '=')* a=yield_expr '=' { RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "assignment to yield expression not possible") }
|
|
| a=star_expressions augassign (yield_expr | star_expressions) {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(
|
|
a,
|
|
"'%s' is an illegal expression for augmented assignment",
|
|
_PyPegen_get_expr_name(a)
|
|
)}
|
|
invalid_ann_assign_target[expr_ty]:
|
|
| list
|
|
| tuple
|
|
| '(' a=invalid_ann_assign_target ')' { a }
|
|
invalid_del_stmt:
|
|
| 'del' a=star_expressions {
|
|
RAISE_SYNTAX_ERROR_INVALID_TARGET(DEL_TARGETS, a) }
|
|
invalid_block:
|
|
| NEWLINE !INDENT { RAISE_INDENTATION_ERROR("expected an indented block") }
|
|
invalid_primary:
|
|
| primary a='{' { RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "invalid syntax") }
|
|
invalid_comprehension:
|
|
| ('[' | '(' | '{') a=starred_expression for_if_clauses {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "iterable unpacking cannot be used in comprehension") }
|
|
| ('[' | '{') a=star_named_expression ',' b=star_named_expressions for_if_clauses {
|
|
RAISE_SYNTAX_ERROR_KNOWN_RANGE(a, PyPegen_last_item(b, expr_ty),
|
|
"did you forget parentheses around the comprehension target?") }
|
|
| ('[' | '{') a=star_named_expression b=',' for_if_clauses {
|
|
RAISE_SYNTAX_ERROR_KNOWN_RANGE(a, b, "did you forget parentheses around the comprehension target?") }
|
|
invalid_dict_comprehension:
|
|
| '{' a='**' bitwise_or for_if_clauses '}' {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "dict unpacking cannot be used in dict comprehension") }
|
|
invalid_parameters:
|
|
| param_no_default* invalid_parameters_helper a=param_no_default {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "non-default argument follows default argument") }
|
|
invalid_parameters_helper: # This is only there to avoid type errors
|
|
| a=slash_with_default { _PyPegen_singleton_seq(p, a) }
|
|
| param_with_default+
|
|
invalid_lambda_parameters:
|
|
| lambda_param_no_default* invalid_lambda_parameters_helper a=lambda_param_no_default {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "non-default argument follows default argument") }
|
|
invalid_lambda_parameters_helper:
|
|
| a=lambda_slash_with_default { _PyPegen_singleton_seq(p, a) }
|
|
| lambda_param_with_default+
|
|
invalid_star_etc:
|
|
| a='*' (')' | ',' (')' | '**')) { RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "named arguments must follow bare *") }
|
|
| '*' ',' TYPE_COMMENT { RAISE_SYNTAX_ERROR("bare * has associated type comment") }
|
|
invalid_lambda_star_etc:
|
|
| '*' (':' | ',' (':' | '**')) { RAISE_SYNTAX_ERROR("named arguments must follow bare *") }
|
|
invalid_double_type_comments:
|
|
| TYPE_COMMENT NEWLINE TYPE_COMMENT NEWLINE INDENT {
|
|
RAISE_SYNTAX_ERROR("Cannot have two type comments on def") }
|
|
invalid_with_item:
|
|
| expression 'as' a=expression &(',' | ')' | ':') {
|
|
RAISE_SYNTAX_ERROR_INVALID_TARGET(STAR_TARGETS, a) }
|
|
|
|
invalid_for_target:
|
|
| ASYNC? 'for' a=star_expressions {
|
|
RAISE_SYNTAX_ERROR_INVALID_TARGET(FOR_TARGETS, a) }
|
|
|
|
invalid_group:
|
|
| '(' a=starred_expression ')' {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "cannot use starred expression here") }
|
|
| '(' a='**' expression ')' {
|
|
RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "cannot use double starred expression here") }
|
|
invalid_import_from_targets:
|
|
| import_from_as_names ',' {
|
|
RAISE_SYNTAX_ERROR("trailing comma not allowed without surrounding parentheses") }
|
|
|
|
invalid_with_stmt:
|
|
| [ASYNC] 'with' ','.(expression ['as' star_target])+ &&':'
|
|
| [ASYNC] 'with' '(' ','.(expressions ['as' star_target])+ ','? ')' &&':'
|
|
invalid_with_stmt_indent:
|
|
| [ASYNC] a='with' ','.(expression ['as' star_target])+ ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'with' statement on line %d", a->lineno) }
|
|
| [ASYNC] a='with' '(' ','.(expressions ['as' star_target])+ ','? ')' ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'with' statement on line %d", a->lineno) }
|
|
|
|
invalid_try_stmt:
|
|
| a='try' ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'try' statement on line %d", a->lineno) }
|
|
invalid_except_stmt:
|
|
| 'except' a=expression ',' expressions ['as' NAME ] ':' {
|
|
RAISE_SYNTAX_ERROR_STARTING_FROM(a, "multiple exception types must be parenthesized") }
|
|
| a='except' expression ['as' NAME ] NEWLINE { RAISE_SYNTAX_ERROR("expected ':'") }
|
|
| a='except' NEWLINE { RAISE_SYNTAX_ERROR("expected ':'") }
|
|
invalid_finally_stmt:
|
|
| a='finally' ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'finally' statement on line %d", a->lineno) }
|
|
invalid_except_stmt_indent:
|
|
| a='except' expression ['as' NAME ] ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'except' statement on line %d", a->lineno) }
|
|
| a='except' ':' NEWLINE !INDENT { RAISE_SYNTAX_ERROR("expected an indented block after except statement on line %d", a->lineno) }
|
|
invalid_match_stmt:
|
|
| "match" subject_expr !':' { CHECK_VERSION(void*, 10, "Pattern matching is", RAISE_SYNTAX_ERROR("expected ':'") ) }
|
|
| a="match" subject=subject_expr ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'match' statement on line %d", a->lineno) }
|
|
invalid_case_block:
|
|
| "case" patterns guard? !':' { RAISE_SYNTAX_ERROR("expected ':'") }
|
|
| a="case" patterns guard? ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'case' statement on line %d", a->lineno) }
|
|
invalid_if_stmt:
|
|
| 'if' named_expression NEWLINE { RAISE_SYNTAX_ERROR("expected ':'") }
|
|
| a='if' a=named_expression ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'if' statement on line %d", a->lineno) }
|
|
invalid_elif_stmt:
|
|
| 'elif' named_expression NEWLINE { RAISE_SYNTAX_ERROR("expected ':'") }
|
|
| a='elif' named_expression ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'elif' statement on line %d", a->lineno) }
|
|
invalid_else_stmt:
|
|
| a='else' ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'else' statement on line %d", a->lineno) }
|
|
invalid_while_stmt:
|
|
| 'while' named_expression NEWLINE { RAISE_SYNTAX_ERROR("expected ':'") }
|
|
| a='while' named_expression ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'while' statement on line %d", a->lineno) }
|
|
invalid_for_stmt:
|
|
| [ASYNC] a='for' star_targets 'in' star_expressions ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after 'for' statement on line %d", a->lineno) }
|
|
invalid_def_raw:
|
|
| [ASYNC] a='def' NAME '(' [params] ')' ['->' expression] ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after function definition on line %d", a->lineno) }
|
|
invalid_class_def_raw:
|
|
| a='class' NAME ['('[arguments] ')'] ':' NEWLINE !INDENT {
|
|
RAISE_INDENTATION_ERROR("expected an indented block after class definition on line %d", a->lineno) }
|
|
|
|
invalid_double_starred_kvpairs:
|
|
| ','.double_starred_kvpair+ ',' invalid_kvpair
|
|
| expression ':' a='*' bitwise_or { RAISE_SYNTAX_ERROR_STARTING_FROM(a, "cannot use a starred expression in a dictionary value") }
|
|
| expression a=':' &('}'|',') { RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "expression expected after dictionary key and ':'") }
|
|
invalid_kvpair:
|
|
| a=expression !(':') {
|
|
RAISE_ERROR_KNOWN_LOCATION(p, PyExc_SyntaxError, a->lineno, a->end_col_offset - 1, a->end_lineno, -1, "':' expected after dictionary key") }
|
|
| expression ':' a='*' bitwise_or { RAISE_SYNTAX_ERROR_STARTING_FROM(a, "cannot use a starred expression in a dictionary value") }
|
|
| expression a=':' {RAISE_SYNTAX_ERROR_KNOWN_LOCATION(a, "expression expected after dictionary key and ':'") }
|