mirror of
https://github.com/python/cpython
synced 2024-11-02 11:04:01 +00:00
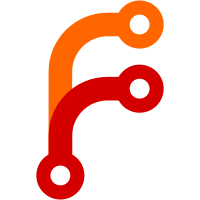
Update the vendored copy of libexpat to 2.4.1 (from 2.2.8) to get the fix for the CVE-2013-0340 "Billion Laughs" vulnerability. This copy is most used on Windows and macOS. Co-authored-by: Łukasz Langa <lukasz@langa.pl>
142 lines
4.6 KiB
C
142 lines
4.6 KiB
C
/*
|
|
__ __ _
|
|
___\ \/ /_ __ __ _| |_
|
|
/ _ \\ /| '_ \ / _` | __|
|
|
| __// \| |_) | (_| | |_
|
|
\___/_/\_\ .__/ \__,_|\__|
|
|
|_| XML parser
|
|
|
|
Copyright (c) 1997-2000 Thai Open Source Software Center Ltd
|
|
Copyright (c) 2000 Clark Cooper <coopercc@users.sourceforge.net>
|
|
Copyright (c) 2002 Karl Waclawek <karl@waclawek.net>
|
|
Copyright (c) 2002 Fred L. Drake, Jr. <fdrake@users.sourceforge.net>
|
|
Copyright (c) 2017 Sebastian Pipping <sebastian@pipping.org>
|
|
Licensed under the MIT license:
|
|
|
|
Permission is hereby granted, free of charge, to any person obtaining
|
|
a copy of this software and associated documentation files (the
|
|
"Software"), to deal in the Software without restriction, including
|
|
without limitation the rights to use, copy, modify, merge, publish,
|
|
distribute, sublicense, and/or sell copies of the Software, and to permit
|
|
persons to whom the Software is furnished to do so, subject to the
|
|
following conditions:
|
|
|
|
The above copyright notice and this permission notice shall be included
|
|
in all copies or substantial portions of the Software.
|
|
|
|
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN
|
|
NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
|
|
DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
|
|
OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
|
|
USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
*/
|
|
|
|
#ifndef XmlRole_INCLUDED
|
|
#define XmlRole_INCLUDED 1
|
|
|
|
#ifdef __VMS
|
|
/* 0 1 2 3 0 1 2 3
|
|
1234567890123456789012345678901 1234567890123456789012345678901 */
|
|
# define XmlPrologStateInitExternalEntity XmlPrologStateInitExternalEnt
|
|
#endif
|
|
|
|
#include "xmltok.h"
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
enum {
|
|
XML_ROLE_ERROR = -1,
|
|
XML_ROLE_NONE = 0,
|
|
XML_ROLE_XML_DECL,
|
|
XML_ROLE_INSTANCE_START,
|
|
XML_ROLE_DOCTYPE_NONE,
|
|
XML_ROLE_DOCTYPE_NAME,
|
|
XML_ROLE_DOCTYPE_SYSTEM_ID,
|
|
XML_ROLE_DOCTYPE_PUBLIC_ID,
|
|
XML_ROLE_DOCTYPE_INTERNAL_SUBSET,
|
|
XML_ROLE_DOCTYPE_CLOSE,
|
|
XML_ROLE_GENERAL_ENTITY_NAME,
|
|
XML_ROLE_PARAM_ENTITY_NAME,
|
|
XML_ROLE_ENTITY_NONE,
|
|
XML_ROLE_ENTITY_VALUE,
|
|
XML_ROLE_ENTITY_SYSTEM_ID,
|
|
XML_ROLE_ENTITY_PUBLIC_ID,
|
|
XML_ROLE_ENTITY_COMPLETE,
|
|
XML_ROLE_ENTITY_NOTATION_NAME,
|
|
XML_ROLE_NOTATION_NONE,
|
|
XML_ROLE_NOTATION_NAME,
|
|
XML_ROLE_NOTATION_SYSTEM_ID,
|
|
XML_ROLE_NOTATION_NO_SYSTEM_ID,
|
|
XML_ROLE_NOTATION_PUBLIC_ID,
|
|
XML_ROLE_ATTRIBUTE_NAME,
|
|
XML_ROLE_ATTRIBUTE_TYPE_CDATA,
|
|
XML_ROLE_ATTRIBUTE_TYPE_ID,
|
|
XML_ROLE_ATTRIBUTE_TYPE_IDREF,
|
|
XML_ROLE_ATTRIBUTE_TYPE_IDREFS,
|
|
XML_ROLE_ATTRIBUTE_TYPE_ENTITY,
|
|
XML_ROLE_ATTRIBUTE_TYPE_ENTITIES,
|
|
XML_ROLE_ATTRIBUTE_TYPE_NMTOKEN,
|
|
XML_ROLE_ATTRIBUTE_TYPE_NMTOKENS,
|
|
XML_ROLE_ATTRIBUTE_ENUM_VALUE,
|
|
XML_ROLE_ATTRIBUTE_NOTATION_VALUE,
|
|
XML_ROLE_ATTLIST_NONE,
|
|
XML_ROLE_ATTLIST_ELEMENT_NAME,
|
|
XML_ROLE_IMPLIED_ATTRIBUTE_VALUE,
|
|
XML_ROLE_REQUIRED_ATTRIBUTE_VALUE,
|
|
XML_ROLE_DEFAULT_ATTRIBUTE_VALUE,
|
|
XML_ROLE_FIXED_ATTRIBUTE_VALUE,
|
|
XML_ROLE_ELEMENT_NONE,
|
|
XML_ROLE_ELEMENT_NAME,
|
|
XML_ROLE_CONTENT_ANY,
|
|
XML_ROLE_CONTENT_EMPTY,
|
|
XML_ROLE_CONTENT_PCDATA,
|
|
XML_ROLE_GROUP_OPEN,
|
|
XML_ROLE_GROUP_CLOSE,
|
|
XML_ROLE_GROUP_CLOSE_REP,
|
|
XML_ROLE_GROUP_CLOSE_OPT,
|
|
XML_ROLE_GROUP_CLOSE_PLUS,
|
|
XML_ROLE_GROUP_CHOICE,
|
|
XML_ROLE_GROUP_SEQUENCE,
|
|
XML_ROLE_CONTENT_ELEMENT,
|
|
XML_ROLE_CONTENT_ELEMENT_REP,
|
|
XML_ROLE_CONTENT_ELEMENT_OPT,
|
|
XML_ROLE_CONTENT_ELEMENT_PLUS,
|
|
XML_ROLE_PI,
|
|
XML_ROLE_COMMENT,
|
|
#ifdef XML_DTD
|
|
XML_ROLE_TEXT_DECL,
|
|
XML_ROLE_IGNORE_SECT,
|
|
XML_ROLE_INNER_PARAM_ENTITY_REF,
|
|
#endif /* XML_DTD */
|
|
XML_ROLE_PARAM_ENTITY_REF
|
|
};
|
|
|
|
typedef struct prolog_state {
|
|
int(PTRCALL *handler)(struct prolog_state *state, int tok, const char *ptr,
|
|
const char *end, const ENCODING *enc);
|
|
unsigned level;
|
|
int role_none;
|
|
#ifdef XML_DTD
|
|
unsigned includeLevel;
|
|
int documentEntity;
|
|
int inEntityValue;
|
|
#endif /* XML_DTD */
|
|
} PROLOG_STATE;
|
|
|
|
void XmlPrologStateInit(PROLOG_STATE *);
|
|
#ifdef XML_DTD
|
|
void XmlPrologStateInitExternalEntity(PROLOG_STATE *);
|
|
#endif /* XML_DTD */
|
|
|
|
#define XmlTokenRole(state, tok, ptr, end, enc) \
|
|
(((state)->handler)(state, tok, ptr, end, enc))
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* not XmlRole_INCLUDED */
|