mirror of
https://invent.kde.org/system/dolphin
synced 2024-09-12 12:54:29 +00:00
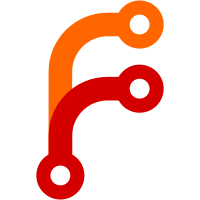
Have the secondary ViewContainer slide into/out of view when split view mode is switched on or off by the user. This should help users understand what split view mode is about. Without the animation it might seem like the only thing the button does is creating a weird vertical line in the middle of the view or something. With the animation it should be clear that the second view is a separate entity that was added. The closing animation will help users understand which of the ViewContainers was just closed.
79 lines
2.2 KiB
C++
79 lines
2.2 KiB
C++
/*
|
|
* SPDX-FileCopyrightText: 2015 Ashish Bansal <bansal.ashish096@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0-or-later
|
|
*/
|
|
|
|
#ifndef GLOBAL_H
|
|
#define GLOBAL_H
|
|
|
|
#include <QList>
|
|
#include <QUrl>
|
|
#include <QWidget>
|
|
|
|
class KConfigGroup;
|
|
class OrgKdeDolphinMainWindowInterface;
|
|
|
|
namespace Dolphin {
|
|
QList<QUrl> validateUris(const QStringList& uriList);
|
|
|
|
/**
|
|
* Returns the home url which is defined in General Settings
|
|
*/
|
|
QUrl homeUrl();
|
|
|
|
enum class OpenNewWindowFlag {
|
|
None = 0,
|
|
Select = 1<<1
|
|
};
|
|
Q_DECLARE_FLAGS(OpenNewWindowFlags, OpenNewWindowFlag)
|
|
|
|
/**
|
|
* Opens a new Dolphin window
|
|
*/
|
|
void openNewWindow(const QList<QUrl> &urls = {}, QWidget *window = nullptr, const OpenNewWindowFlags &flags = OpenNewWindowFlag::None);
|
|
|
|
/**
|
|
* Attaches URLs to an existing Dolphin instance if possible.
|
|
* If @p preferredService is a valid dbus service, it will be tried first.
|
|
* @p preferredService needs to support the org.kde.dolphin.MainWindow dbus interface with the /dolphin/Dolphin_1 path.
|
|
* Returns true if the URLs were successfully attached.
|
|
*/
|
|
bool attachToExistingInstance(const QList<QUrl>& inputUrls, bool openFiles, bool splitView, const QString& preferredService = QString());
|
|
|
|
/**
|
|
* Returns a QVector with all GUI-capable Dolphin instances
|
|
*/
|
|
QVector<QPair<QSharedPointer<OrgKdeDolphinMainWindowInterface>, QStringList>> dolphinGuiInstances(const QString& preferredService);
|
|
|
|
/**
|
|
* TODO: Move this somewhere global to all KDE apps, not just Dolphin
|
|
*/
|
|
const int VERTICAL_SPACER_HEIGHT = 12;
|
|
const int LAYOUT_SPACING_SMALL = 2;
|
|
}
|
|
|
|
class GlobalConfig : public QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
GlobalConfig() = delete;
|
|
|
|
/**
|
|
* @return a value from the global KDE config that should be
|
|
* multiplied with every animation duration once.
|
|
* 0.0 is returned if animations are globally turned off.
|
|
* 1.0 is the default value.
|
|
*/
|
|
static double animationDurationFactor();
|
|
|
|
private:
|
|
static void updateAnimationDurationFactor(const KConfigGroup &group, const QByteArrayList &names);
|
|
|
|
private:
|
|
static double s_animationDurationFactor;
|
|
};
|
|
|
|
#endif //GLOBAL_H
|