--- obj: application repo: https://github.com/charmbracelet/gum rev: 2024-03-27 --- # gum Gum provides highly configurable, ready-to-use utilities to help you write useful [shell](Shell.md) scripts and dotfiles aliases with just a few lines of code. 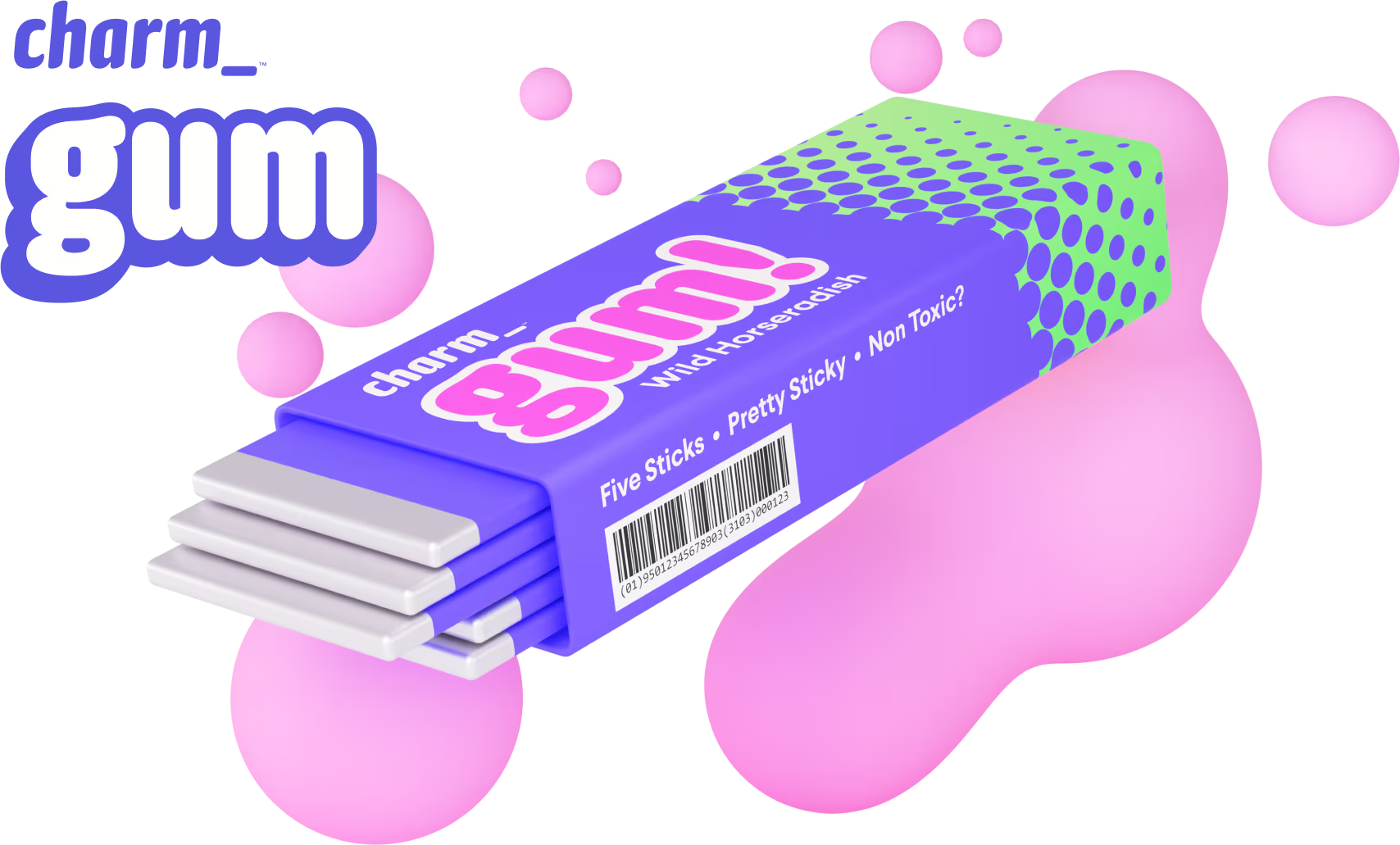 ## Usage **Example:** ```shell #!/bin/sh TYPE=$(gum choose "fix" "feat" "docs" "style" "refactor" "test" "chore" "revert") SCOPE=$(gum input --placeholder "scope") # Since the scope is optional, wrap it in parentheses if it has a value. test -n "$SCOPE" && SCOPE="($SCOPE)" # Pre-populate the input with the type(scope): so that the user may change it SUMMARY=$(gum input --value "$TYPE$SCOPE: " --placeholder "Summary of this change") DESCRIPTION=$(gum write --placeholder "Details of this change (CTRL+D to finish)") # Commit these changes gum confirm "Commit changes?" && git commit -m "$SUMMARY" -m "$DESCRIPTION" ``` ### gum choose Choose an option from a list of choices Usage: `gum choose [<options> ...]` | Option | Environment Variable | Description | | ----------------------------- | --------------------------------- | -------------------------------------------------------------- | | `--ordered` | `$GUM_CHOOSE_ORDERED` | Maintain the order of the selected options | | `--height=10` | `$GUM_CHOOSE_HEIGHT` | Height of the list | | `--cursor="> "` | `$GUM_CHOOSE_CURSOR` | Prefix to show on item that corresponds to the cursor position | | `--header=""` | `$GUM_CHOOSE_HEADER` | Header value | | `--cursor-prefix="○ "` | `$GUM_CHOOSE_CURSOR_PREFIX` | Prefix to show on the cursor item (hidden if limit is 1) | | `--selected-prefix="◉ "` | `$GUM_CHOOSE_SELECTED_PREFIX` | Prefix to show on selected items (hidden if limit is 1) | | `--unselected-prefix="○ "` | `$GUM_CHOOSE_UNSELECTED_PREFIX` | Prefix to show on unselected items (hidden if limit is 1) | | `--selected=,...` | `$GUM_CHOOSE_SELECTED` | Options that should start as selected | | `--timeout=0` | `$GUM_CCHOOSE_TIMEOUT` | Timeout until choose returns selected element | | `--limit=1` | - | Maximum number of options to pick | | `--no-limit` | - | Pick unlimited number of options (ignores limit) | | `--select-if-one` | - | Select the given option if there is only one | | `--cursor.foreground="212"` | `$GUM_CHOOSE_CURSOR_FOREGROUND` | Foreground Color | | `--header.foreground="240"` | `$GUM_CHOOSE_HEADER_FOREGROUND` | Foreground Color | | `--item.foreground=""` | `$GUM_CHOOSE_ITEM_FOREGROUND` | Foreground Color | | `--selected.foreground="212"` | `$GUM_CHOOSE_SELECTED_FOREGROUND` | Foreground Color | **Example**: Choose an option from a list of choices. ```shell echo "Pick a card, any card..." CARD=$(gum choose --height 15 {{A,K,Q,J},{10..2}}" "{♠,♥,♣,♦}) echo "Was your card the $CARD?" ``` ### gum confirm Ask a user to confirm an action Usage: `gum confirm [<prompt>]` | Option | Environment Variable | Description | | ------------------------------- | ------------------------------------ | ------------------------------------------------------------------- | | `--default` | - | Default confirmation action | | `--affirmative="Yes"` | - | The title of the affirmative action | | `--negative="No"` | - | The title of the negative action | | `--timeout=0` | `$GUM_CONFIRM_TIMEOUT` | Timeout until confirm returns selected value or default if provided | | `--prompt.foreground=""` | `$GUM_CONFIRM_PROMPT_FOREGROUND` | Foreground Color | | `--selected.foreground="230"` | `$GUM_CONFIRM_SELECTED_FOREGROUND` | Foreground Color | | `--unselected.foreground="254"` | `$GUM_CONFIRM_UNSELECTED_FOREGROUND` | Foreground Color | **Example**: Confirm whether to perform an action. Exits with code 0 (affirmative) or 1 (negative) depending on selection. ```shell gum confirm && rm file.txt || echo "File not removed" ``` ### gum file Pick a file from a folder Usage: `gum file [<path>]` | Option | Environment Variable | Description | | -------------------------------- | ---------------------------------- | ------------------------------------------------ | | `[<path>]` | `$GUM_FILE_PATH` | The path to the folder to begin traversing | | `-c, --cursor=">"` | `$GUM_FILE_CURSOR` | The cursor character | | `-a, --all` | `$GUM_FILE_ALL` | Show hidden and 'dot' files | | `--file` | `$GUM_FILE_FILE` | Allow files selection | | `--directory` | `$GUM_FILE_DIRECTORY` | Allow directories selection | | `--height=0` | `$GUM_FILE_HEIGHT` | Maximum number of files to display | | `--timeout=0` | `$GUM_FILE_TIMEOUT` | Timeout until command aborts without a selection | | `--cursor.foreground="212"` | `$GUM_FILE_CURSOR_FOREGROUND` | Foreground Color | | `--symlink.foreground="36"` | `$GUM_FILE_SYMLINK_FOREGROUND` | Foreground Color | | `--directory.foreground="99"` | `$GUM_FILE_DIRECTORY_FOREGROUND` | Foreground Color | | `--file.foreground="" ` | `$GUM_FILE_FILE_FOREGROUND` | Foreground Color | | `--permissions.foreground="244"` | `$GUM_FILE_PERMISSIONS_FOREGROUND` | Foreground Color | | `--selected.foreground="212"` | `$GUM_FILE_SELECTED_FOREGROUND` | Foreground Color | | `--file-size.foreground="240"` | `$GUM_FILE_FILE_SIZE_FOREGROUND` | Foreground Color | **Example**: Prompt the user to select a file from the file tree. ```shell EDITOR $(gum file $HOME) ``` ### gum filter Filter items from a list Usage: `gum filter [<options> ...]` | Option | Environment Variable | Description | | --------------------------------------- | ------------------------------------------ | ------------------------------------------------------------- | | `--indicator="•`" | `$GUM_FILTER_INDICATOR` | Character for selection | | `--selected-prefix=" ◉ "` | `$GUM_FILTER_SELECTED_PREFIX` | Character to indicate selected items (hidden if limit is 1) | | `--unselected-prefix=" ○ "` | `$GUM_FILTER_UNSELECTED_PREFIX` | Character to indicate unselected items (hidden if limit is 1) | | `--header=""` | `$GUM_FILTER_HEADER` | Header value | | `--placeholder="Filter..."` | `$GUM_FILTER_PLACEHOLDER` | Placeholder value | | `--prompt="> "` | `$GUM_FILTER_PROMPT` | Prompt to display | | `--width=20` | `$GUM_FILTER_WIDTH` | Input width | | `--height=0` | `$GUM_FILTER_HEIGHT` | Input height | | `--value=""` | `$GUM_FILTER_VALUE` | Initial filter value | | `--reverse` | `$GUM_FILTER_REVERSE` | Display from the bottom of the screen | | `--[no-]fuzzy` | `$GUM_FILTER_FUZZY` | Enable fuzzy matching | | `--[no-]sort` | `$GUM_FILTER_SORT` | Sort the results | | `--timeout=0` | `$GUM_FILTER_TIMEOUT` | Timeout until filter command aborts | | `--indicator.foreground="212"` | `$GUM_FILTER_INDICATOR_FOREGROUND` | Foreground Color | | `--selected-indicator.foreground="212"` | `$GUM_FILTER_SELECTED_PREFIX_FOREGROUND` | Foreground Color | | `--unselected-prefix.foreground="240"` | `$GUM_FILTER_UNSELECTED_PREFIX_FOREGROUND` | Foreground Color | | `--header.foreground="240"` | `$GUM_FILTER_HEADER_FOREGROUND` | Foreground Color | | `--text.foreground=""` | `$GUM_FILTER_TEXT_FOREGROUND` | Foreground Color | | `--cursor-text.foreground=""` | `$GUM_FILTER_CURSOR_TEXT_FOREGROUND` | Foreground Color | | `--match.foreground="212"` | `$GUM_FILTER_MATCH_FOREGROUND` | Foreground Color | | `--prompt.foreground="240"` | `$GUM_FILTER_PROMPT_FOREGROUND` | Foreground Color | | `--limit=1` | - | Maximum number of options to pick | | `--no-limit` | - | Pick unlimited number of options (ignores limit) | | `--select-if-one` | - | Select the given option if there is only one | | `--[no-]strict` | - | Only returns if anything matched. Otherwise return Filter | **Example**: Use fuzzy matching to filter a list of values: ```shell echo Strawberry >> flavors.txt echo Banana >> flavors.txt echo Cherry >> flavors.txt cat flavors.txt | gum filter > selection.txt ``` ### gum format Format a string using a template Usage: `gum format [<template> ...]` | Option | Environment Variable | Description | | ----------------------- | ---------------------- | ----------------------------------------------------------------------- | | `[<template> ...]` | - | Template string to format (can also be provided via stdin) | | `--theme="pink"` | `$GUM_FORMAT_THEME` | Glamour theme to use for [markdown](../../files/Markdown.md) formatting | | `-l, --language="" ` | `$GUM_FORMAT_LANGUAGE` | Programming language to parse code | | `-t, --type="markdown"` | `$GUM_FORMAT_TYPE` | Format to use (`markdown`, `template`, `code`, `emoji`) | **Example**: format processes and formats bodies of text. `gum format` can parse [markdown](../../files/Markdown.md), template strings, and named emojis. ```shell # Format some markdown gum format -- "# Gum Formats" "- Markdown" "- Code" "- Template" "- Emoji" echo "# Gum Formats\n- Markdown\n- Code\n- Template\n- Emoji" | gum format # Syntax highlight some code cat main.go | gum format -t code # Render text any way you want with templates echo '{{ Bold "Tasty" }} {{ Italic "Bubble" }} {{ Color "99" "0" " Gum " }}' \ | gum format -t template # Display your favorite emojis! echo 'I :heart: Bubble Gum :candy:' | gum format -t emoji ``` ### gum input Prompt for some input Usage: `gum input` | Option | Environment Variable | Description | | ----------------------------------- | ------------------------------ | -------------------------------------------- | | `--placeholder="Type something..."` | `$GUM_INPUT_PLACEHOLDER` | Placeholder value | | `--prompt="> "` | `$GUM_INPUT_PROMPT` | Prompt to display | | `--cursor.mode="blink"` | `$GUM_INPUT_CURSOR_MODE` | Cursor mode | | `--value=""` | - | Initial value (can also be passed via stdin) | | `--char-limit=400` | - | Maximum value length (0 for no limit) | | `--width=40` | `$GUM_INPUT_WIDTH` | Input width (0 for terminal width) | | `--password` | - | Mask input characters | | `--header=""` | `$GUM_INPUT_HEADER` | Header value | | `--timeout=0` | `$GUM_INPUT_TIMEOUT` | Timeout until input aborts | | `--prompt.foreground=""` | `$GUM_INPUT_PROMPT_FOREGROUND` | Foreground Color | | `--cursor.foreground="212"` | `$GUM_INPUT_CURSOR_FOREGROUND` | Foreground Color | | `--header.foreground="240"` | `$GUM_INPUT_HEADER_FOREGROUND` | Foreground Color | **Example**: Prompt for input with a simple command. ```shell gum input > answer.txt ``` Prompt for sensitive input with the --password flag. ```shell gum input --password > password.txt ``` ### gum join Join text vertically or horizontally Usage: `gum join <text> ...` | Option | Description | | ---------------- | -------------------------------------------------- | | `--align="left"` | Text alignment | | `--horizontal` | Join (potentially multi-line) strings horizontally | | `--vertical` | Join (potentially multi-line) strings vertically | **Example**: Combine text vertically or horizontally. Use this command with `gum style` to build layouts and pretty output. > Tip: Always wrap the output of `gum style` in quotes to preserve newlines (`\n`) when using it as an argument in the join command. ```shell I=$(gum style --padding "1 5" --border double --border-foreground 212 "I") LOVE=$(gum style --padding "1 4" --border double --border-foreground 57 "LOVE") BUBBLE=$(gum style --padding "1 8" --border double --border-foreground 255 "Bubble") GUM=$(gum style --padding "1 5" --border double --border-foreground 240 "Gum") I_LOVE=$(gum join "$I" "$LOVE") BUBBLE_GUM=$(gum join "$BUBBLE" "$GUM") gum join --align center --vertical "$I_LOVE" "$BUBBLE_GUM" ``` ### gum pager Scroll through a file Usage: `gum pager [<content>]` | Option | Environment Variable | Description | | ------------------------------------ | ----------------------------------- | --------------------------- | | `--show-line-numbers` | - | Show line numbers | | `--soft-wrap` | - | Soft wrap lines | | `--timeout=0` | `$GUM_PAGER_TIMEOUT` | Timeout until command exits | | `--foreground=""` | `$GUM_PAGER_FOREGROUND` | Foreground Color | | `--help.foreground="241"` | `$GUM_PAGER_HELP_FOREGROUND` | Foreground Color | | `--line-number.foreground="237"` | `$GUM_PAGER_LINE_NUMBER_FOREGROUND` | Foreground Color | | `--match.foreground="212"` | `$GUM_PAGER_MATCH_FOREGROUND` | Foreground Color | | `--match-highlight.foreground="235"` | `$GUM_PAGER_MATCH_HIGH_FOREGROUND` | Foreground Color | **Example**: Scroll through a long document with line numbers and a fully customizable viewport. ```shell gum pager < README.md ``` ### gum spin Display spinner while running a command Usage: `gum spin <command> ...` | Option | Environment Variable | Description | | ---------------------------- | ------------------------------ | ---------------------------------------------------------------------------------------------------------------------------------------------------- | | `--show-output` | `$GUM_SPIN_SHOW_OUTPUT` | Show or pipe output of command during execution | | `-s, --spinner="dot"` | `$GUM_SPIN_SPINNER` | Spinner type. Available spinner types include: `line`, `dot`, `minidot`, `jump`, `pulse`, `points`, `globe`, `moon`, `monkey`, `meter`, `hamburger`. | | `--title="Loading..."` | `$GUM_SPIN_TITLE` | Text to display to user while spinning | | `-a, --align="left"` | `$GUM_SPIN_ALIGN` | Alignment of spinner with regard to the title | | `--timeout=0` | `$GUM_SPIN_TIMEOUT` | Timeout until spin command aborts | | `--spinner.foreground="212"` | `$GUM_SPIN_SPINNER_FOREGROUND` | Foreground Color | | `--title.foreground=""` | `$GUM_SPIN_TITLE_FOREGROUND` | Foreground Color | **Example**: To view or pipe the command's output, use the `--show-output` flag. ```shell gum spin --spinner dot --title "Buying Bubble Gum..." -- sleep 5 ``` ### gum style Apply coloring, borders, spacing to text Usage: `gum style [<text> ...]` | Option | Environment Variable | Description | | ------------------------ | -------------------- | ----------------------- | | `--foreground=""` | `$FOREGROUND` | Foreground Color | | `--background=""` | `$BACKGROUND` | Background Color | | `--border="none"` | `$BORDER` | Border Style | | `--border-background=""` | `$BORDER_BACKGROUND` | Border Background Color | | `--border-foreground=""` | `$BORDER_FOREGROUND` | Border Foreground Color | | `--align="left"` | `$ALIGN` | Text Alignment | | `--height=0` | `$HEIGHT` | Text height | | `--width=0` | `$WIDTH` | Text width | | `--margin="0 0"` | `$MARGIN` | Text margin | | `--padding="0 0"` | `$PADDING` | Text padding | | `--bold` | `$BOLD` | Bold text | | `--faint` | `$FAINT` | Faint text | | `--italic` | `$ITALIC` | Italicize text | | `--strikethrough` | `$STRIKETHROUGH` | Strikethrough text | | `--underline` | `$UNDERLINE` | Underline text | **Example**: Pretty print any string with any layout with one command. ```shell gum style \ --foreground 212 --border-foreground 212 --border double \ --align center --width 50 --margin "1 2" --padding "2 4" \ 'Bubble Gum (1¢)' 'So sweet and so fresh!' ``` ### gum table Render a table of data Usage: `gum table` | Option | Environment Variable | Description | | ----------------------------- | -------------------------------- | ---------------- | | `-s, --separator=","` | - | Row separator | | `-c, --columns=COLUMNS,...` | - | Column names | | `-w, --widths=WIDTHS,...` | - | Column widths | | `--height=10` | - | Table height | | `-p, --print` | - | static print | | `-f, --file=""` | - | file path | | `-b, --border="rounded"` | - | border style | | `--border.foreground=""` | `$GUM_TABLE_BORDER_FOREGROUND` | Foreground Color | | `--cell.foreground=""` | `$GUM_TABLE_CELL_FOREGROUND` | Foreground Color | | `--header.foreground=""` | `$GUM_TABLE_HEADER_FOREGROUND` | Foreground Color | | `--selected.foreground="212"` | `$GUM_TABLE_SELECTED_FOREGROUND` | Foreground Color | **Example**: Select a row from some tabular data. ```shell gum table < flavors.csv | cut -d ',' -f 1 ``` ### gum write Prompt for long-form text Usage: `gum write` | Option | Environment Variable | Description | | ------------------------------------- | ------------------------------------------ | --------------------------------------- | | `--width=50` | `$GUM_WRITE_WIDTH` | Text area width (0 for terminal width) | | `--height=5` | `$GUM_WRITE_HEIGHT` | Text area height | | `--header=""` | `$GUM_WRITE_HEADER` | Header value | | `--placeholder="Write something..."` | `$GUM_WRITE_PLACEHOLDER` | Placeholder value | | `--prompt="┃ "` | `$GUM_WRITE_PROMPT` | Prompt to display | | `--show-cursor-line` | `$GUM_WRITE_SHOW_CURSOR_LINE` | Show cursor line | | `--show-line-numbers` | `$GUM_WRITE_SHOW_LINE_NUMBERS` | Show line numbers | | `--value=""` | `$GUM_WRITE_VALUE` | Initial value (can be passed via stdin) | | `--char-limit=400` | - | Maximum value length (0 for no limit) | | `--cursor.mode="blink"` | `$GUM_WRITE_CURSOR_MODE` | Cursor mode | | `--base.foreground=""` | `$GUM_WRITE_BASE_FOREGROUND` | Foreground Color | | `--cursor-line-number.foreground="7"` | `$GUM_WRITE_CURSOR_LINE_NUMBER_FOREGROUND` | Foreground Color | | `--cursor-line.foreground=""` | `$GUM_WRITE_CURSOR_LINE_FOREGROUND` | Foreground Color | | `--cursor.foreground="212"` | `$GUM_WRITE_CURSOR_FOREGROUND` | Foreground Color | | `--end-of-buffer.foreground="0"` | `$GUM_WRITE_END_OF_BUFFER_FOREGROUND` | Foreground Color | | `--line-number.foreground="7"` | `$GUM_WRITE_LINE_NUMBER_FOREGROUND` | Foreground Color | | `--header.foreground="240"` | `$GUM_WRITE_HEADER_FOREGROUND` | Foreground Color | | `--placeholder.foreground="240"` | `$GUM_WRITE_PLACEHOLDER_FOREGROUND` | Foreground Color | | `--prompt.foreground="7"` | `$GUM_WRITE_PROMPT_FOREGROUND` | Foreground Color | **Example**: > Note: `CTRL+D` is used to complete text entry. `CTRL+C` and `esc` will cancel. ```shell gum write > story.txt ``` ### gum log [Log](../../dev/Log.md) messages to output Usage: `gum log <text> ...` | Option | Environment Variable | Description | | --------------------------- | ------------------------------- | ----------------------------------------------------------------------- | | `-o, --file=STRING` | - | Log to file | | `-f, --format` | - | Format message using printf | | `--formatter="text"` | - | The log formatter to use | | `-l, --level="none"` | - | The log level to use | | `--prefix=STRING` | - | Prefix to print before the message | | `-s, --structured` | - | Use structured logging | | `-t, --time=""` | - | The time format to use (`kitchen`, `layout`, `ansic`, `rfc822`, etc...) | | `--level.foreground=""` | `$GUM_LOG_LEVEL_FOREGROUND` | Foreground Color | | `--time.foreground=""` | `$GUM_LOG_TIME_FOREGROUND` | Foreground Color | | `--prefix.foreground=""` | `$GUM_LOG_PREFIX_FOREGROUND` | Foreground Color | | `--message.foreground=""` | `$GUM_LOG_MESSAGE_FOREGROUND` | Foreground Color | | `--key.foreground=""` | `$GUM_LOG_KEY_FOREGROUND` | Foreground Color | | `--value.foreground=""` | `$GUM_LOG_VALUE_FOREGROUND` | Foreground Color | | `--separator.foreground=""` | `$GUM_LOG_SEPARATOR_FOREGROUND` | Foreground Color | **Example**: `log` logs messages to the terminal at using different levels and styling using the charmbracelet/log library. ```shell # Log some debug information. gum log --structured --level debug "Creating file..." name file.txt # DEBUG Unable to create file. name=temp.txt # Log some error. gum log --structured --level error "Unable to create file." name file.txt # ERROR Unable to create file. name=temp.txt # Include a timestamp. gum log --time rfc822 --level error "Unable to create file." ``` ## Examples ### Connect to a [TMUX](tmux.md) session Pick from a running [tmux](tmux.md) session and attach to it. Or, if you're already in a [tmux](tmux.md) session, switch sessions. ```shell SESSION=$(tmux list-sessions -F \#S | gum filter --placeholder "Pick session...") tmux switch-client -t $SESSION || tmux attach -t $SESSION ``` ### Pick commit hash from your [Git](../../dev/Git.md) history Filter through your [git](../../dev/Git.md) history searching for commit messages, copying the commit hash of the commit you select. ```shell git log --oneline | gum filter | cut -d' ' -f1 # | copy ``` ### Choose packages to uninstall List all packages installed by your package manager (we'll use [brew](../package%20managers/Brew.md)) and choose which packages to uninstall. ```shell brew list | gum choose --no-limit | xargs brew uninstall ``` ### Choose branches to delete List all branches and choose which branches to delete. ```shell git branch | cut -c 3- | gum choose --no-limit | xargs git branch -D ```