mirror of
https://github.com/python/cpython
synced 2024-09-05 16:38:56 +00:00
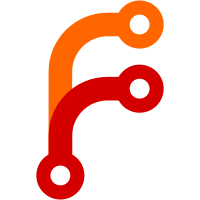
* Add tokenization of :=
- Add token to Include/token.h. Add token to documentation in Doc/library/token.rst.
- Run `./python Lib/token.py` to regenerate Lib/token.py.
- Update Parser/tokenizer.c: add case to handle `:=`.
* Add initial usage of := in grammar.
* Update Python.asdl to match the grammar updates. Regenerated Include/Python-ast.h and Python/Python-ast.c
* Update AST and compiler files in Python/ast.c and Python/compile.c. Basic functionality, this isn't scoped properly
* Regenerate Lib/symbol.py using `./python Lib/symbol.py`
* Tests - Fix failing tests in test_parser.py due to changes in token numbers for internal representation
* Tests - Add simple test for := token
* Tests - Add simple tests for named expressions using expr and suite
* Tests - Update number of levels for nested expressions to prevent stack overflow
* Update symbol table to handle NamedExpr
* Update Grammar to allow assignment expressions in if statements.
Regenerate Python/graminit.c accordingly using `make regen-grammar`
* Tests - Add additional tests for named expressions in RoundtripLegalSyntaxTestCase, based on examples and information directly from PEP 572
Note: failing tests are currently commented out (4 out of 24 tests currently fail)
* Tests - Add temporary syntax test failure tests in test_parser.py
Note: There is an outstanding TODO for this -- syntax tests need to be
moved to a different file (presumably test_syntax.py), but this is
covering what needs to be tested at the moment, and it's more convenient
to run a single test for the time being
* Add support for allowing assignment expressions as function argument annotations. Uncomment tests for these cases because they all pass now!
* Tests - Move existing syntax tests out of test_parser.py and into test_named_expressions.py. Refactor syntax tests to use unittest
* Add TargetScopeError exception to extend SyntaxError
Note: This simply creates the TargetScopeError exception, it is not yet
used anywhere
* Tests - Update tests per PEP 572
Continue refactoring test suite:
The named expression test suite now checks for any invalid cases that
throw exceptions (no longer limited to SyntaxErrors), assignment tests
to ensure that variables are properly assigned, and scope tests to
ensure that variable availability and values are correct
Note:
- There are still tests that are marked to skip, as they are not yet
implemented
- There are approximately 300 lines of the PEP that have not yet been
addressed, though these may be deferred
* Documentation - Small updates to XXX/todo comments
- Remove XXX from child description in ast.c
- Add comment with number of previously supported nested expressions for
3.7.X in test_parser.py
* Fix assert in seq_for_testlist()
* Cleanup - Denote "Not implemented -- No keyword args" on failing test case. Fix PEP8 error for blank lines at beginning of test classes in test_parser.py
* Tests - Wrap all file opens in `with...as` to ensure files are closed
* WIP: handle f(a := 1)
* Tests and Cleanup - No longer skips keyword arg test. Keyword arg test now uses a simpler test case and does not rely on an external file. Remove print statements from ast.c
* Tests - Refactor last remaining test case that relied on on external file to use a simpler test case without the dependency
* Tests - Add better description of remaning skipped tests. Add test checking scope when using assignment expression in a function argument
* Tests - Add test for nested comprehension, testing value and scope. Fix variable name in skipped comprehension scope test
* Handle restriction of LHS for named expressions - can only assign to LHS of type NAME. Specifically, restrict assignment to tuples
This adds an alternative set_context specifically for named expressions,
set_namedexpr_context. Thus, context is now set differently for standard
assignment versus assignment for named expressions in order to handle
restrictions.
* Tests - Update negative test case for assigning to lambda to match new error message. Add negative test case for assigning to tuple
* Tests - Reorder test cases to group invalid syntax cases and named assignment target errors
* Tests - Update test case for named expression in function argument - check that result and variable are set correctly
* Todo - Add todo for TargetScopeError based on Guido's comment (2b3acd37bd (r30472562)
)
* Tests - Add named expression tests for assignment operator in function arguments
Note: One of two tests are skipped, as function arguments are currently treating
an assignment expression inside of parenthesis as one child, which does
not properly catch the named expression, nor does it count arguments
properly
* Add NamedStore to expr_context. Regenerate related code with `make regen-ast`
* Add usage of NamedStore to ast_for_named_expr in ast.c. Update occurances of checking for Store to also handle NamedStore where appropriate
* Add ste_comprehension to _symtable_entry to track if the namespace is a comprehension. Initialize ste_comprehension to 0. Set set_comprehension to 1 in symtable_handle_comprehension
* s/symtable_add_def/symtable_add_def_helper. Add symtable_add_def to handle grabbing st->st_cur and passing it to symtable_add_def_helper. This now allows us to call the original code from symtable_add_def by instead calling symtable_add_def_helper with a different ste.
* Refactor symtable_record_directive to take lineno and col_offset as arguments instead of stmt_ty. This allows symtable_record_directive to be used for stmt_ty and expr_ty
* Handle elevating scope for named expressions in comprehensions.
* Handle error for usage of named expression inside a class block
* Tests - No longer skip scope tests. Add additional scope tests
* Cleanup - Update error message for named expression within a comprehension within a class. Update comments. Add assert for symtable_extend_namedexpr_scope to validate that we always find at least a ModuleScope if we don't find a Class or FunctionScope
* Cleanup - Add missing case for NamedStore in expr_context_name. Remove unused var in set_namedexpr_content
* Refactor - Consolidate set_context and set_namedexpr_context to reduce duplicated code. Special cases for named expressions are handled by checking if ctx is NamedStore
* Cleanup - Add additional use cases for ast_for_namedexpr in usage comment. Fix multiple blank lines in test_named_expressions
* Tests - Remove unnecessary test case. Renumber test case function names
* Remove TargetScopeError for now. Will add back if needed
* Cleanup - Small comment nit for consistency
* Handle positional argument check with named expression
* Add TargetScopeError exception definition. Add documentation for TargetScopeError in c-api docs. Throw TargetScopeError instead of SyntaxError when using a named expression in a comprehension within a class scope
* Increase stack size for parser by 200. This is a minimal change (approx. 5kb) and should not have an impact on any systems. Update parser test to allow 99 nested levels again
* Add TargetScopeError to exception_hierarchy.txt for test_baseexception.py_
* Tests - Major update for named expression tests, both in test_named_expressions and test_parser
- Add test for TargetScopeError
- Add tests for named expressions in comprehension scope and edge cases
- Add tests for named expressions in function arguments (declarations
and call sites)
- Reorganize tests to group them more logically
* Cleanup - Remove unnecessary comment
* Cleanup - Comment nitpicks
* Explicitly disallow assignment expressions to a name inside parentheses, e.g.: ((x) := 0)
- Add check for LHS types to detect a parenthesis then a name (see note)
- Add test for this scenario
- Update tests for changed error message for named assignment to a tuple
(also, see note)
Note: This caused issues with the previous error handling for named assignment
to a LHS that contained an expression, such as a tuple. Thus, the check
for the LHS of a named expression must be changed to be more specific if
we wish to maintain the previous error messages
* Cleanup - Wrap lines more strictly in test file
* Revert "Explicitly disallow assignment expressions to a name inside parentheses, e.g.: ((x) := 0)"
This reverts commit f1531400ca7d7a2d148830c8ac703f041740896d.
* Add NEWS.d entry
* Tests - Fix error in test_pickle.test_exceptions by adding TargetScopeError to list of exceptions
* Tests - Update error message tests to reflect improved messaging convention (s/can't/cannot)
* Remove cases that cannot be reached in compile.c. Small linting update.
* Update Grammar/Tokens to add COLONEQUAL. Regenerate all files
* Update TargetScopeError PRE_INIT and POST_INIT, as this was purposefully left out when fixing rebase conflicts
* Add NamedStore back and regenerate files
* Pass along line number and end col info for named expression
* Simplify News entry
* Fix compiler warning and explicity mark fallthrough
804 lines
41 KiB
Modula-2
804 lines
41 KiB
Modula-2
; This file specifies the import forwarding for python3.dll
|
|
; It is used when building python3dll.vcxproj
|
|
LIBRARY "python3"
|
|
EXPORTS
|
|
PyArg_Parse=python38.PyArg_Parse
|
|
PyArg_ParseTuple=python38.PyArg_ParseTuple
|
|
PyArg_ParseTupleAndKeywords=python38.PyArg_ParseTupleAndKeywords
|
|
PyArg_UnpackTuple=python38.PyArg_UnpackTuple
|
|
PyArg_VaParse=python38.PyArg_VaParse
|
|
PyArg_VaParseTupleAndKeywords=python38.PyArg_VaParseTupleAndKeywords
|
|
PyArg_ValidateKeywordArguments=python38.PyArg_ValidateKeywordArguments
|
|
PyBaseObject_Type=python38.PyBaseObject_Type DATA
|
|
PyBool_FromLong=python38.PyBool_FromLong
|
|
PyBool_Type=python38.PyBool_Type DATA
|
|
PyByteArrayIter_Type=python38.PyByteArrayIter_Type DATA
|
|
PyByteArray_AsString=python38.PyByteArray_AsString
|
|
PyByteArray_Concat=python38.PyByteArray_Concat
|
|
PyByteArray_FromObject=python38.PyByteArray_FromObject
|
|
PyByteArray_FromStringAndSize=python38.PyByteArray_FromStringAndSize
|
|
PyByteArray_Resize=python38.PyByteArray_Resize
|
|
PyByteArray_Size=python38.PyByteArray_Size
|
|
PyByteArray_Type=python38.PyByteArray_Type DATA
|
|
PyBytesIter_Type=python38.PyBytesIter_Type DATA
|
|
PyBytes_AsString=python38.PyBytes_AsString
|
|
PyBytes_AsStringAndSize=python38.PyBytes_AsStringAndSize
|
|
PyBytes_Concat=python38.PyBytes_Concat
|
|
PyBytes_ConcatAndDel=python38.PyBytes_ConcatAndDel
|
|
PyBytes_DecodeEscape=python38.PyBytes_DecodeEscape
|
|
PyBytes_FromFormat=python38.PyBytes_FromFormat
|
|
PyBytes_FromFormatV=python38.PyBytes_FromFormatV
|
|
PyBytes_FromObject=python38.PyBytes_FromObject
|
|
PyBytes_FromString=python38.PyBytes_FromString
|
|
PyBytes_FromStringAndSize=python38.PyBytes_FromStringAndSize
|
|
PyBytes_Repr=python38.PyBytes_Repr
|
|
PyBytes_Size=python38.PyBytes_Size
|
|
PyBytes_Type=python38.PyBytes_Type DATA
|
|
PyCFunction_Call=python38.PyCFunction_Call
|
|
PyCFunction_ClearFreeList=python38.PyCFunction_ClearFreeList
|
|
PyCFunction_GetFlags=python38.PyCFunction_GetFlags
|
|
PyCFunction_GetFunction=python38.PyCFunction_GetFunction
|
|
PyCFunction_GetSelf=python38.PyCFunction_GetSelf
|
|
PyCFunction_New=python38.PyCFunction_New
|
|
PyCFunction_NewEx=python38.PyCFunction_NewEx
|
|
PyCFunction_Type=python38.PyCFunction_Type DATA
|
|
PyCallIter_New=python38.PyCallIter_New
|
|
PyCallIter_Type=python38.PyCallIter_Type DATA
|
|
PyCallable_Check=python38.PyCallable_Check
|
|
PyCapsule_GetContext=python38.PyCapsule_GetContext
|
|
PyCapsule_GetDestructor=python38.PyCapsule_GetDestructor
|
|
PyCapsule_GetName=python38.PyCapsule_GetName
|
|
PyCapsule_GetPointer=python38.PyCapsule_GetPointer
|
|
PyCapsule_Import=python38.PyCapsule_Import
|
|
PyCapsule_IsValid=python38.PyCapsule_IsValid
|
|
PyCapsule_New=python38.PyCapsule_New
|
|
PyCapsule_SetContext=python38.PyCapsule_SetContext
|
|
PyCapsule_SetDestructor=python38.PyCapsule_SetDestructor
|
|
PyCapsule_SetName=python38.PyCapsule_SetName
|
|
PyCapsule_SetPointer=python38.PyCapsule_SetPointer
|
|
PyCapsule_Type=python38.PyCapsule_Type DATA
|
|
PyClassMethodDescr_Type=python38.PyClassMethodDescr_Type DATA
|
|
PyCodec_BackslashReplaceErrors=python38.PyCodec_BackslashReplaceErrors
|
|
PyCodec_Decode=python38.PyCodec_Decode
|
|
PyCodec_Decoder=python38.PyCodec_Decoder
|
|
PyCodec_Encode=python38.PyCodec_Encode
|
|
PyCodec_Encoder=python38.PyCodec_Encoder
|
|
PyCodec_IgnoreErrors=python38.PyCodec_IgnoreErrors
|
|
PyCodec_IncrementalDecoder=python38.PyCodec_IncrementalDecoder
|
|
PyCodec_IncrementalEncoder=python38.PyCodec_IncrementalEncoder
|
|
PyCodec_KnownEncoding=python38.PyCodec_KnownEncoding
|
|
PyCodec_LookupError=python38.PyCodec_LookupError
|
|
PyCodec_NameReplaceErrors=python38.PyCodec_NameReplaceErrors
|
|
PyCodec_Register=python38.PyCodec_Register
|
|
PyCodec_RegisterError=python38.PyCodec_RegisterError
|
|
PyCodec_ReplaceErrors=python38.PyCodec_ReplaceErrors
|
|
PyCodec_StreamReader=python38.PyCodec_StreamReader
|
|
PyCodec_StreamWriter=python38.PyCodec_StreamWriter
|
|
PyCodec_StrictErrors=python38.PyCodec_StrictErrors
|
|
PyCodec_XMLCharRefReplaceErrors=python38.PyCodec_XMLCharRefReplaceErrors
|
|
PyComplex_FromDoubles=python38.PyComplex_FromDoubles
|
|
PyComplex_ImagAsDouble=python38.PyComplex_ImagAsDouble
|
|
PyComplex_RealAsDouble=python38.PyComplex_RealAsDouble
|
|
PyComplex_Type=python38.PyComplex_Type DATA
|
|
PyDescr_NewClassMethod=python38.PyDescr_NewClassMethod
|
|
PyDescr_NewGetSet=python38.PyDescr_NewGetSet
|
|
PyDescr_NewMember=python38.PyDescr_NewMember
|
|
PyDescr_NewMethod=python38.PyDescr_NewMethod
|
|
PyDictItems_Type=python38.PyDictItems_Type DATA
|
|
PyDictIterItem_Type=python38.PyDictIterItem_Type DATA
|
|
PyDictIterKey_Type=python38.PyDictIterKey_Type DATA
|
|
PyDictIterValue_Type=python38.PyDictIterValue_Type DATA
|
|
PyDictKeys_Type=python38.PyDictKeys_Type DATA
|
|
PyDictProxy_New=python38.PyDictProxy_New
|
|
PyDictProxy_Type=python38.PyDictProxy_Type DATA
|
|
PyDictValues_Type=python38.PyDictValues_Type DATA
|
|
PyDict_Clear=python38.PyDict_Clear
|
|
PyDict_Contains=python38.PyDict_Contains
|
|
PyDict_Copy=python38.PyDict_Copy
|
|
PyDict_DelItem=python38.PyDict_DelItem
|
|
PyDict_DelItemString=python38.PyDict_DelItemString
|
|
PyDict_GetItem=python38.PyDict_GetItem
|
|
PyDict_GetItemString=python38.PyDict_GetItemString
|
|
PyDict_GetItemWithError=python38.PyDict_GetItemWithError
|
|
PyDict_Items=python38.PyDict_Items
|
|
PyDict_Keys=python38.PyDict_Keys
|
|
PyDict_Merge=python38.PyDict_Merge
|
|
PyDict_MergeFromSeq2=python38.PyDict_MergeFromSeq2
|
|
PyDict_New=python38.PyDict_New
|
|
PyDict_Next=python38.PyDict_Next
|
|
PyDict_SetItem=python38.PyDict_SetItem
|
|
PyDict_SetItemString=python38.PyDict_SetItemString
|
|
PyDict_Size=python38.PyDict_Size
|
|
PyDict_Type=python38.PyDict_Type DATA
|
|
PyDict_Update=python38.PyDict_Update
|
|
PyDict_Values=python38.PyDict_Values
|
|
PyEllipsis_Type=python38.PyEllipsis_Type DATA
|
|
PyEnum_Type=python38.PyEnum_Type DATA
|
|
PyErr_BadArgument=python38.PyErr_BadArgument
|
|
PyErr_BadInternalCall=python38.PyErr_BadInternalCall
|
|
PyErr_CheckSignals=python38.PyErr_CheckSignals
|
|
PyErr_Clear=python38.PyErr_Clear
|
|
PyErr_Display=python38.PyErr_Display
|
|
PyErr_ExceptionMatches=python38.PyErr_ExceptionMatches
|
|
PyErr_Fetch=python38.PyErr_Fetch
|
|
PyErr_Format=python38.PyErr_Format
|
|
PyErr_FormatV=python38.PyErr_FormatV
|
|
PyErr_GetExcInfo=python38.PyErr_GetExcInfo
|
|
PyErr_GivenExceptionMatches=python38.PyErr_GivenExceptionMatches
|
|
PyErr_NewException=python38.PyErr_NewException
|
|
PyErr_NewExceptionWithDoc=python38.PyErr_NewExceptionWithDoc
|
|
PyErr_NoMemory=python38.PyErr_NoMemory
|
|
PyErr_NormalizeException=python38.PyErr_NormalizeException
|
|
PyErr_Occurred=python38.PyErr_Occurred
|
|
PyErr_Print=python38.PyErr_Print
|
|
PyErr_PrintEx=python38.PyErr_PrintEx
|
|
PyErr_ProgramText=python38.PyErr_ProgramText
|
|
PyErr_ResourceWarning=python38.PyErr_ResourceWarning
|
|
PyErr_Restore=python38.PyErr_Restore
|
|
PyErr_SetExcFromWindowsErr=python38.PyErr_SetExcFromWindowsErr
|
|
PyErr_SetExcFromWindowsErrWithFilename=python38.PyErr_SetExcFromWindowsErrWithFilename
|
|
PyErr_SetExcFromWindowsErrWithFilenameObject=python38.PyErr_SetExcFromWindowsErrWithFilenameObject
|
|
PyErr_SetExcFromWindowsErrWithFilenameObjects=python38.PyErr_SetExcFromWindowsErrWithFilenameObjects
|
|
PyErr_SetExcInfo=python38.PyErr_SetExcInfo
|
|
PyErr_SetFromErrno=python38.PyErr_SetFromErrno
|
|
PyErr_SetFromErrnoWithFilename=python38.PyErr_SetFromErrnoWithFilename
|
|
PyErr_SetFromErrnoWithFilenameObject=python38.PyErr_SetFromErrnoWithFilenameObject
|
|
PyErr_SetFromErrnoWithFilenameObjects=python38.PyErr_SetFromErrnoWithFilenameObjects
|
|
PyErr_SetFromWindowsErr=python38.PyErr_SetFromWindowsErr
|
|
PyErr_SetFromWindowsErrWithFilename=python38.PyErr_SetFromWindowsErrWithFilename
|
|
PyErr_SetImportError=python38.PyErr_SetImportError
|
|
PyErr_SetImportErrorSubclass=python38.PyErr_SetImportErrorSubclass
|
|
PyErr_SetInterrupt=python38.PyErr_SetInterrupt
|
|
PyErr_SetNone=python38.PyErr_SetNone
|
|
PyErr_SetObject=python38.PyErr_SetObject
|
|
PyErr_SetString=python38.PyErr_SetString
|
|
PyErr_SyntaxLocation=python38.PyErr_SyntaxLocation
|
|
PyErr_SyntaxLocationEx=python38.PyErr_SyntaxLocationEx
|
|
PyErr_WarnEx=python38.PyErr_WarnEx
|
|
PyErr_WarnExplicit=python38.PyErr_WarnExplicit
|
|
PyErr_WarnFormat=python38.PyErr_WarnFormat
|
|
PyErr_WriteUnraisable=python38.PyErr_WriteUnraisable
|
|
PyEval_AcquireLock=python38.PyEval_AcquireLock
|
|
PyEval_AcquireThread=python38.PyEval_AcquireThread
|
|
PyEval_CallFunction=python38.PyEval_CallFunction
|
|
PyEval_CallMethod=python38.PyEval_CallMethod
|
|
PyEval_CallObjectWithKeywords=python38.PyEval_CallObjectWithKeywords
|
|
PyEval_EvalCode=python38.PyEval_EvalCode
|
|
PyEval_EvalCodeEx=python38.PyEval_EvalCodeEx
|
|
PyEval_EvalFrame=python38.PyEval_EvalFrame
|
|
PyEval_EvalFrameEx=python38.PyEval_EvalFrameEx
|
|
PyEval_GetBuiltins=python38.PyEval_GetBuiltins
|
|
PyEval_GetCallStats=python38.PyEval_GetCallStats
|
|
PyEval_GetFrame=python38.PyEval_GetFrame
|
|
PyEval_GetFuncDesc=python38.PyEval_GetFuncDesc
|
|
PyEval_GetFuncName=python38.PyEval_GetFuncName
|
|
PyEval_GetGlobals=python38.PyEval_GetGlobals
|
|
PyEval_GetLocals=python38.PyEval_GetLocals
|
|
PyEval_InitThreads=python38.PyEval_InitThreads
|
|
PyEval_ReInitThreads=python38.PyEval_ReInitThreads
|
|
PyEval_ReleaseLock=python38.PyEval_ReleaseLock
|
|
PyEval_ReleaseThread=python38.PyEval_ReleaseThread
|
|
PyEval_RestoreThread=python38.PyEval_RestoreThread
|
|
PyEval_SaveThread=python38.PyEval_SaveThread
|
|
PyEval_ThreadsInitialized=python38.PyEval_ThreadsInitialized
|
|
PyExc_ArithmeticError=python38.PyExc_ArithmeticError DATA
|
|
PyExc_AssertionError=python38.PyExc_AssertionError DATA
|
|
PyExc_AttributeError=python38.PyExc_AttributeError DATA
|
|
PyExc_BaseException=python38.PyExc_BaseException DATA
|
|
PyExc_BlockingIOError=python38.PyExc_BlockingIOError DATA
|
|
PyExc_BrokenPipeError=python38.PyExc_BrokenPipeError DATA
|
|
PyExc_BufferError=python38.PyExc_BufferError DATA
|
|
PyExc_BytesWarning=python38.PyExc_BytesWarning DATA
|
|
PyExc_ChildProcessError=python38.PyExc_ChildProcessError DATA
|
|
PyExc_ConnectionAbortedError=python38.PyExc_ConnectionAbortedError DATA
|
|
PyExc_ConnectionError=python38.PyExc_ConnectionError DATA
|
|
PyExc_ConnectionRefusedError=python38.PyExc_ConnectionRefusedError DATA
|
|
PyExc_ConnectionResetError=python38.PyExc_ConnectionResetError DATA
|
|
PyExc_DeprecationWarning=python38.PyExc_DeprecationWarning DATA
|
|
PyExc_EOFError=python38.PyExc_EOFError DATA
|
|
PyExc_EnvironmentError=python38.PyExc_EnvironmentError DATA
|
|
PyExc_Exception=python38.PyExc_Exception DATA
|
|
PyExc_FileExistsError=python38.PyExc_FileExistsError DATA
|
|
PyExc_FileNotFoundError=python38.PyExc_FileNotFoundError DATA
|
|
PyExc_FloatingPointError=python38.PyExc_FloatingPointError DATA
|
|
PyExc_FutureWarning=python38.PyExc_FutureWarning DATA
|
|
PyExc_GeneratorExit=python38.PyExc_GeneratorExit DATA
|
|
PyExc_IOError=python38.PyExc_IOError DATA
|
|
PyExc_ImportError=python38.PyExc_ImportError DATA
|
|
PyExc_ImportWarning=python38.PyExc_ImportWarning DATA
|
|
PyExc_IndentationError=python38.PyExc_IndentationError DATA
|
|
PyExc_IndexError=python38.PyExc_IndexError DATA
|
|
PyExc_InterruptedError=python38.PyExc_InterruptedError DATA
|
|
PyExc_IsADirectoryError=python38.PyExc_IsADirectoryError DATA
|
|
PyExc_KeyError=python38.PyExc_KeyError DATA
|
|
PyExc_KeyboardInterrupt=python38.PyExc_KeyboardInterrupt DATA
|
|
PyExc_LookupError=python38.PyExc_LookupError DATA
|
|
PyExc_MemoryError=python38.PyExc_MemoryError DATA
|
|
PyExc_ModuleNotFoundError=python38.PyExc_ModuleNotFoundError DATA
|
|
PyExc_NameError=python38.PyExc_NameError DATA
|
|
PyExc_NotADirectoryError=python38.PyExc_NotADirectoryError DATA
|
|
PyExc_NotImplementedError=python38.PyExc_NotImplementedError DATA
|
|
PyExc_OSError=python38.PyExc_OSError DATA
|
|
PyExc_OverflowError=python38.PyExc_OverflowError DATA
|
|
PyExc_PendingDeprecationWarning=python38.PyExc_PendingDeprecationWarning DATA
|
|
PyExc_PermissionError=python38.PyExc_PermissionError DATA
|
|
PyExc_ProcessLookupError=python38.PyExc_ProcessLookupError DATA
|
|
PyExc_RecursionError=python38.PyExc_RecursionError DATA
|
|
PyExc_ReferenceError=python38.PyExc_ReferenceError DATA
|
|
PyExc_ResourceWarning=python38.PyExc_ResourceWarning DATA
|
|
PyExc_RuntimeError=python38.PyExc_RuntimeError DATA
|
|
PyExc_RuntimeWarning=python38.PyExc_RuntimeWarning DATA
|
|
PyExc_StopAsyncIteration=python38.PyExc_StopAsyncIteration DATA
|
|
PyExc_StopIteration=python38.PyExc_StopIteration DATA
|
|
PyExc_SyntaxError=python38.PyExc_SyntaxError DATA
|
|
PyExc_SyntaxWarning=python38.PyExc_SyntaxWarning DATA
|
|
PyExc_SystemError=python38.PyExc_SystemError DATA
|
|
PyExc_SystemExit=python38.PyExc_SystemExit DATA
|
|
PyExc_TabError=python38.PyExc_TabError DATA
|
|
PyExc_TargetScopeError=python38.PyExc_TargetScopeError DATA
|
|
PyExc_TimeoutError=python38.PyExc_TimeoutError DATA
|
|
PyExc_TypeError=python38.PyExc_TypeError DATA
|
|
PyExc_UnboundLocalError=python38.PyExc_UnboundLocalError DATA
|
|
PyExc_UnicodeDecodeError=python38.PyExc_UnicodeDecodeError DATA
|
|
PyExc_UnicodeEncodeError=python38.PyExc_UnicodeEncodeError DATA
|
|
PyExc_UnicodeError=python38.PyExc_UnicodeError DATA
|
|
PyExc_UnicodeTranslateError=python38.PyExc_UnicodeTranslateError DATA
|
|
PyExc_UnicodeWarning=python38.PyExc_UnicodeWarning DATA
|
|
PyExc_UserWarning=python38.PyExc_UserWarning DATA
|
|
PyExc_ValueError=python38.PyExc_ValueError DATA
|
|
PyExc_Warning=python38.PyExc_Warning DATA
|
|
PyExc_WindowsError=python38.PyExc_WindowsError DATA
|
|
PyExc_ZeroDivisionError=python38.PyExc_ZeroDivisionError DATA
|
|
PyExceptionClass_Name=python38.PyExceptionClass_Name
|
|
PyException_GetCause=python38.PyException_GetCause
|
|
PyException_GetContext=python38.PyException_GetContext
|
|
PyException_GetTraceback=python38.PyException_GetTraceback
|
|
PyException_SetCause=python38.PyException_SetCause
|
|
PyException_SetContext=python38.PyException_SetContext
|
|
PyException_SetTraceback=python38.PyException_SetTraceback
|
|
PyFile_FromFd=python38.PyFile_FromFd
|
|
PyFile_GetLine=python38.PyFile_GetLine
|
|
PyFile_WriteObject=python38.PyFile_WriteObject
|
|
PyFile_WriteString=python38.PyFile_WriteString
|
|
PyFilter_Type=python38.PyFilter_Type DATA
|
|
PyFloat_AsDouble=python38.PyFloat_AsDouble
|
|
PyFloat_FromDouble=python38.PyFloat_FromDouble
|
|
PyFloat_FromString=python38.PyFloat_FromString
|
|
PyFloat_GetInfo=python38.PyFloat_GetInfo
|
|
PyFloat_GetMax=python38.PyFloat_GetMax
|
|
PyFloat_GetMin=python38.PyFloat_GetMin
|
|
PyFloat_Type=python38.PyFloat_Type DATA
|
|
PyFrozenSet_New=python38.PyFrozenSet_New
|
|
PyFrozenSet_Type=python38.PyFrozenSet_Type DATA
|
|
PyGC_Collect=python38.PyGC_Collect
|
|
PyGILState_Ensure=python38.PyGILState_Ensure
|
|
PyGILState_GetThisThreadState=python38.PyGILState_GetThisThreadState
|
|
PyGILState_Release=python38.PyGILState_Release
|
|
PyGetSetDescr_Type=python38.PyGetSetDescr_Type DATA
|
|
PyImport_AddModule=python38.PyImport_AddModule
|
|
PyImport_AddModuleObject=python38.PyImport_AddModuleObject
|
|
PyImport_AppendInittab=python38.PyImport_AppendInittab
|
|
PyImport_Cleanup=python38.PyImport_Cleanup
|
|
PyImport_ExecCodeModule=python38.PyImport_ExecCodeModule
|
|
PyImport_ExecCodeModuleEx=python38.PyImport_ExecCodeModuleEx
|
|
PyImport_ExecCodeModuleObject=python38.PyImport_ExecCodeModuleObject
|
|
PyImport_ExecCodeModuleWithPathnames=python38.PyImport_ExecCodeModuleWithPathnames
|
|
PyImport_GetImporter=python38.PyImport_GetImporter
|
|
PyImport_GetMagicNumber=python38.PyImport_GetMagicNumber
|
|
PyImport_GetMagicTag=python38.PyImport_GetMagicTag
|
|
PyImport_GetModule=python38.PyImport_GetModule
|
|
PyImport_GetModuleDict=python38.PyImport_GetModuleDict
|
|
PyImport_Import=python38.PyImport_Import
|
|
PyImport_ImportFrozenModule=python38.PyImport_ImportFrozenModule
|
|
PyImport_ImportFrozenModuleObject=python38.PyImport_ImportFrozenModuleObject
|
|
PyImport_ImportModule=python38.PyImport_ImportModule
|
|
PyImport_ImportModuleLevel=python38.PyImport_ImportModuleLevel
|
|
PyImport_ImportModuleLevelObject=python38.PyImport_ImportModuleLevelObject
|
|
PyImport_ImportModuleNoBlock=python38.PyImport_ImportModuleNoBlock
|
|
PyImport_ReloadModule=python38.PyImport_ReloadModule
|
|
PyIndex_Check=python38.PyIndex_Check
|
|
PyInterpreterState_Clear=python38.PyInterpreterState_Clear
|
|
PyInterpreterState_Delete=python38.PyInterpreterState_Delete
|
|
PyInterpreterState_New=python38.PyInterpreterState_New
|
|
PyIter_Check=python38.PyIter_Check
|
|
PyIter_Next=python38.PyIter_Next
|
|
PyListIter_Type=python38.PyListIter_Type DATA
|
|
PyListRevIter_Type=python38.PyListRevIter_Type DATA
|
|
PyList_Append=python38.PyList_Append
|
|
PyList_AsTuple=python38.PyList_AsTuple
|
|
PyList_GetItem=python38.PyList_GetItem
|
|
PyList_GetSlice=python38.PyList_GetSlice
|
|
PyList_Insert=python38.PyList_Insert
|
|
PyList_New=python38.PyList_New
|
|
PyList_Reverse=python38.PyList_Reverse
|
|
PyList_SetItem=python38.PyList_SetItem
|
|
PyList_SetSlice=python38.PyList_SetSlice
|
|
PyList_Size=python38.PyList_Size
|
|
PyList_Sort=python38.PyList_Sort
|
|
PyList_Type=python38.PyList_Type DATA
|
|
PyLongRangeIter_Type=python38.PyLongRangeIter_Type DATA
|
|
PyLong_AsDouble=python38.PyLong_AsDouble
|
|
PyLong_AsLong=python38.PyLong_AsLong
|
|
PyLong_AsLongAndOverflow=python38.PyLong_AsLongAndOverflow
|
|
PyLong_AsLongLong=python38.PyLong_AsLongLong
|
|
PyLong_AsLongLongAndOverflow=python38.PyLong_AsLongLongAndOverflow
|
|
PyLong_AsSize_t=python38.PyLong_AsSize_t
|
|
PyLong_AsSsize_t=python38.PyLong_AsSsize_t
|
|
PyLong_AsUnsignedLong=python38.PyLong_AsUnsignedLong
|
|
PyLong_AsUnsignedLongLong=python38.PyLong_AsUnsignedLongLong
|
|
PyLong_AsUnsignedLongLongMask=python38.PyLong_AsUnsignedLongLongMask
|
|
PyLong_AsUnsignedLongMask=python38.PyLong_AsUnsignedLongMask
|
|
PyLong_AsVoidPtr=python38.PyLong_AsVoidPtr
|
|
PyLong_FromDouble=python38.PyLong_FromDouble
|
|
PyLong_FromLong=python38.PyLong_FromLong
|
|
PyLong_FromLongLong=python38.PyLong_FromLongLong
|
|
PyLong_FromSize_t=python38.PyLong_FromSize_t
|
|
PyLong_FromSsize_t=python38.PyLong_FromSsize_t
|
|
PyLong_FromString=python38.PyLong_FromString
|
|
PyLong_FromUnsignedLong=python38.PyLong_FromUnsignedLong
|
|
PyLong_FromUnsignedLongLong=python38.PyLong_FromUnsignedLongLong
|
|
PyLong_FromVoidPtr=python38.PyLong_FromVoidPtr
|
|
PyLong_GetInfo=python38.PyLong_GetInfo
|
|
PyLong_Type=python38.PyLong_Type DATA
|
|
PyMap_Type=python38.PyMap_Type DATA
|
|
PyMapping_Check=python38.PyMapping_Check
|
|
PyMapping_GetItemString=python38.PyMapping_GetItemString
|
|
PyMapping_HasKey=python38.PyMapping_HasKey
|
|
PyMapping_HasKeyString=python38.PyMapping_HasKeyString
|
|
PyMapping_Items=python38.PyMapping_Items
|
|
PyMapping_Keys=python38.PyMapping_Keys
|
|
PyMapping_Length=python38.PyMapping_Length
|
|
PyMapping_SetItemString=python38.PyMapping_SetItemString
|
|
PyMapping_Size=python38.PyMapping_Size
|
|
PyMapping_Values=python38.PyMapping_Values
|
|
PyMem_Calloc=python38.PyMem_Calloc
|
|
PyMem_Free=python38.PyMem_Free
|
|
PyMem_Malloc=python38.PyMem_Malloc
|
|
PyMem_Realloc=python38.PyMem_Realloc
|
|
PyMemberDescr_Type=python38.PyMemberDescr_Type DATA
|
|
PyMemoryView_FromMemory=python38.PyMemoryView_FromMemory
|
|
PyMemoryView_FromObject=python38.PyMemoryView_FromObject
|
|
PyMemoryView_GetContiguous=python38.PyMemoryView_GetContiguous
|
|
PyMemoryView_Type=python38.PyMemoryView_Type DATA
|
|
PyMethodDescr_Type=python38.PyMethodDescr_Type DATA
|
|
PyModuleDef_Init=python38.PyModuleDef_Init
|
|
PyModuleDef_Type=python38.PyModuleDef_Type DATA
|
|
PyModule_AddFunctions=python38.PyModule_AddFunctions
|
|
PyModule_AddIntConstant=python38.PyModule_AddIntConstant
|
|
PyModule_AddObject=python38.PyModule_AddObject
|
|
PyModule_AddStringConstant=python38.PyModule_AddStringConstant
|
|
PyModule_Create2=python38.PyModule_Create2
|
|
PyModule_ExecDef=python38.PyModule_ExecDef
|
|
PyModule_FromDefAndSpec2=python38.PyModule_FromDefAndSpec2
|
|
PyModule_GetDef=python38.PyModule_GetDef
|
|
PyModule_GetDict=python38.PyModule_GetDict
|
|
PyModule_GetFilename=python38.PyModule_GetFilename
|
|
PyModule_GetFilenameObject=python38.PyModule_GetFilenameObject
|
|
PyModule_GetName=python38.PyModule_GetName
|
|
PyModule_GetNameObject=python38.PyModule_GetNameObject
|
|
PyModule_GetState=python38.PyModule_GetState
|
|
PyModule_New=python38.PyModule_New
|
|
PyModule_NewObject=python38.PyModule_NewObject
|
|
PyModule_SetDocString=python38.PyModule_SetDocString
|
|
PyModule_Type=python38.PyModule_Type DATA
|
|
PyNullImporter_Type=python38.PyNullImporter_Type DATA
|
|
PyNumber_Absolute=python38.PyNumber_Absolute
|
|
PyNumber_Add=python38.PyNumber_Add
|
|
PyNumber_And=python38.PyNumber_And
|
|
PyNumber_AsSsize_t=python38.PyNumber_AsSsize_t
|
|
PyNumber_Check=python38.PyNumber_Check
|
|
PyNumber_Divmod=python38.PyNumber_Divmod
|
|
PyNumber_Float=python38.PyNumber_Float
|
|
PyNumber_FloorDivide=python38.PyNumber_FloorDivide
|
|
PyNumber_InPlaceAdd=python38.PyNumber_InPlaceAdd
|
|
PyNumber_InPlaceAnd=python38.PyNumber_InPlaceAnd
|
|
PyNumber_InPlaceFloorDivide=python38.PyNumber_InPlaceFloorDivide
|
|
PyNumber_InPlaceLshift=python38.PyNumber_InPlaceLshift
|
|
PyNumber_InPlaceMatrixMultiply=python38.PyNumber_InPlaceMatrixMultiply
|
|
PyNumber_InPlaceMultiply=python38.PyNumber_InPlaceMultiply
|
|
PyNumber_InPlaceOr=python38.PyNumber_InPlaceOr
|
|
PyNumber_InPlacePower=python38.PyNumber_InPlacePower
|
|
PyNumber_InPlaceRemainder=python38.PyNumber_InPlaceRemainder
|
|
PyNumber_InPlaceRshift=python38.PyNumber_InPlaceRshift
|
|
PyNumber_InPlaceSubtract=python38.PyNumber_InPlaceSubtract
|
|
PyNumber_InPlaceTrueDivide=python38.PyNumber_InPlaceTrueDivide
|
|
PyNumber_InPlaceXor=python38.PyNumber_InPlaceXor
|
|
PyNumber_Index=python38.PyNumber_Index
|
|
PyNumber_Invert=python38.PyNumber_Invert
|
|
PyNumber_Long=python38.PyNumber_Long
|
|
PyNumber_Lshift=python38.PyNumber_Lshift
|
|
PyNumber_MatrixMultiply=python38.PyNumber_MatrixMultiply
|
|
PyNumber_Multiply=python38.PyNumber_Multiply
|
|
PyNumber_Negative=python38.PyNumber_Negative
|
|
PyNumber_Or=python38.PyNumber_Or
|
|
PyNumber_Positive=python38.PyNumber_Positive
|
|
PyNumber_Power=python38.PyNumber_Power
|
|
PyNumber_Remainder=python38.PyNumber_Remainder
|
|
PyNumber_Rshift=python38.PyNumber_Rshift
|
|
PyNumber_Subtract=python38.PyNumber_Subtract
|
|
PyNumber_ToBase=python38.PyNumber_ToBase
|
|
PyNumber_TrueDivide=python38.PyNumber_TrueDivide
|
|
PyNumber_Xor=python38.PyNumber_Xor
|
|
PyODictItems_Type=python38.PyODictItems_Type DATA
|
|
PyODictIter_Type=python38.PyODictIter_Type DATA
|
|
PyODictKeys_Type=python38.PyODictKeys_Type DATA
|
|
PyODictValues_Type=python38.PyODictValues_Type DATA
|
|
PyODict_DelItem=python38.PyODict_DelItem
|
|
PyODict_New=python38.PyODict_New
|
|
PyODict_SetItem=python38.PyODict_SetItem
|
|
PyODict_Type=python38.PyODict_Type DATA
|
|
PyOS_AfterFork=python38.PyOS_AfterFork
|
|
PyOS_CheckStack=python38.PyOS_CheckStack
|
|
PyOS_FSPath=python38.PyOS_FSPath
|
|
PyOS_InitInterrupts=python38.PyOS_InitInterrupts
|
|
PyOS_InputHook=python38.PyOS_InputHook DATA
|
|
PyOS_InterruptOccurred=python38.PyOS_InterruptOccurred
|
|
PyOS_ReadlineFunctionPointer=python38.PyOS_ReadlineFunctionPointer DATA
|
|
PyOS_double_to_string=python38.PyOS_double_to_string
|
|
PyOS_getsig=python38.PyOS_getsig
|
|
PyOS_mystricmp=python38.PyOS_mystricmp
|
|
PyOS_mystrnicmp=python38.PyOS_mystrnicmp
|
|
PyOS_setsig=python38.PyOS_setsig
|
|
PyOS_snprintf=python38.PyOS_snprintf
|
|
PyOS_string_to_double=python38.PyOS_string_to_double
|
|
PyOS_strtol=python38.PyOS_strtol
|
|
PyOS_strtoul=python38.PyOS_strtoul
|
|
PyOS_vsnprintf=python38.PyOS_vsnprintf
|
|
PyObject_ASCII=python38.PyObject_ASCII
|
|
PyObject_AsCharBuffer=python38.PyObject_AsCharBuffer
|
|
PyObject_AsFileDescriptor=python38.PyObject_AsFileDescriptor
|
|
PyObject_AsReadBuffer=python38.PyObject_AsReadBuffer
|
|
PyObject_AsWriteBuffer=python38.PyObject_AsWriteBuffer
|
|
PyObject_Bytes=python38.PyObject_Bytes
|
|
PyObject_Call=python38.PyObject_Call
|
|
PyObject_CallFunction=python38.PyObject_CallFunction
|
|
PyObject_CallFunctionObjArgs=python38.PyObject_CallFunctionObjArgs
|
|
PyObject_CallMethod=python38.PyObject_CallMethod
|
|
PyObject_CallMethodObjArgs=python38.PyObject_CallMethodObjArgs
|
|
PyObject_CallObject=python38.PyObject_CallObject
|
|
PyObject_Calloc=python38.PyObject_Calloc
|
|
PyObject_CheckReadBuffer=python38.PyObject_CheckReadBuffer
|
|
PyObject_ClearWeakRefs=python38.PyObject_ClearWeakRefs
|
|
PyObject_DelItem=python38.PyObject_DelItem
|
|
PyObject_DelItemString=python38.PyObject_DelItemString
|
|
PyObject_Dir=python38.PyObject_Dir
|
|
PyObject_Format=python38.PyObject_Format
|
|
PyObject_Free=python38.PyObject_Free
|
|
PyObject_GC_Del=python38.PyObject_GC_Del
|
|
PyObject_GC_Track=python38.PyObject_GC_Track
|
|
PyObject_GC_UnTrack=python38.PyObject_GC_UnTrack
|
|
PyObject_GenericGetAttr=python38.PyObject_GenericGetAttr
|
|
PyObject_GenericSetAttr=python38.PyObject_GenericSetAttr
|
|
PyObject_GenericSetDict=python38.PyObject_GenericSetDict
|
|
PyObject_GetAttr=python38.PyObject_GetAttr
|
|
PyObject_GetAttrString=python38.PyObject_GetAttrString
|
|
PyObject_GetItem=python38.PyObject_GetItem
|
|
PyObject_GetIter=python38.PyObject_GetIter
|
|
PyObject_HasAttr=python38.PyObject_HasAttr
|
|
PyObject_HasAttrString=python38.PyObject_HasAttrString
|
|
PyObject_Hash=python38.PyObject_Hash
|
|
PyObject_HashNotImplemented=python38.PyObject_HashNotImplemented
|
|
PyObject_Init=python38.PyObject_Init
|
|
PyObject_InitVar=python38.PyObject_InitVar
|
|
PyObject_IsInstance=python38.PyObject_IsInstance
|
|
PyObject_IsSubclass=python38.PyObject_IsSubclass
|
|
PyObject_IsTrue=python38.PyObject_IsTrue
|
|
PyObject_Length=python38.PyObject_Length
|
|
PyObject_Malloc=python38.PyObject_Malloc
|
|
PyObject_Not=python38.PyObject_Not
|
|
PyObject_Realloc=python38.PyObject_Realloc
|
|
PyObject_Repr=python38.PyObject_Repr
|
|
PyObject_RichCompare=python38.PyObject_RichCompare
|
|
PyObject_RichCompareBool=python38.PyObject_RichCompareBool
|
|
PyObject_SelfIter=python38.PyObject_SelfIter
|
|
PyObject_SetAttr=python38.PyObject_SetAttr
|
|
PyObject_SetAttrString=python38.PyObject_SetAttrString
|
|
PyObject_SetItem=python38.PyObject_SetItem
|
|
PyObject_Size=python38.PyObject_Size
|
|
PyObject_Str=python38.PyObject_Str
|
|
PyObject_Type=python38.PyObject_Type
|
|
PyParser_SimpleParseFileFlags=python38.PyParser_SimpleParseFileFlags
|
|
PyParser_SimpleParseStringFlags=python38.PyParser_SimpleParseStringFlags
|
|
PyParser_SimpleParseStringFlagsFilename=python38.PyParser_SimpleParseStringFlagsFilename
|
|
PyProperty_Type=python38.PyProperty_Type DATA
|
|
PyRangeIter_Type=python38.PyRangeIter_Type DATA
|
|
PyRange_Type=python38.PyRange_Type DATA
|
|
PyReversed_Type=python38.PyReversed_Type DATA
|
|
PySeqIter_New=python38.PySeqIter_New
|
|
PySeqIter_Type=python38.PySeqIter_Type DATA
|
|
PySequence_Check=python38.PySequence_Check
|
|
PySequence_Concat=python38.PySequence_Concat
|
|
PySequence_Contains=python38.PySequence_Contains
|
|
PySequence_Count=python38.PySequence_Count
|
|
PySequence_DelItem=python38.PySequence_DelItem
|
|
PySequence_DelSlice=python38.PySequence_DelSlice
|
|
PySequence_Fast=python38.PySequence_Fast
|
|
PySequence_GetItem=python38.PySequence_GetItem
|
|
PySequence_GetSlice=python38.PySequence_GetSlice
|
|
PySequence_In=python38.PySequence_In
|
|
PySequence_InPlaceConcat=python38.PySequence_InPlaceConcat
|
|
PySequence_InPlaceRepeat=python38.PySequence_InPlaceRepeat
|
|
PySequence_Index=python38.PySequence_Index
|
|
PySequence_Length=python38.PySequence_Length
|
|
PySequence_List=python38.PySequence_List
|
|
PySequence_Repeat=python38.PySequence_Repeat
|
|
PySequence_SetItem=python38.PySequence_SetItem
|
|
PySequence_SetSlice=python38.PySequence_SetSlice
|
|
PySequence_Size=python38.PySequence_Size
|
|
PySequence_Tuple=python38.PySequence_Tuple
|
|
PySetIter_Type=python38.PySetIter_Type DATA
|
|
PySet_Add=python38.PySet_Add
|
|
PySet_Clear=python38.PySet_Clear
|
|
PySet_Contains=python38.PySet_Contains
|
|
PySet_Discard=python38.PySet_Discard
|
|
PySet_New=python38.PySet_New
|
|
PySet_Pop=python38.PySet_Pop
|
|
PySet_Size=python38.PySet_Size
|
|
PySet_Type=python38.PySet_Type DATA
|
|
PySlice_AdjustIndices=python38.PySlice_AdjustIndices
|
|
PySlice_GetIndices=python38.PySlice_GetIndices
|
|
PySlice_GetIndicesEx=python38.PySlice_GetIndicesEx
|
|
PySlice_New=python38.PySlice_New
|
|
PySlice_Type=python38.PySlice_Type DATA
|
|
PySlice_Unpack=python38.PySlice_Unpack
|
|
PySortWrapper_Type=python38.PySortWrapper_Type DATA
|
|
PyInterpreterState_GetID=python38.PyInterpreterState_GetID
|
|
PyState_AddModule=python38.PyState_AddModule
|
|
PyState_FindModule=python38.PyState_FindModule
|
|
PyState_RemoveModule=python38.PyState_RemoveModule
|
|
PyStructSequence_GetItem=python38.PyStructSequence_GetItem
|
|
PyStructSequence_New=python38.PyStructSequence_New
|
|
PyStructSequence_NewType=python38.PyStructSequence_NewType
|
|
PyStructSequence_SetItem=python38.PyStructSequence_SetItem
|
|
PySuper_Type=python38.PySuper_Type DATA
|
|
PySys_AddWarnOption=python38.PySys_AddWarnOption
|
|
PySys_AddWarnOptionUnicode=python38.PySys_AddWarnOptionUnicode
|
|
PySys_AddXOption=python38.PySys_AddXOption
|
|
PySys_FormatStderr=python38.PySys_FormatStderr
|
|
PySys_FormatStdout=python38.PySys_FormatStdout
|
|
PySys_GetObject=python38.PySys_GetObject
|
|
PySys_GetXOptions=python38.PySys_GetXOptions
|
|
PySys_HasWarnOptions=python38.PySys_HasWarnOptions
|
|
PySys_ResetWarnOptions=python38.PySys_ResetWarnOptions
|
|
PySys_SetArgv=python38.PySys_SetArgv
|
|
PySys_SetArgvEx=python38.PySys_SetArgvEx
|
|
PySys_SetObject=python38.PySys_SetObject
|
|
PySys_SetPath=python38.PySys_SetPath
|
|
PySys_WriteStderr=python38.PySys_WriteStderr
|
|
PySys_WriteStdout=python38.PySys_WriteStdout
|
|
PyThreadState_Clear=python38.PyThreadState_Clear
|
|
PyThreadState_Delete=python38.PyThreadState_Delete
|
|
PyThreadState_DeleteCurrent=python38.PyThreadState_DeleteCurrent
|
|
PyThreadState_Get=python38.PyThreadState_Get
|
|
PyThreadState_GetDict=python38.PyThreadState_GetDict
|
|
PyThreadState_New=python38.PyThreadState_New
|
|
PyThreadState_SetAsyncExc=python38.PyThreadState_SetAsyncExc
|
|
PyThreadState_Swap=python38.PyThreadState_Swap
|
|
PyThread_tss_alloc=python38.PyThread_tss_alloc
|
|
PyThread_tss_create=python38.PyThread_tss_create
|
|
PyThread_tss_delete=python38.PyThread_tss_delete
|
|
PyThread_tss_free=python38.PyThread_tss_free
|
|
PyThread_tss_get=python38.PyThread_tss_get
|
|
PyThread_tss_is_created=python38.PyThread_tss_is_created
|
|
PyThread_tss_set=python38.PyThread_tss_set
|
|
PyTraceBack_Here=python38.PyTraceBack_Here
|
|
PyTraceBack_Print=python38.PyTraceBack_Print
|
|
PyTraceBack_Type=python38.PyTraceBack_Type DATA
|
|
PyTupleIter_Type=python38.PyTupleIter_Type DATA
|
|
PyTuple_ClearFreeList=python38.PyTuple_ClearFreeList
|
|
PyTuple_GetItem=python38.PyTuple_GetItem
|
|
PyTuple_GetSlice=python38.PyTuple_GetSlice
|
|
PyTuple_New=python38.PyTuple_New
|
|
PyTuple_Pack=python38.PyTuple_Pack
|
|
PyTuple_SetItem=python38.PyTuple_SetItem
|
|
PyTuple_Size=python38.PyTuple_Size
|
|
PyTuple_Type=python38.PyTuple_Type DATA
|
|
PyType_ClearCache=python38.PyType_ClearCache
|
|
PyType_FromSpec=python38.PyType_FromSpec
|
|
PyType_FromSpecWithBases=python38.PyType_FromSpecWithBases
|
|
PyType_GenericAlloc=python38.PyType_GenericAlloc
|
|
PyType_GenericNew=python38.PyType_GenericNew
|
|
PyType_GetFlags=python38.PyType_GetFlags
|
|
PyType_GetSlot=python38.PyType_GetSlot
|
|
PyType_IsSubtype=python38.PyType_IsSubtype
|
|
PyType_Modified=python38.PyType_Modified
|
|
PyType_Ready=python38.PyType_Ready
|
|
PyType_Type=python38.PyType_Type DATA
|
|
PyUnicodeDecodeError_Create=python38.PyUnicodeDecodeError_Create
|
|
PyUnicodeDecodeError_GetEncoding=python38.PyUnicodeDecodeError_GetEncoding
|
|
PyUnicodeDecodeError_GetEnd=python38.PyUnicodeDecodeError_GetEnd
|
|
PyUnicodeDecodeError_GetObject=python38.PyUnicodeDecodeError_GetObject
|
|
PyUnicodeDecodeError_GetReason=python38.PyUnicodeDecodeError_GetReason
|
|
PyUnicodeDecodeError_GetStart=python38.PyUnicodeDecodeError_GetStart
|
|
PyUnicodeDecodeError_SetEnd=python38.PyUnicodeDecodeError_SetEnd
|
|
PyUnicodeDecodeError_SetReason=python38.PyUnicodeDecodeError_SetReason
|
|
PyUnicodeDecodeError_SetStart=python38.PyUnicodeDecodeError_SetStart
|
|
PyUnicodeEncodeError_GetEncoding=python38.PyUnicodeEncodeError_GetEncoding
|
|
PyUnicodeEncodeError_GetEnd=python38.PyUnicodeEncodeError_GetEnd
|
|
PyUnicodeEncodeError_GetObject=python38.PyUnicodeEncodeError_GetObject
|
|
PyUnicodeEncodeError_GetReason=python38.PyUnicodeEncodeError_GetReason
|
|
PyUnicodeEncodeError_GetStart=python38.PyUnicodeEncodeError_GetStart
|
|
PyUnicodeEncodeError_SetEnd=python38.PyUnicodeEncodeError_SetEnd
|
|
PyUnicodeEncodeError_SetReason=python38.PyUnicodeEncodeError_SetReason
|
|
PyUnicodeEncodeError_SetStart=python38.PyUnicodeEncodeError_SetStart
|
|
PyUnicodeIter_Type=python38.PyUnicodeIter_Type DATA
|
|
PyUnicodeTranslateError_GetEnd=python38.PyUnicodeTranslateError_GetEnd
|
|
PyUnicodeTranslateError_GetObject=python38.PyUnicodeTranslateError_GetObject
|
|
PyUnicodeTranslateError_GetReason=python38.PyUnicodeTranslateError_GetReason
|
|
PyUnicodeTranslateError_GetStart=python38.PyUnicodeTranslateError_GetStart
|
|
PyUnicodeTranslateError_SetEnd=python38.PyUnicodeTranslateError_SetEnd
|
|
PyUnicodeTranslateError_SetReason=python38.PyUnicodeTranslateError_SetReason
|
|
PyUnicodeTranslateError_SetStart=python38.PyUnicodeTranslateError_SetStart
|
|
PyUnicode_Append=python38.PyUnicode_Append
|
|
PyUnicode_AppendAndDel=python38.PyUnicode_AppendAndDel
|
|
PyUnicode_AsASCIIString=python38.PyUnicode_AsASCIIString
|
|
PyUnicode_AsCharmapString=python38.PyUnicode_AsCharmapString
|
|
PyUnicode_AsDecodedObject=python38.PyUnicode_AsDecodedObject
|
|
PyUnicode_AsDecodedUnicode=python38.PyUnicode_AsDecodedUnicode
|
|
PyUnicode_AsEncodedObject=python38.PyUnicode_AsEncodedObject
|
|
PyUnicode_AsEncodedString=python38.PyUnicode_AsEncodedString
|
|
PyUnicode_AsEncodedUnicode=python38.PyUnicode_AsEncodedUnicode
|
|
PyUnicode_AsLatin1String=python38.PyUnicode_AsLatin1String
|
|
PyUnicode_AsMBCSString=python38.PyUnicode_AsMBCSString
|
|
PyUnicode_AsRawUnicodeEscapeString=python38.PyUnicode_AsRawUnicodeEscapeString
|
|
PyUnicode_AsUCS4=python38.PyUnicode_AsUCS4
|
|
PyUnicode_AsUCS4Copy=python38.PyUnicode_AsUCS4Copy
|
|
PyUnicode_AsUTF16String=python38.PyUnicode_AsUTF16String
|
|
PyUnicode_AsUTF32String=python38.PyUnicode_AsUTF32String
|
|
PyUnicode_AsUTF8String=python38.PyUnicode_AsUTF8String
|
|
PyUnicode_AsUnicodeEscapeString=python38.PyUnicode_AsUnicodeEscapeString
|
|
PyUnicode_AsWideChar=python38.PyUnicode_AsWideChar
|
|
PyUnicode_AsWideCharString=python38.PyUnicode_AsWideCharString
|
|
PyUnicode_BuildEncodingMap=python38.PyUnicode_BuildEncodingMap
|
|
PyUnicode_ClearFreeList=python38.PyUnicode_ClearFreeList
|
|
PyUnicode_Compare=python38.PyUnicode_Compare
|
|
PyUnicode_CompareWithASCIIString=python38.PyUnicode_CompareWithASCIIString
|
|
PyUnicode_Concat=python38.PyUnicode_Concat
|
|
PyUnicode_Contains=python38.PyUnicode_Contains
|
|
PyUnicode_Count=python38.PyUnicode_Count
|
|
PyUnicode_Decode=python38.PyUnicode_Decode
|
|
PyUnicode_DecodeASCII=python38.PyUnicode_DecodeASCII
|
|
PyUnicode_DecodeCharmap=python38.PyUnicode_DecodeCharmap
|
|
PyUnicode_DecodeCodePageStateful=python38.PyUnicode_DecodeCodePageStateful
|
|
PyUnicode_DecodeFSDefault=python38.PyUnicode_DecodeFSDefault
|
|
PyUnicode_DecodeFSDefaultAndSize=python38.PyUnicode_DecodeFSDefaultAndSize
|
|
PyUnicode_DecodeLatin1=python38.PyUnicode_DecodeLatin1
|
|
PyUnicode_DecodeLocale=python38.PyUnicode_DecodeLocale
|
|
PyUnicode_DecodeLocaleAndSize=python38.PyUnicode_DecodeLocaleAndSize
|
|
PyUnicode_DecodeMBCS=python38.PyUnicode_DecodeMBCS
|
|
PyUnicode_DecodeMBCSStateful=python38.PyUnicode_DecodeMBCSStateful
|
|
PyUnicode_DecodeRawUnicodeEscape=python38.PyUnicode_DecodeRawUnicodeEscape
|
|
PyUnicode_DecodeUTF16=python38.PyUnicode_DecodeUTF16
|
|
PyUnicode_DecodeUTF16Stateful=python38.PyUnicode_DecodeUTF16Stateful
|
|
PyUnicode_DecodeUTF32=python38.PyUnicode_DecodeUTF32
|
|
PyUnicode_DecodeUTF32Stateful=python38.PyUnicode_DecodeUTF32Stateful
|
|
PyUnicode_DecodeUTF7=python38.PyUnicode_DecodeUTF7
|
|
PyUnicode_DecodeUTF7Stateful=python38.PyUnicode_DecodeUTF7Stateful
|
|
PyUnicode_DecodeUTF8=python38.PyUnicode_DecodeUTF8
|
|
PyUnicode_DecodeUTF8Stateful=python38.PyUnicode_DecodeUTF8Stateful
|
|
PyUnicode_DecodeUnicodeEscape=python38.PyUnicode_DecodeUnicodeEscape
|
|
PyUnicode_EncodeCodePage=python38.PyUnicode_EncodeCodePage
|
|
PyUnicode_EncodeFSDefault=python38.PyUnicode_EncodeFSDefault
|
|
PyUnicode_EncodeLocale=python38.PyUnicode_EncodeLocale
|
|
PyUnicode_FSConverter=python38.PyUnicode_FSConverter
|
|
PyUnicode_FSDecoder=python38.PyUnicode_FSDecoder
|
|
PyUnicode_Find=python38.PyUnicode_Find
|
|
PyUnicode_FindChar=python38.PyUnicode_FindChar
|
|
PyUnicode_Format=python38.PyUnicode_Format
|
|
PyUnicode_FromEncodedObject=python38.PyUnicode_FromEncodedObject
|
|
PyUnicode_FromFormat=python38.PyUnicode_FromFormat
|
|
PyUnicode_FromFormatV=python38.PyUnicode_FromFormatV
|
|
PyUnicode_FromObject=python38.PyUnicode_FromObject
|
|
PyUnicode_FromOrdinal=python38.PyUnicode_FromOrdinal
|
|
PyUnicode_FromString=python38.PyUnicode_FromString
|
|
PyUnicode_FromStringAndSize=python38.PyUnicode_FromStringAndSize
|
|
PyUnicode_FromWideChar=python38.PyUnicode_FromWideChar
|
|
PyUnicode_GetDefaultEncoding=python38.PyUnicode_GetDefaultEncoding
|
|
PyUnicode_GetLength=python38.PyUnicode_GetLength
|
|
PyUnicode_GetSize=python38.PyUnicode_GetSize
|
|
PyUnicode_InternFromString=python38.PyUnicode_InternFromString
|
|
PyUnicode_InternImmortal=python38.PyUnicode_InternImmortal
|
|
PyUnicode_InternInPlace=python38.PyUnicode_InternInPlace
|
|
PyUnicode_IsIdentifier=python38.PyUnicode_IsIdentifier
|
|
PyUnicode_Join=python38.PyUnicode_Join
|
|
PyUnicode_Partition=python38.PyUnicode_Partition
|
|
PyUnicode_RPartition=python38.PyUnicode_RPartition
|
|
PyUnicode_RSplit=python38.PyUnicode_RSplit
|
|
PyUnicode_ReadChar=python38.PyUnicode_ReadChar
|
|
PyUnicode_Replace=python38.PyUnicode_Replace
|
|
PyUnicode_Resize=python38.PyUnicode_Resize
|
|
PyUnicode_RichCompare=python38.PyUnicode_RichCompare
|
|
PyUnicode_Split=python38.PyUnicode_Split
|
|
PyUnicode_Splitlines=python38.PyUnicode_Splitlines
|
|
PyUnicode_Substring=python38.PyUnicode_Substring
|
|
PyUnicode_Tailmatch=python38.PyUnicode_Tailmatch
|
|
PyUnicode_Translate=python38.PyUnicode_Translate
|
|
PyUnicode_Type=python38.PyUnicode_Type DATA
|
|
PyUnicode_WriteChar=python38.PyUnicode_WriteChar
|
|
PyWeakref_GetObject=python38.PyWeakref_GetObject
|
|
PyWeakref_NewProxy=python38.PyWeakref_NewProxy
|
|
PyWeakref_NewRef=python38.PyWeakref_NewRef
|
|
PyWrapperDescr_Type=python38.PyWrapperDescr_Type DATA
|
|
PyWrapper_New=python38.PyWrapper_New
|
|
PyZip_Type=python38.PyZip_Type DATA
|
|
Py_AddPendingCall=python38.Py_AddPendingCall
|
|
Py_AtExit=python38.Py_AtExit
|
|
Py_BuildValue=python38.Py_BuildValue
|
|
Py_CompileString=python38.Py_CompileString
|
|
Py_DecRef=python38.Py_DecRef
|
|
Py_DecodeLocale=python38.Py_DecodeLocale
|
|
Py_EncodeLocale=python38.Py_EncodeLocale
|
|
Py_EndInterpreter=python38.Py_EndInterpreter
|
|
Py_Exit=python38.Py_Exit
|
|
Py_FatalError=python38.Py_FatalError
|
|
Py_FileSystemDefaultEncodeErrors=python38.Py_FileSystemDefaultEncodeErrors DATA
|
|
Py_FileSystemDefaultEncoding=python38.Py_FileSystemDefaultEncoding DATA
|
|
Py_Finalize=python38.Py_Finalize
|
|
Py_FinalizeEx=python38.Py_FinalizeEx
|
|
Py_GetBuildInfo=python38.Py_GetBuildInfo
|
|
Py_GetCompiler=python38.Py_GetCompiler
|
|
Py_GetCopyright=python38.Py_GetCopyright
|
|
Py_GetExecPrefix=python38.Py_GetExecPrefix
|
|
Py_GetPath=python38.Py_GetPath
|
|
Py_GetPlatform=python38.Py_GetPlatform
|
|
Py_GetPrefix=python38.Py_GetPrefix
|
|
Py_GetProgramFullPath=python38.Py_GetProgramFullPath
|
|
Py_GetProgramName=python38.Py_GetProgramName
|
|
Py_GetPythonHome=python38.Py_GetPythonHome
|
|
Py_GetRecursionLimit=python38.Py_GetRecursionLimit
|
|
Py_GetVersion=python38.Py_GetVersion
|
|
Py_HasFileSystemDefaultEncoding=python38.Py_HasFileSystemDefaultEncoding DATA
|
|
Py_IncRef=python38.Py_IncRef
|
|
Py_Initialize=python38.Py_Initialize
|
|
Py_InitializeEx=python38.Py_InitializeEx
|
|
Py_IsInitialized=python38.Py_IsInitialized
|
|
Py_Main=python38.Py_Main
|
|
Py_MakePendingCalls=python38.Py_MakePendingCalls
|
|
Py_NewInterpreter=python38.Py_NewInterpreter
|
|
Py_ReprEnter=python38.Py_ReprEnter
|
|
Py_ReprLeave=python38.Py_ReprLeave
|
|
Py_SetPath=python38.Py_SetPath
|
|
Py_SetProgramName=python38.Py_SetProgramName
|
|
Py_SetPythonHome=python38.Py_SetPythonHome
|
|
Py_SetRecursionLimit=python38.Py_SetRecursionLimit
|
|
Py_SymtableString=python38.Py_SymtableString
|
|
Py_UTF8Mode=python38.Py_UTF8Mode DATA
|
|
Py_VaBuildValue=python38.Py_VaBuildValue
|
|
_PyArg_ParseTupleAndKeywords_SizeT=python38._PyArg_ParseTupleAndKeywords_SizeT
|
|
_PyArg_ParseTuple_SizeT=python38._PyArg_ParseTuple_SizeT
|
|
_PyArg_Parse_SizeT=python38._PyArg_Parse_SizeT
|
|
_PyArg_VaParseTupleAndKeywords_SizeT=python38._PyArg_VaParseTupleAndKeywords_SizeT
|
|
_PyArg_VaParse_SizeT=python38._PyArg_VaParse_SizeT
|
|
_PyErr_BadInternalCall=python38._PyErr_BadInternalCall
|
|
_PyObject_CallFunction_SizeT=python38._PyObject_CallFunction_SizeT
|
|
_PyObject_CallMethod_SizeT=python38._PyObject_CallMethod_SizeT
|
|
_PyObject_GC_Malloc=python38._PyObject_GC_Malloc
|
|
_PyObject_GC_New=python38._PyObject_GC_New
|
|
_PyObject_GC_NewVar=python38._PyObject_GC_NewVar
|
|
_PyObject_GC_Resize=python38._PyObject_GC_Resize
|
|
_PyObject_New=python38._PyObject_New
|
|
_PyObject_NewVar=python38._PyObject_NewVar
|
|
_PyState_AddModule=python38._PyState_AddModule
|
|
_PyThreadState_Init=python38._PyThreadState_Init
|
|
_PyThreadState_Prealloc=python38._PyThreadState_Prealloc
|
|
_PyTrash_delete_later=python38._PyTrash_delete_later DATA
|
|
_PyTrash_delete_nesting=python38._PyTrash_delete_nesting DATA
|
|
_PyTrash_deposit_object=python38._PyTrash_deposit_object
|
|
_PyTrash_destroy_chain=python38._PyTrash_destroy_chain
|
|
_PyTrash_thread_deposit_object=python38._PyTrash_thread_deposit_object
|
|
_PyTrash_thread_destroy_chain=python38._PyTrash_thread_destroy_chain
|
|
_PyWeakref_CallableProxyType=python38._PyWeakref_CallableProxyType DATA
|
|
_PyWeakref_ProxyType=python38._PyWeakref_ProxyType DATA
|
|
_PyWeakref_RefType=python38._PyWeakref_RefType DATA
|
|
_Py_BuildValue_SizeT=python38._Py_BuildValue_SizeT
|
|
_Py_CheckRecursionLimit=python38._Py_CheckRecursionLimit DATA
|
|
_Py_CheckRecursiveCall=python38._Py_CheckRecursiveCall
|
|
_Py_Dealloc=python38._Py_Dealloc
|
|
_Py_EllipsisObject=python38._Py_EllipsisObject DATA
|
|
_Py_FalseStruct=python38._Py_FalseStruct DATA
|
|
_Py_NoneStruct=python38._Py_NoneStruct DATA
|
|
_Py_NotImplementedStruct=python38._Py_NotImplementedStruct DATA
|
|
_Py_SwappedOp=python38._Py_SwappedOp DATA
|
|
_Py_TrueStruct=python38._Py_TrueStruct DATA
|
|
_Py_VaBuildValue_SizeT=python38._Py_VaBuildValue_SizeT
|