mirror of
https://github.com/python/cpython
synced 2024-11-02 09:48:08 +00:00
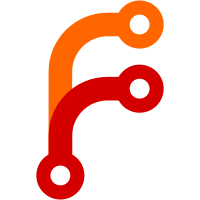
Replace <ctype.h> locale dependent functions with Python "pyctype.h" locale independent functions: * Replace isalpha() with Py_ISALPHA(). * Replace isdigit() with Py_ISDIGIT(). * Replace isxdigit() with Py_ISXDIGIT(). * Replace tolower() with Py_TOLOWER(). Leave Modules/_sre/sre.c unchanged, it uses locale dependent functions on purpose. Include explicitly <ctype.h> in _decimal.c to get isascii().
30 lines
777 B
C
30 lines
777 B
C
/* Cross platform case insensitive string compare functions
|
|
*/
|
|
|
|
#include "Python.h"
|
|
|
|
int
|
|
PyOS_mystrnicmp(const char *s1, const char *s2, Py_ssize_t size)
|
|
{
|
|
const unsigned char *p1, *p2;
|
|
if (size == 0)
|
|
return 0;
|
|
p1 = (const unsigned char *)s1;
|
|
p2 = (const unsigned char *)s2;
|
|
for (; (--size > 0) && *p1 && *p2 && (Py_TOLOWER(*p1) == Py_TOLOWER(*p2));
|
|
p1++, p2++) {
|
|
;
|
|
}
|
|
return Py_TOLOWER(*p1) - Py_TOLOWER(*p2);
|
|
}
|
|
|
|
int
|
|
PyOS_mystricmp(const char *s1, const char *s2)
|
|
{
|
|
const unsigned char *p1 = (const unsigned char *)s1;
|
|
const unsigned char *p2 = (const unsigned char *)s2;
|
|
for (; *p1 && *p2 && (Py_TOLOWER(*p1) == Py_TOLOWER(*p2)); p1++, p2++) {
|
|
;
|
|
}
|
|
return (Py_TOLOWER(*p1) - Py_TOLOWER(*p2));
|
|
}
|